Understanding Basic Java Code Structure
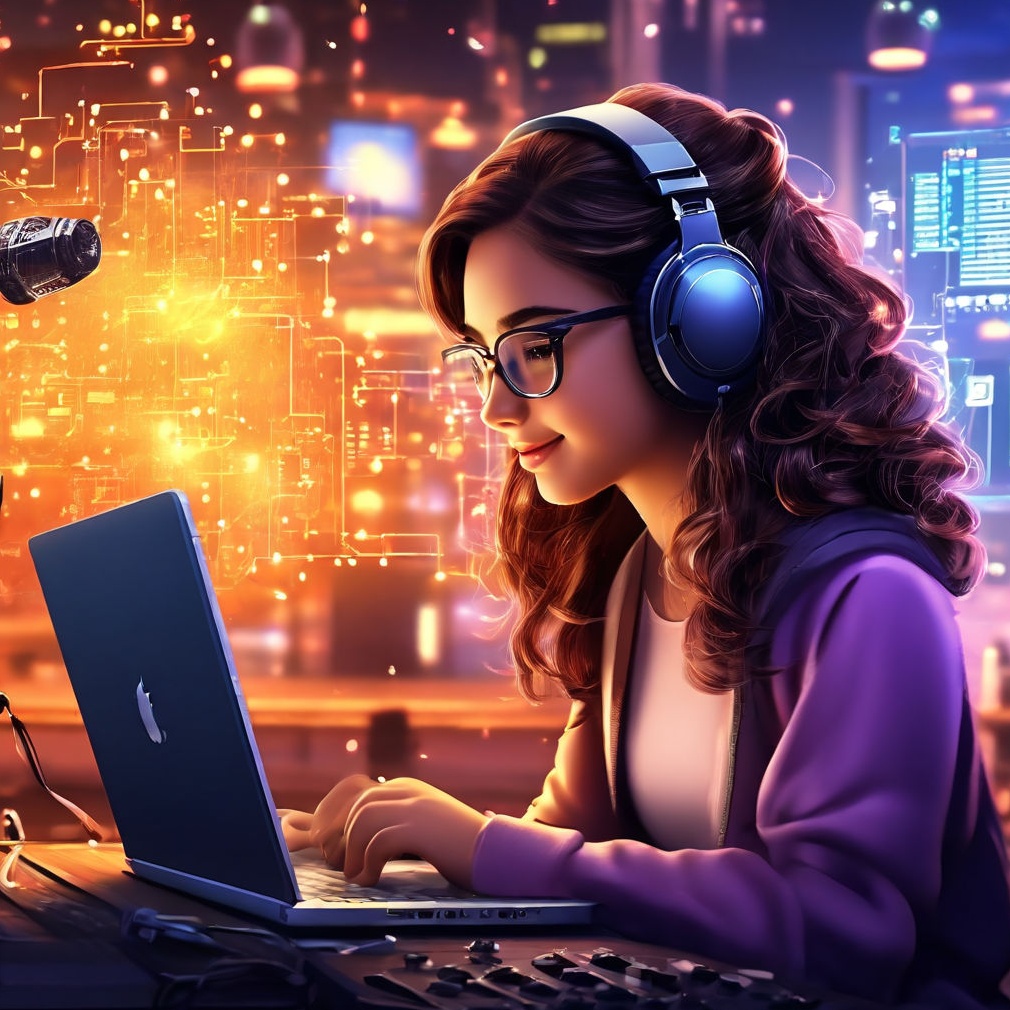
Java:
Java is a versatile and powerful programming language that has become a cornerstone in the world of software development. Known for its object-oriented structure, Java enables developers to create robust, secure, and platform-independent applications. Its "write once, run anywhere" capability allows Java programs to run on any device equipped with a Java Virtual Machine (JVM), making it a popular choice for web applications, mobile apps, and enterprise solutions. With a rich set of libraries and a strong community, Java continues to evolve, offering developers the tools they need to build innovative and efficient software solutions.
Java Structure:
- Function:
void main(){
......
}
or
returnValue main(){
......
}
In Java, the main method is defined as the entry point of any standalone application. The method is written as void main(String[] args)
. Inside the parentheses ()
, you can specify parameters, which in this case is an array of String
objects named args
. This array allows the program to accept command-line arguments when it runs. Following the method declaration, you use curly braces {}
to define the body of the method. Inside these curly braces, you write the code that performs the specific tasks or operations you want the program to execute. This structure is crucial because it organizes the code and ensures that the Java Virtual Machine (JVM) knows where to begin executing the program.
- classes:
class Main {
static void main(String[] args) {
// Your code here
}
}
In Java, a class defines the structure and behavior of objects, and within a class, you can define functions known as methods. These methods are written inside curly braces {}. The method encapsulates a specific set of instructions or operations that the program can perform. When you write a method within a class, it becomes a part of that class and can be used to manipulate the data or perform actions related to the objects created from the class. This organization helps in maintaining a clean and modular code structure, allowing developers to easily manage and expand their applications.
Access Modifier:
public class Main { public static void main(String[] args) { // Your code here } } or private class Main { private static void main(String[] args) { // Your code here } }
In Java, access modifiers are keywords that set the accessibility of classes, methods, and other members. The two most common access modifiers are
public
andprivate
.Public:
The
public
access modifier allows the class, method, or variable to be accessible from any other class in any package. It provides the widest level of accessibility.When a member is declared as
public
, it can be accessed from outside the class, making it visible to all other classes.
Private:
The
private
access modifier restricts the visibility of the class, method, or variable to within the class itself. It is the most restrictive access level.Members declared as
private
cannot be accessed directly from outside the class. This is useful for encapsulating the internal state and behavior of a class, allowing control over how data is accessed or modified.
First Code:
public class firstCode{
public static void main(String[] args){
System.out.println("Hello World");
}
}
The class in this example is declared with the public access modifier, which means it can be accessed from any other class in any package. The class is named firstCode
, and it contains a main
method, which serves as the entry point for the program. Within the main
method, there is a simple line of code that uses System.out.println
to print the string "Hello World" to the console. This basic code demonstrates how to output text in Java and serves as a foundational example for understanding how public classes and methods operate within the Java programming language.
Follow for more Java Learings!
Happy Coding!
Subscribe to my newsletter
Read articles from VaithegiV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
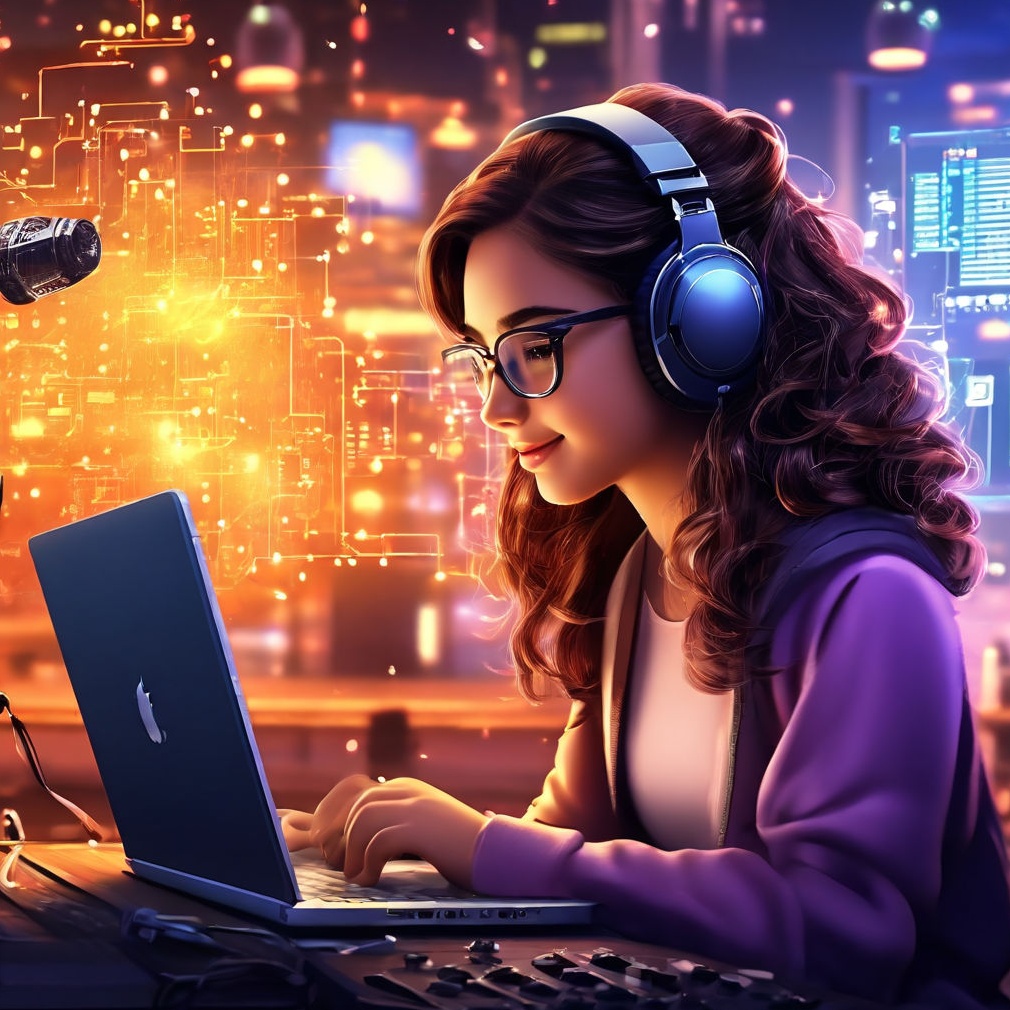
VaithegiV
VaithegiV
Hi, I'm VaithegiV Frontend developer. B.Tech graduate with a passion for web development. Proficient in HTML, CSS, and JavaScript, I strive to create intuitive and visually appealing digital experiences. With expertise in Git and GitHub, I ensure efficient collaboration and version control in projects. My dedication to continuous learning drives me to stay updated with the latest trends in technology and design. I am eager to contribute my skills and creativity to innovative projects that push boundaries and deliver exceptional user experiences.