π Understanding Object.is() in JavaScript: A Comprehensive Guide π―

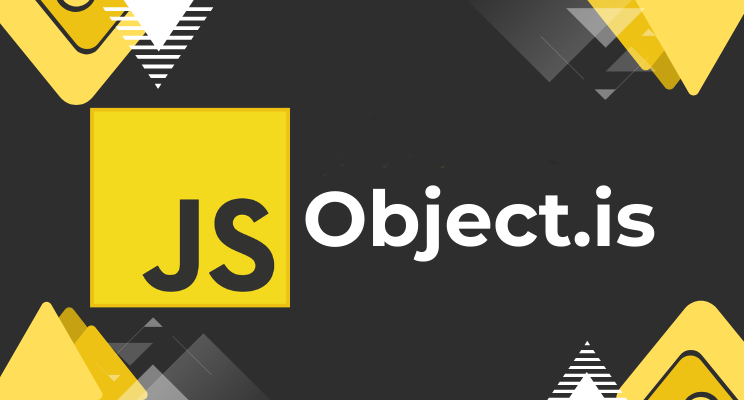
In JavaScript, comparing values is an everyday task. We often use operators like ==
(loose equality) or ===
(strict equality) for comparison. However, JavaScript also provides another method called Object.is
()
, which adds unique behavior to equality checks. In this article, weβll explore how Object.is
()
works, how it differs from other operators, and when you should use it. π
π Table of Contents:
β¨ What is
Object.is
()
?π€ Differences Between
Object.is
()
,==
, and===
π‘ When to Use
Object.is
()
π οΈ Common Use Cases
π― Conclusion
1. β¨ What is Object.is
()
?
Object.is
()
is a method in JavaScript used to determine whether two values are the same. Itβs a simple yet powerful method that takes two arguments:
javascriptCopy codeObject.is(value1, value2);
If the two values are exactly the same, it returns true
; otherwise, it returns false
. β
Example:
javascriptCopy codeObject.is(5, 5); // true
Object.is('hello', 'hello'); // true
Object.is({}, {}); // false (different references)
At first glance, this looks similar to ===
, but as weβll see, there are important differences! π
2. π€ Differences Between Object.is
()
, ==
, and ===
Even though both Object.is
()
and ===
check for strict equality, they behave differently in specific cases.
2.1 Key Differences from ===
:
NaN Comparison: In JavaScript,
NaN === NaN
evaluates tofalse
, which can be confusing. However,Object.is
()
correctly treatsNaN
as equal to itself. πjavascriptCopy codeNaN === NaN; // false β Object.is(NaN, NaN); // true β
Positive and Negative Zero: JavaScript considers
0 === -0
astrue
, butObject.is
()
distinguishes between positive and negative zero.javascriptCopy code0 === -0; // true β Object.is(0, -0); // false β
These cases make Object.is
()
more accurate for specific comparisons than ===
. π₯
2.2 Differences from ==
:
The ==
operator performs type coercion, meaning it converts values to the same type before comparing them. This can lead to unexpected results:
javascriptCopy code1 == '1'; // true β (because of type coercion)
Object.is(1, '1'); // false β
(no type coercion)
Object.is
()
doesnβt perform type coercion, so it only returns true
when values are exactly the same. π
3. π‘ When to Use Object.is
()
Now that we know the differences, letβs see where Object.is
()
shines! β¨
3.1 Detecting NaN
:
If you need to check for NaN
, Object.is
()
is the way to go because ===
wonβt catch it!
javascriptCopy codeconst result = NaN;
if (Object.is(result, NaN)) {
console.log('The result is NaN');
}
3.2 Handling Positive and Negative Zero:
In calculations or measurements where 0
and -0
have different meanings, Object.is
()
can distinguish between them. βοΈ
javascriptCopy codeObject.is(0, -0); // false β
3.3 Accurate Object Comparisons:
For advanced cases where precision matters (like when working with custom objects or floating-point math), Object.is
()
helps avoid the pitfalls of ===
.
4. π οΈ Common Use Cases
4.1 Avoiding Bugs in Complex Apps π:
In large applications dealing with various data types, using Object.is
()
prevents unexpected behavior, especially around NaN
, 0
, and -0
.
4.2 Ensuring Cross-Environment Compatibility π:
Some libraries and polyfills use Object.is
()
to ensure equality checks behave consistently across different environments.
4.3 React's Use of Object.is
()
βοΈ:
In React, the useState
hook internally uses Object.is
()
to check if state values have changed, ensuring edge cases like NaN
or -0
are handled properly.
javascriptCopy codeconst [state, setState] = useState(0);
setState(-0); // React uses Object.is() to compare values
5. π― Conclusion
Object.is
()
may not be as widely known as ==
or ===
, but it offers more accurate value comparison in certain cases. It excels when dealing with tricky edge cases like NaN
and distinguishing between 0
and -0
. πͺ
Whenever you need precise comparisons, especially in complex applications or special data handling, consider using Object.is
()
to avoid subtle bugs. The next time ===
or ==
doesnβt behave as expected, remember that Object.is
()
has your back! π
π¬ What are your thoughts on Object.is
()
? Letβs discuss in the comments!
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.