Unleashing the Power of Neural Networks in Artificial Intelligence

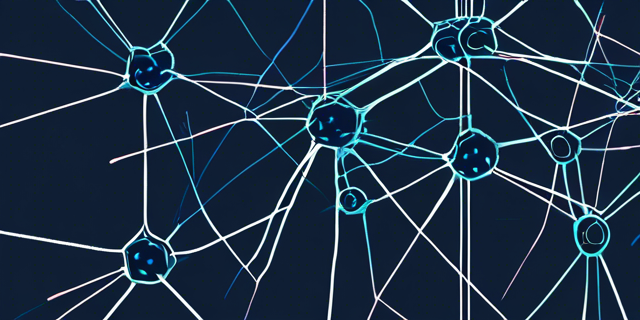
In recent years, Artificial Intelligence (AI) has achieved unprecedented advancements, with neural networks serving as a cornerstone of many breakthroughs. These models are transforming industries like healthcare, finance, and transportation, enabling innovations such as self-driving cars, facial recognition, and natural language processing (NLP). Neural networks empower machines to learn from data, recognize patterns, and make decisions in ways previously deemed impossible.
This comprehensive guide will delve into the role of neural networks in AI, how they emulate the human brain's functions, and provide a hands-on example of constructing a neural network using Python along with the powerful libraries TensorFlow and Keras. By the end of this article, you will gain a solid understanding of how neural networks operate and how to implement them for practical applications.
What Are Neural Networks?
Neural networks are a class of machine learning models inspired by the structure and function of the human brain. They consist of interconnected layers of nodes (neurons), each responsible for processing information and contributing to predictions. These models form the foundation of deep learning, a subset of machine learning that has revolutionized fields such as computer vision, speech recognition, and autonomous systems.
Biological Inspiration
Neural networks draw inspiration from the human brain, where billions of neurons interconnect to process sensory information, generate thoughts, and make decisions. Each biological neuron receives input signals from neighboring neurons through synapses. When the cumulative input surpasses a specific threshold, the neuron "fires," sending output signals to other neurons. This intricate process facilitates complex computations and learning within the brain.
In artificial neural networks (ANNs), neurons are represented as mathematical functions that process input data, while the connections between them are represented by weights. These weights are adjusted during training, allowing the network to learn patterns from the data.
Key Components of a Neural Network
To better understand how neural networks function, let's explore their essential components:
Neuron (Node): A mathematical function that receives one or more inputs, processes them, and generates an output. The output is typically passed through an activation function, determining whether the neuron should "fire."
Weights: These represent the strength of connections between neurons. Each input to a neuron is multiplied by its corresponding weight, learned during training. Adjustments to these weights minimize the difference between predicted outputs and actual targets.
Bias: Each neuron has a bias term that allows the model to better fit the data by shifting the output of the activation function.
Activation Function: This function defines the output of a neuron. Common activation functions include Sigmoid, ReLU (Rectified Linear Unit), and Tanh. The choice of activation function significantly impacts the network's ability to model complex, non-linear relationships.
Layers: Neural networks consist of multiple layers of neurons:
Input Layer: The first layer that receives the raw input data.
Hidden Layers: One or more layers that process data and learn intermediate representations.
Output Layer: The final layer that produces the predicted output.
Forward Propagation: This process involves data flowing from the input layer through hidden layers to the output layer. Each neuron receives input, processes it, and passes the output to the next layer.
Loss Function: The loss function quantifies the difference between predicted outputs and true target values. This difference is used to adjust the network's weights during training.
Backpropagation: This is the method by which the neural network learns through weight adjustments. After calculating the error from the loss function, the algorithm works backward from the output layer to update the weights according to their contribution to the error.
The Role of Neural Networks in AI
Neural networks are pivotal in AI because they excel at tasks where traditional algorithms falter, such as image recognition, natural language processing, and time series prediction. These tasks involve complex patterns and non-linear relationships that neural networks effectively model.
Neural networks' true power lies in their ability to autonomously learn features from raw data. For example:
In computer vision, neural networks identify objects in images by learning hierarchical features—from detecting edges in earlier layers to recognizing more abstract patterns like faces or animals in deeper layers.
In speech recognition, neural networks learn to map audio signals to text, facilitating applications like voice assistants and transcription services.
In natural language processing (NLP), they analyze and comprehend human language, enabling AI to perform tasks such as language translation, sentiment analysis, and text generation.
Types of Neural Networks
Neural networks come in various architectures, each tailored to specific problem types. Some common neural network types include:
Feedforward Neural Networks (FNN): The simplest type, where data flows from the input layer to the output layer without loops or cycles. FNNs are widely used for classification and regression tasks.
Convolutional Neural Networks (CNN): Specialized for image and video processing, CNNs employ convolutional layers to automatically extract features from images, such as edges and objects. They are commonly used in object detection and image classification tasks.
Recurrent Neural Networks (RNN): Designed for sequential data, such as time series or natural language, RNNs maintain a memory of previous inputs. They are used in speech recognition and machine translation tasks.
Generative Adversarial Networks (GANs): Comprising two networks—a generator and a discriminator—that compete against each other, GANs are employed for generating new data, such as images or text.
Autoencoders: Unsupervised neural networks used for data compression and reconstruction, useful for tasks like dimensionality reduction and anomaly detection.
How Neural Networks Mimic the Human Brain
Neural networks reflect certain aspects of the human brain's structure and function, despite significant differences between biological and artificial neurons. The human brain contains approximately 86 billion neurons, each capable of forming thousands of synaptic connections, enabling complex information processing, learning from experiences, and decision-making.
While artificial neural networks are simplified models, they emulate key aspects of neural processing:
Learning from Experience: Like humans learn from experience, neural networks learn from data. As the model trains, it adjusts its weights to minimize errors, leading to more accurate predictions in the future.
Parallel Processing: Neurons in the brain operate in parallel, processing information simultaneously from various sources. Similarly, neural networks handle data in parallel across multiple layers, enabling efficient large-scale problem-solving.
Generalization: Neural networks generalize from training data, allowing them to make predictions on new, unseen data—akin to how humans apply past experiences to novel situations.
Hierarchical Learning: The brain learns hierarchically, processing simple information in early stages and more complex information in later stages. Neural networks also follow a hierarchical learning structure, with early layers detecting simple patterns and deeper layers recognizing complex features.
Despite these similarities, artificial neural networks are far less complex than the human brain. Nevertheless, they have proven remarkably effective for specific tasks, particularly in pattern recognition and decision-making.
Building Neural Networks in Python: A Practical Example
Now that we have covered the theoretical foundations of neural networks, let’s proceed to a practical example. We will construct a neural network using Python, leveraging TensorFlow and Keras—two of the most popular deep learning libraries.
Setting Up the Environment
Ensure that Python is installed on your system along with the required libraries. You can install TensorFlow and Keras using the following command:
bashCopy codepip install tensorflow
Loading the MNIST Dataset
We will begin by loading the MNIST dataset, which is conveniently included in the tensorflow.keras.datasets
module.
pythonCopy codeimport tensorflow as tf
from tensorflow.keras import layers, models
from tensorflow.keras.datasets import mnist
# Load the MNIST dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize the data to the range [0, 1]
x_train, x_test = x_train / 255.0, x_test / 255.0
In the code snippet above, we load the dataset and normalize the pixel values of the images to the range [0, 1]. Normalization enhances training efficiency.
Building the Neural Network
Next, we will define the architecture of our neural network. We’ll implement a simple feedforward neural network with two hidden layers and an output layer for classification.
pythonCopy code# Build a simple feedforward neural network
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)), # Flatten the input image (28x28) into a 1D array
layers.Dense(128, activation='relu'), # First hidden layer with 128 neurons and ReLU activation
layers.Dense(64, activation='relu'), # Second hidden layer with 64 neurons and ReLU activation
layers.Dense(10, activation='softmax') # Output layer with 10 neurons (one for each digit) and softmax activation
])
Layer Overview:
Flatten Layer: Converts the 28x28 image into a 1D array of 784 pixels, suitable for feeding into fully connected layers.
Dense Layers: Fully connected layers with ReLU activation, which introduce non-linearity. The first hidden layer has 128 neurons, while the second has 64.
Output Layer: A fully connected layer with 10 neurons (representing each digit class) and softmax activation to convert outputs into probabilities.
Compiling the Model
Once the architecture is defined, the next step is to compile the model. During this process, we specify the optimizer, loss function, and metrics that will be used to evaluate the model's performance.
pythonCopy code# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Optimizer: We employ the Adam optimizer, which is an adaptive learning rate optimizer commonly used in deep learning. It adjusts the learning rate during training to improve convergence.
Loss Function: Given that this is a multi-class classification problem, we utilize
sparse_categorical_crossentropy
as the loss function. This function measures the discrepancy between the predicted and true target values.Metrics: We track the accuracy metric to monitor the model's performance throughout training and evaluation.
Training the Model
Now that the model has been compiled, we can proceed to train it using the training dataset. During this phase, the model learns to classify images by adjusting its weights to minimize the loss function.
pythonCopy code# Train the model
model.fit(x_train, y_train, epochs=5)
In this example, we train the model for 5 epochs, meaning it will pass through the entire training dataset five times, updating its weights after each pass.
Evaluating the Model
After training, we evaluate the model's performance on the test dataset, which it has not encountered before. This evaluation helps us assess how well the model generalizes to new data.
pythonCopy code# Evaluate the model on the test data
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"Test accuracy: {test_acc:.2f}")
The evaluate()
function computes both the loss and accuracy on the test data. Here, we print the test accuracy to determine how well the model performs.
Interpreting the Results
The model's performance is assessed based on its accuracy on the test dataset. If the accuracy is high (e.g., above 95%), it indicates that the model has effectively learned to classify the digits. Conversely, a low accuracy may suggest that the model requires further tuning, such as adjustments to the number of layers, neurons, or hyperparameters like the learning rate.
Common Issues in Neural Network Training
Several challenges may arise during neural network training, including:
Overfitting: Overfitting occurs when the model performs well on training data but poorly on test data, often due to excessive complexity (e.g., too many layers or neurons). Regularization techniques, such as dropout or L2 regularization, can help mitigate this issue.
Underfitting: Underfitting happens when the model performs poorly on both training and test data, typically due to insufficient complexity. Addressing underfitting may involve increasing the model's complexity or extending the training duration.
Learning Rate: Selecting an appropriate learning rate is crucial. A rate that is too high may lead to rapid convergence to a suboptimal solution, while a rate that is too low may slow down training or prevent convergence altogether. The Adam optimizer dynamically adjusts the learning rate but may still require fine-tuning.
Advanced Neural Network Techniques
After mastering the basics of building simple neural networks, consider exploring advanced techniques to enhance model performance:
Convolutional Neural Networks (CNNs): Specially designed for image processing, CNNs excel in tasks like object detection and facial recognition by utilizing convolutional layers to automatically learn spatial hierarchies of features.
Recurrent Neural Networks (RNNs): Ideal for sequential data (e.g., time series or text), RNNs maintain memory of previous inputs. Variants like Long Short-Term Memory (LSTM) networks effectively capture long-term dependencies in sequences.
Transfer Learning: This technique involves using a pre-trained model (e.g., VGG16 or ResNet) as a starting point for a new task. By leveraging knowledge gained from previous tasks, transfer learning can save time and resources.
Dropout: As a regularization technique, dropout randomly deactivates neurons during training to reduce reliance on specific neurons, thereby helping to prevent overfitting.
Batch Normalization: This technique normalizes layer inputs, which can accelerate training and improve overall model performance.
Subscribe to my newsletter
Read articles from Temitope Ologunbaba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Temitope Ologunbaba
Temitope Ologunbaba
I am Temitope Ologunbaba, a software engineer with 5 years of experience specializing in developing innovative solutions and enhancing user experiences built over 30 apps on both playstore and app store, and 2 models currently being used by the companies in app for giving their user better experience. Proficient in Python, Java and Dart(Flutter). As an enthusiast of machine learning and artificial intelligence, I am passionate about leveraging technology to derive insights and improve decision-making processes. My expertise allows me to understand user needs and preferences deeply, facilitating effective collaboration with cross-functional teams to deliver diverse perspectives on projects. I am committed to continuous learning and applying my skills to drive impactful solutions in the tech industry.