Understanding Bitwise Operations in Python

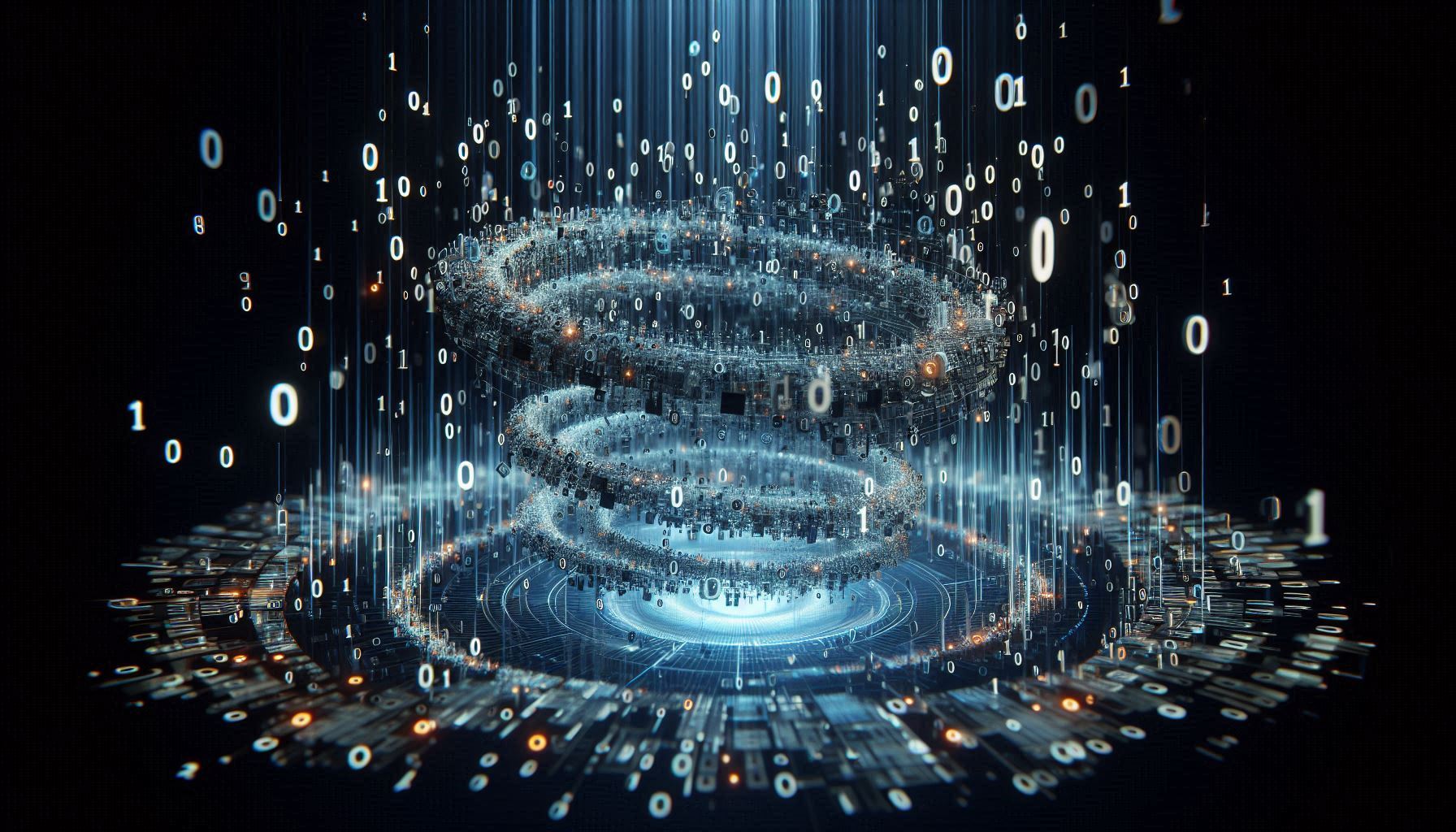
Bitwise operations are a powerful tool in programming that allow you to perform operations directly on the binary representation of numbers. In Python, these operations are particularly useful for tasks requiring low-level data manipulation, such as optimizing performance, working with binary data, or implementing specific algorithms efficiently.
Let’s dive into the main bitwise operations available in Python and see how they work with examples.
1. Bitwise AND (&
)
Syntax: a & b
The bitwise AND operation compares each bit of two integers and returns a 1 in each position where both bits are 1. Otherwise, it returns 0.
Example:
result = 5 & 3 # 5 = 101, 3 = 011 in binary
print(result) # Output: 1 (in binary: 001)
In this example, only the last bit is 1 in both numbers, so the result is 1.
2. Bitwise OR (|
)
Syntax: a | b
The bitwise OR operation compares each bit of two integers and returns a 1 if at least one of the bits in that position is 1; otherwise, it returns 0.
Example:
result = 5 | 3 # 5 = 101, 3 = 011 in binary
print(result) # Output: 7 (in binary: 111)
Here, each bit position has at least one 1, so the result is 111 in binary (or 7 in decimal).
3. Bitwise XOR (^
)
Syntax: a ^ b
The bitwise XOR (exclusive OR) operation returns a 1 in each bit position where the corresponding bits are different, and 0 where they are the same.
Example:
result = 5 ^ 3 # 5 = 101, 3 = 011 in binary
print(result) # Output: 6 (in binary: 110)
In this example, the second and third bits differ, resulting in 110 in binary (or 6 in decimal).
4. Bitwise NOT (~
)
Syntax: ~a
The bitwise NOT operation flips all bits in the number, effectively inverting it. In Python, this is equivalent to -a - 1
, due to the way Python handles negative integers in binary.
Example:
result = ~5 # 5 = 00000101 in binary
print(result) # Output: -6 (in binary: 11111010)
Flipping all the bits in 5 results in a binary representation of -6.
5. Left Shift (<<
)
Syntax: a << n
The left shift operation shifts the bits of a
to the left by n
positions. Each shift to the left is equivalent to multiplying a
by (2^n).
Example:
result = 5 << 1 # 5 = 101 in binary
print(result) # Output: 10 (in binary: 1010)
Shifting left by one position moves each bit one place to the left, effectively doubling the number.
6. Right Shift (>>
)
Syntax: a >> n
The right shift operation shifts the bits of a
to the right by n
positions. For positive numbers, this is equivalent to integer division by (2^n). For negative numbers, the sign bit is preserved, so it effectively rounds down.
Example:
result = 5 >> 1 # 5 = 101 in binary
print(result) # Output: 2 (in binary: 10)
Shifting right by one position divides the number by 2, discarding any remainder.
Additional Notes on Bitwise Operations
Using Bitwise Operations for Common Tasks
Bitwise operations can simplify certain tasks in programming. Here are some common uses:
Checking Even/Odd: Use
x & 1
. If the result is 0,x
is even; if the result is 1,x
is odd.x = 5 if x & 1 == 0: print("Even") else: print("Odd") # Output: Odd
Swapping Without a Temp Variable: You can swap two variables,
a
andb
, without a temporary variable using XOR:a = 5 b = 3 a = a ^ b b = a ^ b a = a ^ b print(a, b) # Output: 3, 5
Masking: Use
x & mask
to keep only specific bits. This is helpful when working with flags or selective bit modifications.
Bit Length
The bit_length()
method returns the number of bits required to represent an integer in binary.
x = 5
print(x.bit_length()) # Output: 3 (since 5 is 101 in binary)
Bitwise operations are efficient and useful tools in Python, especially when working with low-level data manipulation tasks. With these examples and explanations, you should be well-equipped to make the most of bitwise operations in your own projects!
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.