Amazon SQS: Producing Messages for Reliable Asynchronous Processing

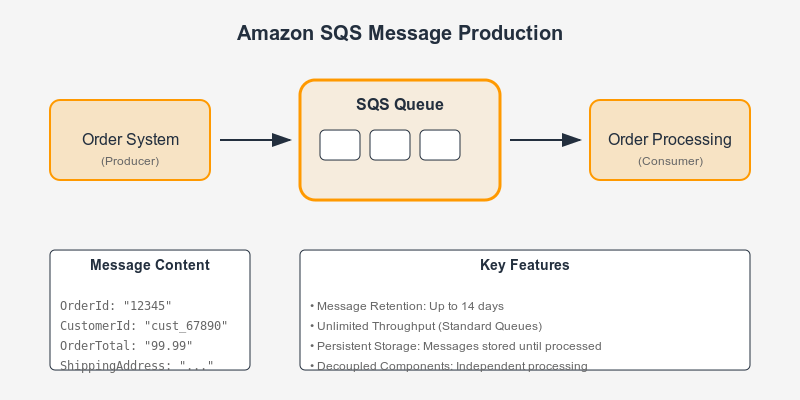
Amazon Simple Queue Service (SQS) is a powerful tool for decoupling the components of your applications and enabling reliable message processing. One of the core functionalities of SQS is producing messages, which allows you to send messages to a queue for asynchronous processing. In this post, I’ll explore how to produce messages to SQS using the SDK, discuss message retention, and illustrate this with a practical example.
Producing Messages to SQS
To produce messages to an SQS queue, you typically use the SendMessage API provided by the AWS SDK. This API is straightforward and allows you to send messages with various attributes to your specified queue.
Key Features of Producing Messages:
Persistence: Once a message is sent to an SQS queue, it is stored persistently until a consumer processes and deletes it. This means that you don’t have to worry about losing messages, even if your consumers are temporarily unavailable.
Message Retention: By default, messages in SQS are retained for 4 days, but you can configure this retention period to last up to 14 days. This flexibility allows you to tune how long messages stay in the queue based on your application’s needs.
Unlimited Throughput (SQS Standard Queues): SQS standard queues support an unlimited number of transactions per second. This means that your application can produce messages at scale without hitting any limits, which is especially beneficial for high-volume applications.
Example: Sending an Order to be Processed
Let’s consider a practical example where we need to send an order to be processed. When a customer places an order, we want to capture the order details and send them to an SQS queue for further processing by another service, such as an order fulfillment system.
Here’s a breakdown of what the message might include:
Order ID: A unique identifier for the order.
Customer ID: The identifier for the customer who placed the order.
Attributes: Any additional attributes you want to include, such as order total, items ordered, shipping address, etc.
Example Code to Produce a Message
Using the AWS SDK for Python (boto3
), we can produce a message to an SQS queue as follows:
pythonCopy codeimport boto3
# Create an SQS client
sqs = boto3.client('sqs')
# Specify the URL of your SQS queue
queue_url = 'https://sqs.us-east-1.amazonaws.com/123456789012/MyQueue'
# Define the order details
order_id = '12345'
customer_id = 'cust_67890'
order_total = '99.99'
shipping_address = '123 Elm St, Anytown, USA'
# Create the message body
message_body = {
'OrderId': order_id,
'CustomerId': customer_id,
'OrderTotal': order_total,
'ShippingAddress': shipping_address
}
# Send the message
response = sqs.send_message(
QueueUrl=queue_url,
MessageBody=str(message_body) # Convert to string
)
# Print the message ID of the sent message
print("Message sent with ID:", response['MessageId'])
In this code:
We create an SQS client to interact with the SQS service.
We specify the URL of the SQS queue where we want to send our message.
We construct a message body that contains the order ID, customer ID, order total, and shipping address.
Finally, we use the
send_message
method to send the message to the queue and print the message ID to confirm it was sent successfully.
Benefits of Using SQS for Message Production
Decoupling Components: By using SQS, you can decouple your application components. For instance, the order placement system can run independently of the order processing system, allowing for more flexible architecture.
Reliable Messaging: With messages being persisted in the queue until consumed, SQS ensures that no messages are lost during processing. This reliability is crucial for business-critical applications.
Scalability: SQS allows for high-throughput message production, making it suitable for applications that need to handle varying loads. As your application scales, SQS can seamlessly accommodate the increased message volume.
Flexible Message Retention: The ability to configure message retention from 4 to 14 days provides options for applications that may need to handle delays in processing.
Subscribe to my newsletter
Read articles from Gedion Daniel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gedion Daniel
Gedion Daniel
I am a Software Developer from Italy.