Building an Event-Handling Form with React: A Simple Guide for Beginners

Table of contents
- Introduction
- Getting Started
- Project Setup
- Step 1: Setting Up the Entry Point (index.html)
- Step 2: Main Entry Point (main.jsx)
- Step 3: Creating the Main Component (App.jsx)
- Step 4: Creating the Button Component (Button.jsx)
- Step 5: Adding CSS for Styling (App.css)
- Explanation of Code and Key Concepts
- Running the Application
- Conclusion
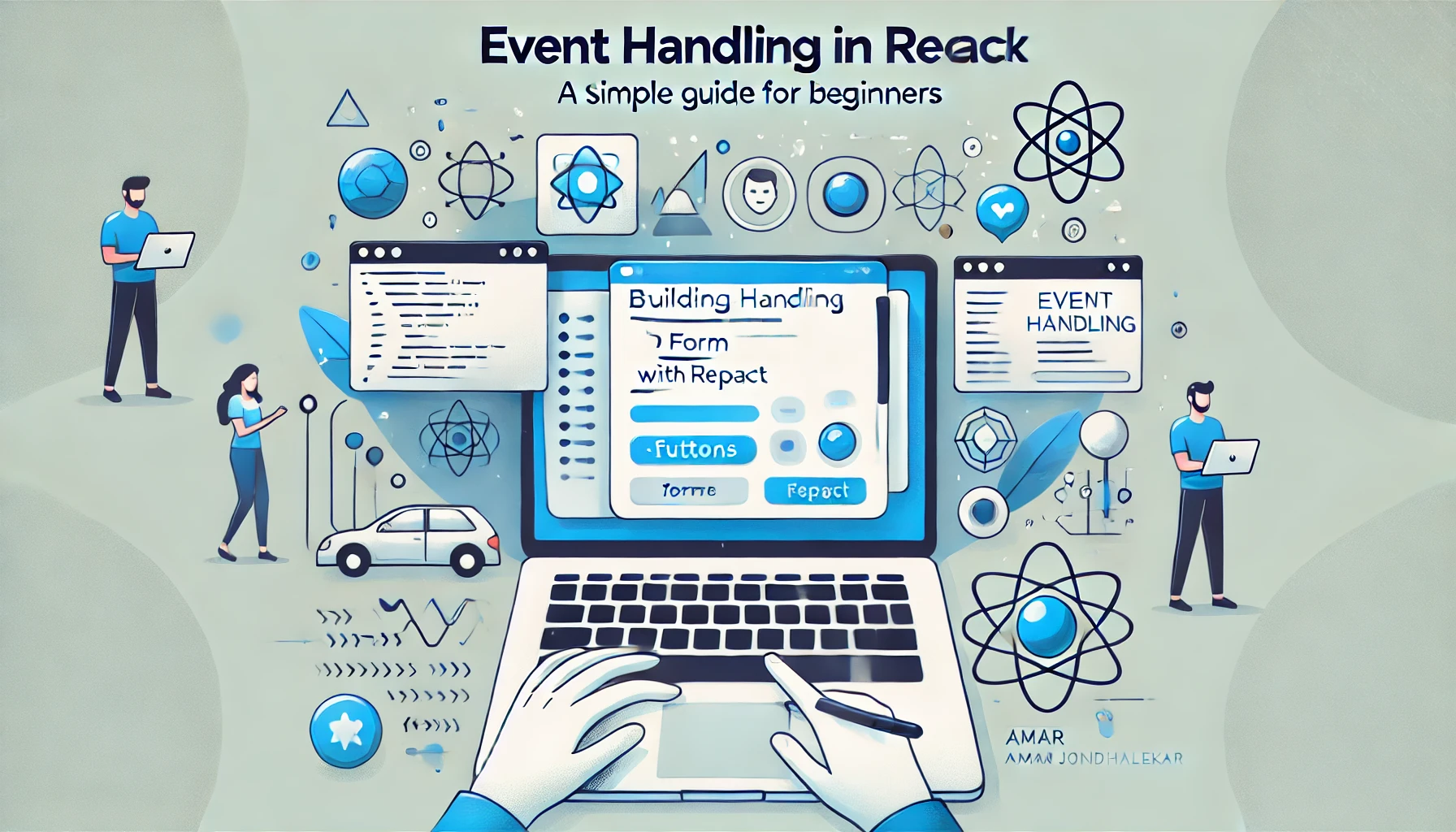
Introduction
Event handling is a core concept in React that allows us to interact with users. This tutorial will walk you through creating a basic React application that manages various event types like form submissions, button clicks, and text input changes. I’ll also show how to structure and style components for a clean, modular design. By the end, you’ll know how to set up event handling in React with a user-friendly approach.
Getting Started
To start, here’s the project structure, dependencies, and initial files needed for our app.
Dependencies: We’ll be using
React
andReact-DOM
as our main dependencies, along withVite
for fast development.Development Tools: ESLint is included to help maintain code quality and consistency.
Project Setup
Your package.json
file should look like this:
{
"name": "event-handling",
"private": true,
"version": "0.0.0",
"type": "module",
"scripts": {
"dev": "vite",
"build": "vite build",
"lint": "eslint .",
"preview": "vite preview"
},
"dependencies": {
"react": "^18.3.1",
"react-dom": "^18.3.1"
},
"devDependencies": {
"@eslint/js": "^9.13.0",
"@types/react": "^18.3.12",
"@types/react-dom": "^18.3.1",
"@vitejs/plugin-react": "^4.3.3",
"eslint": "^9.13.0",
"eslint-plugin-react": "^7.37.2",
"eslint-plugin-react-hooks": "^5.0.0",
"eslint-plugin-react-refresh": "^0.4.14",
"globals": "^15.11.0",
"vite": "^5.4.10"
}
}
After installing dependencies, let’s structure the core components and functions for event handling.
Step 1: Setting Up the Entry Point (index.html
)
Your HTML file initializes the React app, loading main.jsx
as the entry point.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Event Handling in React</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
</body>
</html>
Step 2: Main Entry Point (main.jsx
)
Using StrictMode
in main.jsx
will help detect issues during development.
import { StrictMode } from 'react';
import { createRoot } from 'react-dom/client';
import './index.css';
import App from './App.jsx';
createRoot(document.getElementById('root')).render(
<StrictMode>
<App />
</StrictMode>,
);
Step 3: Creating the Main Component (App.jsx
)
The App
component will house our input forms and buttons, allowing us to handle various events. We define functions to handle each type of event, such as button clicks and form submissions.
import { useState } from 'react';
import './App.css';
import Button from './components/Button';
function App() {
// Function to handle input changes
function handleInputChange(e) {
console.log('Current value:', e.target.value);
}
// Function to handle form submissions
function handleSubmit(e) {
e.preventDefault();
alert('Form submitted');
}
return (
<>
<form onSubmit={handleSubmit}>
<input type="text" onChange={handleInputChange} />
<button type="submit">Submit</button>
</form>
<form onSubmit={handleSubmit}>
<Button clickMe={handleInputChange}></Button>
</form>
</>
);
}
export default App;
Step 4: Creating the Button Component (Button.jsx
)
The Button
component allows us to handle clicks, text input changes, and mouse-over events.
import React from 'react';
const Button = (props) => {
return (
<div>
<h2>Click the button:</h2>
<button onClick={props.clickMe}>
I don't do anything
</button>
<input type="text" onChange={props.clickMe} />
<button onSubmit={props.onSubmit} type="submit">Submit</button>
<p onMouseOver={props.clickMe} style={{border: "1px solid black"}}>
I am a paragraph
</p>
</div>
);
};
export default Button;
Step 5: Adding CSS for Styling (App.css
)
Let’s add some basic CSS to make our form and button components look cleaner and more user-friendly.
/* App.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 20px;
background-color: #f5f5f5;
}
form {
margin-bottom: 20px;
}
button {
padding: 10px 15px;
background-color: #6200ea;
color: white;
border: none;
cursor: pointer;
border-radius: 4px;
}
button:hover {
background-color: #3700b3;
}
Explanation of Code and Key Concepts
Event Handlers: We use
handleInputChange
to monitor text input,handleSubmit
for form submission, andclickMe
in theButton
component to respond to different events like clicking a button or hovering over a paragraph.Preventing Default Behavior: The
handleSubmit
function usese.preventDefault()
to stop the form’s default submission and display a custom alert instead.Modular Components: Breaking down UI into reusable components like
Button
simplifies our code and promotes code reuse.Props in Components: The
Button
component acceptsclickMe
as a prop to trigger events, keeping the code clean and allowing flexible event handling.
Running the Application
Development Server: To start the app, run
npm run dev
. Vite will launch a development server, and you can view your application in the browser at the provided local URL.Testing Events: Interact with the form by typing text, clicking the buttons, or hovering over the paragraph. Check the console to see logged values and behavior in real time.
Conclusion
Congratulations! You’ve now built a simple event-handling form in React with custom functions for each type of user interaction. Understanding these fundamentals will help you create more dynamic and interactive web applications in React. This example sets up the basic structure, allowing you to expand it as needed for more complex applications.
Feel free to add additional events and modify styles to match your project requirements!
This tutorial should give you a good foundation for handling events in React, keeping the code organized and reusable. Let me know how this worked for you in the comments!
Subscribe to my newsletter
Read articles from Amar Jondhalekar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amar Jondhalekar
Amar Jondhalekar
👨💻 Amar Jondhalekar | Front End Web Developer at Cognizant | Content Creator | Founder at Campuslight With over 3 years of experience in web development, I specialize in HTML5, CSS3, JavaScript (ES6+), React.js, and Node.js. As a Full Stack Developer at Cognizant, I gained hands-on experience building dynamic, responsive applications focused on user-centric design and performance. I also founded Campuslight, where I create impactful digital solutions in the education sector, driven by a mission to make learning more accessible. Through my blogs, I share daily technical insights, coding tips, and career advice, aiming to inspire developers and impress recruiters alike. I’m dedicated to leveraging technology to create a more connected and accessible world. Let’s connect, collaborate, and make the web a better place for everyone!