Understanding Flutter App Lifecycle for Better App Performance
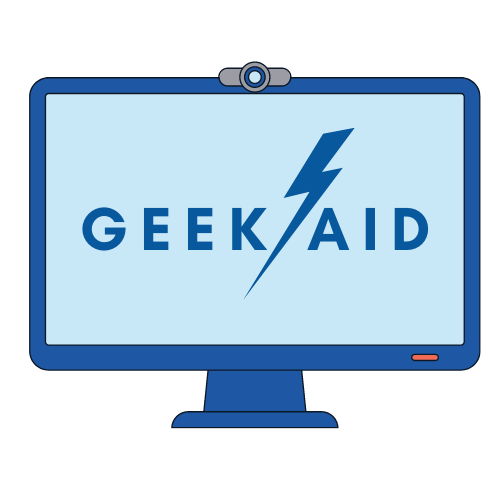
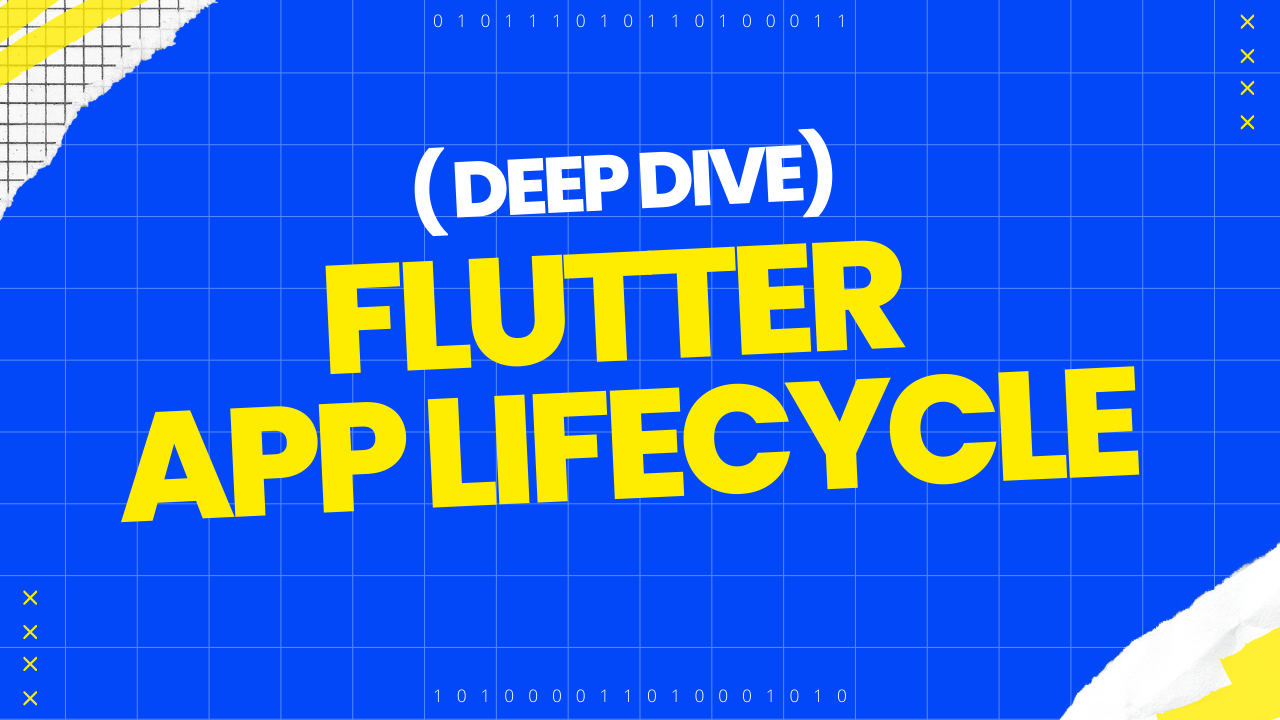
Flutter is an open-source SDK that allows you to build cross-platform applications with a single code base. Many beginners skip learning about the app lifecycle while creating apps, just as they skip documentation. However, understanding and managing the app lifecycle is crucial for optimizing app performance and providing a smooth user experience.
The app lifecycle states the current status of the application. Let's explore each state and its importance.
The Flutter App Lifecycle
Resumed: The app is running in the foreground and receiving user input.
Inactive: The app is in the foreground but the user is not interacting with the app. This can occur when the user opens the notification shade or receives a phone call.
Paused: The app is in the background and not visible to the user. This happens when the user switches to another app or presses the home button.
Hidden: This is an intermediate state between Inactive and paused. This state is active when all the views of the apps are hidden usually when the app is minimized or hidden.
Detached: The app is completely terminated. The app can be terminated either by the user or the operating system.
These states tell how the app can transition from one state to another when certain conditions are met.
Handling App Lifecycle Events
Flutter provides the didChangeAppLifecycleState
method, which you can override by using the WidgetsBindingObserver
class. This method is called whenever the app’s lifecycle state changes, passing an AppLifecycleState
object that allows you to handle different scenarios accordingly.
didChangeAppLifecycleState
: Called whenever the app’s lifecycle state changes. Use this to manage resources or respond to state transitions.
Note: Don’t forget to dispose of any listeners to prevent memory leaks.
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> with WidgetsBindingObserver {
@override
void initState() {
super.initState();
WidgetsBinding.instance.addObserver(this);
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
super.dispose();
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
super.didChangeAppLifecycleState(state);
if (state == AppLifecycleState.paused) {
// App is in background
} else if (state == AppLifecycleState.resumed) {
// App is in foreground
}else if (state == AppLifecycleState.inactive){
// App is in inactive
}else if(state == AppLifecycleState.detached){
// App is in detached
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(child: Text('Hello, Geeks!')),
),
);
}
}
Why App Lifecycle Management is Important
Performance Optimization: By managing lifecycle events, you can reduce unnecessary rebuilds and memory usage, leading to a faster app.
Resource Management: Properly handling lifecycle events ensures efficient use of resources, preventing memory leaks and improving app stability.
User Experience: Managing lifecycle transitions allows you to save the app state when it goes to the background and restore it when it resumes, enhancing user experience.
Real-World Scenarios for Using App Lifecycle Management
Data Refresh: Automatically fetch or update data when the app state changes.
Resource Efficiency: Manage resources like network connections effectively, releasing them when the app is inactive or paused.
State Management: Save and restore the app’s state for a seamless user experience.
My Experience Using AppLifecycleState
In one of my projects, I needed to manage internet connection checks. I used the internet_connection_checker
package to monitor connectivity. However, every time the app was paused or inactive, the “no internet” logic would trigger. When the app resumed the connection was updated to connected, which led to fetching the data from the server, this was necessary for many situations, but in this case, it was unnecessary and just an overhead. So I used the AppLifecycle state disposed of the listed inactive state and started lining again when the lifecycle changed to resume state.
Conclusion
We have understand what is Flutter AppLifeCycle how we can use it to make efficient apps and why understanding the life cycle is important.
Just like my use case if you have any share with us.
Subscribe to my newsletter
Read articles from Geek Aid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
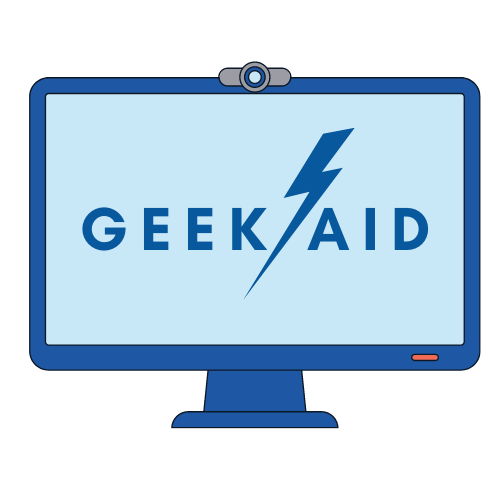