Collections Galore: Arrays Explained!
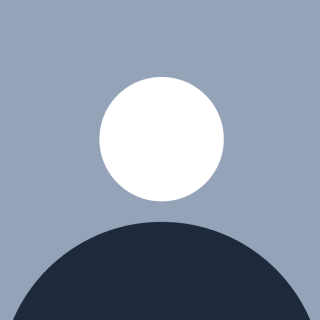
Table of contents
- Introduction to Arrays
- Creating and Using Arrays
- Common Array Methods — Adding and Removing Friends
- Flowchart: Array Manipulation Flow
- Building Your Friends List — Hands-On Challenge
- Mastering the Basics of Arrays
- Advanced Array Methods — Fine-Tuning the Guest List
- Looping Through Arrays — Inviting All Your Friends
- Challenge Time — Array Manipulation Challenges
- Mastering Arrays in JavaScript
- Let’s explore Advanced Array Methods!
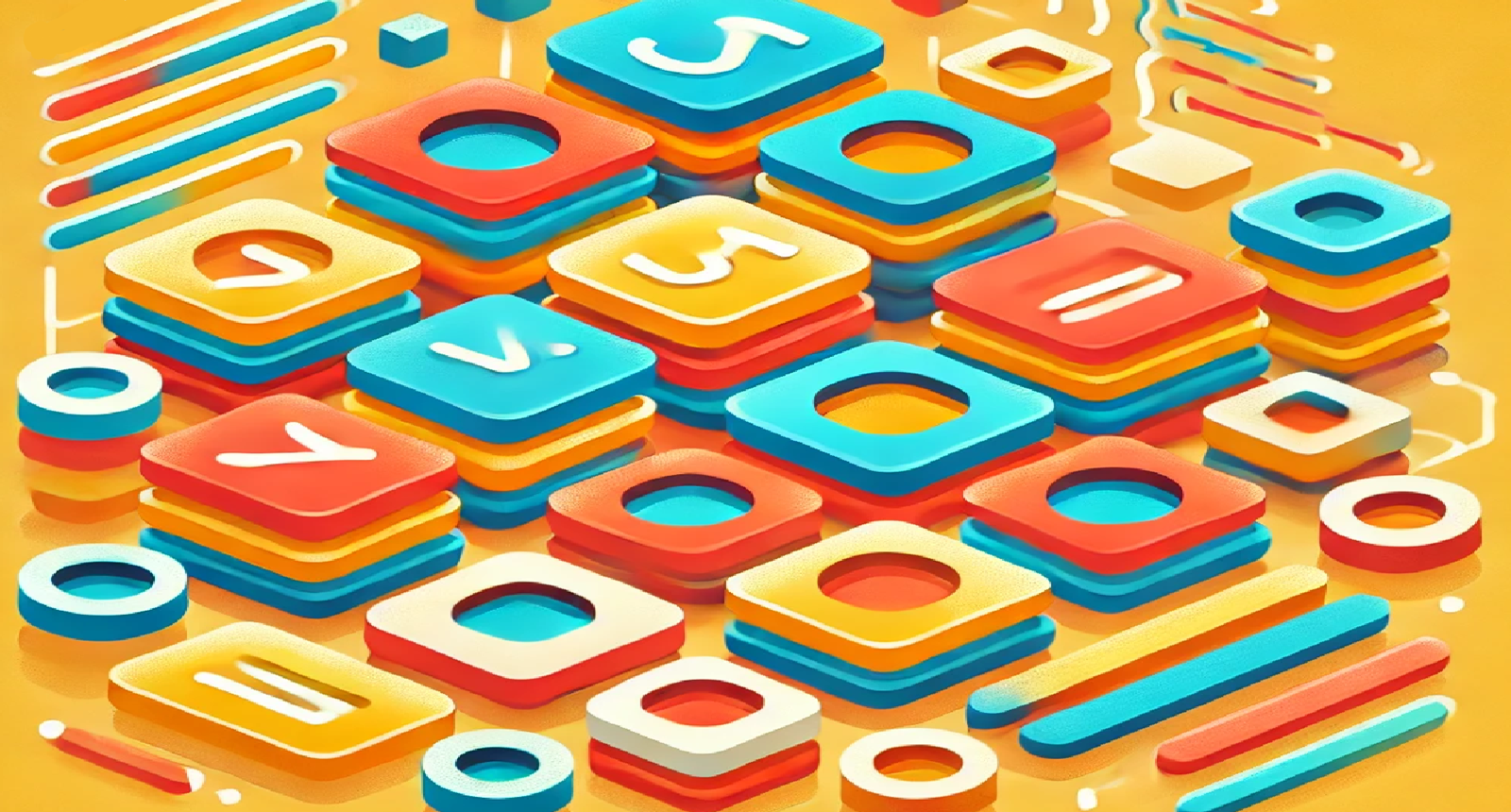
Imagine you’re hosting a party, and all your friends are lined up in a neat list. You want to know who’s attending, add new friends, remove a few, or maybe even shuffle them around. In JavaScript, arrays are the perfect tool for managing collections of data, just like your list of friends at the party!
Arrays are one of the most versatile data structures in JavaScript. They allow you to store multiple values in a single variable, access and modify those values, and perform a wide variety of operations using built-in methods.
Let’s explore the Array of Friends and learn how arrays work, how to manipulate them, and how they make your code more powerful.
Introduction to Arrays
In JavaScript, an array is a special kind of object that can hold a collection of values, called elements. Each element in the array is assigned a numerical index, starting from 0. You can think of an array as a group of friends, each with a unique position in the line, making it easy to access any of them by their position.
For example, an array might store the names of friends attending your party:
let friends = ["Alice", "Bob", "Charlie"];
In this case, the array friends
has three elements: "Alice"
, "Bob"
, and "Charlie"
. Each friend is assigned an index, starting from 0:
friends[0]
is"Alice"
friends[1]
is"Bob"
friends[2]
is"Charlie"
Creating and Using Arrays
Code Snippet: Array Initialization
Let’s start by creating an array of friends. There are several ways to initialize arrays in JavaScript.
Method 1: Array Literal
The most common way to create an array is using array literals:
let friends = ["Alice", "Bob", "Charlie"];
Method 2: Array Constructor
You can also use the Array constructor, but this is less commonly used for simple arrays:
let friends = new Array("Alice", "Bob", "Charlie");
Accessing Array Elements
To access an individual element in the array, you use its index:
console.log(friends[0]); // Output: "Alice"
console.log(friends[2]); // Output: "Charlie"
You can modify array elements by assigning new values to their respective index:
friends[1] = "David"; // Replaces "Bob" with "David"
console.log(friends); // Output: ["Alice", "David", "Charlie"]
Common Array Methods — Adding and Removing Friends
Arrays come with a variety of methods that allow you to manipulate their elements. Let’s explore some of the most commonly used array methods:
1. push() — Add a New Friend
The push()
method adds a new element to the end of the array.
friends.push("Eva");
console.log(friends); // Output: ["Alice", "David", "Charlie", "Eva"]
2. pop() — Remove the Last Friend
The pop()
method removes the last element from the array.
friends.pop();
console.log(friends); // Output: ["Alice", "David", "Charlie"]
3. shift() — Remove the First Friend
The shift()
method removes the first element of the array.
friends.shift();
console.log(friends); // Output: ["David", "Charlie"]
4. unshift() — Add a New Friend at the Beginning
The unshift()
method adds a new element to the beginning of the array.
friends.unshift("George");
console.log(friends); // Output: ["George", "David", "Charlie"]
Flowchart: Array Manipulation Flow
Here’s a flowchart that visually represents the manipulation of your array of friends using these methods:
[ Start ]
|
V
[ Initial Friends: "Alice", "Bob", "Charlie" ]
|
+-----------+
| |
push() pop()
Add "Eva" Remove last element
| |
V V
["Alice", "Bob", "Charlie", "Eva"] ["Alice", "Bob"]
| |
shift() unshift()
Remove first Add "George" at start
| |
V V
["Bob", "Charlie"] ["George", "Bob", "Charlie"]
This flowchart shows how you can dynamically manipulate the array as you manage your list of friends, adding or removing elements as needed.
Building Your Friends List — Hands-On Challenge
Now it’s time for you to practice what you’ve learned. Imagine you’re building a simple app that allows you to manage a list of friends. Your task is to create an app that can add, remove, and display friends from an array.
Challenge: Build a Friends List App
Initialize an empty array to hold the names of friends.
Add friends using
push()
andunshift()
.Remove friends using
pop()
andshift()
.Display the updated list of friends after each operation.
Here’s a starting point for your code:
let friends = [];
// Add friends
friends.push("Alice");
friends.unshift("Bob");
console.log(friends); // Output: ["Bob", "Alice"]
// Remove friends
friends.pop();
console.log(friends); // Output: ["Bob"]
Try adding more friends, removing them, and experimenting with different methods to see how your list changes. By the end, you’ll have a fully functional friends list app!
Mastering the Basics of Arrays
In this part, you’ve learned how to create arrays, access their elements, and use common methods like push()
, pop()
, shift()
, and unshift()
to manipulate them. Arrays are an essential part of JavaScript, helping you manage collections of data with ease—whether that’s a list of friends, numbers, or any other type of information.
In the next part, we’ll dive deeper into more advanced array methods like splice()
, slice()
, and concat()
to expand your skills further. Plus, we’ll explore how to loop through arrays effectively.
Advanced Array Methods — Fine-Tuning the Guest List
Now that we’ve covered the basics of adding and removing elements, it’s time to explore more advanced array methods. These will give you greater control over how you manage and manipulate your arrays. Let’s dive deeper into some key methods like splice(), slice(), and concat().
1. splice() — Adding or Removing Friends from Anywhere
The splice()
method allows you to add, remove, or replace elements in an array at any position. Think of it like rearranging or trimming your guest list before the party starts.
array.splice(startIndex, deleteCount, item1, item2, ...)
startIndex: Where to begin changing the array.
deleteCount: How many elements to remove (0 if you’re just adding).
item1, item2, ...: The elements to add, if any.
Example: Let’s say you want to invite "Eva" between "Bob" and "Charlie" on your list:
let friends = ["Alice", "Bob", "Charlie"];
friends.splice(2, 0, "Eva"); // Adds "Eva" at index 2
console.log(friends); // Output: ["Alice", "Bob", "Eva", "Charlie"]
You can also use splice()
to remove elements:
friends.splice(1, 1); // Removes "Bob"
console.log(friends); // Output: ["Alice", "Eva", "Charlie"]
2. slice() — Slicing Off a Section of Friends
The slice()
method creates a shallow copy of a portion of an array into a new array, leaving the original array untouched. It’s perfect when you want to make a smaller guest list without modifying the original.
array.slice(startIndex, endIndex);
startIndex: The index to begin extraction (inclusive).
endIndex: The index to stop extraction (exclusive).
Example: Let’s slice off the first two friends from the list:
let friends = ["Alice", "Bob", "Charlie", "David"];
let closeFriends = friends.slice(0, 2);
console.log(closeFriends); // Output: ["Alice", "Bob"]
The slice()
method returns a new array containing "Alice" and "Bob," but the original friends
array remains unchanged.
3. concat() — Merging Friends Lists
The concat()
method combines two or more arrays into one. Imagine merging two groups of friends from different parties into one big guest list.
let newArray = array1.concat(array2);
Example: Let’s merge two arrays of friends:
let friends1 = ["Alice", "Bob"];
let friends2 = ["Charlie", "David"];
let allFriends = friends1.concat(friends2);
console.log(allFriends); // Output: ["Alice", "Bob", "Charlie", "David"]
Looping Through Arrays — Inviting All Your Friends
Now that you’ve mastered adding, removing, and modifying friends in your array, let’s talk about looping through arrays to perform actions on each element. Just as you’d greet each guest at the party, you can loop through an array to access and manipulate each element.
There are several ways to loop through arrays in JavaScript, including the for loop, forEach method, and for-of loop.
Code Snippet: Using For Loop to Greet Friends
Let’s use a traditional for loop
to print a greeting for each friend in your array:
let friends = ["Alice", "Bob", "Charlie"];
for (let i = 0; i < friends.length; i++) {
console.log("Hello, " + friends[i] + "!");
}
Output:
Hello, Alice!
Hello, Bob!
Hello, Charlie!
The for loop
runs from the first element to the last, greeting each friend along the way.
Code Snippet: Using forEach() Method
The forEach()
method is another way to loop through an array. It’s a cleaner and more functional approach to iterating over each element:
friends.forEach(friend => {
console.log("Welcome, " + friend + "!");
});
Output:
Welcome, Alice!
Welcome, Bob!
Welcome, Charlie!
The forEach()
method is perfect for when you want to apply the same action to every element in the array.
Code Snippet: Using for-of Loop
The for-of
loop is an elegant way to iterate over arrays, directly accessing the elements rather than dealing with indices:
for (let friend of friends) {
console.log("Hey, " + friend + "!");
}
Output:
Hey, Alice!
Hey, Bob!
Hey, Charlie!
The for-of
loop is concise and easy to read, making it a great option when looping through arrays.
Challenge Time — Array Manipulation Challenges
Now that you've learned about advanced methods and looping, it's time for some challenges to test your array skills.
Challenge 1: Create a Guest List with Custom Methods
Scenario: Create an array of friends and use the methods push()
, splice()
, slice()
, and concat()
to manipulate the guest list.
Example:
let friends = ["Alice", "Bob", "Charlie"];
friends.push("Eva");
friends.splice(1, 0, "David"); // Adds David at index 1
let newFriends = friends.slice(0, 3); // Slices off the first three friends
console.log(newFriends); // Output: ["Alice", "David", "Bob"]
Challenge 2: Remove Specific Friends
Scenario: You want to remove all friends whose names start with the letter "A". Use a loop and splice()
to remove them.
Example:
let friends = ["Alice", "Bob", "Andrew", "Charlie"];
for (let i = 0; i < friends.length; i++) {
if (friends[i][0] === "A") {
friends.splice(i, 1);
i--; // Adjust index after removal
}
}
console.log(friends); // Output: ["Bob", "Charlie"]
Challenge 3: Combine and Sort Friends
Scenario: Combine two arrays of friends and then sort them alphabetically.
Example:
let friends1 = ["Charlie", "Alice"];
let friends2 = ["Bob", "David"];
let allFriends = friends1.concat(friends2).sort();
console.log(allFriends); // Output: ["Alice", "Bob", "Charlie", "David"]
Challenge 4: Find a Specific Friend
Scenario: Loop through the array and find the index of a specific friend (e.g., "Charlie").
Example:
let friends = ["Alice", "Bob", "Charlie", "David"];
let index = friends.indexOf("Charlie");
console.log("Charlie is at index: " + index); // Output: Charlie is at index: 2
Mastering Arrays in JavaScript
Arrays are one of the most versatile and powerful tools in JavaScript. Whether you’re adding new elements, removing unwanted ones, or iterating over the entire list, arrays give you the flexibility to manage data efficiently. With the methods and techniques you’ve learned in this article, you’re well on your way to mastering arrays.
From splice() and slice() to forEach() and for-of loops, arrays are your gateway to managing collections of data—whether it’s a guest list or more complex datasets.
Let’s explore Advanced Array Methods!
In the next article, we’ll dive deeper into advanced array methods like map()
, filter()
, and reduce()
—powerful tools that will take your array manipulation skills to the next level.
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by