Kubernetes Probes: Liveness, Readiness, and Startup

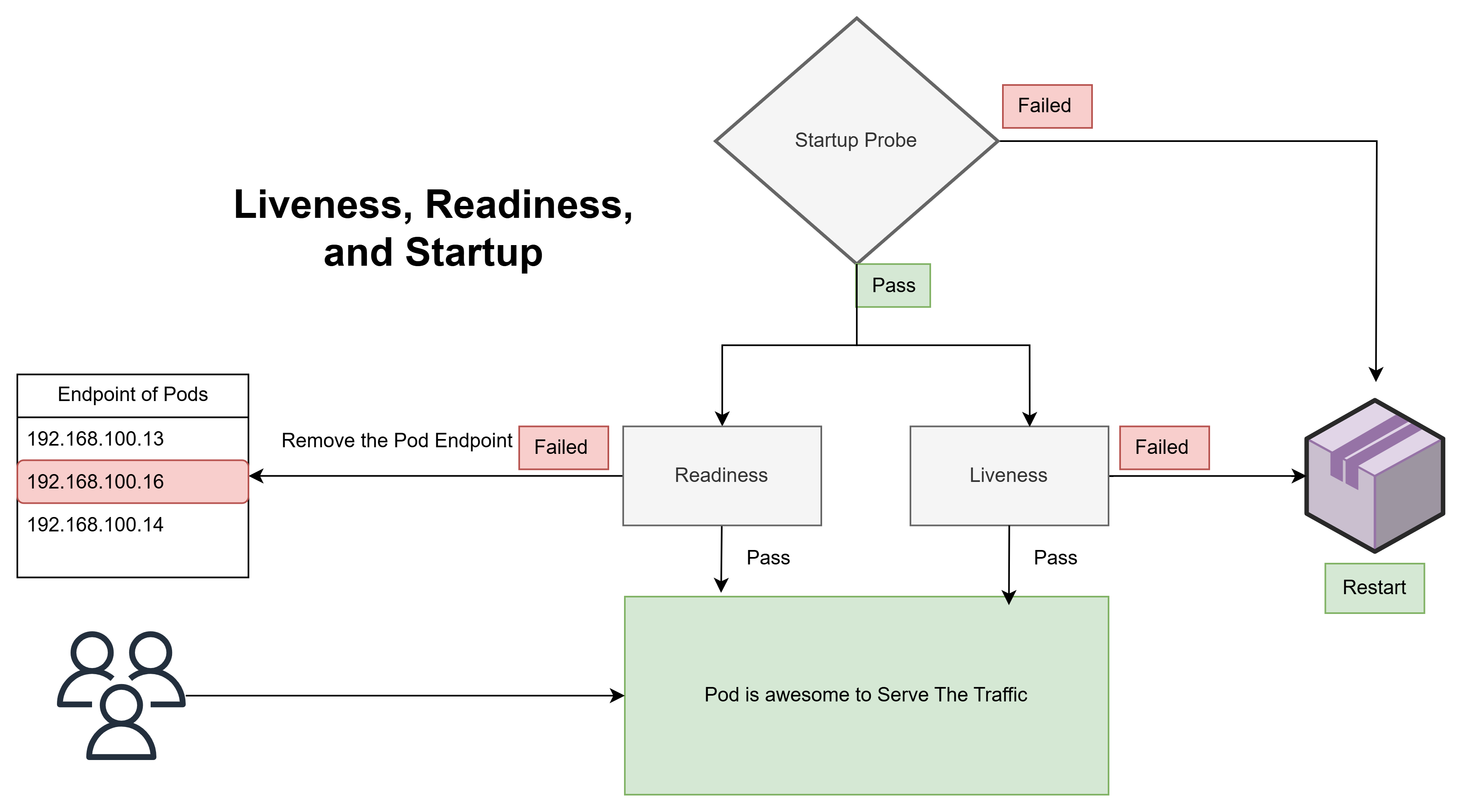
Kubernetes liveness, readiness, and startup probes are like health check mechanisms for your applications running in containers, ensuring theyโre operating smoothly. Hereโs a simple explanation of each using an analogy of running a business.
๐๐ถ๐๐ฒ๐ป๐ฒ๐๐ ๐ฃ๐ฟ๐ผ๐ฏ๐ฒ (๐๐ ๐๐ต๐ฒ ๐๐ฒ๐ฟ๐๐ถ๐ฐ๐ฒ ๐๐๐ถ๐น๐น ๐ฎ๐น๐ถ๐๐ฒ?)
๐๐ป๐ฎ๐น๐ผ๐ด๐: Imagine youโre running a coffee shop. The liveness probe is like checking whether your coffee machine is still functioning throughout the day. If the machine breaks down and stops making coffee, you need to either fix it or replace it, otherwise, customers wonโt get coffee.
๐๐ป ๐๐๐ฏ๐ฒ๐ฟ๐ป๐ฒ๐๐ฒ๐: The liveness probe ensures that your application is still alive and not stuck or crashed. If it fails, Kubernetes restarts the container automatically. For example, if a container goes into a deadlock, the liveness probe detects this and restarts the container to fix the issue.
๐ช๐ต๐ฒ๐ป ๐๐ผ ๐๐๐ฒ: If your application can sometimes get into an unrecoverable state, like a crash or deadlock, this probe can automatically fix it by restarting the container.
๐ฅ๐ฒ๐ฎ๐ฑ๐ถ๐ป๐ฒ๐๐ ๐ฃ๐ฟ๐ผ๐ฏ๐ฒ (๐๐ ๐๐ต๐ฒ ๐๐ฒ๐ฟ๐๐ถ๐ฐ๐ฒ ๐ฟ๐ฒ๐ฎ๐ฑ๐ ๐๐ผ ๐๐ฒ๐ฟ๐๐ฒ ๐ฐ๐๐๐๐ผ๐บ๐ฒ๐ฟ๐?)
๐๐ป๐ฎ๐น๐ผ๐ด๐: In the coffee shop, the readiness probe checks if your coffee machine is ready to serve customers. For example, after turning on the machine, it might take a few minutes to warm up before it can make coffee. If the machine isnโt ready yet, customers shouldnโt be directed to it.
๐๐ป ๐๐๐ฏ๐ฒ๐ฟ๐ป๐ฒ๐๐ฒ๐: The readiness probe checks if the container is ready to handle traffic. It might take time for your app to fully initialize or connect to dependencies (like a database). Kubernetes wonโt send any traffic to your app until the readiness probe passes.
๐ช๐ต๐ฒ๐ป ๐๐ผ ๐๐๐ฒ: Use it when your application needs some time to initialize before being able to serve requests (e.g., loading configuration, warming up cache).
๐ฆ๐๐ฎ๐ฟ๐๐๐ฝ ๐ฃ๐ฟ๐ผ๐ฏ๐ฒ (๐๐ ๐๐ต๐ฒ ๐๐ฒ๐ฟ๐๐ถ๐ฐ๐ฒ ๐๐ฝ ๐ฎ๐ป๐ฑ ๐ฟ๐๐ป๐ป๐ถ๐ป๐ด ๐ฎ๐ ๐๐๐ฎ๐ฟ๐๐๐ฝ?)
๐๐ป๐ฎ๐น๐ผ๐ด๐: When you first open the coffee shop in the morning, the startup probe is like checking if everything (coffee machine, lights, etc.) is set up and ready for business. Until this initial setup is complete, no customers can come in.
๐๐ป ๐๐๐ฏ๐ฒ๐ฟ๐ป๐ฒ๐๐ฒs: The startup probe checks if your containerโs application has started successfully. Itโs especially useful for apps that have slow startup times. Until the startup probe passes, Kubernetes wonโt send any other probes (liveness or readiness), ensuring that the app doesnโt get prematurely killed or sent traffic before itโs ready.
๐ช๐ต๐ฒ๐ป ๐๐ผ ๐๐๐ฒ: Ideal for apps that have a long initialization process. This prevents Kubernetes from misjudging slow-starting apps as unhealthy.
Example in Node.js API
Here's how to set up liveness, readiness, and startup probes for a Node.js API in Kubernetes
Suppose you have a simple Node.js API with an endpoint /health
for health checks.
// server.js
const express = require("express");
const app = express();
const PORT = process.env.PORT || 3000;
let isReady = false; // Used to simulate readiness
app.get("/health", (req, res) => {
res.status(200).send("API is alive");
});
app.get("/readiness", (req, res) => {
if (isReady) {
res.status(200).send("API is ready");
} else {
res.status(503).send("API is not ready");
}
});
// Simulate delayed readiness
setTimeout(() => {
isReady = true;
}, 10000); // API becomes ready after 10 seconds
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
This API has
/health
: Returns200 OK
to indicate the API is alive./readiness
: Returns503 Service Unavailable
until the API is fully ready, then returns200 OK
after 10 seconds.
Kubernetes Probes
Liveness Probe: Checks if the app is alive by hitting
/health
. If this probe fails, Kubernetes restarts the container.Readiness Probe: Checks if the app is ready to handle traffic by hitting
/readiness
. Only after the app is fully initialized does this probe return a200 OK
, allowing Kubernetes to route traffic to it.Startup Probe: For apps with a slow start, the startup probe waits until the app is completely initialized before allowing other probes to execute.
Hereโs how you can define these probes in your Kubernetes deployment YAML file
apiVersion: apps/v1
kind: Deployment
metadata:
name: nodejs-api
spec:
replicas: 1
selector:
matchLabels:
app: nodejs-api
template:
metadata:
labels:
app: nodejs-api
spec:
containers:
- name: nodejs-api
image: node:14
command: ["node", "server.js"]
ports:
- containerPort: 3000
livenessProbe:
httpGet:
path: /health
port: 3000
initialDelaySeconds: 5
periodSeconds: 10
readinessProbe:
httpGet:
path: /readiness
port: 3000
initialDelaySeconds: 5
periodSeconds: 5
startupProbe:
httpGet:
path: /health
port: 3000
initialDelaySeconds: 5
periodSeconds: 5
failureThreshold: 20
Explanation of Each Probe Configuration
Liveness Probe:
Path:
/health
initialDelaySeconds
: Waits 5 seconds before the first check.periodSeconds
: Checks every 10 seconds to see if the app is alive. If it fails, Kubernetes restarts the container.
Readiness Probe:
Path:
/readiness
initialDelaySeconds
: Waits 5 seconds before the first check.periodSeconds
: Checks every 5 seconds to see if the app is ready to serve traffic. Traffic isnโt sent until this probe passes.
Startup Probe:
Path:
/health
initialDelaySeconds
: Waits 5 seconds before the first check.periodSeconds
: Checks every 5 seconds during startup.failureThreshold
: Allows up to 20 checks (100 seconds) to ensure the app has fully initialized before liveness or readiness probes start, preventing premature restarts.
This setup helps Kubernetes manage your Node.js APIโs health effectively, restarting it if it crashes, waiting until itโs ready before routing traffic, and allowing time for complete startup if initialization is slow.
Which probes failled restart the container?
Liveness Probe: If the liveness probe fails, Kubernetes restarts the container. This probe checks if the application is still "alive" (not in a crashed or deadlocked state). If it detects an issue, it considers the container unhealthy and restarts it to try to resolve the issue.
Readiness Probe: A readiness probe failure does not restart the container. Instead, it marks the container as "unready," meaning Kubernetes will stop routing traffic to it until the probe passes again. This is useful if the application temporarily cannot serve requests (e.g., during maintenance or reconfiguration).
Startup Probe: If the startup probe fails, it also triggers a container restart. The startup probe is intended for applications that need extra time to initialize. It prevents the liveness and readiness probes from prematurely failing until the app has fully started up.
So, only the liveness and startup probes trigger a restart (Also depending on the Restart Policy default to Always ) when they fail. The readiness probe only affects traffic routing.
Execution of of a probes
In Kubernetes, probes are checked in a specific sequence based on the presence of a startup probe
Startup Probe (if configured): This probe is checked first. When a startup probe is defined, Kubernetes will wait until it passes successfully before starting to run the liveness and readiness probes. This avoids premature restarts or traffic routing failures for applications with slow initialization. While the startup probe is running, the liveness and readiness probes are disabled.
Liveness and Readiness Probes (after Startup Probe passes):
Liveness Probe: Checks if the container is still healthy and able to function.
Readiness Probe: Determines if the container is ready to serve traffic.
Once the startup probe passes (or if it isnโt defined), the liveness and readiness probes begin their checks according to their configured intervals.
Priority Summary
Startup Probe has the highest priority. Until it succeeds, the liveness and readiness probes are ignored.
Liveness and Readiness Probes then run independently of each other on their own schedules once the startup probe has passed.
Summary
Liveness Probe restarts the container on failure.
Readiness Probe controls traffic routing based on readiness.
Startup Probe provides a grace period for slow-starting apps before liveness and readiness checks.
Sources
https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle/
Subscribe to my newsletter
Read articles from Muhammad Usama directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Muhammad Usama
Muhammad Usama
I am a DevOps Engineer passionate about cloud computing.