Three.js: A Deep Dive into 3D Web Development

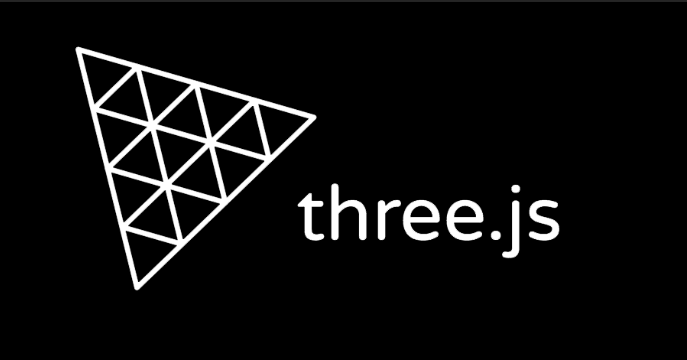
Three.js is a powerful JavaScript library that allows you to create stunning 3D graphics and animations in your web browser. It provides a simple and intuitive API for working with 3D objects, materials, lights, and cameras. With Three.js, you can build immersive experiences like interactive 3D models, virtual reality scenes, and augmented reality applications.
Getting Started with Three.js
Install Three.js: To use Three.js in your project, you'll need to install it using a package manager like npm:
$ npm install three
Create a Basic Scene
import * as THREE from 'three'; // Create a Scene const scene = new THREE.Scene(); // Create a Camera const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); // Create a Renderer const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); // Create a Cube const geometry = new THREE.BoxGeometry(1, 1, 1); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); // Render the Scene camera.position.z = 5; function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); } animate();
Key Concepts in Three.js:
Scene: The root object of the 3D world.
Camera: Defines the viewpoint of the scene.
Renderer: Renders the scene to the screen.
Geometry: Defines the shape of an object.
Material: Defines the appearance of an object.
Mesh: Combines geometry and material to create 3D objects.
Light: Illuminates the scene.
Real-World Use Cases of Three.js
Interactive Product Visualizations: Create 3D models of products to allow customers to explore them from different angles.
Virtual Reality Experiences: Develop immersive VR experiences for gaming, training, and education.
Augmented Reality Applications: Create AR experiences that overlay digital content onto the real world.
Data Visualization: Visualize complex data sets in 3D to uncover insights.
Architectural Visualization: Create stunning 3D visualizations of buildings and urban environments.
Advantages of Using Three.js
Cross-Platform Compatibility: Three.js can be used to create 3D experiences that work on a wide range of devices, including desktops, tablets, and mobile phones.
Open-Source and Free: Three.js is an open-source library, which means it's free to use and modify.
Large and Active Community: A large and active community of developers provides support, tutorials, and resources.
Flexibility and Customization: Three.js offers a high degree of flexibility and customization, allowing you to create unique and innovative 3D experiences.
Performance Optimization: Three.js provides tools and techniques for optimizing performance, ensuring smooth and responsive 3D experiences.
By mastering the fundamentals of Three.js and exploring its advanced features, you can create immersive and interactive 3D experiences that captivate your audience.
Subscribe to my newsletter
Read articles from Little Prince directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Little Prince
Little Prince
I'm an aspiring developer eager to learn more and building new projects