Activity 39: Python Flask Cookie (SIA2)
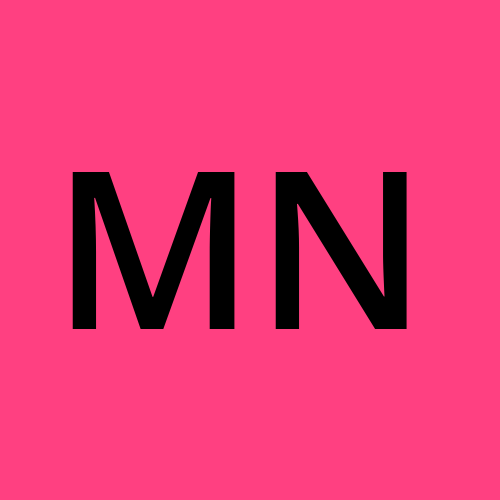
2 min read
Implementation of a Flask application that handles cookies using both POST and GET methods as per the Activity 39 requirements.
Implementing Cookies in Flask
Step 1: Set Up Your Flask Project
Create a New Project Folder:
mkdir nicolas_python_flask_cookies cd nicolas_python_flask_cookies
Set Up a Virtual Environment:
python -m venv venv venv\Scripts\activate # For Windows users pip install Flask
Create the Main Application File: Create a file named
app.py
and add the following code:from flask import Flask, request, make_response, jsonify app = Flask(__name__) @app.route('/setcookies', methods=['POST']) def set_cookies(): # Get the cookie value from the POST request cookie_value = request.form.get('cookie_value') response = make_response(jsonify({"message": "Cookie has been set!"})) # Set the cookie response.set_cookie('my_cookie', cookie_value) return response @app.route('/getcookies', methods=['GET']) def get_cookies(): # Retrieve the cookie from the request cookie_value = request.cookies.get('my_cookie') if cookie_value: return jsonify({"cookie_value": cookie_value}) return jsonify({"message": "No cookie found!"}) if __name__ == '__main__': app.run(debug=True)
Step 2: Test Your Application
Run the Flask Application: Execute the following command in your terminal:
python app.py
Setting the Cookie: You can use a tool like Postman.
Set method to
POST
http://127.0.0.1:5000/setcookies -d "cookie_value=your_cookie_value_here"
Getting the Cookie: To retrieve the cookie, send a GET request:
http://127.0.0.1:5000/getcookies
Step 3: Initialize Git and Push to GitHub
git init
git add .
git commit -m "Initial commit for Python Flask cookie implementation"
git remote add origin https://github.com/MonetForProgrammingPurposes/nicolas_python_flask_cookies.git
git branch -M master
git push -u origin master
LINK
REPOSITORY GITHUB:
https://github.com/MonetForProgrammingPurposes/nicolas_python_flask_cookies.git
0
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
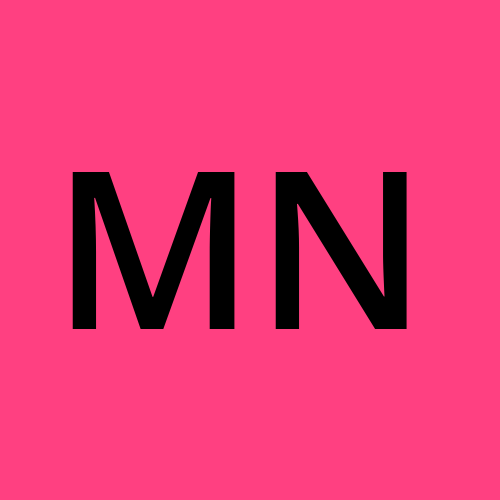