Day 27 Jenkins Declarative Pipeline with Docker

Table of contents
- 🛠️ What You’ll Need
- 🏗️ Task 1: Create a Docker-Integrated Jenkins Declarative Pipeline
- 🛠️ Task 2: Use Docker Groovy Syntax to Avoid Errors
- 🌐 Step 1: Create a Simple Node.js Application
- 📑 Step 2: Jenkins Declarative Pipeline with Shell Commands (Task 1)
- 🔄 Step 3: Using Docker Groovy Syntax to Avoid Conflicts (Task 2)
- 🧹 Step 4: Clean Up Containers to Save Resources (Optional)
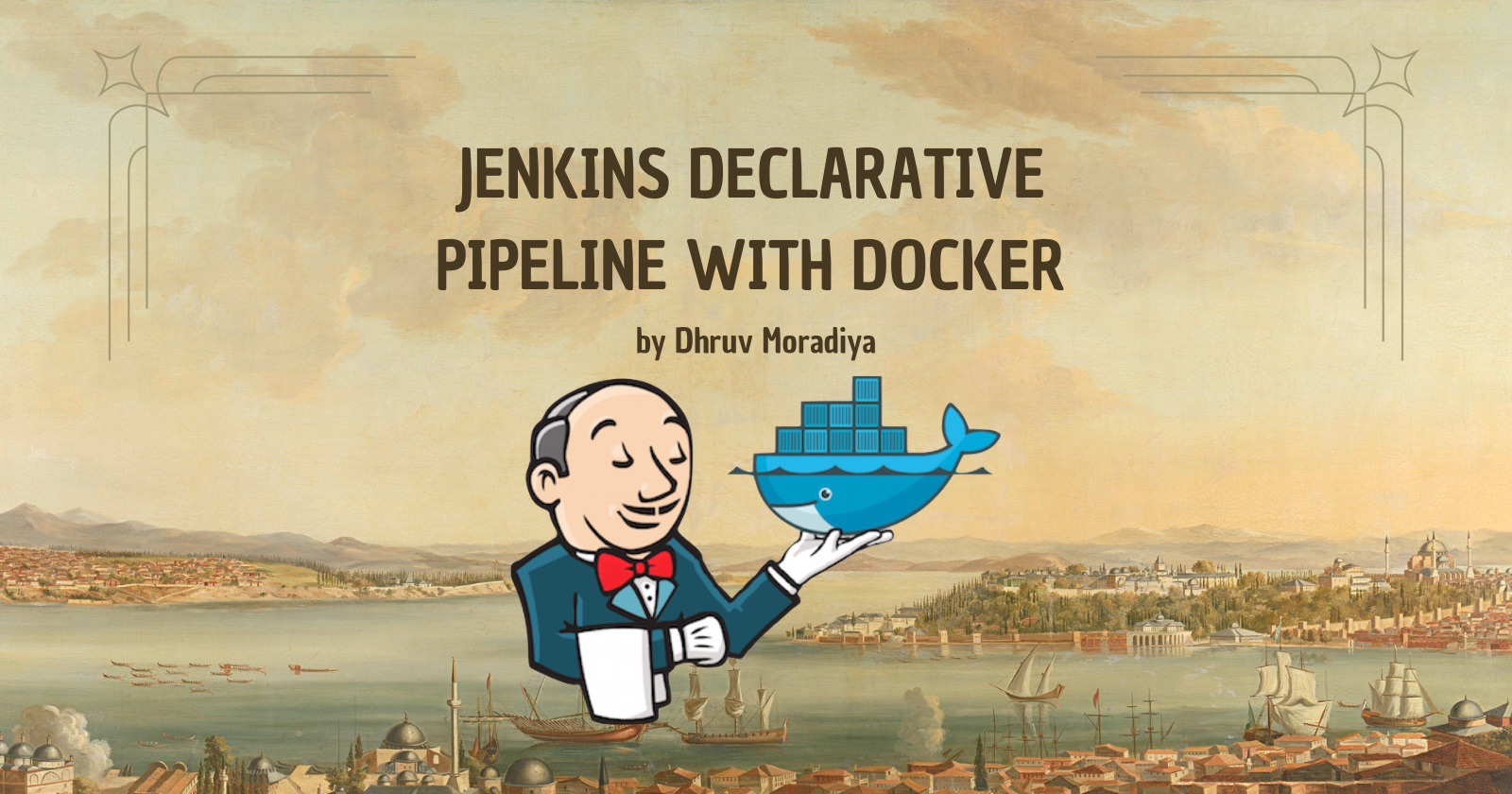
Following up on Day 26’s deep dive into Jenkins Declarative pipelines, it’s time to level up by integrating Docker! 🌊 This guide will take you through creating a Docker-integrated Jenkins Declarative pipeline, letting Jenkins handle Docker images and containers as part of your automation setup.
Whether you're new to Docker or simply polishing your pipeline skills, this task will empower you with skills in building and running Docker containers directly from Jenkins pipelines.
🛠️ What You’ll Need
Jenkins up and running 💻
Docker installed on your server with the correct permissions 🐳
🏗️ Task 1: Create a Docker-Integrated Jenkins Declarative Pipeline
Now, it’s time to create a Jenkins Declarative pipeline that integrates Docker using the syntax above. Here’s how:
Open Jenkins and create a new pipeline job.
Add the Declarative Pipeline Syntax above in the Pipeline script section.
Run the Job to build your Docker image using Jenkins.
⚠️ Heads Up! You might see errors if you run the job twice since Docker containers from the previous run could still be active. Let’s handle this in Task 2.
🛠️ Task 2: Use Docker Groovy Syntax to Avoid Errors
In Jenkins, you can also write Docker commands using Groovy syntax within your pipeline. This can help prevent errors if a container already exists. Here’s how:
Add Docker Groovy Syntax instead of shell commands (
sh
) to manage Docker.Check out Jenkins' documentation for guidance on using Docker within Groovy pipelines.
🔗 Helpful Link: Jenkins Docker Groovy Documentation
⚠️Note: given below project complete both task.
🌐 Step 1: Create a Simple Node.js Application
1.1 Set Up Your Node.js Project
Create a project directory for your app:
mkdir jenkins-docker-project cd jenkins-docker-project
Initialize a Node.js application and install Express:
npm init -y npm install express
Create a simple Express server in
index.js
:const express = require('express'); const app = express(); const PORT = process.env.PORT || 3000; app.get('/', (req, res) => { res.send('Hello from Jenkins and Docker!'); }); app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Add a Dockerfile to containerize your application:
# Dockerfile for Node.js App FROM node:14 WORKDIR /app COPY package*.json ./ RUN npm install COPY . . EXPOSE 3000 CMD ["node", "index.js"]
Test the Docker image locally:
docker build -t jenkins-docker-app . docker run -d -p 3000:3000 jenkins-docker-app
📑 Step 2: Jenkins Declarative Pipeline with Shell Commands (Task 1)
In this step, we’ll set up a Jenkins Declarative Pipeline to build and run our Docker container using shell commands.
Open Jenkins and create a new Pipeline project called
Jenkins-Docker-Pipeline
.In the Pipeline section, use the following script to define your pipeline:
pipeline { agent any stages { stage('Build Docker Image') { steps { // Use shell command to build the Docker image sh 'docker build -t jenkins-docker-app .' } } stage('Run Docker Container') { steps { // Use shell command to run the Docker container sh 'docker run -d -p 3000:3000 jenkins-docker-app' } } } }
Run the pipeline by clicking Build Now.
⚠️ Note: If you rerun this pipeline, you may encounter errors due to an existing container with the same name. This happens because Docker doesn’t allow duplicate container names. Let’s solve this in Task 2!
🔄 Step 3: Using Docker Groovy Syntax to Avoid Conflicts (Task 2)
To avoid container conflicts, use Docker Groovy syntax to manage Docker containers directly in Jenkins, ensuring smoother execution.
Update your Jenkins pipeline with the following script, which uses Docker Groovy syntax:
pipeline { agent any stages { stage('Build Docker Image') { steps { script { // Build the Docker image and assign it to a variable def appImage = docker.build("jenkins-docker-app") } } } stage('Run Docker Container') { steps { script { // Run the container from the image without conflict appImage.run('-d -p 3000:3000') } } } } }
Explanation:
def appImage = docker.build("jenkins-docker-app")
builds and tags the Docker image, storing it inappImage
.appImage.run('-d -p 3000:3000')
runs the container, avoiding shell commands and potential conflicts by using Jenkins’ Docker integration.
Run the updated pipeline by clicking Build Now. You should see the pipeline build and run the container without error.
🧹 Step 4: Clean Up Containers to Save Resources (Optional)
Add a cleanup stage to stop and remove any running containers of the same image after each build:
stage('Cleanup') {
steps {
sh 'docker stop $(docker ps -q --filter ancestor=jenkins-docker-app) || true'
sh 'docker rm $(docker ps -a -q --filter ancestor=jenkins-docker-app) || true'
}
}
This ensures that you free up system resources by stopping and removing previous containers.
🧩 Optional: Apply This to Previous Projects
Now that you’re familiar with Docker integration in Jenkins, try applying this Declarative pipeline approach to your previous projects. Practice makes perfect, and applying these steps to other projects will reinforce your skills! 👏
🏆 Key Takeaways
Use
docker build
anddocker run
commands in Jenkins pipelines to automate Docker tasks.Prevent errors by using Groovy syntax for Docker stages in Jenkins.
Apply these techniques across other projects for more practice and mastery.
Happy Building! 🎉
Subscribe to my newsletter
Read articles from Dhruv Moradiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
