Activity 36: Research Python Flask Project Structure (SIA2)
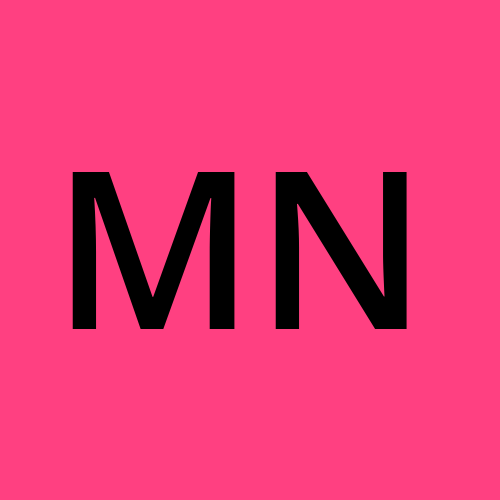
1. Schema
Purpose: The
schema
directory (or sometimesschemas
) is where you define the structure or schema of the data models used in your project.Contents:
Typically, you’d define schemas using libraries like
marshmallow
orPydantic
, which are used for data validation and serialization.These schemas ensure that data sent and received by the API meets certain standards (e.g., correct types, required fields).
Example:
UserSchema
in this directory might define what a valid user object looks like, specifying fields likeid
,name
,email
, etc.
2. Repository
Purpose: The
repository
layer is responsible for interacting with the database. This directory holds functions that handle the actual database queries.Contents:
Contains functions to add, retrieve, update, and delete records in the database. Instead of directly placing database queries inside the Flask views, you use repository functions.
Helps maintain separation between the database logic and the rest of the application, making the codebase easier to manage.
Example:
- A
UserRepository
might contain methods likeget_user_by_id(id)
,create_user(data)
,delete_user(id)
, etc.
- A
3. Blueprint
Purpose: Flask’s
Blueprint
is a way to organize the routes in your application, especially for larger projects. Blueprints allow you to define multiple sets of routes in different files.Contents:
Blueprints help you split your application into modules, each with its own routes. For example, you might have a
user
blueprint for user-related routes and apost
blueprint for post-related routes.They also allow you to register groups of routes in the main application file, keeping your app modular.
Example:
- A
user_
blueprint.py
might define routes like/user/register
,/user/login
, etc. Each blueprint can be registered in the mainapp.py
to enable these routes.
- A
4. Model
Purpose: The
model
directory contains the ORM (Object Relational Mapping) models, which represent the structure of your database tables as Python classes.Contents:
Models are usually built using libraries like SQLAlchemy. Each class in this directory represents a table in your database.
Models define fields, data types, relationships, and basic validation at the database level.
Example:
- A
User
model might have attributes likeid
,username
,email
, andpassword
. These attributes would correspond to columns in a database table.
- A
5. Services
Purpose: The
services
layer contains business logic that handles the functionality of the application. It processes data, applies logic, and then interacts with the repository.Contents:
This directory is where you handle all non-database-related logic that doesn't belong in the routes or database functions. Services call repository functions and apply logic as needed before sending responses back to the client.
Helps keep routes (views) clean and maintains a clear separation of concerns.
Example:
- A
UserService
might contain methods likeregister_user(data)
, which might validate the user data, hash the password, and then call a repository function to add the user to the database.
- A
6. Migration
Purpose: The
migration
directory holds files that track changes to the database schema over time, making it easier to manage changes and keep databases up-to-date.Contents:
Typically managed by a library like Flask-Migrate, which uses Alembic under the hood, the
migration
folder keeps track of database versions and changes. It contains migration files that are automatically generated when you make changes to the models.Makes it easy to apply, upgrade, or downgrade database schema changes across multiple environments (e.g., development, staging, production).
Example:
- Running
flask db migrate
might create a new migration file with instructions to add a new column to theUser
table. You can then apply this change to the database withflask db upgrade
.
- Running
Summary Table
Directory | Purpose |
schema | Defines data structure for validation and serialization. |
repository | Manages direct database queries (CRUD operations). |
blueprint | Organizes routes by grouping them into modules. |
model | Defines database tables as classes with ORM. |
services | Handles business logic and application functionality. |
migration | Tracks database schema changes for version control. |
This structure is ideal for a clean, organized, and scalable Flask project where each part has a distinct role, making the codebase easier to manage and expand as your project grows.
References
https://flask-migrate.readthedocs.io/
https://flask-sqlalchemy.palletsprojects.com/
https://flask.palletsprojects.com/en/stable/
https://blog.miguelgrinberg.com/post/the-flask-mega-tutorial-part-i-hello-world
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
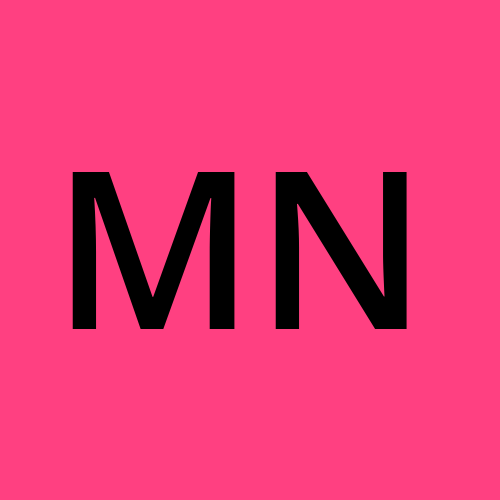