Flag, Is It Always Efficient?
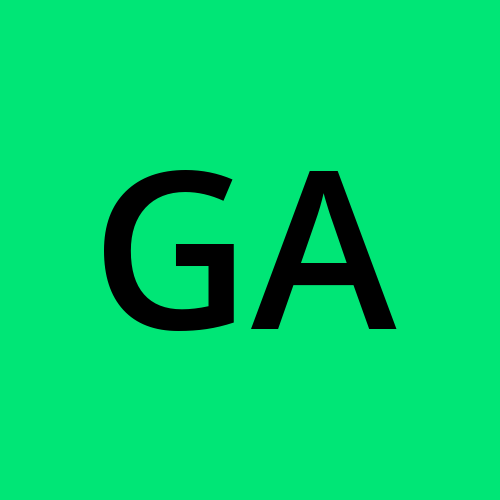
//variables
int value;
int numOfValue = 0;
int sum = 0;
int stop = 100;
int flag = 1;
//first code with flag
do
{
if (sum <= 100 && flag == 1 )
{
Console.Write("Enter the value: ");
value = Convert.ToInt32(Console.ReadLine());
sum += value;
numOfValue++;
}
else
{
flag = 0;
}
}
while (flag == 1);
Console.WriteLine("This is sum: " + sum);
Console.WriteLine("This is average: " + sum / numOfValue);
//second code without flag
numOfValue = 0;
sum = 0;
do
{
Console.Write("Enter the value: ");
value = Convert.ToInt32(Console.ReadLine());
sum += value;
numOfValue++;
}
while (sum <= 100);
Console.WriteLine("This is sum: " + sum);
Console.WriteLine("This is average: " + sum / numOf
Value);
Both code examples achieve the goal of summing user input values until the cumulative sum reaches or exceeds 100. However, each code has different advantages and limitations.
Comparison of the Two Codes
Logic Clarity and Efficiency:
First Code: The
flag
variable controls the loop, adding complexity. This variable (flag
) is unnecessary since the same result can be achieved by thewhile
condition. The first code also performs an extra check (sum <= 100 && flag == 1
) in each iteration, which slightly decreases efficiency.Second Code: This code is simpler, using only the condition
sum <= 100
in thewhile
loop to control the flow. This makes the code clearer and easier to maintain because there are fewer variables and conditions to track.
Control Flow:
First Code: This code’s use of a
flag
variable allows it to check the stopping condition within the loop body. This can be helpful if other logic needs to be added inside the loop, but it adds complexity.Second Code: The condition
sum <= 100
directly in the loop header is more efficient. However, it doesn't provide as much flexibility for further logic within the loop.
User Input Handling:
- Both Codes: Both codes handle user input similarly, repeatedly prompting the user for a value and updating
sum
andnumOfValue
.
- Both Codes: Both codes handle user input similarly, repeatedly prompting the user for a value and updating
Readability:
First Code: Slightly more complex because of the additional
flag
variable and conditional check within the loop.Second Code: More readable due to fewer conditions, making it more straightforward to understand the control flow.
Conclusion
The second code is generally better due to its simplicity and efficiency. It achieves the same result with fewer variables and conditions, making it easier to read, maintain, and understand. The first code could be preferable if you need flexibility to modify or add conditions within the loop, but otherwise, the second code is the better choice here.
Subscribe to my newsletter
Read articles from GaramYoon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
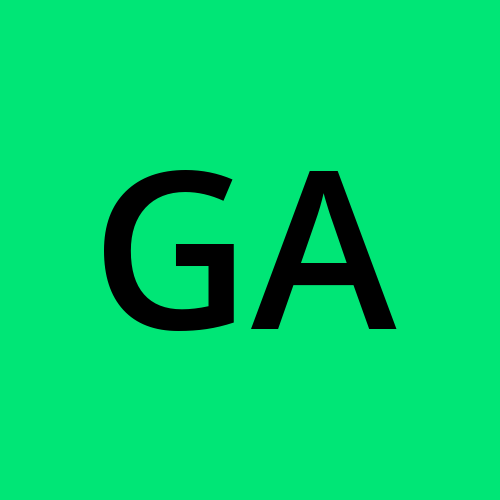