Advanced Array Methods: Mapping the Future!
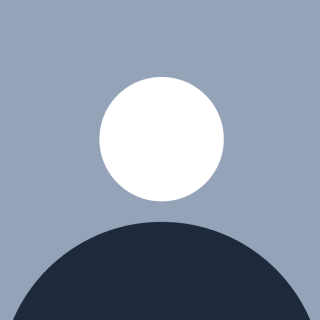
Table of contents
- Map, Filter, and Reduce Explained
- Using Map to Transform Your Journey
- Filtering Out the Noise
- Flowchart: Array Transformation Process
- Reduce — Summarizing Your Adventure
- Transforming Arrays with Power
- Advanced Array Transformations
- Challenge Time — Create a Simple Budget Tracker
- Additional Challenges for Mastery
- Conclusion — Powerful Array Transformations
- Let’s explore Maps and Sets next!
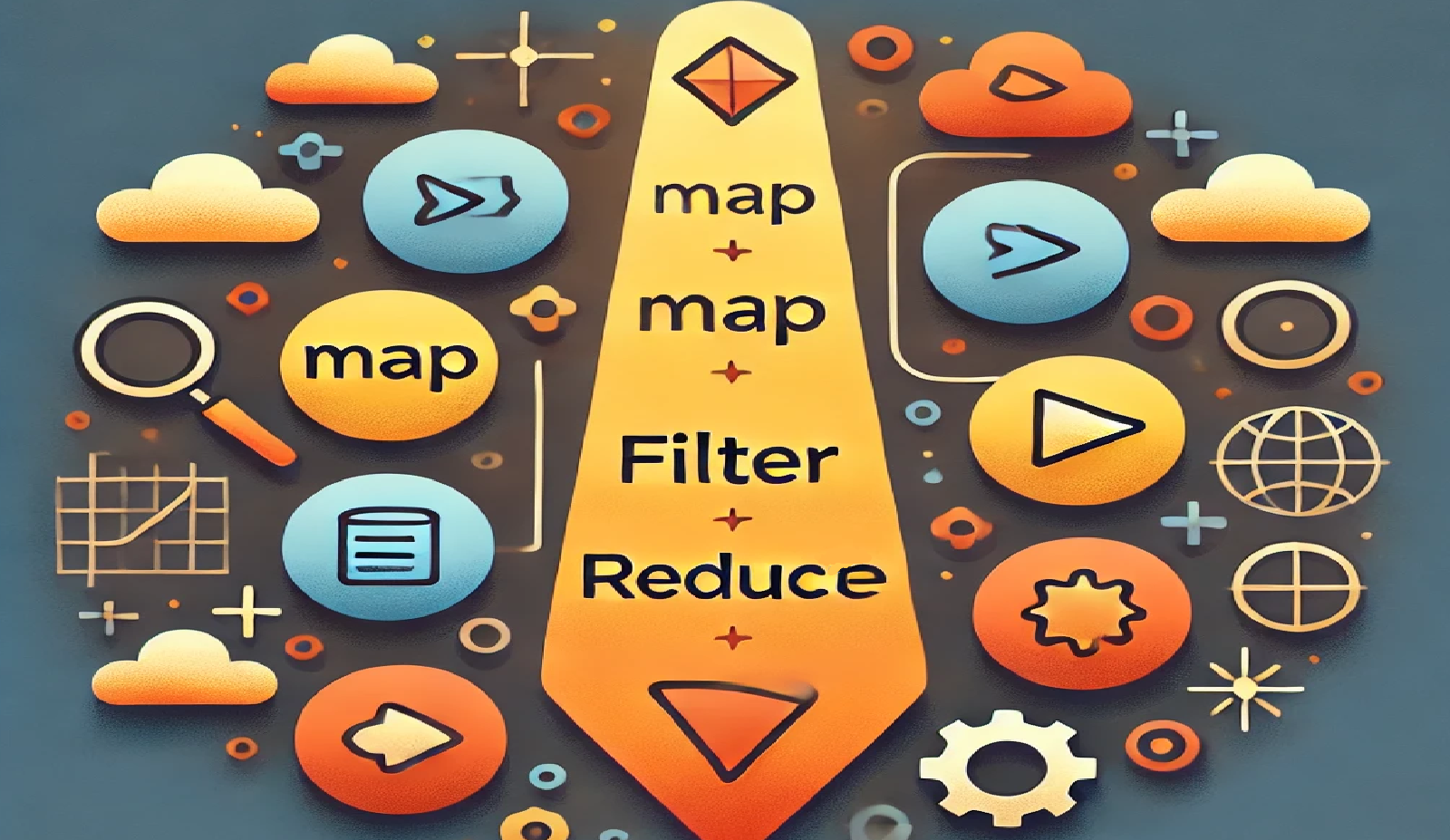
Imagine you’re about to embark on an epic journey, where you can shape the world around you. You’re given a map, and along the way, you decide which paths to take, what to gather, and how to calculate the resources you collect. In JavaScript, advanced array methods like map, filter, and reduce give you the power to transform, filter, and summarize arrays—much like navigating through and transforming your journey.
Arrays in JavaScript are more than just simple lists. With these advanced methods, you can reshape data, extract meaningful pieces, and generate entirely new arrays from existing ones. These methods allow you to take your JavaScript skills to the next level, whether you're processing large datasets or optimizing your code.
Let’s start the adventure by exploring map(), filter(), and reduce()—the three powerful tools that will help you map out and manipulate your arrays with precision.
Map, Filter, and Reduce Explained
Before we dive into the examples, let’s understand the purpose of each method:
map(): Transforms each element of an array into a new value, creating a new array of the same size.
filter(): Selects elements from an array that meet certain criteria, creating a new array with the filtered elements.
reduce(): Accumulates values from an array to produce a single result, such as a sum, product, or even an object.
Think of these methods as different tools in your adventurer’s backpack, each with a specific function for navigating through your array-based journey.
Using Map to Transform Your Journey
The map() method allows you to create a new array by applying a function to each element of an existing array. Imagine you’re mapping out your route for a journey, and for each destination you visit, you collect a new piece of information.
Code Snippet: Map Example
Let’s say you have an array of distances (in kilometers) between various locations on your journey. You want to convert these distances to miles:
let distancesKm = [10, 25, 50, 100]; // Distances in kilometers
let distancesMiles = distancesKm.map(distance => distance * 0.621371); // Convert to miles
console.log(distancesMiles); // Output: [6.21371, 15.534275, 31.06855, 62.1371]
Explanation:
The
map()
method transforms each distance in kilometers to miles using a conversion factor.The result is a new array,
distancesMiles
, containing the converted distances.
Filtering Out the Noise
The filter() method is like a pathfinder—it helps you select only the relevant elements from an array, filtering out everything else that doesn’t meet the criteria. Imagine you’re collecting supplies along your journey, but you only want to keep the useful ones.
Code Snippet: Filter Example
Let’s say you have an array of items you’ve gathered, and you only want to keep the items that are classified as "essential":
let items = [
{ name: "Map", type: "essential" },
{ name: "Water Bottle", type: "essential" },
{ name: "Compass", type: "essential" },
{ name: "Sunglasses", type: "optional" }
];
let essentialItems = items.filter(item => item.type === "essential");
console.log(essentialItems); // Output: [{name: "Map", type: "essential"}, {name: "Water Bottle", type: "essential"}, {name: "Compass", type: "essential"}]
Explanation:
The
filter()
method creates a new array by selecting only the items that are labeled as "essential".This is useful when you need to extract a subset of data from a larger array.
Flowchart: Array Transformation Process
Let’s visualize the journey of an array as it goes through map(), filter(), and reduce(). Imagine the following flowchart showing how each method transforms the array:
[ Original Array ]
|
[ map() Transformation ]
|
[ filter() Selection ]
|
[ reduce() Accumulation ]
map(): Transforms every element into something new.
filter(): Selects only the elements that meet specific criteria.
reduce(): Accumulates or summarizes the transformed and filtered elements into a single value.
Reduce — Summarizing Your Adventure
The reduce() method is like summarizing your entire journey into one meaningful result. Whether you’re counting supplies, totaling distances, or calculating expenses, reduce()
helps you condense an entire array into a single value.
Code Snippet: Reduce Example
Imagine you’re summing up the total distance you’ve traveled on your journey:
let distancesKm = [10, 25, 50, 100];
let totalDistance = distancesKm.reduce((total, distance) => total + distance, 0);
console.log(totalDistance); // Output: 185
Explanation:
The
reduce()
method adds up all the distances in the array and returns the total.The second argument (
0
) is the initial value for the accumulator (total
).
Transforming Arrays with Power
In this first part, you’ve learned how to wield the power of map(), filter(), and reduce() to transform arrays. These methods give you the ability to reshape data, extract useful elements, and summarize values with ease—essential tools for any JavaScript developer.
In the next part, we’ll dive deeper into practical challenges and more complex transformations, helping you master these advanced array methods in real-world scenarios.
Advanced Array Transformations
Now that you understand the basics of map(), filter(), and reduce(), let’s explore how these methods can be combined to perform more complex transformations. By chaining these methods together, you can create powerful pipelines that transform your arrays in a single, concise flow—just like mapping out your entire journey from start to finish.
Combining Map, Filter, and Reduce
Imagine you’re planning a trip, and you need to prepare a list of items for your journey. Each item has a price, but you only want to take the items marked as "essential," and you need to calculate the total cost.
Let’s combine map(), filter(), and reduce() to achieve this:
let items = [
{ name: "Map", type: "essential", price: 10 },
{ name: "Water Bottle", type: "essential", price: 15 },
{ name: "Compass", type: "essential", price: 20 },
{ name: "Sunglasses", type: "optional", price: 25 }
];
// Step 1: Filter for essential items
let essentialItems = items.filter(item => item.type === "essential");
// Step 2: Map to extract only the price
let itemPrices = essentialItems.map(item => item.price);
// Step 3: Reduce to calculate total price
let totalPrice = itemPrices.reduce((total, price) => total + price, 0);
console.log(totalPrice); // Output: 45
Explanation:
We first filter out non-essential items using
filter()
.Then we use
map()
to create an array of prices.Finally, we use
reduce()
to sum up the total cost of the essential items.
This combination allows you to manipulate data in a clear, streamlined way.
Challenge Time — Create a Simple Budget Tracker
Now it’s time to put your skills to the test. In this challenge, you’ll create a simple budget tracker using advanced array methods to manage and calculate expenses.
Challenge: Build a Budget Tracker
Step 1: Create an array of objects where each object represents an expense with a name, type (e.g., "food", "entertainment"), and amount.
Step 2: Use filter() to filter out only the essential expenses.
Step 3: Use reduce() to calculate the total amount of essential expenses.
Here’s a starter example for your budget tracker:
let expenses = [
{ name: "Groceries", type: "essential", amount: 50 },
{ name: "Movies", type: "entertainment", amount: 20 },
{ name: "Rent", type: "essential", amount: 500 },
{ name: "Concert Tickets", type: "entertainment", amount: 100 }
];
// Step 1: Filter essential expenses
let essentialExpenses = expenses.filter(expense => expense.type === "essential");
// Step 2: Calculate total amount
let totalEssential = essentialExpenses.reduce((total, expense) => total + expense.amount, 0);
console.log("Total Essential Expenses: $" + totalEssential); // Output: $550
Additional Challenges for Mastery
Here are a few more challenges to further enhance your understanding of map(), filter(), and reduce():
Challenge 1: Double the Numbers
Scenario: You have an array of numbers, and you want to create a new array where each number is doubled using map().
Example:
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Challenge 2: Filter for Adults
Scenario: You have an array of people with their ages, and you want to filter out only those who are 18 or older using filter().
Example:
let people = [
{ name: "Alice", age: 17 },
{ name: "Bob", age: 22 },
{ name: "Charlie", age: 19 }
];
let adults = people.filter(person => person.age >= 18);
console.log(adults); // Output: [{ name: "Bob", age: 22 }, { name: "Charlie", age: 19 }]
Challenge 3: Sum of Even Numbers
Scenario: You want to calculate the sum of all even numbers in an array using reduce() and filter().
Example:
let numbers = [1, 2, 3, 4, 5, 6];
let sumOfEvens = numbers
.filter(num => num % 2 === 0) // Filter out even numbers
.reduce((total, num) => total + num, 0); // Sum the even numbers
console.log(sumOfEvens); // Output: 12
Conclusion — Powerful Array Transformations
In this article, you’ve explored how map(), filter(), and reduce() are powerful tools that allow you to reshape, filter, and summarize arrays with ease. These methods are essential for handling complex data transformations in JavaScript, whether you're calculating totals, filtering important elements, or generating new arrays from existing data.
By mastering these advanced methods, you gain the ability to process and manipulate data efficiently—whether you’re building a budget tracker, analyzing datasets, or optimizing your code.
Let’s explore Maps and Sets next!
In the next article, we’ll explore Maps and Sets—two powerful data structures that allow you to store unique values, map keys to values, and handle collections in even more advanced ways. Stay tuned for more exciting JavaScript insights!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by