Let’s Build a Tiny Bank in Go!
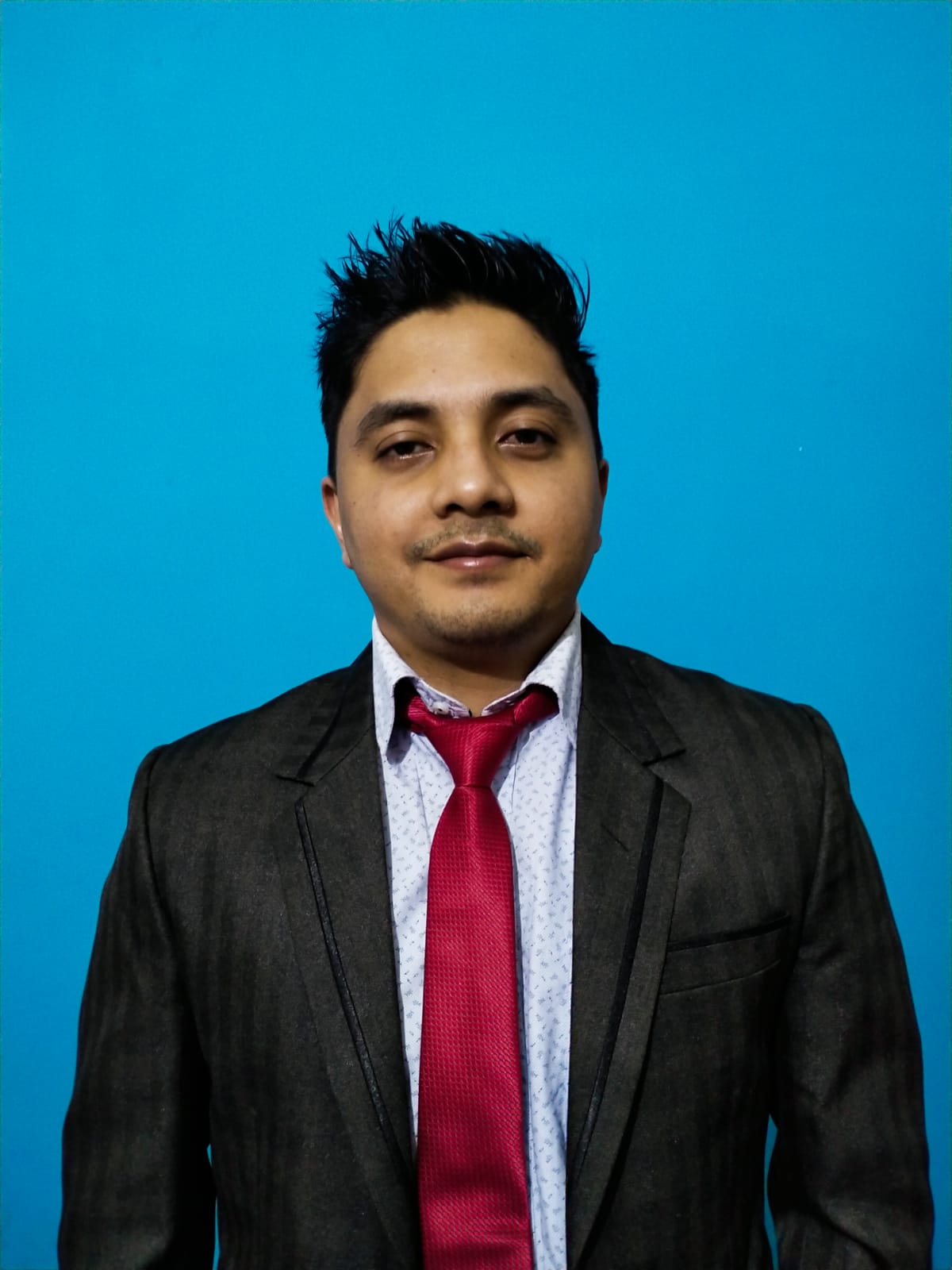
Table of contents
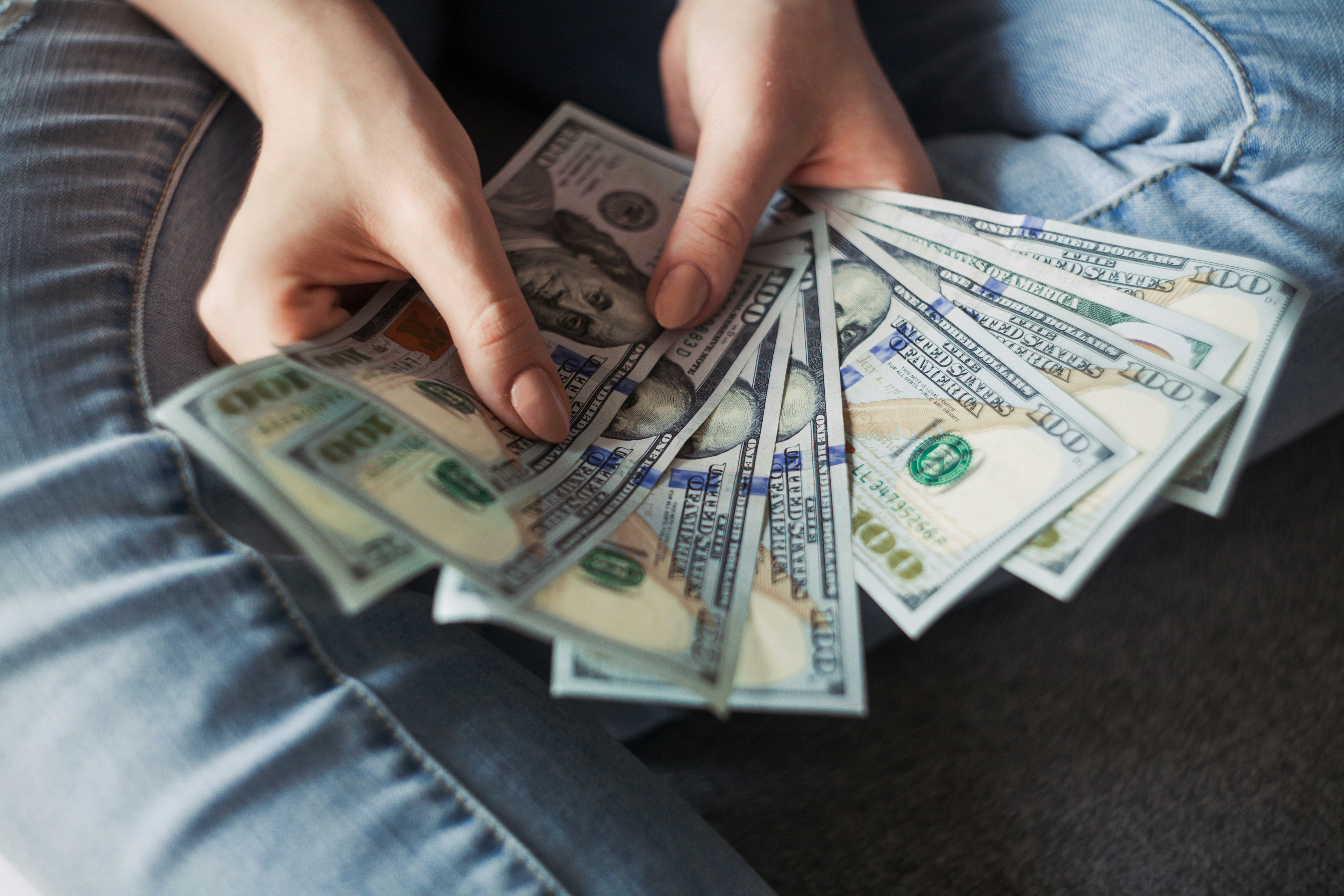
Alright, let’s dive into creating our own mini-banking system with Go! Imagine this: You’re running a bank (on your computer, of course). You want users to check their balance, make deposits, and withdraw cash. Plus, you’ll be saving their account balance in a file, so their cash doesn’t just disappear into the code abyss when they close the program.
Step 1: The Plan
We’ll need three main things:
A way to get the account balance from a file.
A way to write the balance back to the file after each transaction.
A loop that interacts with users for their banking operations.
So, grab a coffee, and let’s get coding!
Step 2: Getting the Balance from File
Let’s start by fetching the balance. We’ll check if there’s an existing balance stored in a file called balance.txt
. If the file doesn’t exist, we’ll assume the account is brand new with a balance of zero (hey, we’re a bank, not a charity!).
Here's the code:
const accountBalanceFile = "balance.txt"
func getBalancefromFile() float64 {
balance, err := os.ReadFile(accountBalanceFile)
if err != nil {
// No file? No worries! Just start fresh.
return 0.0
}
accountBalance, _ := strconv.ParseFloat(string(balance), 64)
return accountBalance
}
Here’s what’s happening:
We try to read
balance.txt
.If it’s there, great! We convert the contents to a float.
If it’s not, we just return
0.0
because new accounts start empty. (Pro tip: Real banks don’t do this!)
Step 3: Saving the Balance Back to the File
Each time there’s a change, we’ll save the balance back to the file so it’s ready for the next time the user logs in. Let’s use writeToFile
to take care of this:
func writeToFile(accountBalance float64) {
os.WriteFile(accountBalanceFile, []byte(fmt.Sprintf("%.2f", accountBalance)), 0666)
}
We format the balance with two decimal places (no one wants to see 9.99999999
). This makes sure everything looks nice and tidy when saved. Also, setting permissions to 0666
is like saying, “Hey, anyone using this program can access this file.” (For serious apps, you’d probably want a bit more security!)
Let’s Build a Tiny Bank in Go!
Alright, let’s dive into creating our own mini-banking system with Go! Imagine this: You’re running a bank (on your computer, of course). You want users to check their balance, make deposits, and withdraw cash. Plus, you’ll be saving their account balance in a file, so their cash doesn’t just disappear into the code abyss when they close the program.
Step 1: The Plan
We’ll need three main things:
A way to get the account balance from a file.
A way to write the balance back to the file after each transaction.
A loop that interacts with users for their banking operations.
So, grab a coffee, and let’s get coding!
Step 2: Getting the Balance from File
Let’s start by fetching the balance. We’ll check if there’s an existing balance stored in a file called balance.txt
. If the file doesn’t exist, we’ll assume the account is brand new with a balance of zero (hey, we’re a bank, not a charity!).
Here's the code:
const accountBalanceFile = "balance.txt"
func getBalancefromFile() float64 {
balance, err := os.ReadFile(accountBalanceFile)
if err != nil {
// No file? No worries! Just start fresh.
return 0.0
}
accountBalance, _ := strconv.ParseFloat(string(balance), 64)
return accountBalance
}
Here’s what’s happening:
We try to read
balance.txt
.If it’s there, great! We convert the contents to a float.
If it’s not, we just return
0.0
because new accounts start empty. (Pro tip: Real banks don’t do this!)
Step 3: Saving the Balance Back to the File
Each time there’s a change, we’ll save the balance back to the file so it’s ready for the next time the user logs in. Let’s use writeToFile
to take care of this:
func writeToFile(accountBalance float64) {
os.WriteFile(accountBalanceFile, []byte(fmt.Sprintf("%.2f", accountBalance)), 0666)
}
We format the balance with two decimal places (no one wants to see 9.99999999
). This makes sure everything looks nice and tidy when saved. Also, setting permissions to 0666
is like saying, “Hey, anyone using this program can access this file.” (For serious apps, you’d probably want a bit more security!)
Step 4: Running the Bank – The Fun Part!
Let’s make it interactive. In main
, we’ll create a loop that greets the user, lets them check their balance, make deposits, withdraw money, or exit. We’ve got our choice structure, so they’ll know exactly what they’re doing (or maybe not – who knows with users, right?).
func main() {
accountBalance := getBalancefromFile()
for {
fmt.Println("Welcome to the bank!")
fmt.Println("What do you want to do?")
fmt.Println("1. Check your balance")
fmt.Println("2. Deposit")
fmt.Println("3. Withdraw")
fmt.Println("4. Exit")
var choice int
fmt.Scan(&choice)
switch choice {
case 1:
fmt.Println("Your balance is: ", accountBalance)
case 2:
fmt.Println("How much do you want to deposit?")
var depositAmount float64
fmt.Scan(&depositAmount)
if depositAmount <= 0 {
fmt.Println("Invalid amount")
continue
}
accountBalance += depositAmount
fmt.Println("Your new balance is: ", accountBalance)
case 3:
fmt.Println("How much do you want to withdraw?")
var withdrawAmount float64
fmt.Scan(&withdrawAmount)
if withdrawAmount > accountBalance {
fmt.Println("You don't have enough money!")
continue
}
if withdrawAmount <= 0 {
fmt.Println("Invalid amount")
continue
}
accountBalance -= withdrawAmount
fmt.Println("Your new balance is: ", accountBalance)
default:
fmt.Println("Thanks for banking with us. See you next time!")
return
}
writeToFile(accountBalance) // Update the balance after each transaction
}
}
Here’s the play-by-play:
The program welcomes the user and presents options. They can check their balance, deposit money, withdraw cash, or exit.
Each case in the
switch
does something fun:Check balance: Just displays the balance.
Deposit: Adds money to their account (but only if they enter a valid amount).
Withdraw: Takes out money, but only if they’ve got enough in there!
Every change gets saved to the file immediately with
writeToFile
, so the user can come back to exactly where they left off.
A Few Notes
Persistence: The balance sticks around in
balance.txt
, so the user’s money doesn’t vanish.Error Handling: We don’t handle every possible error here, but for a basic app, this keeps things simple.
User Experience: By prompting the user for each choice, we’re making sure they know exactly what they’re doing (and making it friendly and fun).
Wrapping Up
And there you have it! A super simple banking app in Go. You now know how to handle files, manage user input, and use a loop to keep the interaction going. As you can see, Go makes it easy to keep code clean, functional, and quick to write.
What’s next? Try adding more features, like transaction history or password protection. Or better yet, let’s open up this little bank to the “public” (your friends) and see how they enjoy managing their digital accounts!
Happy coding, and welcome to the banking business (kind of)!
Subscribe to my newsletter
Read articles from Rahul Das Sarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
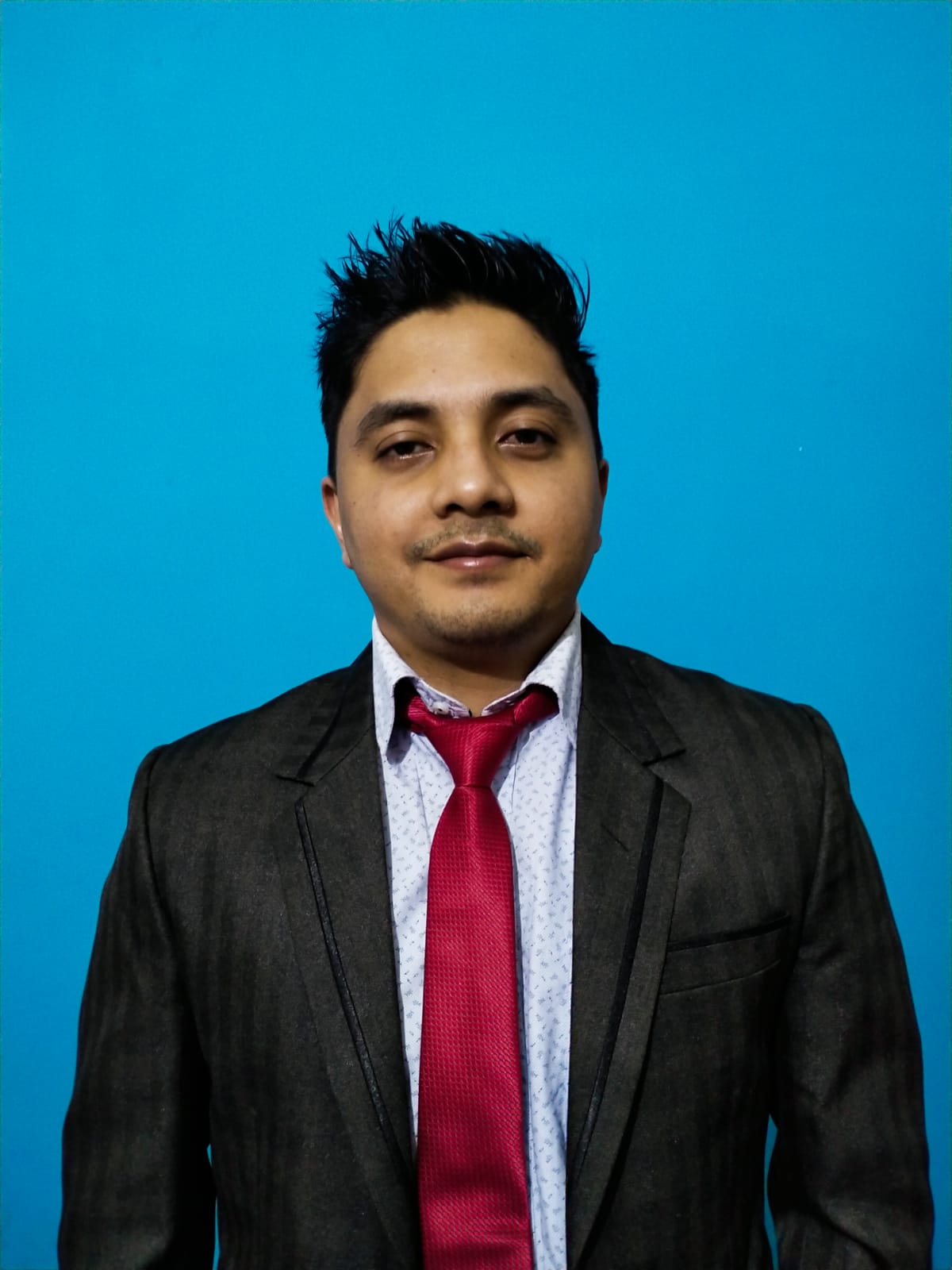
Rahul Das Sarma
Rahul Das Sarma
A react developer with dreams!