Code Design: Chapter 2 - Development Methodologies

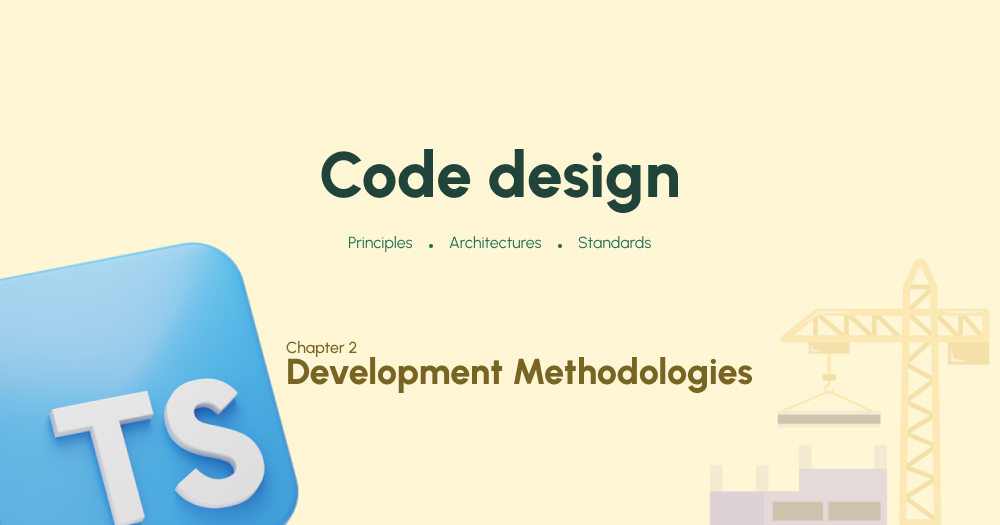
Introduction
Software development methodologies help organize and optimize the process of building software. They shape how teams approach coding, testing, and collaboration, ultimately leading to higher-quality software with lower maintenance costs. Here’s a breakdown of key methodologies with their core concepts and benefits.
TDD (Test-Driven Development
Write tests before coding each feature or function, building code around the tests to meet predefined expectations.
Benefits: Reduces bugs, promotes a test-first mindset, and encourages a high standard of code quality.
// Test (written before code)
test('should add two numbers', () => {
expect(add(2, 3)).toBe(5);
});
// Code (written to pass the test)
function add(a: number, b: number): number {
return a + b;
}
BDD (Behavior-Driven Development)
Focuses on the behavior of an application from the user’s perspective, using readable scenarios to outline expected behaviors.
Benefits: Enhances collaboration between technical and non-technical team members by using human-readable tests that bridge the communication gap.
// Scenario written in a readable format (BDD style)
// "Given a user logs in, when they add an item to the cart, then the cart count should increase by 1."
test('should increase cart count by 1 when an item is added', () => {
user.login();
user.addToCart(item);
expect(user.cart.count).toBe(1);
});
DDD (Domain-Driven Design)
Models software closely around the business domain, making the code reflect the business logic and structure. DDD is especially useful in complex applications.
Key Concepts: Domains, sub-domains, entities, and value objects help create a shared language between developers and domain experts.
Benefits: Keeps code aligned with business needs, making it easier to adapt and manage complex systems over time.
// Defining a 'Student' as an entity with unique characteristics relevant to the domain.
class Student extends User {
constructor(public email: string, public name: string) {}
joinGroup(groupID: string) {
// Logic to attch the student to a group
}
}
const groupA = new Group('group-a');
const student = new Student('alice@student.com', 'Alice');
student.joinGroup(groupA.id);
Event-Driven Development
Organizes applications around events that trigger actions in response to specific occurrences (e.g., user actions or system updates), making components decoupled and responsive to state changes.
Benefits: Increases scalability and flexibility, especially in complex workflows or real-time applications.
This kind of architecture is used in Real-time NoSQL databases like Firebase.
// Event-based system example
interface Event {
eventSignature: string;
data: any;
}
class EventBus {
private listeners: { [signature: string]: Function[] } = {};
subscribe(eventSignature: string, callback: Function) {
if (!this.listeners[eventSignature]) this.listeners[eventSignature] = [];
this.listeners[eventSignature].push(callback);
}
publish(event: Event) {
const callbacks = this.listeners[event.eventSignature];
if (callbacks) callbacks.forEach(callback => callback(event.data));
}
}
const eventBus = new EventBus();
// It will only be triggred when it's published
eventBus.subscribe('userRegistered', (data) => {
console.log(`Welcome email sent to ${data.email}`);
});
eventBus.publish({ type: 'userRegistered', data: { email: 'user@example.com' } });
Summary
Each of these methodologies brings unique advantages, supporting developers and teams to manage code quality, complexity, and collaboration. TDD and BDD enforce a testing-first approach, helping teams catch issues early. DDD aligns code with the business, making it more comprehensible and adaptable. Event-Driven Development improves system flexibility, allowing for highly responsive and decoupled components. Together, they form a toolkit that can adapt to various project needs and complexities, especially in large, dynamic applications.
Series Chapters
Subscribe to my newsletter
Read articles from Mehdi Jai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mehdi Jai
Mehdi Jai
Result-oriented Lead Full-Stack Web Developer & UI/UX designer with 5+ years of experience in designing, developing and deploying web applications.