Project-8: ๐ Deploying 2048 Game to AWS Elastic Beanstalk with GitHub Actions
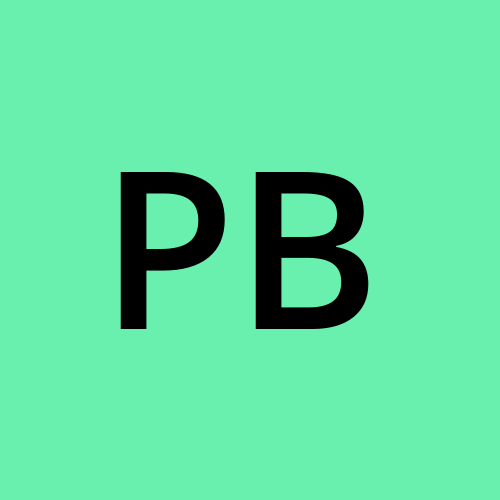
Introduction
Welcome to this step-by-step tutorial where we'll learn how to deploy the popular 2048 game to AWS Elastic Beanstalk using GitHub Actions! ๐ AWS Elastic Beanstalk simplifies the deployment and management of web applications, while GitHub Actions automates the deployment process with Continuous Integration and Continuous Deployment (CICD) workflows. Let's get ready for an exciting journey! ๐
Why Use AWS Elastic Beanstalk and GitHub Actions?
AWS Elastic Beanstalk ๐
AWS Elastic Beanstalk is a fully managed Platform-as-a-Service (PaaS) offering from Amazon Web Services (AWS). It simplifies the process of deploying and scaling applications by automatically managing the underlying infrastructure, such as EC2 instances, load balancers, and databases. Elastic Beanstalk supports multiple programming languages and platforms, making it suitable for various types of applications.
Benefits of using AWS Elastic Beanstalk include:
Simplicity: Elastic Beanstalk abstracts away the complexities of infrastructure management, allowing developers to focus on writing code and deploying applications with ease.
Automatic Scaling: Elastic Beanstalk can automatically scale your application based on traffic or performance metrics, ensuring that it can handle varying workloads efficiently.
Integrated Monitoring: Elastic Beanstalk provides built-in monitoring capabilities to help you track the health and performance of your applications.
Platform Choice: With support for multiple platforms, Elastic Beanstalk accommodates developers working with different programming languages and frameworks.
GitHub Actions โ๏ธ
GitHub Actions is a powerful workflow automation tool integrated with GitHub repositories. It allows developers to define custom workflows, including building, testing, and deploying applications. GitHub Actions provides a seamless CI/CD solution for software development projects, enabling teams to automate repetitive tasks and improve collaboration.
Benefits of using GitHub Actions include:
Continuous Integration: GitHub Actions allows developers to automatically build and test code changes whenever they are pushed to the repository, promoting early detection of issues.
Continuous Deployment: With GitHub Actions, you can automate the deployment process to different environments, such as staging and production, based on predefined conditions.
Flexibility: GitHub Actions supports custom workflows with various triggers, allowing you to define complex build and deployment pipelines.
Integration with GitHub: Being integrated directly into GitHub, GitHub Actions provides a familiar interface for developers to manage their workflows and monitor their progress.
Prerequisites
Before we dive into the deployment process, make sure you have the following prerequisites:
An AWS account with Elastic Beanstalk access.
A GitHub repository with the 2048 game application code. (We have this docker file where there is no need for code, the docker file explicitly pulls the code and creates a container out of it. )
Basic knowledge of Git, Docker, and AWS services.
Install Docker and docker-compose on your EC2 instance:
sudo apt update sudo apt install -y docker.io sudo usermod -aG docker $USER sudo systemctl enable docker sudo systemctl restart docker sudo apt install -y docker-compose
Step 1: ๐ฆ Setting up the Dockerfile ๐ณ
To begin, we'll create a Dockerfile that packages our 2048 game application. ๐ (Make sure you have docker and docker-compose installed ๐ณ)The Dockerfile will specify the necessary instructions to build a Docker container for our game. Follow these steps:
Create a new file named
Dockerfile
in the root of your 2048 game repository.Copy and paste the following code into your Dockerfile:
FROM ubuntu:22.04
RUN apt-get update && apt-get install -y nginx zip curl
RUN sed -i '/daemon off;/d' /etc/nginx/nginx.conf
RUN echo "daemon off;" >> /etc/nginx/nginx.conf
RUN curl -o /var/www/html/master.zip -L https://github.com/gabrielecirulli/2048/archive/master.zip
RUN cd /var/www/html/ && unzip master.zip && mv 2048-master/* . && rm -rf 2048-master master.zip
EXPOSE 80
CMD ["nginx", "-c", "/etc/nginx/nginx.conf"]
Step 2: ๐ณ Developing 2048 Application inside a Docker Container ๐ป
Now, let's develop our 2048 game application inside a Docker container. ๐ณ If you don't have Docker installed on your local system, we'll set up an EC2 instance with Docker for this purpose. Follow these steps:
Set up an EC2 instance on AWS with Docker installed. You can find guides on how to do this in the AWS documentation.
Once the EC2 instance is ready, SSH into the instance and create a new directory for our game.
Copy the Dockerfile created in Step 1 into the new directory.
Build the Docker container using the following command:
docker build -t 2048-game .
Step 3: Creating a Docker Compose File ๐
With our Docker container ready, let's create a Docker Compose file to define the services and configurations for our 2048 game application. Follow these steps:
Create a new file named
docker-compose.yml
in the root of your 2048 game repository.Copy and paste the following code into the
docker-compose.yml
file:
version: "3.8"
services:
nginx:
build:
context: .
dockerfile: Dockerfile
ports:
- "80:80"
Step 4: ๐ Testing Your Application on EC2 Instance ๐งช
Great job! It's time to test our 2048 game application to make sure it's running smoothly on our EC2 instance. ๐งช Follow these steps:
- Deploy the application on the EC2 instance using Docker Compose:
docker-compose up -d
- Open your web browser and navigate to the public IP of your EC2 instance. You should see the 2048 game running and ready to play! ๐
Step 5: โ๏ธ Deploying to AWS Elastic Beanstalk with GitHub Actions ๐
Now comes the exciting part โ deploying our 2048 game to AWS Elastic Beanstalk using GitHub Actions! โ๏ธ Let's create the GitHub Actions workflow. Follow these steps:
In your GitHub repository, create a new folder named
.github
.Inside the
.github
folder, create another folder namedworkflows
.In the
workflows
folder, create a new file nameddeploy-aws.yml
.
Step 6: ๐๏ธ Configuring GitHub Actions ๐
Now, let's configure the deploy-aws.yml
file to define our GitHub Actions workflow for CICD. Follow these steps:
- Copy and paste the following code into the
deploy-aws.yml
file:
name: Deploy to AWS Elastic Beanstalk
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Set up AWS CLI
run: |
sudo apt update
sudo apt install -y python3-pip
pip3 install --user awscli
- name: Configure AWS Credentials
run: |
aws configure set aws_access_key_id ${{ secrets.AWS_ACCESS_KEY_ID }}
aws configure set aws_secret_access_key ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws configure set region us-east-1
- name: Deploy to AWS Elastic Beanstalk
run: |
aws elasticbeanstalk create-application-version --application-name YOUR_APPLICATION_NAME --version-label v${{ github.sha }} --source-bundle S3Bucket=elasticbeanstalk-us-east-1-YOUR_ACCOUNT_ID, S3Key=2048-game.tar
aws elasticbeanstalk update-environment --environment-name YOUR_ENVIRONMENT_NAME --version-label v${{ github.sha }}
Replace YOUR_APPLICATION_NAME
, YOUR_ENVIRONMENT_NAME
, and YOUR_ACCOUNT_ID
with the appropriate values for your AWS Elastic Beanstalk application and environment.
Step 7: ๐ Configuring AWS Elastic Beanstalk Environment ๐
Before we deploy our 2048 game application to AWS Elastic Beanstalk, we need to configure the Elastic Beanstalk environment to run our application smoothly. Follow these steps to set up the environment:
Login to AWS Console: Sign in to your AWS Management Console using your AWS account credentials.
Navigate to Elastic Beanstalk: Once logged in, navigate to the AWS Elastic Beanstalk service by clicking on "Services" in the top navigation bar, then selecting "Elastic Beanstalk" under the "Compute" section.
Create a New Application: In the Elastic Beanstalk dashboard, click on "Create a new application." Give your application a name, such as "2048-game," and choose a platform that matches your Docker container (e.g., "Docker" for our case). Click "Create."
Create an Environment: After creating the application, click on the "Create environment" button to create a new environment for your application.
Environment Type: Choose "Web server environment" to create an environment with a load balancer.
Base Configuration: Select "Docker" as the platform and choose the appropriate Docker version.
Upload Your Code: click on sample application
Environment Information: Provide a unique and descriptive name for your environment, such as "2048-game-environment."
Instance Type: Choose an instance type that suits your application's requirements. For testing purposes, you can select the default instance type.
Security: Configure the security settings based on your project requirements. You can use the default settings for testing purposes.
Additional Configuration: Optionally, you can configure additional settings like scaling options, environment variables, and more based on your application's needs.
Create Environment: Click on the "Create environment" button to create the environment.
Wait for Environment Creation: AWS Elastic Beanstalk will now start creating the environment for your application. This process may take a few minutes to complete. Once the environment is successfully created, you'll see the status as "Ready" in the Elastic Beanstalk dashboard.
Test the Environment: After the environment is ready, you can test your application by navigating to the URL provided in the Elastic Beanstalk dashboard. It should display the demo application running on AWS Elastic Beanstalk.
Congratulations! ๐ You have successfully configured your AWS Elastic Beanstalk environment to run the demo application. Now, we're all set to deploy the application using GitHub Actions.
In the next steps, we'll continue with the deployment using GitHub Actions. We'll create the necessary GitHub Actions workflow that automatically triggers the deployment process whenever changes are pushed to the main branch of your GitHub repository. Let's proceed with the final steps!
Step 8: ๐โโ๏ธ Deploying with GitHub Actions ๐
With all the configurations in place, we're now ready to deploy our 2048 game application to AWS Elastic Beanstalk using GitHub Actions! ๐โโ๏ธ
Commit your changes and push to the
main
branch in your GitHub repository.GitHub Actions will automatically trigger the deployment workflow, and your application will be deployed to AWS Elastic Beanstalk.
##
Subscribe to my newsletter
Read articles from Pooja Bhavani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
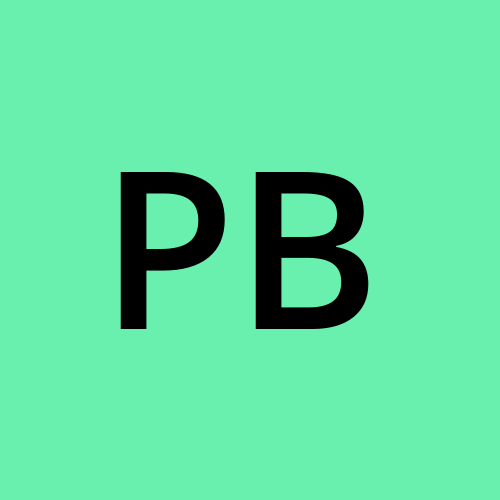