How to Isolate Python Package Management with pipx
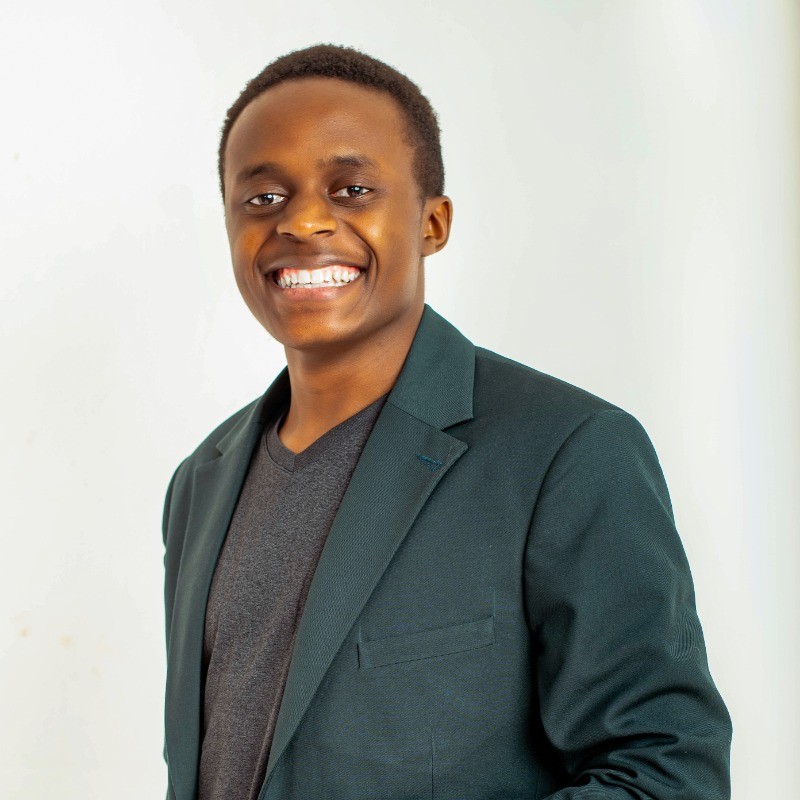
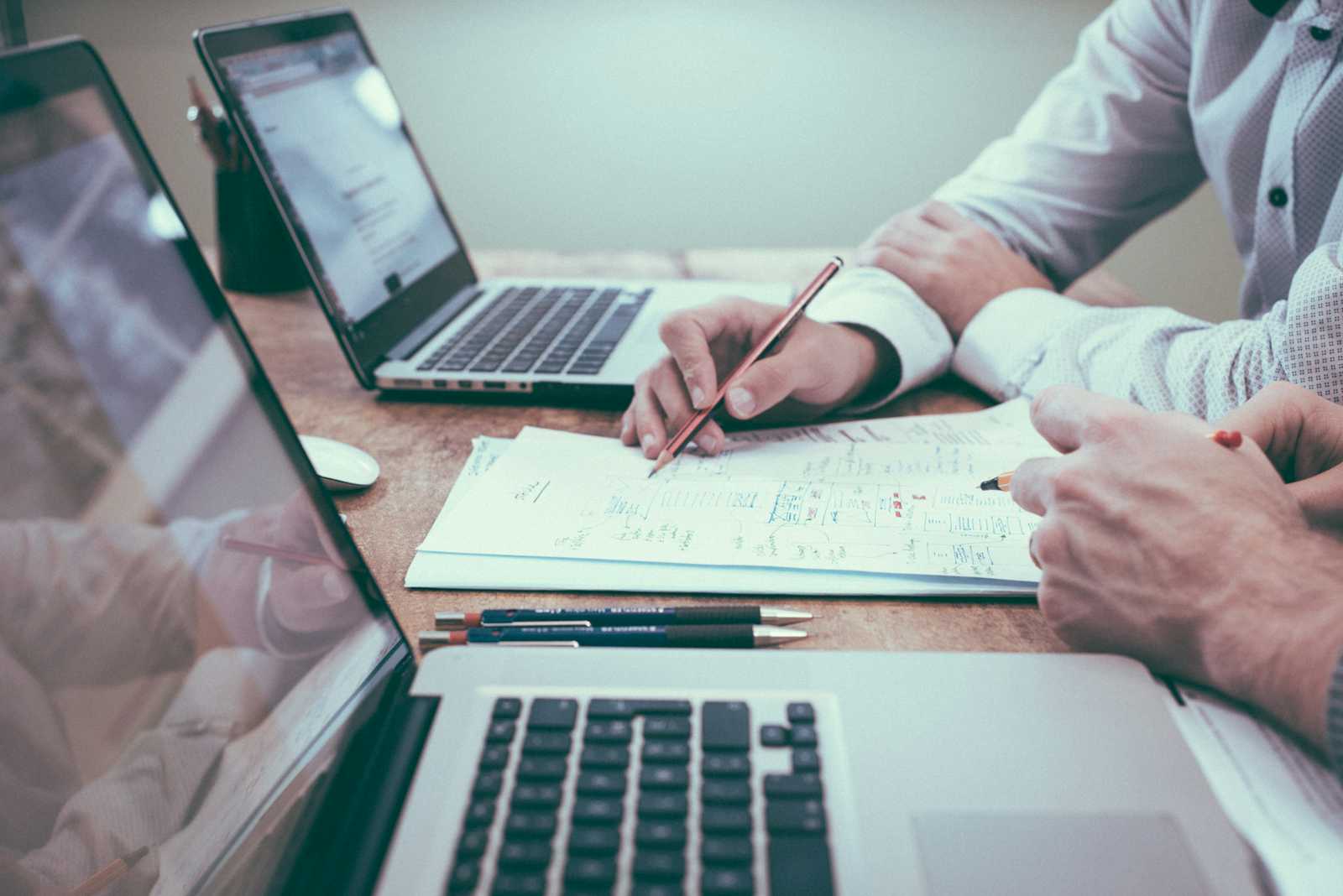
Python has a vast ecosystem of command-line tools, but installing these globally with pip
can lead to version conflicts and dependency issues. pipx
offers a modern solution by enabling global installations in isolated environments. In this guide, we'll dive deep into pipx
, its features, installation, usage, and best practices to leverage its full potential.
What is pipx
?
pipx
is a command-line tool that installs Python applications in isolated virtual environments, allowing you to run them globally without risking conflicts in your system’s Python environment. Each tool installed via pipx
has its own environment, meaning dependencies are contained and don't interfere with other applications. Launched in 2018, pipx
has become a popular choice for managing command-line applications like django
, httpie
, and black
.
Why Use pipx
?
Traditional package managers like pip
install Python applications and libraries system-wide or in virtual environments, which can lead to dependency issues or version conflicts. With pipx
, you can:
Install tools globally without conflicts: Each tool is installed in its own virtual environment.
Access tools globally from any directory: Unlike virtual environments, you don’t need to activate anything to use a tool installed with
pipx
.Easily update, uninstall, and manage tools: Keep tools up-to-date or remove them without affecting other packages or the system Python.
Getting Started with pipx
Here’s how to install, configure, and start using pipx
.
Step 1: Install pipx
To install pipx
, run:
python3 -m pip install --user pipx
python3 -m pipx ensurepath
Note: The
ensurepath
command adds thepipx
installation directory to your system's PATH, enabling you to runpipx
commands from any location.
Once installed, you can confirm with:
pipx --version
Step 2: Installing Python Applications with pipx
The pipx
syntax is straightforward for installing, running, and managing packages. Let’s walk through common commands:
Installing a Tool
To install a tool globally, use:
pipx install <tool_name>
For example, to install httpie
(a command-line HTTP client):
pipx install httpie
This command creates an isolated environment for httpie
and makes it globally accessible.
Running a Tool Without Installation
If you want to try a tool without installing it permanently, pipx
offers a convenient run
command:
pipx run <tool_name>
Example:
pipx run cowsay Hello, pipx!
This downloads and executes the command without adding it permanently to your system.
Listing Installed Packages
To view all packages installed with pipx
, run:
pipx list
This command shows each package’s path, version, and other details.
Step 3: Updating and Uninstalling Tools
Managing tools with pipx
is simple and won’t impact other applications or your system’s Python environment.
Updating a Tool
To update a tool to the latest version, use:
pipx upgrade <tool_name>
Or, to upgrade all installed tools:
pipx upgrade-all
Uninstalling a Tool
To remove a tool and its virtual environment:
pipx uninstall <tool_name>
Example:
pipx uninstall httpie
Reinstalling All Tools
If you need to refresh all installations, perhaps after a Python version upgrade:
pipx reinstall-all
Step 4: Using pipx run
for Temporary Environments
The pipx run
command is useful for running one-off commands without committing to a full installation. For instance, you can use it to run a script or CLI tool without leaving it installed on your system. Here’s how:
pipx run black my_script.py
This command will temporarily install black
, execute it on my_
script.py
, and then remove the installation, keeping your environment clean.
Advanced pipx
Commands and Features
Beyond basic installs, pipx
has more capabilities to help with nuanced package management.
Specifying a Python Version
By default, pipx
uses the Python version it was installed with, but you can specify a different Python version for each tool. This is handy if a tool requires a specific Python version:
pipx install <tool_name> --python <path_to_python_version>
Example:
pipx install poetry --python /usr/local/bin/python3.8
Installing a Package in Development Mode
If you’re developing a Python tool and want to test it with pipx
, you can install it in editable mode. Just navigate to the package directory and run:
pipx install --editable .
This command installs the package from your local directory, allowing you to test changes without reinstalling each time.
Injecting Extra Dependencies
Sometimes, a tool might need additional packages. With pipx
, you can inject these without modifying the main package:
pipx inject <tool_name> <extra_package>
For example:
pipx inject jupyterlab matplotlib
This installs matplotlib
into the isolated environment created for jupyterlab
.
Real-World Examples with pipx
To highlight pipx
’s flexibility, let’s look at a few practical applications.
Example 1: Install and Use black
for Code Formatting
Install
black
withpipx
:pipx install black
Format a Python file:
black script.py
Later, if you don’t need
black
anymore:pipx uninstall black
Example 2: Use httpie
for Quick HTTP Requests
httpie
simplifies HTTP requests. Install and make a request:
pipx install httpie
http https://jsonplaceholder.typicode.com/posts/1
Example 3: Running Temporary Scripts with pipx run
You can use pipx run
to try new tools or run scripts that you don’t plan to keep permanently. For example:
pipx run flake8 script.py
Best Practices for Using pipx
Use
pipx
for CLI Tools:pipx
is optimized for command-line applications, not libraries. Use it to install tools you need to call from the terminal.Isolate Each Tool: With
pipx
, each tool has its environment. Don’t worry about dependencies or conflicts between tools.Update Regularly: Use
pipx upgrade-all
to keep your tools updated.Avoid System Interference: Because each tool is in its own environment,
pipx
minimizes risk to system stability.
Troubleshooting Common Issues
Command Not Found: Ensure
pipx
's path is added to your shell’s PATH variable by runningpipx ensurepath
again or restarting your shell.Permission Errors: If you encounter permission issues, verify that
pipx
was installed for your user (not globally) and that the paths are correct.Package Compatibility: Occasionally, some packages may not work as expected in isolated environments due to system dependencies. Check the package’s documentation for requirements.
Conclusion
pipx
brings the best of both worlds: global access to Python applications with the isolation of virtual environments. It provides a secure, hassle-free way to manage CLI tools and avoid dependency conflicts, perfect for Python users who frequently rely on command-line applications.
Subscribe to my newsletter
Read articles from Bonaventure Ogeto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
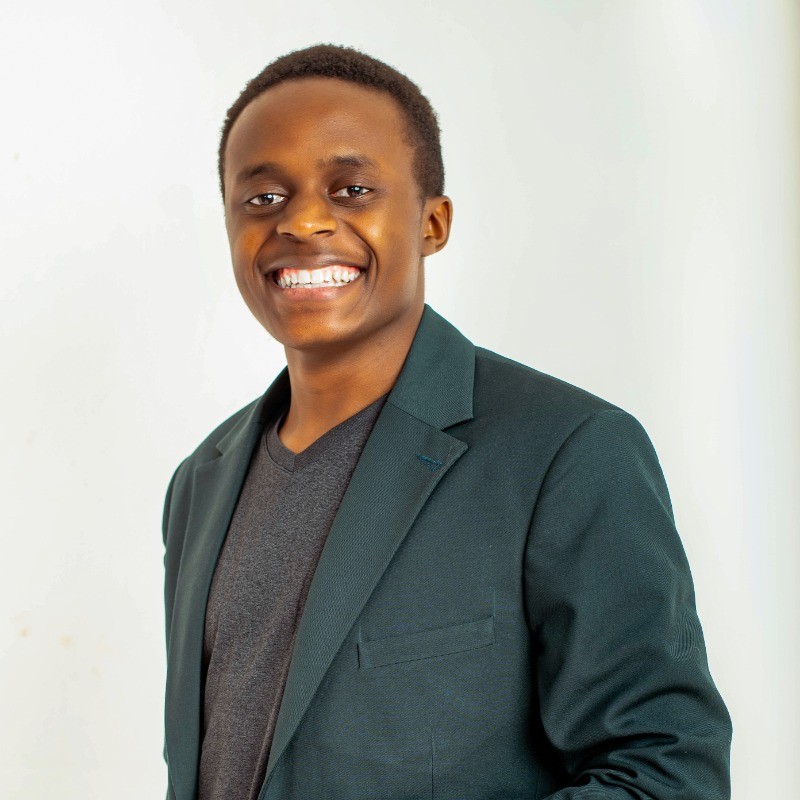
Bonaventure Ogeto
Bonaventure Ogeto
Software Engineer & Technical Writer