Building a Streamlit App to Query MindsDB for Research Papers
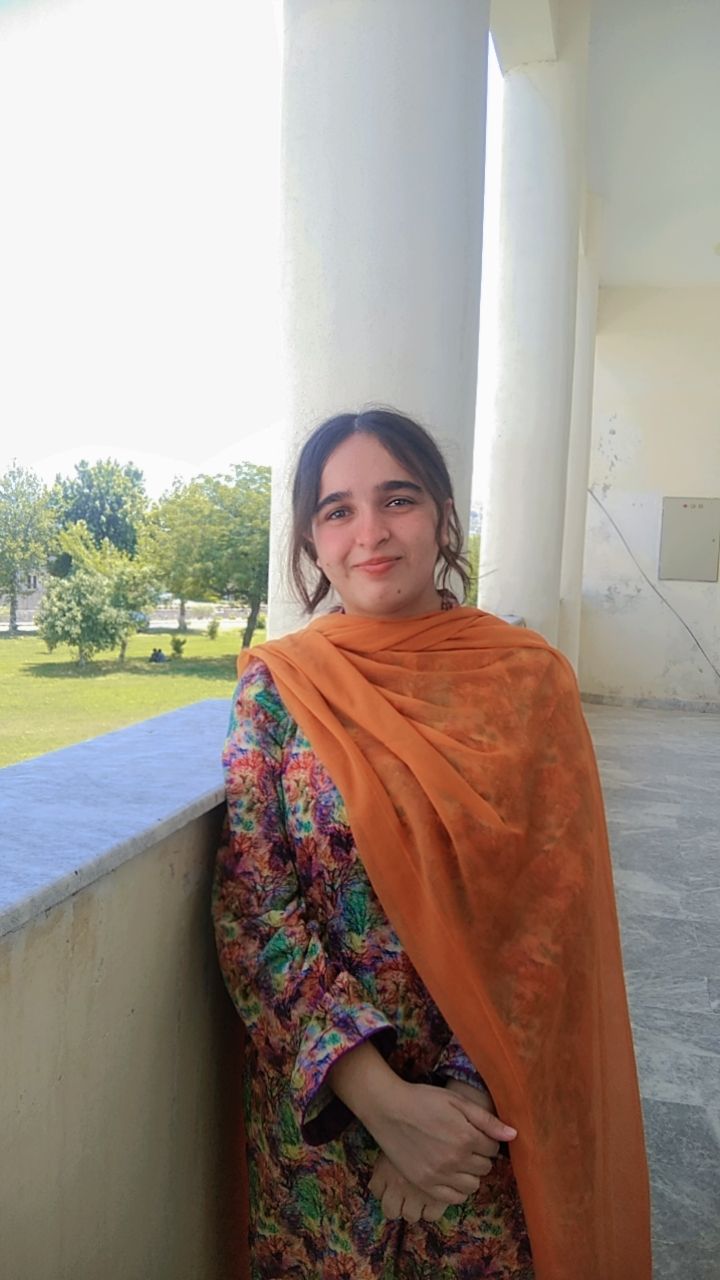
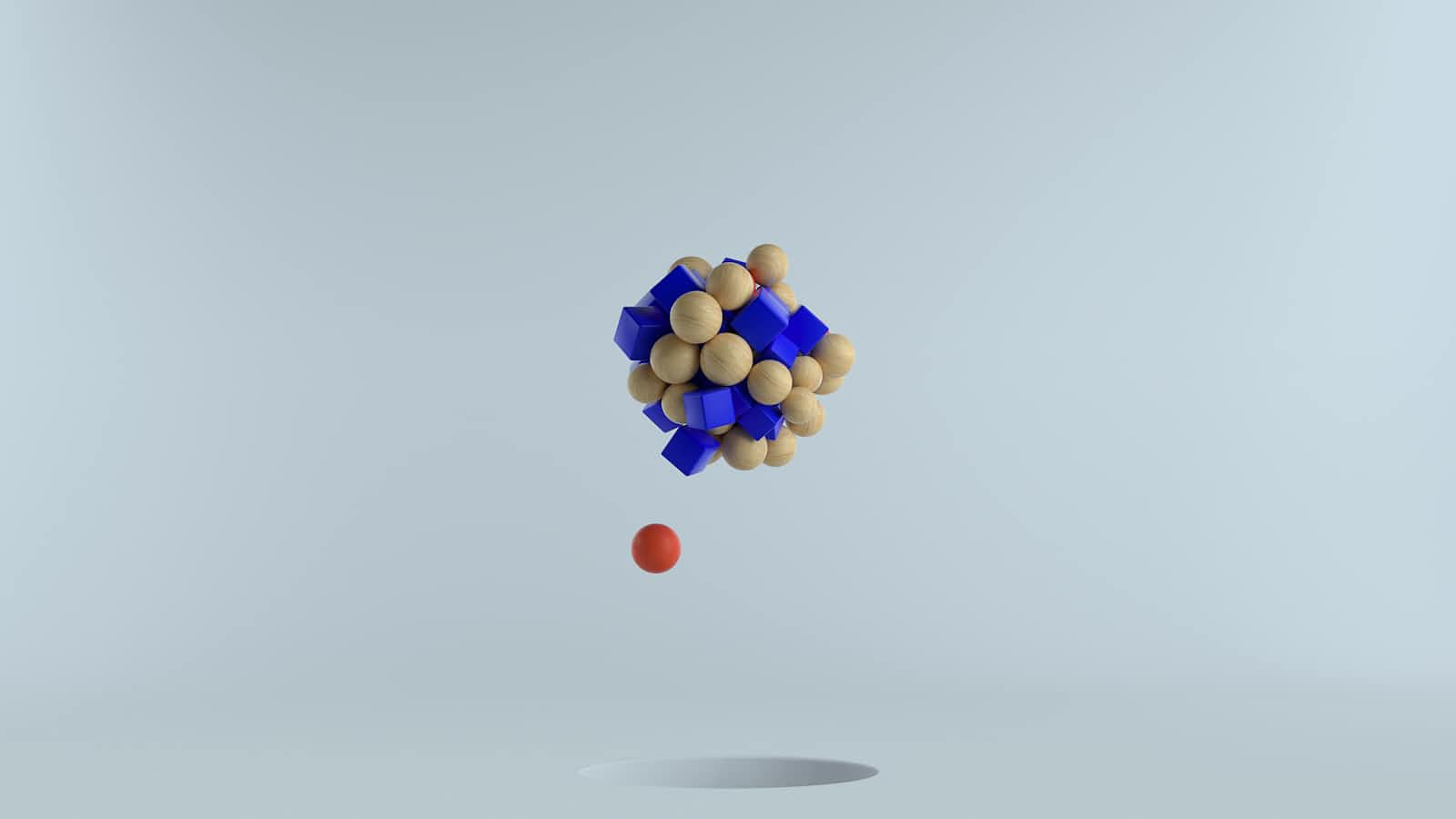
I wanted an easy way to analyze all the papers presented at CVPR 2024—so I built an app to streamline this process! In this guide, I'll walk you through creating a Streamlit app that uses MindsDB (via the OpenAI SDK) to query a custom Mind I created. This app allows users to ask questions related to CVPR 2024 research papers and receive answers, with the final app hosted on Hugging Face Spaces.
Step 1: Setting Up MindsDB and Your Mind
What is a AI-Data Mind ?
An AI-Data Mind is like an LLM that answers questions and engages in conversations using data from your database. Creating a Mind is simple: you just configure how it accesses the database, and then you can interact with it through OpenAI's standard Completions or Assistants API.
Create Your Mind on MindsDB
Start by signing up or logging into MindsDB.
Create a new Mind using MindsDB’s documentation, setting it up with relevant data from CVPR 2024, like keywords, summaries, and other important details.
Write name of mind as CVPR_Data.
Now we have to connect a data source with it. Click on PostgreSQL.
For hosting the database I used Supabase, click on new project and then click on Table Editor,
You will get an option to create table, you can upload csv or excel file too.
Now you just need to go to settings, select database from side bar. and then note these details as they will be used to connect data source to the mind.
Enter the database connection details in the minds options.
Now you can even test your mind here before integrating it in yoru own application.
Configure API Access
- In MindsDB, generate an API key—we’ll use it later to connect our Streamlit app to MindsDB.
Step 2: Setting Up Your Environment
For this project, you’ll need:
Python (version 3.7 or above)
OpenAI Python SDK: This SDK allows us to interact with MindsDB seamlessly.
Step 3: Write the Streamlit App Code
Create a file named app.py
and add the following code. This app will prompt users to enter a query about CVPR 2024, send the query to MindsDB, and display the response in Streamlit.
import streamlit as st
from openai import OpenAI
import os
# Set up MindsDB API key and Mind name
api_key = os.getenv("MINDS_API_KEY") # Set this environment variable on Hugging Face
mind_name = "<NAME_YOU_GAVE_TO_YOUR_MIND>"
# Initialize the OpenAI SDK for MindsDB
client = OpenAI(api_key=api_key, base_url='https://mdb.ai/')
st.title("CVPR Insight Hub")
st.write("Query the database for information related to CVPR 2024 research papers, including keywords and summaries.")
# User input for querying research papers
query = st.text_input("Enter your query about CVPR 2024 (e.g., 'keywords in CVPR 2024 papers'):")
if st.button("Search"):
if query:
st.write("Answering the question may take up to 30 seconds...")
try:
# Chat with the Mind (non-streamed, single output)
completion = client.chat.completions.create(
model=mind_name,
messages=[
{'role': 'user', 'content': query}
],
stream=False # Disable streaming for a single, final output
)
# Display the single answer from the Mind
st.write(completion.choices[0].message.content)
except Exception as e:
st.error(f"Error: {e}")
else:
st.warning("Please enter a query.")
Explanation of the Code
API Key: The
MINDS_API_KEY
is securely set as an environment variable.Query Input: Users enter questions related to CVPR 2024, and the app sends them to MindsDB upon pressing "Search."
Completion Call: Using
client.chat.completions.create
withstream=False
, we receive only the final answer as output.Display Result: The response is displayed in Streamlit.
Step 4: Creating requirements.txt
To run the app on Hugging Face Spaces, we need a requirements.txt
file with dependencies:
streamlit
openai
Step 5: Deploying on Hugging Face Spaces
Hugging Face Spaces is a great platform to host Streamlit apps for free.
Create a New Space
- Go to Hugging Face Spaces and create a new Space. Set it to “Public” or “Private” and choose “Streamlit” as the SDK.
Upload Files
- Upload
app.py
andrequirements.txt
to the root of the Space.
Set Environment Variables
- Add your MindsDB API key as a secret environment variable named MINDS_API_KEY in Hugging Face’s Settings tab.
Run the App
- Click Deploy once everything is uploaded. Your app should be live in a few minutes.
Step 6: Using the App
Once the app is live:
Enter a question related to CVPR 2024 in the text input field (e.g., “What are the top keywords in CVPR 2024 research papers?”).
Click “Search” to query MindsDB, and see the results displayed neatly in Streamlit.
Conclusion
Congratulations! You've built and deployed an app that allows users to query MindsDB for information on CVPR 2024 research topics. This guide has shown you how to create a functional query app, deploy it, and use environment variables for secure API key handling.
Subscribe to my newsletter
Read articles from Amna Hassan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
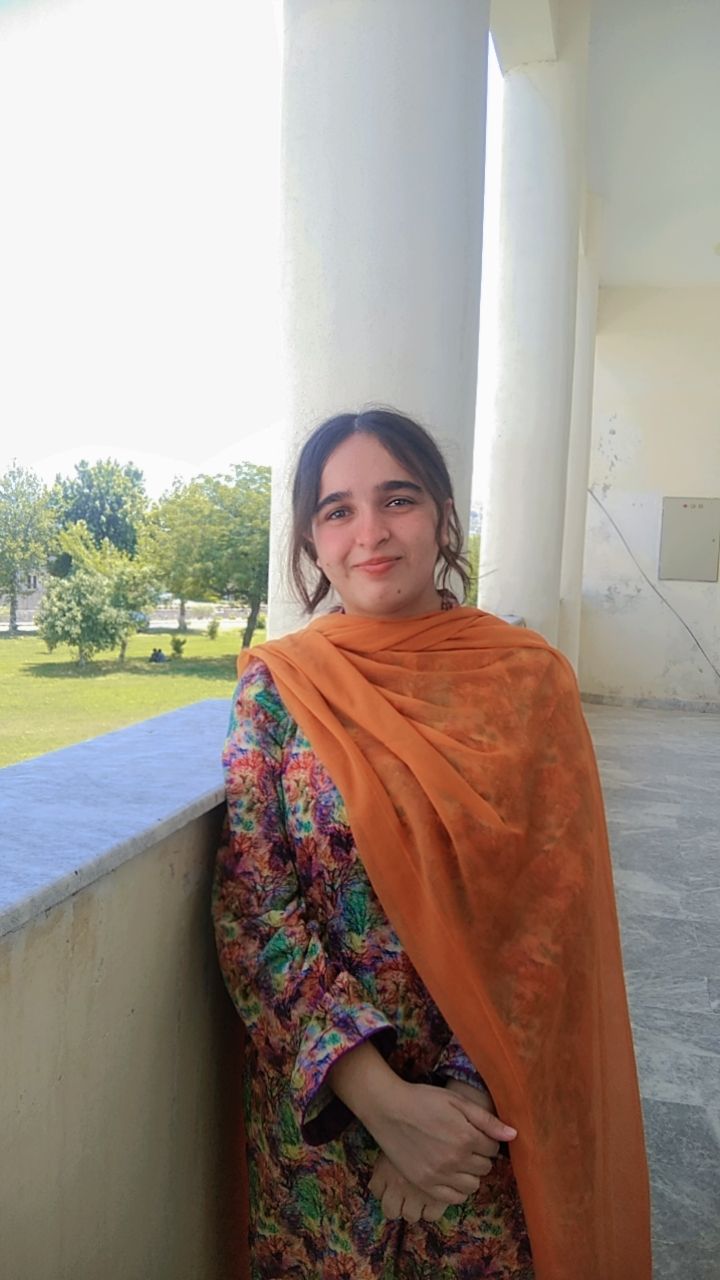
Amna Hassan
Amna Hassan
As the Section Leader for Stanford's Code In Place and a winner of the 2024 CS50 Puzzle Day at Harvard, I bring a passion for coding and problem-solving to every project I undertake. My achievements include winning a hackathon at LabLab.ai and participating in nine international hackathons, showcasing my dedication to continuous learning and innovation. As the Women in Tech Lead for GDSC at UET Taxila, I advocate for diversity and empowerment in the tech industry. With a strong background in game development, web development, and AI, I am committed to leveraging technology to create impactful solutions and drive positive change.