Arrays and ArrayList in Java

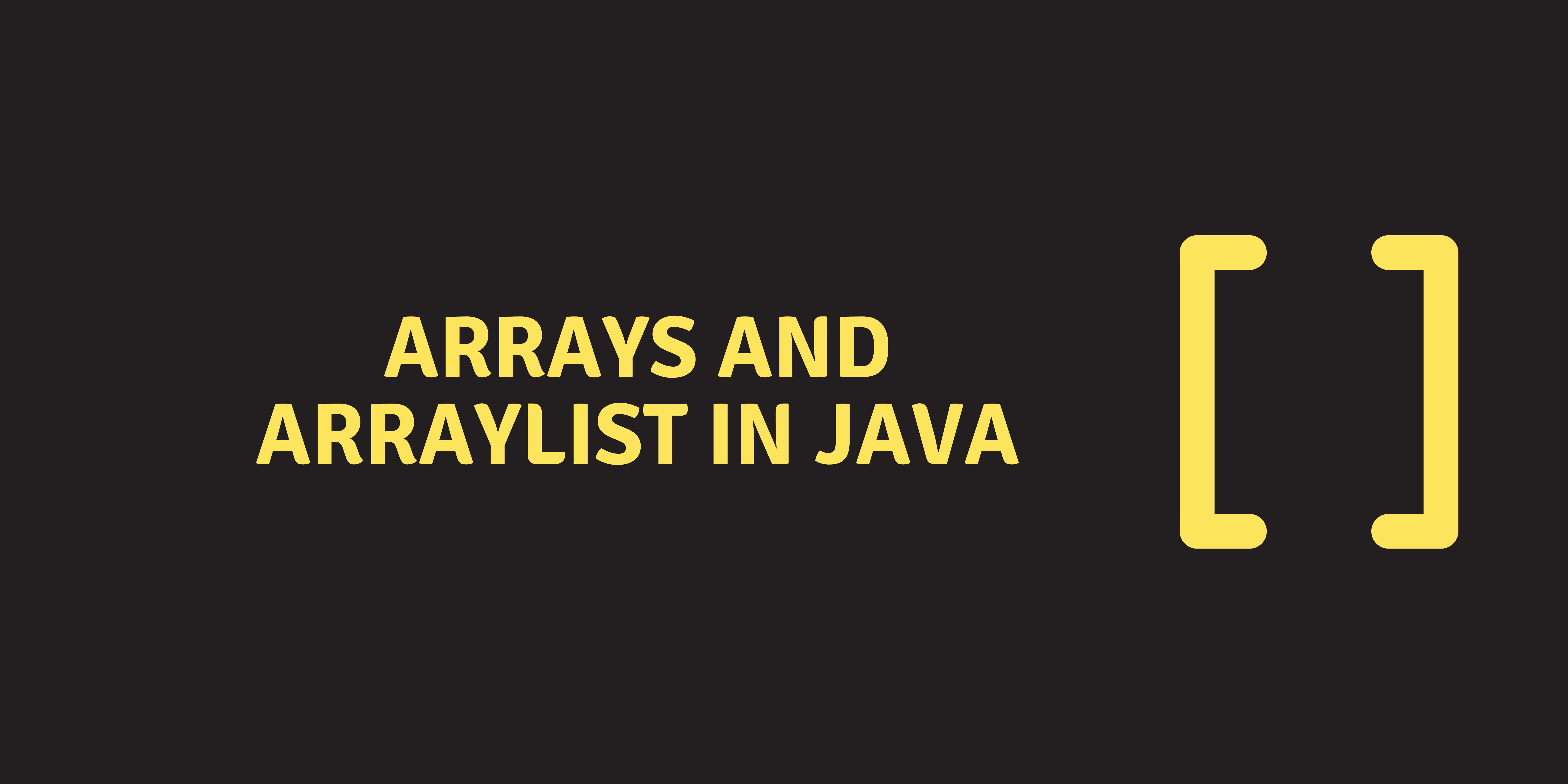
Why do we need Array?
It is very simple when we need to store just five integer numbers. Now let us assume we have to store 1000 integer numbers. Is it possible to use 1000 variables? No.
To handle these situations, in almost all programming language we have a concept called Array.
What is an array?
An array is a collection of similar data type values.
Syntax:
datatype[] variable_name = new datatype[size];
Understanding the Syntax
Let's say we want to store some numbers. Let's take the below example to understand how an array works.
Example:
int[] rollnos = new int[5];
In Java, when you create an array using new int[5]
, you're allocating memory for an array that can hold 5 integers. Here's a breakdown of what's happening:
int[]
: represents the type of data stored in the array.rollnos
: This is the name of the array.new int[5]
: Stores 5 roll nos.
So, after this line of code executes, you have an array named rollnos
that can hold 5 integers. However, at this point, all elements in the array will be initialized to their default value, which is 0 for integers in Java. If you want to assign values to the elements of the array, you need to do that explicitly, like so:
rollnos[0] = 10;
rollnos[1] = 20;
rollnos[2] = 30;
rollnos[3] = 40;
rollnos[4] = 50;
Now, rollnos
array contains: [10, 20, 30, 40, 50]
.
It feels like a hassle to assign values to the elements of an array explicitly. You can declare and initialize an array in a single line. It's particularly useful when you know the values of the array elements at the time of declaration.
int[] rollnos = {10,20,30,40,50};
[10, 20, 30, 40, 50]
-> All the type of data in an array should be same.Internal working of array
datatype[] variable_name;
This step will initialize the variable and it will get initialized in the stack during compile time.
Declaration of array:
int[] rollnos;
:- rollnos
are getting defined in the stack.
Initialization of array:
rollnos = new int[5];
:- Actual memory allocation happens here. Here, object is being created in heap memory.
This above concept is known as Dynamic memory allocation which means at runtime or execution time memory is allocated.
Internal Representation of Array
Internally in Java, memory allocation totally depends on JVM whether it be continuous or not!
Reason 1: Objects are stored in heap memory
Reason 2: In JLS(Java language specification) it is mentioned that heap objects are not continuous
Reason 3: Due to dynamic memory allocation. Hence, array objects in Java may not be continuous(depends on JVM)
Index of an array:
Let's understand with the code below:
// Arrays are used to store multiple values in a single variable.
// Each value has an associated index.
String[] cars = {"Volvo", "BMW", "Ford"};
// Explanation:
System.out.println(cars[0]); // Output: Volvo (Access the value at index 0)
System.out.println(cars[2]); // Output: Ford (Access the value at index 2)
// Indexes start at 0 in Java
// Changing a value at an index
cars[1] = "Toyota";
System.out.println(Arrays.toString(cars)); // Output: [Volvo, Toyota, Ford]
new keyword:
Let's take an array of size 5:
int [] arr = new int[5];
new
: It is used to create an Object. It will create an object in heap memory of array size 5.
If we don't provide values in an array, internally by default it stores [0,0,0,0,0] for the above size of the array.
Let's take an example to understand this:
String[] arr = new String[4];
Some points to remember:
Primitive datatypes like int, char, etc are stored in stack.
All other objects are stored in heap memory.
Arrays.toString(array) -> Internally uses for loop and gives the output in proper format
In an array, since we can change the objects, hence they are mutable.
String are immutable
2D Arrays
A 2D array can be visualized as a matrix. Technically, a 2D array in Java is an array where each element is itself another array. This means it is a collection of one-dimensional arrays. You can also imagine a 2D array as a table with rows and columns. Each cell in this table can store a piece of data(numbers, characters, or even objects).
Syntax:
datatype[][] variable_name = new datatype[row_size][column_size];
Declaration of an array:
// Data type followed by two sets of square brackets
int[][] numbers; // Declares a 2D array to hold integers
Creating and Initializing a 2D Array:
// Method 1: Initialization at declaration
int[][] numbers = {
{1, 2, 3}, // Row 0
{4, 5, 6} // Row 1
};
// Method 2: Creation, then initialization
int[][] scores = new int[3][4]; // Creates a 3x4 array
scores[0][0] = 95; // Assign value to first row, first column
Accessing Elements:
int value = numbers[1][2]; // Accesses the element at row 1, column 2
Visual Example:
Think of a 2D array numbers
like this:
numbers:
+---+---+---+
| 1 | 2 | 3 | (Row 0)
+---+---+---+
| 4 | 5 | 6 | (Row 1)
+---+---+---+
Internal working of 2D array:
datatype[][] variable_name;
This step will declare the variable and it will be declared in the stack during compile time.
Example: int[][] num;
variable_name = new datatype[row_size][column_size];
In this step, a new object will be created/initialized in the heap memory during runtime. The new
keyword is used to create a new object.
Example: num = new int[2][2];
ArrayLists
Let's say you don't want to set a fixed size or length of an array because either you don't know how many elements you may add or you may want to update the size in the future. ArrayList is a part of collection framework and is present in java.util.package . It provides us with dynamic array in Java. It is slower than standard arrays.
Syntax:
Array vs ArrayList:
Array | ArrayList |
An Array has a fixed length or size. | An ArrayList can contain as many elements as you want even though an initial size is specified |
An Array can be created using both primitive datatypes as well as non-primitive datatypes. | An ArrayList cannot be created using primitive datatypes. It can only be created using objects or wrapper classes. |
Some methods to remember while using ArrayList:
1. add(element)
This method adds an element to the end of the ArrayList
.
// Create an ArrayList
ArrayList<String> fruits = new ArrayList<>();
// Add elements to the ArrayList
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
System.out.println(fruits); // Output: [Apple, Banana, Orange]
2. set(index, value)
This method replaces the element at the specified index
with the given value
.
// Set the element at index 1 to "Mango"
fruits.set(1, "Mango");
System.out.println(fruits); // Output: [Apple, Mango, Orange]
3. get(index)
This method returns the element at the specified index
.
// Get the element at index 0
String firstFruit = fruits.get(0);
System.out.println(firstFruit); // Output: Apple
Internal working of ArrayList
The size of the ArrayList is internally fixed, but not permanent. It can change according to the input you provide. Suppose ArrayList gets filled by some amount, then:
It will make an ArrayList of say double the size of ArrayList initially.
Old elements are copied into the new ArrayList.
Old ones are deleted.
Example:
- You provide the input for the first time and the initial size is set to 5.
Then, you decide that you want to add another four elements to the ArrayList.
But we don't have enough space. So, what will Java do?
The answer is, that it will create a new list with a new size(it depends on JVM) enough to accommodate new elements.
Then, it will copy the old list to the new list and will delete the old list.
It might look like this:
Thanks for reading:)
Subscribe to my newsletter
Read articles from Saswat Pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saswat Pal
Saswat Pal
Hi there! I'm a computer science student with a keen interest in Java programming and Data Structures & Algorithms. Currently, I am honing my skills in these areas and also familiar with C, C++. As an aspiring developer, I am always open to new opportunities and collaborations that allow me to learn and grow. Let's connect and create something great together!