Day 14: Mastering Error Handling in JavaScript
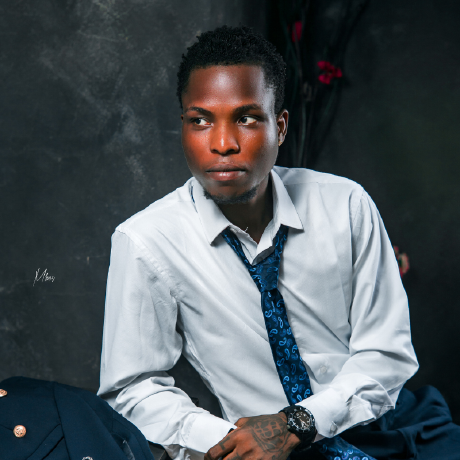
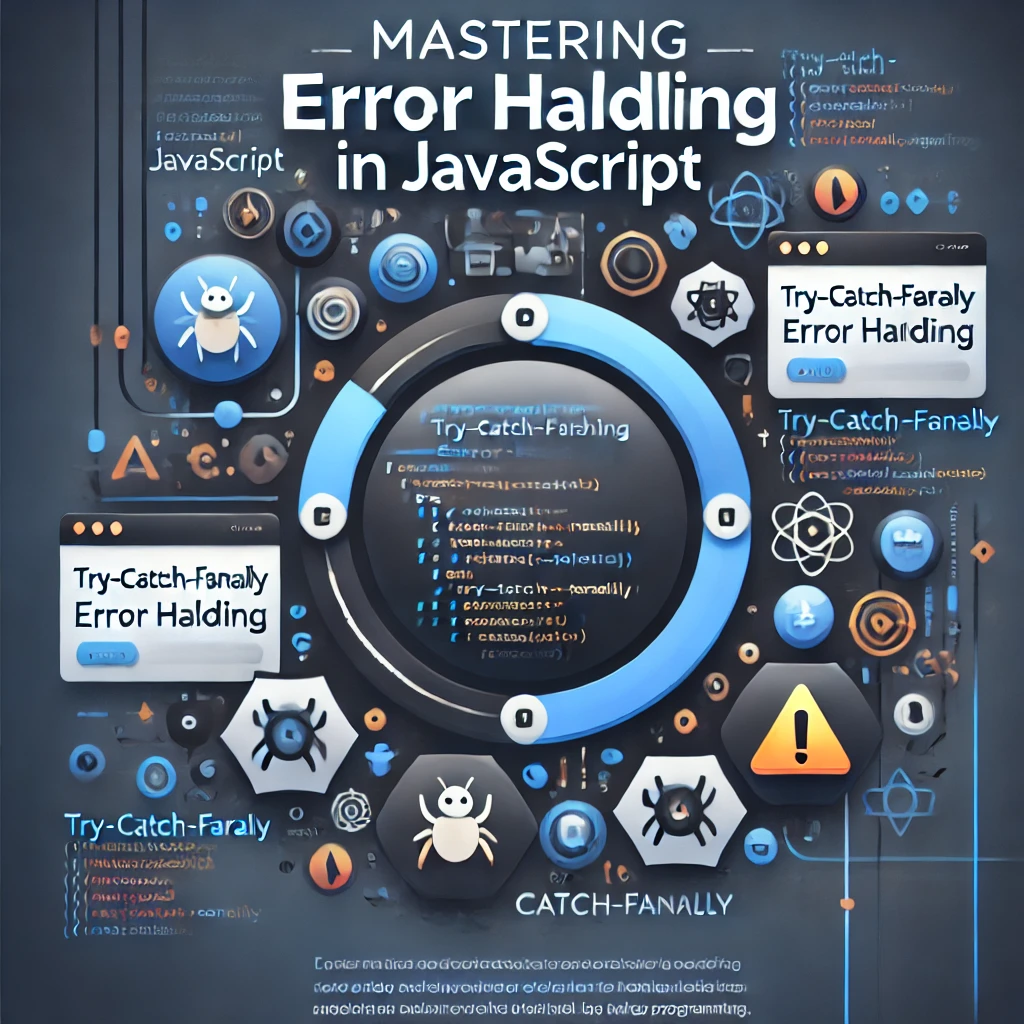
Welcome to Day 14 of our #30DaysOfJavaScript journey! Today, we’re tackling a crucial topic for any developer: error handling. This guide will walk you through the essentials of handling, identifying, and troubleshooting errors in JavaScript, so your code runs more reliably and predictably.
What is Error Handling in JavaScript?
Error handling refers to the techniques and methods used to manage, log, and respond to runtime issues that arise while a program executes. JavaScript provides specific tools for error management, allowing you to handle unexpected situations without crashing the entire application.
Common Types of Errors in JavaScript
Syntax Errors: Mistakes in the code syntax that prevent the script from running.
Runtime Errors: Errors that occur during code execution, often due to unexpected input or system conditions.
Logical Errors: Flaws in the code logic that result in incorrect outcomes without necessarily throwing an error.
How Do We Handle Errors in JavaScript?
JavaScript provides structures like try...catch
, throw
, and finally
to handle errors:
try...catch
: The code inside thetry
block executes normally, but if an error occurs, control transfers to thecatch
block, where you can handle the error.throw
: You can manually create an error by using thethrow
keyword.finally
: This block runs aftertry
andcatch
, regardless of the outcome, and is useful for cleanup tasks.
Example:
try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error("An error occurred:", error.message);
} finally {
console.log("Execution completed.");
}
Testing Error Handling in JavaScript
To ensure your error handling works, test for different scenarios:
Input Validation: Pass invalid or unexpected inputs to check if your error handling catches them.
Boundary Cases: Test your code with edge cases, such as extreme values.
Mocking Errors: Use tools like Sinon or Jest to simulate errors and validate your handling approach.
The Importance of Effective Error Handling
Proper error handling keeps your applications user-friendly and secure by:
Ensuring that users see friendly error messages rather than technical issues.
They are allowing applications to continue running or recover gracefully.
Preventing potential security risks by handling unexpected or malicious input.
Tips for Error Handling
Use Specific Error Messages: Ensure error messages are descriptive to help with debugging.
Fail Gracefully: Ensure that errors don't break the entire app.
Log Errors: Use tools like Sentry or LogRocket to monitor and analyze errors.
Document Known Issues: Maintaining documentation helps prevent and quickly resolve recurring errors.
Wrapping Up
Mastering error handling will make you a more resilient and reliable JavaScript developer! Embrace errors as opportunities to improve your code, and your projects will become more robust and user-friendly.
Stay tuned for tomorrow's lesson, where we’ll explore JSON handling in JavaScript and learn how to parse and format JSON data for seamless data exchange.
Happy coding!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
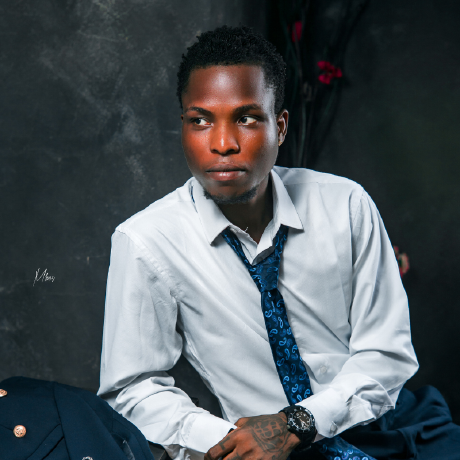
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com