Activity 34: Basic Crud Python FLask API (SIA2)
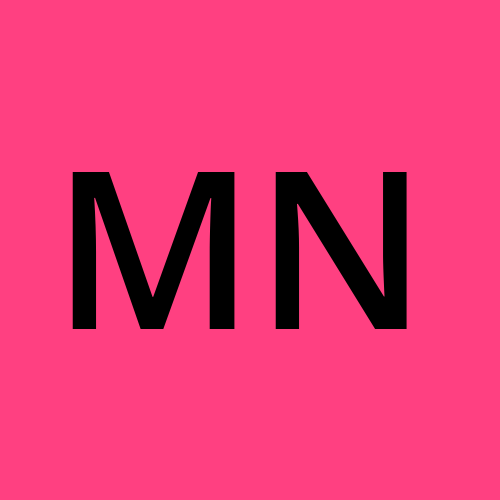
Create a Project Folder:
First, create a new folder where you want to store your project files.
Open a terminal or command prompt and navigate to that folder.
Commands:
mkdir flask_api_project
cd flask_api_project
Create the Virtual Environment:
- In your project folder, create a virtual environment to keep your dependencies isolated.
Commands:
python -m venv myenv
Activate the Virtual Environment:
On Windows:
.\myenv\Scripts\activate
After activating, you should see (myenv)
in the command line, indicating the virtual environment is active.
Install Flask:
- Once the virtual environment is activated, install Flask using
pip
.
- Once the virtual environment is activated, install Flask using
Command:
pip install Flask
Create the
app.py
file:- In your project folder, create a new file named
app.py
. This file will contain the Flask API code.
- In your project folder, create a new file named
Write the Flask API Code:
- Open
app.py
in a text editor and add the following code:
- Open
# Import necessary libraries
from flask import Flask, jsonify, request
# Initialize the Flask application
app = Flask(__name__)
# Sample data: list of students
students = [
{"name": "Juan Carlos", "section": "BSIT-4B", "id": 1, "email": "juan@gmail.com", "age": 22},
{"name": "Jose Rizal", "section": "BSIT-2A", "id": 2, "email": "jose@gmail.com", "age": 21}
# Add more students if needed
]
# GET all students
@app.route('/students', methods=['GET'])
def get_students():
return jsonify(students)
# GET a specific student by ID
@app.route('/students/<int:student_id>', methods=['GET'])
def get_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
return jsonify(student)
else:
return jsonify({"message": "Student not found"}), 404
# POST: Add a new student
@app.route('/students', methods=['POST'])
def add_student():
new_student = request.get_json()
new_student['id'] = max(student['id'] for student in students) + 1 # Generate new ID
students.append(new_student)
return jsonify(new_student), 201
# PUT: Update an existing student by ID
@app.route('/students/<int:student_id>', methods=['PUT'])
def update_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
data = request.get_json()
student.update(data)
return jsonify(student)
else:
return jsonify({"message": "Student not found"}), 404
# DELETE: Remove a student by ID
@app.route('/students/<int:student_id>', methods=['DELETE'])
def delete_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
students.remove(student)
return jsonify({"message": "Student deleted"})
else:
return jsonify({"message": "Student not found"}), 404
# Run the application
if __name__ == '__main__':
app.run(debug=True)
Run the Server:
In the terminal, ensure you are still in the same directory as
app.py
.Start the Flask server by running:
Command:
python app.py
Test the API:
Open your POSTMAN
GET
/students
: Lists all students.GET
/students/<id>
: Retrieves a specific student by ID.POST
/students
: Adds a new student (requires JSON data).PUT
/students/<id>
: Updates an existing student.DELETE
/students/<id>
: Deletes a student.
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
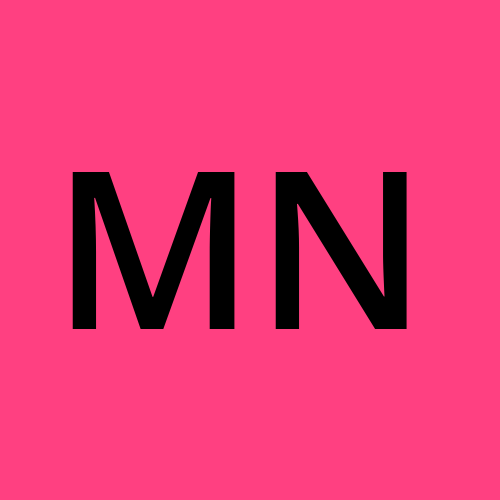