Exploring Python's dataclasses: Building Powerful and Concise Classes
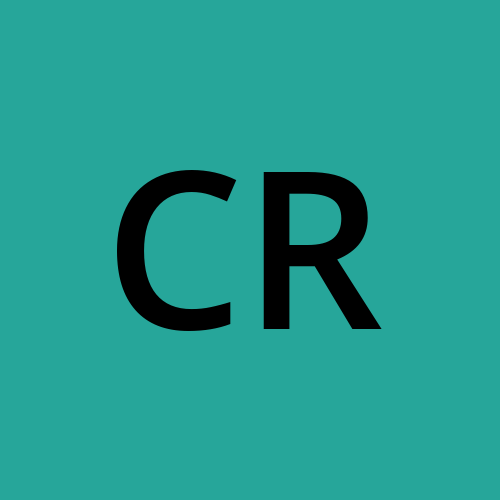
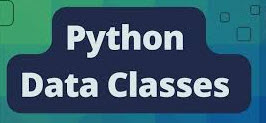
Introduction
Python's dataclasses module, introduced in Python 3.7, provides a concise way to create classes for managing data. With dataclasses, you can easily define classes that handle common tasks like comparisons, conversions to dictionaries, and more—all with minimal code. In this blog post, we’ll explore dataclasses through four practical examples: defining a Square with properties, comparing students based on attributes, and converting a Book instance into a dictionary and adding custom logic to the dataclass initialization process. Each example showcases useful features that simplify code for common tasks.
Here’s a quick overview of some of the methods that dataclasses generates for you:
__init__: Automatically creates an initializer based on the attributes you define, so you don’t need to manually write a constructor.
__repr__: Provides a readable string representation of the instance, useful for debugging (e.g., Book(title='The Great Gatsby', author='F. Scott Fitzgerald', pages=180)).
__str__: If not explicitly defined, __str__ defaults to the output of __repr__, but you can customize it if you want a different display format.
Example 1: A Square Class with Calculated Properties
Let’s start by creating a Square class that includes calculated properties for area, perimeter, and diagonal. Here, we’ll use dataclasses to store the side length and define methods to calculate these properties.
from dataclasses import dataclass
@dataclass
class Square:
_side: float
@property
def side(self):
return self._side
@side.setter
def side(self, value):
self._side = value
@property
def area(self):
return self._side ** 2
@property
def perimeter(self):
return 4 * self._side
@property
def diagonal(self):
return (2 ** 0.5) * self._side
# Create an instance of Square
square = Square(5)
# Test the properties
print("Side:", square.side) # Output: 5
square.side = 10 # Modify the side length
print("Area:", square.area) # Output: 100
print("Perimeter:", square.perimeter) # Output: 40
print("Diagonal:", square.diagonal) # Output: 14.142135623730951
Explanation:
In the Square class:
We use a private attribute _side to store the side length.
The
@property
decorator makes side accessible and modifiable like an attribute.area, perimeter, and diagonal are calculated properties, providing useful measurements based on the side length.
Using properties allows for a clean interface where values are calculated dynamically but accessed like simple attributes.
Example 2: Comparing Students with Custom Equality
In many cases, you may want to create objects that are comparable. In the following example, we create a Student dataclass where each student has a name, id, and gpa. By default, dataclasses automatically generate comparison methods for instances, including __eq__, which checks equality based on all attributes.
from dataclasses import dataclass
@dataclass(eq=True)
class Student:
name: str
id: int
gpa: float
# Example usage
student1 = Student("Alice", 101, 3.8)
student2 = Student("Alice", 101, 3.5)
print(student1 == student2) # Output: False (name and id are the same but gpa is different)
Explanation:
With eq=True (the default setting), the dataclass generates an __eq__ method, which compares all attributes of the Student class. In this example:
student1 and student2 have the same name and id, but different gpa.
Since all attributes are compared, student1 and student2 are not considered equal.
If you wanted to consider two students equal based only on name and id, you could customize the equality method. However, by default, all attributes are included in the comparison, which is helpful when each attribute is relevant to the comparison.
Example 3: Converting a Book Dataclass to a Dictionary
For many applications, you’ll need to convert an object into a dictionary, such as for JSON serialization. The dataclasses module provides a built-in function, asdict, which converts a dataclass instance into a dictionary. Let’s see how this works with a Book dataclass.
from dataclasses import dataclass, asdict
@dataclass
class Book:
title: str
author: str
pages: int
# Example usage
book = Book("The Great Gatsby", "F. Scott Fitzgerald", 180)
print(asdict(book)) # Output: {'title': 'The Great Gatsby', 'author': 'F. Scott Fitzgerald', 'pages': 180}
Explanation:
Here:
The asdict function takes a Book instance and converts it to a dictionary with the attribute names as keys and their values as dictionary values.
This is particularly useful when you need to convert data to JSON or pass it as a dictionary in API calls.
With asdict, you avoid manually constructing dictionaries, making the code more efficient and less error prone.
Example 4: Validating Attributes with __post_init__
In this example, we’ll create a Rectangle dataclass with attributes width and height. We’ll use __post_init__ to validate that both width and height are positive, as it wouldn’t make sense to have a rectangle with negative or zero dimensions. If either attribute is invalid, __post_init__ will raise a ValueError.
from dataclasses import dataclass
@dataclass
class Rectangle:
width: float
height: float
def __post_init__(self):
if self.width <= 0 or self.height <= 0:
raise ValueError("Both width and height must be positive.")
@property
def area(self):
return self.width * self.height
@property
def perimeter(self):
return 2 * (self.width + self.height)
# Example usage
try:
rect1 = Rectangle(5, 10)
print("Area:", rect1.area) # Output: 50
print("Perimeter:", rect1.perimeter) # Output: 30
# Attempt to create a rectangle with invalid dimensions
rect2 = Rectangle(-5, 10) # This will raise a ValueError
except ValueError as e:
print(e) # Output: Both width and height must be positive.
Explanation:
Initialization Validation: The __post_init__ method runs immediately after the __init__ method. It checks that width and height are positive. If either value is non-positive, __post_init__ raises a ValueError with a clear error message.
Dynamic Calculations: We added area and perimeter as properties that calculate their values based on width and height. These properties make it easy to access useful measurements without storing them directly.
Summary
The Python dataclasses module simplifies working with structured data. By using dataclasses, you can quickly create classes with custom properties, manage comparisons, and convert instances to dictionaries—all with minimal boilerplate code. Here’s a quick recap of what we covered:
Square Class with Properties: Calculated properties (area, perimeter, diagonal) make it easy to access derived measurements based on side.
Student Comparison: Using the default eq=True setting, we can compare all attributes of a Student or customize comparison behavior if needed.
Book to Dictionary: The asdict function converts dataclass instances to dictionaries, ideal for serialization or data manipulation.
Using __post_init__ ensures that any Rectangle instance created with invalid dimensions will fail at instantiation, providing immediate feedback on errors. This is a great way to add custom logic to your dataclass initialization process.
Using these techniques, you can build more powerful and concise classes, making your Python code cleaner, more readable, and easier to maintain. Experiment with dataclasses and you’ll find they’re an invaluable tool for managing structured data.
Subscribe to my newsletter
Read articles from Chandrasekar(Chan) Rajaram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
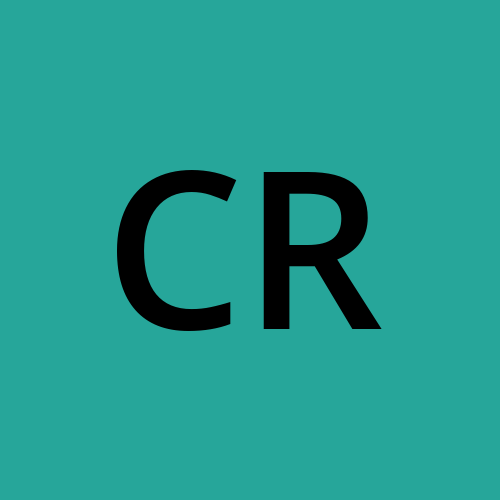