Building TCP Servers in Go: A Simple Guide
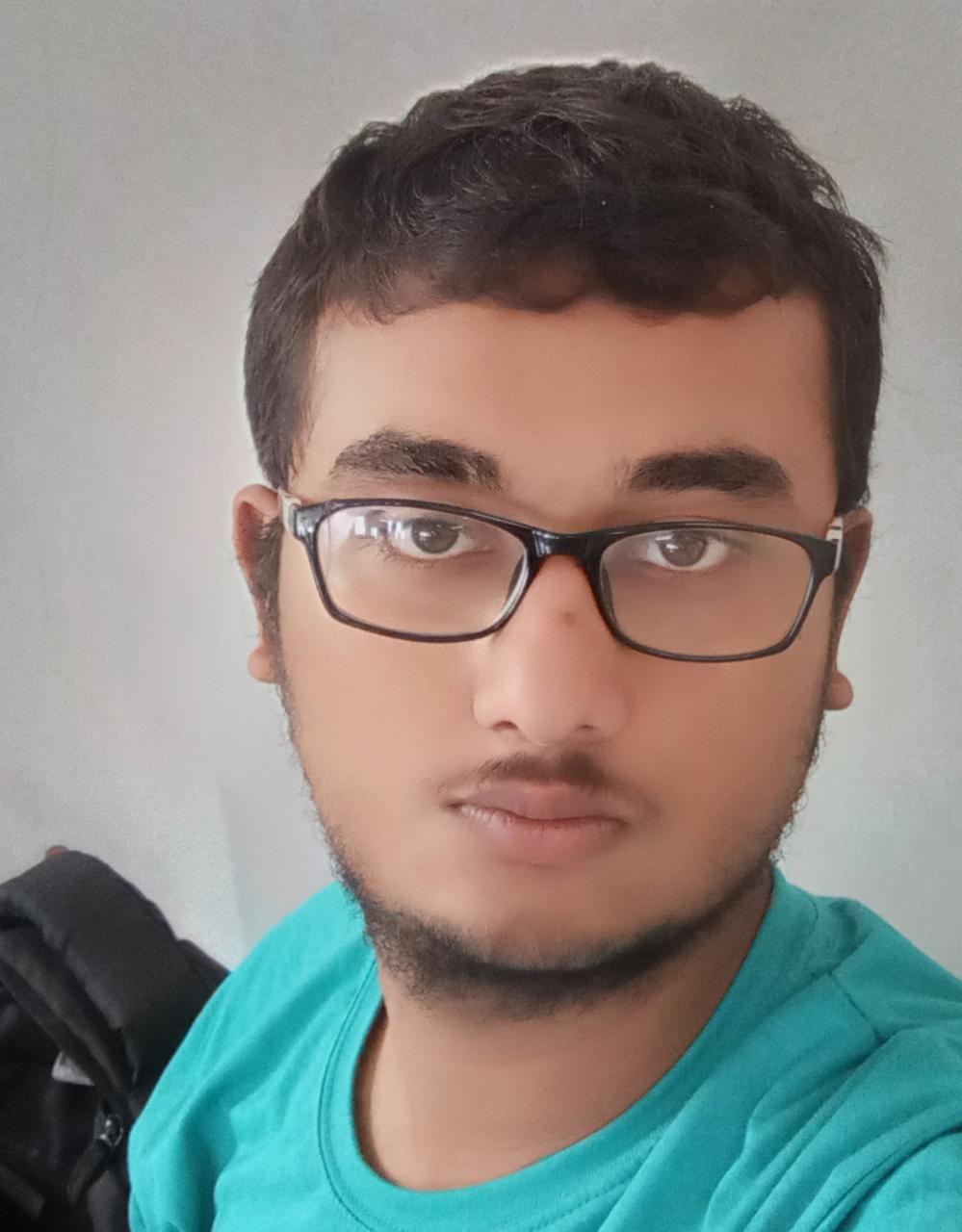
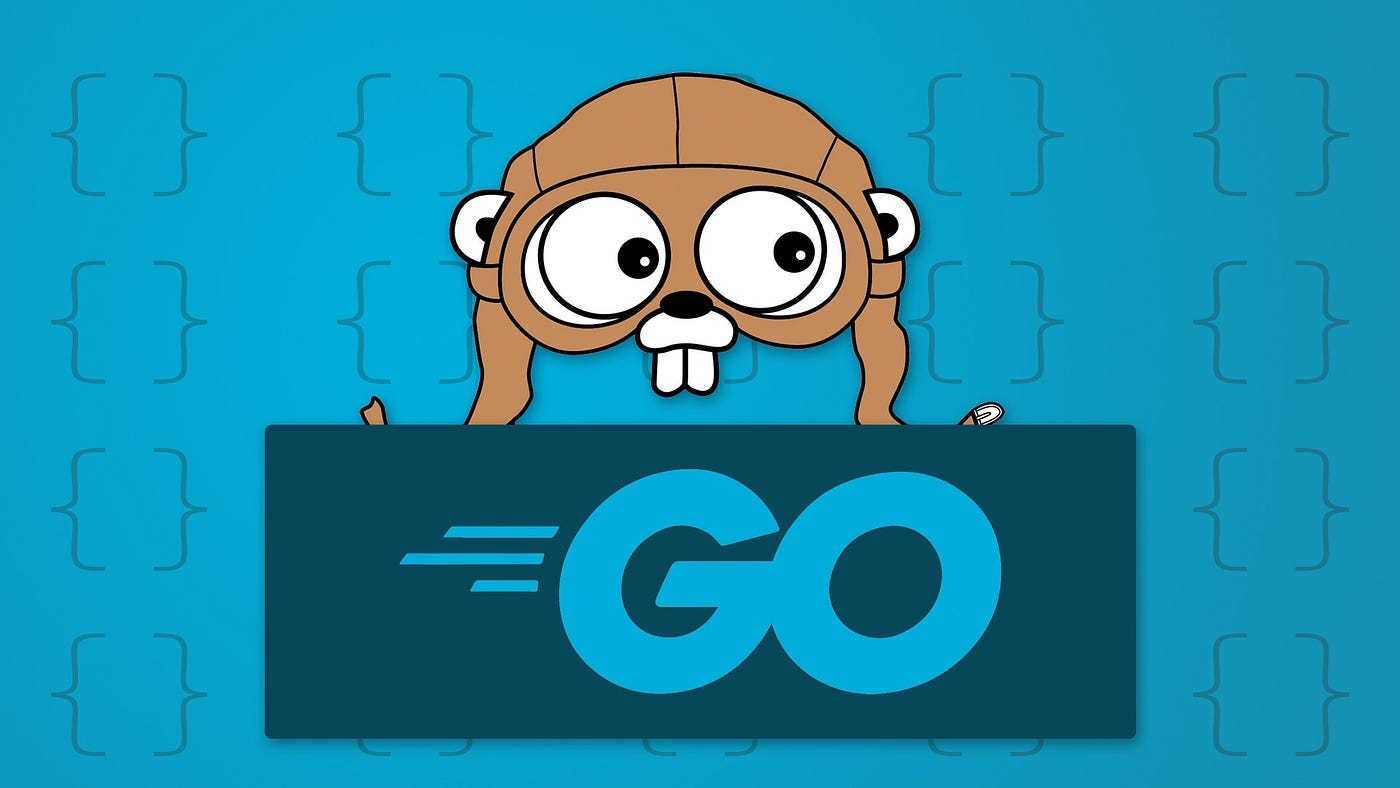
Have you ever wondered how applications communicate over networks? The unsung hero behind many of these interactions is the Transmission Control Protocol (TCP). This blog post will guide you through creating a basic TCP server in Go using the powerful net
package.
What is TCP?
TCP is a fundamental building block of the internet. It's a reliable, connection-oriented protocol, ensuring data packets are delivered in the order they were sent. Think of it like a secure postal service for your digital information.
Building a TCP Server with Go
Go's net
package provides the tools needed to construct TCP servers. Here's a breakdown of the key functions:
net.Dial: This function establishes outgoing connections, allowing your program to act as a client.
net.Listen: This is the workhorse for creating TCP servers. It "listens" on a specific port, waiting for incoming client connections.
Let's Code!
Here's a simple example of a TCP server that echoes back any data it receives:
package main
import (
"fmt"
"net"
)
func main() {
// Listen on port 8080
listener, err := net.Listen("tcp", "localhost:8080")
if err != nil {
fmt.Println("Error:", err)
return
}
// Close listener when program exits
defer listener.Close()
fmt.Println("Server listening on port 8080")
for {
// Wait for a client connection
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error:", err)
continue
}
// Handle the client connection
go handleClient(conn)
}
}
func handleClient(conn net.Conn) {
// Close connection when done
defer conn.Close()
// Read data from client
buf := make([]byte, 1024)
n, err := conn.Read(buf)
if err != nil {
return
}
fmt.Println("Received data:", string(buf[:n]))
// Echo the data back
conn.Write(buf[:n])
}
Explanation:
net.Listen
: We usenet.Listen
to create a listener object on port 8080.Infinite Loop: An infinite loop keeps the server running, waiting for incoming connections.
listener.Accept
: Inside the loop,listener.Accept
blocks until a client connects. Upon connection, it returns anet.Conn
object representing the established communication channel.handleClient
: A separate goroutine is launched for each client usinggo handleClient(conn)
. This allows the server to handle multiple connections concurrently.conn.Read
: InsidehandleClient
, we read data sent by the client usingconn.Read
. The data is stored in a byte slicebuf
.Echo Data: We print the received data and then send it back to the client using
conn.Write
.Closing Connections: Both
main
andhandleClient
usedefer
statements to ensure connections are closed properly when the program exits or a client disconnects.
Summary
This example demonstrates the basic building blocks for creating TCP servers in Go. With the net
package, you can build powerful and scalable network applications. Remember, this is a simple example. Real-world servers often involve additional functionalities like error handling, logging, and more complex data processing.
Ready to Explore Further?
The Go documentation provides extensive details on the net
package and network programming concepts. Now that you have the foundation, you can delve deeper and build more intricate applications!
Want to try this out yourself? Check out this interactive tutorial to get your hands dirty: https://app.codecrafters.io/tracks/go
Subscribe to my newsletter
Read articles from Swarnim Pratap Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
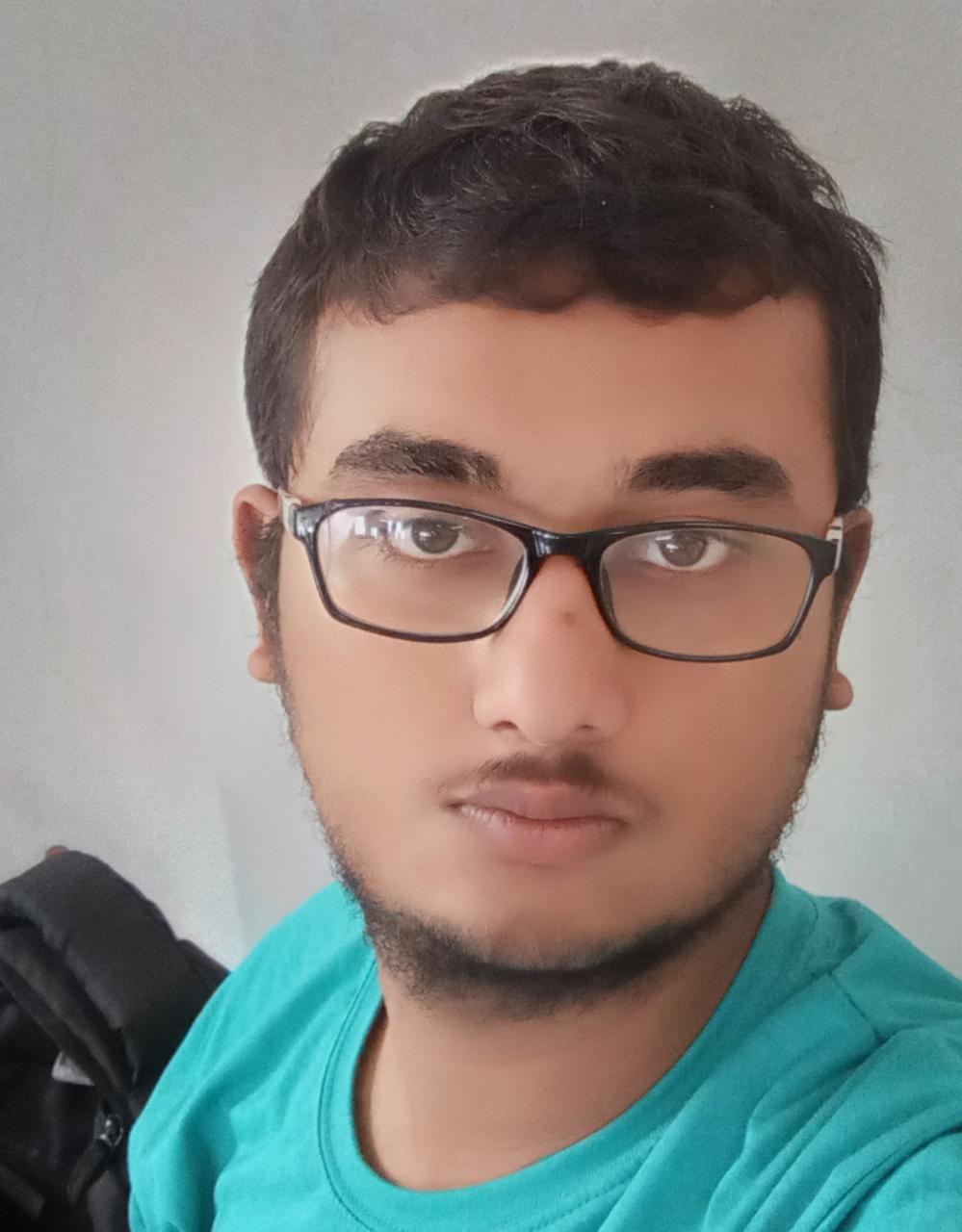
Swarnim Pratap Singh
Swarnim Pratap Singh
I'm a software developer and open-source contributor