CRUD and Authentication Mock APIs with JSON Server
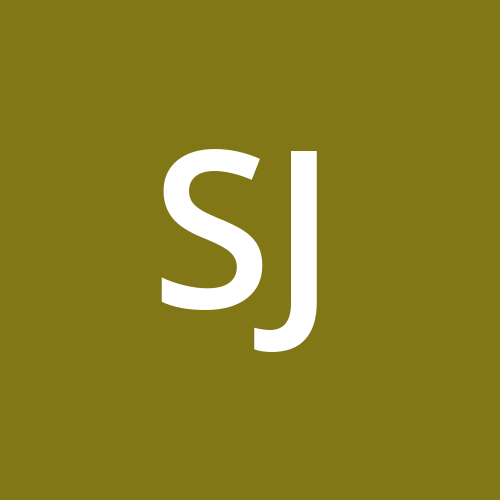
We can use JSON Server to mock an API that simulate adding, editing, and deleting user data in a development environment.
Steps to Set Up JSON Server for CRUD Mock API
Install JSON Server: JSON Server is a Node module that helps you create a REST API using a JSON file. First, install it globally:
npm install -g json-server
Create a JSON Database: Create a
db.json
file to act as your database.{ "users": [ { "id": 1, "email": "john.doe@example.com", "password": "123456", "name": "John Doe", "balance": { "current": 4836.00, "income": 3814.25, "expenses": 1700.50 }, "transactions": [ { "id": 1, "avatar": "./assets/images/avatars/emma-richardson.jpg", "name": "Emma Richardson", "category": "General", "date": "2024-08-19T14:23:11Z", "amount": 75.50, "recurring": false }, { "id": 2, "avatar": "./assets/images/avatars/savory-bites-bistro.jpg", "name": "Savory Bites Bistro", "category": "Dining Out", "date": "2024-08-19T20:23:11Z", "amount": -55.50, "recurring": false }, { "id": 3, "avatar": "./assets/images/avatars/daniel-carter.jpg", "name": "Daniel Carter", "category": "General", "date": "2024-08-18T09:45:32Z", "amount": -42.30, "recurring": false } ], "budgets": [ { "id": 1, "category": "Entertainment", "maximum": 50.00, "theme": "#277C78" }, { "id": 2, "category": "Bills", "maximum": 750.00, "theme": "#82C9D7" }, { "id": 3, "category": "Dining Out", "maximum": 75.00, "theme": "#F2CDAC" } ], "pots": [ { "id": 1, "name": "Savings", "target": 2000.00, "total": 159.00, "theme": "#277C78" }, { "id": 2, "name": "Concert Ticket", "target": 150.00, "total": 110.00, "theme": "#626070" }, { "id": 3, "name": "New Laptop", "target": 1000.00, "total": 10.00, "theme": "#F2CDAC" } ] }, { "id": 2, "email": "jane.smith@example.com", "password": "abcdef", "name": "Jane Smith", "balance": { "current": 5632.00, "income": 4120.25, "expenses": 1850.50 }, "transactions": [ { "id": 1, "avatar": "./assets/images/avatars/liam-hughes.jpg", "name": "Liam Hughes", "category": "Groceries", "date": "2024-08-15T18:20:33Z", "amount": 65.75, "recurring": false }, { "id": 2, "avatar": "./assets/images/avatars/green-plate-eatery.jpg", "name": "Green Plate Eatery", "category": "Groceries", "date": "2024-08-06T08:25:44Z", "amount": -78.50, "recurring": false }, { "id": 3, "avatar": "./assets/images/avatars/sofia-peterson.jpg", "name": "Sofia Peterson", "category": "Transportation", "date": "2024-08-08T08:55:17Z", "amount": -15.00, "recurring": false } ], "budgets": [ { "id": 1, "category": "Personal Care", "maximum": 100.00, "theme": "#626070" }, { "id": 2, "category": "Groceries", "maximum": 200.00, "theme": "#277C78" } ], "pots": [ { "id": 1, "name": "Vacation", "target": 1500.00, "total": 600.00, "theme": "#82C9D7" }, { "id": 2, "name": "New Phone", "target": 800.00, "total": 150.00, "theme": "#826CB0" } ] }, { "id": 3, "email": "sam.lee@example.com", "password": "password123", "name": "Sam Lee", "balance": { "current": 3200.00, "income": 2800.00, "expenses": 1400.00 }, "transactions": [ { "id": 1, "avatar": "./assets/images/avatars/sun-park.jpg", "name": "Sun Park", "category": "General", "date": "2024-08-17T16:12:05Z", "amount": 120.00, "recurring": false }, { "id": 2, "avatar": "./assets/images/avatars/mason-martinez.jpg", "name": "Mason Martinez", "category": "Lifestyle", "date": "2024-08-07T17:40:29Z", "amount": -35.25, "recurring": false }, { "id": 3, "avatar": "./assets/images/avatars/ethan-clark.jpg", "name": "Ethan Clark", "category": "Dining Out", "date": "2024-08-13T20:15:59Z", "amount": -32.50, "recurring": false } ], "budgets": [ { "id": 1, "category": "Lifestyle", "maximum": 150.00, "theme": "#F2CDAC" }, { "id": 2, "category": "Dining Out", "maximum": 100.00, "theme": "#F2A9D2" } ], "pots": [ { "id": 1, "name": "Emergency Fund", "target": 5000.00, "total": 1200.00, "theme": "#277C78" }, { "id": 2, "name": "Gym Membership", "target": 300.00, "total": 75.00, "theme": "#626070" } ] } ] }
In this setup:
Each user has a unique
id
and personal data.Transactions, budgets, and pots are nested arrays within each user and contain unique
id
fields for CRUD operations.
Start JSON Server: Start JSON Server and specify
db.json
as the database file:json-server --watch db.json --port 3000
This command will start a server at
http://localhost:3000
, where you can interact with your data via a REST API.API Endpoints:
Get all users:
GET /users
Get a single user:
GET /users/{userId}
Add a user:
POST /users
Edit a user:
PUT /users/{userId}
Delete a user:
DELETE /users/{userId}
Get a user’s transactions:
GET /users/{userId}/transactions
Add a transaction:
POST /users/{userId}/transactions
Edit a transaction:
PUT /users/{userId}/transactions/{transactionId}
Delete a transaction:
DELETE /users/{userId}/transactions/{transactionId}
Similarly, you can create, read, update, and delete items in budgets and pots with similar endpoints.
To simulate a real-world scenario for login and sign-up using JSON Server with JWT (JSON Web Tokens), we need to create endpoints for authentication and user management. Since JSON Server doesn’t directly support JWT, we’ll need to use a middleware like json-server-auth
to add JWT authentication functionality.
Step 1: Install JSON Server and JSON Server Auth
npm install -g json-server json-server-auth
Step 2: Set Up db.json
for Users and Sample Data
Modify the db.json
file to include users
, which will store user credentials and other data, such as balance
, transactions
, budgets
, and pots
.
Here’s an example of db.json
:
{
"users": [
{
"id": 1,
"email": "john.doe@example.com",
"password": "123456",
"name": "John Doe",
"balance": {
"current": 4836.00,
"income": 3814.25,
"expenses": 1700.50
},
"transactions": [
{
"id": 1,
"avatar": "./assets/images/avatars/emma-richardson.jpg",
"name": "Emma Richardson",
"category": "General",
"date": "2024-08-19T14:23:11Z",
"amount": 75.50,
"recurring": false
}
],
"budgets": [
{
"id": 1,
"category": "Entertainment",
"maximum": 50.00,
"theme": "#277C78"
}
],
"pots": [
{
"id": 1,
"name": "Savings",
"target": 2000.00,
"total": 159.00,
"theme": "#277C78"
}
]
},
{
"id": 2,
"email": "jane.smith@example.com",
"password": "abcdef",
"name": "Jane Smith",
"balance": {
"current": 5632.00,
"income": 4120.25,
"expenses": 1850.50
},
"transactions": [],
"budgets": [],
"pots": []
}
]
}
Note: Passwords are stored in plain text for demonstration purposes only. In a real-world application, they should be securely hashed.
Step 3: Configure JSON Server Auth Middleware
Create a new file, server.js
, to start JSON Server with JSON Server Auth.
// server.js
const jsonServer = require('json-server');
const auth = require('json-server-auth');
const server = jsonServer.create();
const router = jsonServer.router('db.json');
const middlewares = jsonServer.defaults();
server.db = router.db;
// Apply the auth middleware
server.use(middlewares);
server.use(auth);
server.use(router);
server.listen(3000, () => {
console.log('JSON Server is running on http://localhost:3000');
});
Step 4: Start the Server
Run the custom server:
node server.js
Step 5: Available Authentication Endpoints
JSON Server Auth provides default authentication endpoints for registration and login:
Sign Up (Register):
POST /register
Login:
POST /login
Using the Endpoints with Example Requests
1. Sign Up (Register)
To register a new user, send a POST request to /register
with the required data (e.g., email
, password
, name
).
Request:
POST http://localhost:3000/register
Content-Type: application/json
{
"email": "new.user@example.com",
"password": "securepassword",
"name": "New User"
}
Response:
The server will return a JWT token if the registration is successful.
{
"accessToken": "<JWT_TOKEN>",
"user": {
"email": "new.user@example.com",
"name": "New User",
"id": 3
}
}
2. Login
To authenticate an existing user, send a POST request to /login
with the user's credentials.
Request:
POST http://localhost:3000/login
Content-Type: application/json
{
"email": "john.doe@example.com",
"password": "123456"
}
Response:
If the credentials are valid, the server will return a JWT token:
{
"accessToken": "<JWT_TOKEN>",
"user": {
"email": "john.doe@example.com",
"name": "John Doe",
"id": 1
}
}
Step 6: Access Protected Routes
You can access the users' data by including the token in the Authorization
header as a Bearer token.
Example: Fetch a user’s transactions:
GET http://localhost:3000/users/1/transactions
Authorization: Bearer <JWT_TOKEN>
Summary of the Setup
Authentication: Users can register and log in, receiving JWT tokens on successful authentication.
Data Segmentation: Each user has personal data, such as
balance
,transactions
,budgets
, andpots
.JWT Authentication: Protects routes, allowing only authenticated users to access or modify data.
This setup with JSON Server and JSON Server Auth provides a realistic environment for managing user authentication and simulating a full CRUD-based application.
Subscribe to my newsletter
Read articles from Sumaya J. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
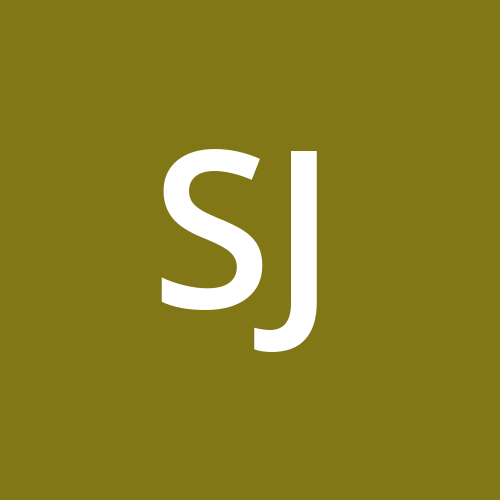