What is PHP Programming & Basic PHP Scripts

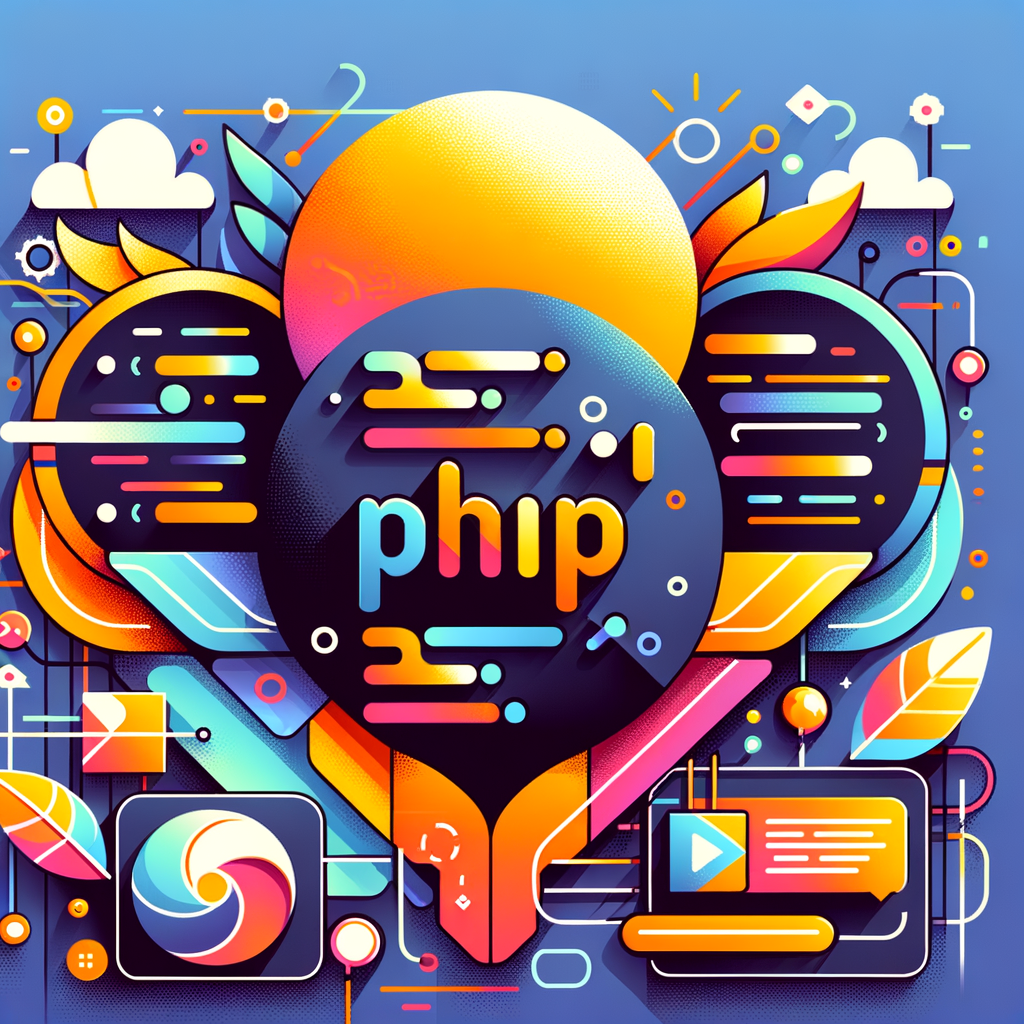
What is PHP Programming & Basic PHP Scripts
PHP, which stands for "Hypertext Preprocessor," is a widely-used open-source scripting language that is especially suited for web development. It is embedded within HTML and is executed on the server, making it a powerful tool for creating dynamic and interactive web pages. PHP is known for its simplicity, speed, and flexibility, which have contributed to its popularity among developers.
PHP programming involves writing scripts that can perform a variety of tasks, such as processing form data, generating dynamic page content, sending and receiving cookies, and interacting with databases. These capabilities make PHP an essential component of many web applications and websites.
Basic PHP scripts typically start with the <?php
tag and end with the ?>
tag. Within these tags, you can write PHP code to perform various operations. For example, a simple PHP script might display a message on a web page using the echo
statement:
<?php
echo "Hello, World!";
?>
This script outputs the text "Hello, World!" to the web page. PHP scripts can also include variables, control structures like loops and conditionals, and functions to perform more complex tasks. Here's an example of a PHP script that uses a variable and a loop:
<?php
$counter = 1;
while ($counter <= 5) {
echo "This is loop iteration number: $counter<br>";
$counter++;
}
?>
In this script, a variable $counter
is initialized to 1, and a while
loop is used to print a message five times, incrementing the counter with each iteration.
Overall, PHP programming offers a robust and versatile platform for building web applications, and understanding basic PHP scripts is the first step in leveraging its full potential.
PHP (Hypertext Preprocessor) is a widely-used, open-source scripting language primarily designed for web development. It allows developers to create dynamic web pages that can interact with databases and perform various server-side functions. PHP is embedded within HTML code and can be executed on the server, generating HTML that is sent to the client's browser.
Key Features of PHP:
Server-Side Scripting: PHP runs on the server, processing requests and generating dynamic content.
Database Integration: Easily connects to databases (like MySQL) for data management.
Cross-Platform: Works on various operating systems, including Windows, Linux, and macOS.
Open Source: Free to use and has a large community for support.
Easy to Learn: Syntax is simple, making it accessible for beginners.
Basic PHP Syntax:
Here's a simple PHP script demonstrating the basics:
phpCopy code<?php
// This is a single-line comment
/*
This is a multi-line comment
*/
// Variables
$greeting = "Hello, World!";
$number = 5;
// Output
echo $greeting; // Displays "Hello, World!"
// Conditional Statement
if ($number > 0) {
echo "The number is positive.";
} else {
echo "The number is not positive.";
}
// Function
function add($a, $b) {
return $a + $b;
}
// Using the function
$result = add(3, 4);
echo "The sum is: " . $result;
?>
Breakdown of the Script:
Comments: Used to explain the code. PHP ignores them.
Variables: Start with
$
and can store different data types.Output:
echo
is used to send output to the browser.Conditional Statements: Control the flow of the program based on conditions.
Functions: Encapsulate reusable code blocks.
Introduction to PHP
PHP, which stands for "Hypertext Preprocessor," is a server-side scripting language designed primarily for web development. Originally created by Danish-Canadian programmer Rasmus Lerdorf in 1993, PHP has evolved significantly over the years. It was initially intended for simple web tasks but has grown into a robust language capable of handling complex applications.
PHP is particularly well-suited for creating dynamic content, interacting with databases, and managing session tracking. It can be embedded within HTML and is known for its flexibility, ease of use, and wide community support.
History of PHP
1993: Rasmus Lerdorf created the original PHP tools to track visitors to his online resume.
1995: The language was officially released as PHP/FI (Personal Home Page/Form Interpreter), adding more features for form processing.
1997: PHP 3 was released, marking a significant improvement and introducing a more robust and extensible architecture.
2004: PHP 5 was launched, introducing support for object-oriented programming, making it more suitable for larger applications.
2015: PHP 7 was released, featuring significant performance improvements and reduced memory consumption.
2021: PHP 8 introduced Just-In-Time (JIT) compilation, enhancing performance further and adding new syntax features.
Key Features of PHP
1. Server-Side Scripting
PHP scripts are executed on the server, generating HTML that is sent to the client’s browser. This allows for the creation of dynamic web pages that can change based on user input or other factors.
2. Database Integration
PHP has built-in support for various databases, especially MySQL. This capability allows developers to create data-driven applications easily.
3. Cross-Platform Compatibility
PHP runs on various operating systems, including Windows, Linux, and macOS. It also supports multiple web servers such as Apache and Nginx.
4. Open Source
Being open-source, PHP is free to use and has a large community that contributes to its development. This community support ensures continuous improvement and a wealth of resources for learning.
5. Rich Library of Functions
PHP offers a vast library of built-in functions, making it easy to perform tasks like string manipulation, file handling, and working with arrays.
6. Ease of Learning
PHP's syntax is straightforward and similar to C and Java, making it accessible for beginners. Developers can quickly start building web applications without extensive background knowledge.
7. Session Management
PHP has excellent support for session management, allowing developers to store user information across multiple pages.
Basic PHP Syntax
To understand PHP, it’s essential to grasp its basic syntax. Here are some fundamental elements:
1. Opening and Closing Tags
PHP code is written within <?php ... ?>
tags. Anything outside these tags is treated as HTML.
2. Variables
Variables in PHP start with a dollar sign ($
). They are loosely typed, meaning you don’t need to declare their type.
phpCopy code<?php
$name = "Alice"; // String
$age = 30; // Integer
$height = 5.6; // Float
$is_student = true; // Boolean
?>
3. Comments
Comments can be single-line (//
) or multi-line (/* ... */
).
phpCopy code<?php
// This is a single-line comment
/*
This is a multi-line comment
*/
?>
4. Control Structures
PHP supports standard control structures like if
, else
, switch
, and loops (for
, while
, foreach
).
phpCopy code<?php
$number = 10;
if ($number > 0) {
echo "Positive number";
} else {
echo "Negative number";
}
?>
5. Functions
Functions allow you to encapsulate reusable code. You define a function with the function
keyword.
phpCopy code<?php
function greet($name) {
return "Hello, " . $name;
}
echo greet("Alice"); // Outputs: Hello, Alice
?>
Basic PHP Scripts
Let’s look at some simple PHP scripts to demonstrate its capabilities.
Example 1: Hello World
This script outputs "Hello, World!" to the browser.
phpCopy code<?php
echo "Hello, World!";
?>
Example 2: Form Handling
This script processes a form submission.
phpCopy code<!DOCTYPE html>
<html>
<body>
<form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>">
Name: <input type="text" name="name">
<input type="submit">
</form>
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = htmlspecialchars($_POST['name']);
echo "Hello, " . $name;
}
?>
</body>
</html>
Example 3: Connecting to a Database
This example demonstrates how to connect to a MySQL database using PDO.
phpCopy code<?php
$host = '127.0.0.1';
$db = 'test_db';
$user = 'root';
$pass = '';
$charset = 'utf8mb4';
$dsn = "mysql:host=$host;dbname=$db;charset=$charset";
$options = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC,
PDO::ATTR_EMULATE_PREPARES => false,
];
try {
$pdo = new PDO($dsn, $user, $pass, $options);
echo "Connected successfully";
} catch (\PDOException $e) {
throw new \PDOException($e->getMessage(), (int)$e->getCode());
}
?>
Conclusion
PHP remains one of the most popular programming languages for web development due to its simplicity, flexibility, and powerful features. Whether you are building a simple website or a complex web application, PHP provides the tools necessary to create dynamic, interactive, and data-driven experiences.
As you continue to learn PHP, consider exploring its frameworks (like Laravel and Symfony) that can further enhance your productivity and code organization. With its extensive community support and resources, the possibilities with PHP are vast, making it a valuable skill for any web developer.
Subscribe to my newsletter
Read articles from Mostafa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
