Python Inner Working

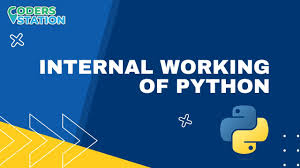
Python’s inner workings, often referred to as the "Python internals," involve the processes and components that the Python interpreter uses to execute code. Here’s a look at some fundamental aspects of these inner workings:
1. Python Bytecode
When you write Python code, the interpreter doesn't execute it directly. Instead, it compiles your code into an intermediate representation known as bytecode. This bytecode is a low-level set of instructions specific to the Python interpreter, not the machine. Here’s the process:
Source Code → Bytecode: Python compiles
.py
files into bytecode when you run them, which can be saved as.pyc
files in the__pycache__
folder.Bytecode can be reused, making execution faster since the interpreter doesn’t need to recompile every time you run the script.
2. CPython Virtual Machine (PVM)
The most common Python interpreter, CPython, uses a Python Virtual Machine (PVM) to execute the bytecode. The PVM is an interpreter loop that reads bytecode instructions and executes them one by one.
- This virtual machine abstracts away details about the underlying hardware, enabling portability across platforms.
3. Stack-Based Execution
Python’s virtual machine operates as a stack-based machine, meaning it uses a stack data structure to keep track of operations. Each operation in Python bytecode typically involves pushing operands onto the stack, performing operations, and then pushing results back onto the stack. This is one reason Python’s execution is slower compared to languages that compile down to machine code, like C or C++.
4. Objects and Memory Management
Python treats everything as an object, from integers to classes. These objects are stored in memory and managed by Python’s memory manager, which handles:
Object Creation and Destruction: Python automatically allocates memory for objects when they are created and deallocates it when they are no longer needed.
Reference Counting and Garbage Collection: Python primarily uses reference counting to manage memory. Every object has a reference count, and when the count drops to zero, the memory is released. For handling circular references (where objects reference each other), Python includes a garbage collector that occasionally runs and clears out unreachable objects.
5. Global Interpreter Lock (GIL)
A unique feature of CPython is the Global Interpreter Lock (GIL). This is a mutex that ensures only one thread executes Python bytecode at a time, which simplifies memory management in multi-threaded programs.
While it ensures thread safety, the GIL limits Python’s ability to leverage multi-core processors for CPU-bound tasks, as only one thread can execute at a time within a single process.
Workarounds include using multiprocessing or running external processes in parallel instead of relying solely on threads.
6. Modules and Import System
When you import a module, Python checks the following locations in order:
Built-in Modules: Python has a set of built-in modules, like
sys
andos
.PYTHONPATH: This environment variable contains paths where Python looks for modules.
Standard Library and Site-Packages: The standard library is a collection of modules included with Python, and site-packages is where external packages are installed.
Python uses a module cache to store loaded modules and avoid redundant imports. This cache is a dictionary called sys.modules
that stores references to each imported module.
7. Compilation and Interpreter Optimizations
The Python interpreter includes several optimizations to improve execution speed:
Constant Folding: This optimization involves evaluating constant expressions at compile time, so Python doesn't need to calculate values like
2 + 3
repeatedly.Short-Circuit Evaluation: Python takes advantage of logical short-circuiting (e.g., in
a and b
, ifa
is False,b
is not evaluated).Inline C Code: Some built-in operations are implemented in C to enhance performance.
8. Exception Handling and Tracebacks
Python includes a powerful exception-handling mechanism. When an exception is raised, the interpreter creates a traceback object that captures the call stack at the point where the exception occurred. The traceback provides details about the error, helping with debugging.
9. Abstract Syntax Trees (AST)
Python transforms source code into an Abstract Syntax Tree (AST) before compiling it into bytecode. The AST represents the syntactic structure of code, and Python’s ast
module allows for introspection and modification of this tree, enabling tools like linters, code formatters, and custom optimizations.
10. Standard Library and Built-in C Extensions
Python’s standard library includes both pure Python modules and C extensions. C extensions are typically written in C and compiled to binary form, allowing Python to run lower-level, performance-critical code.
Subscribe to my newsletter
Read articles from Cap Vision directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
