Building a Dynamic React Application with Routing

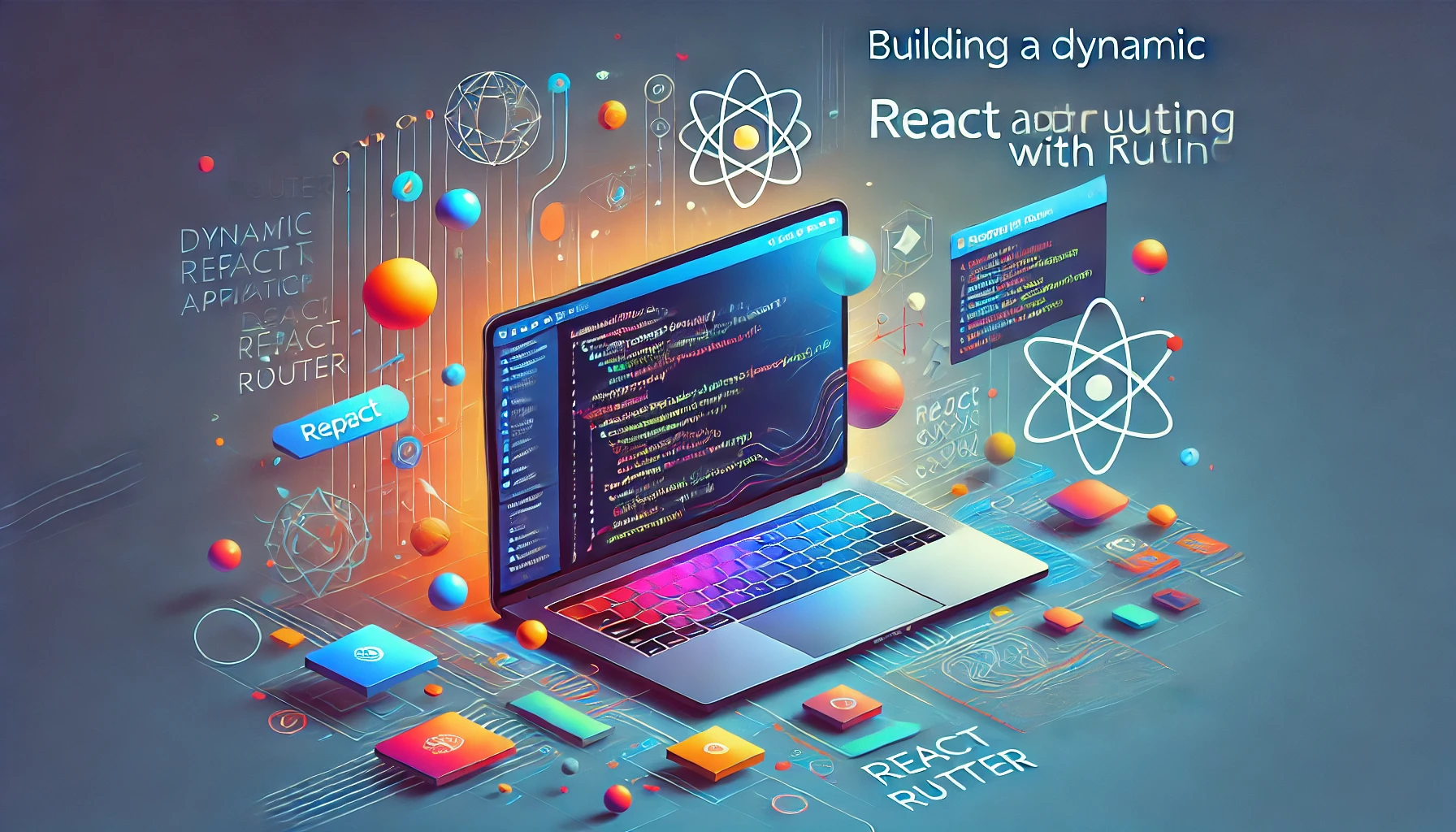
Hello, everyone! It’s Amar Jondhalekar, and today I’m excited to take you through building a dynamic React application using React Router. This will help you create a seamless user experience by navigating between different components easily. Let’s dive in!
What is React Router?
React Router is a powerful library that enables routing in React applications. It allows you to create a single-page application (SPA) where users can navigate through different views without refreshing the page. This is crucial for maintaining a smooth user experience.
Setting Up Your React Application
Before we get started, ensure you have a React application set up. You can create one using Create React App. Once you have your application ready, you need to install React Router.
npm install react-router-dom
Now, let’s jump into the code!
Code Walkthrough
App Component
First, let’s create the main App
component where we’ll set up our routes.
import './App.css';
import { createBrowserRouter, RouterProvider } from "react-router-dom";
import Dashboard from './components/Dashboard';
import Home from './components/Home';
import About from './components/About';
import Navbar from './components/Navbar';
import Params from './components/Params';
import MockTest from './components/MockTest';
import Reports from './components/Reports';
import Courses from './components/Courses';
import NotFound from './components/NotFound';
function App() {
const router = createBrowserRouter([
{
path: '/',
element: (
<div>
<Navbar />
<Home />
</div>
),
},
{
path: '/about',
element: (
<div>
<Navbar />
<About />
</div>
),
},
{
path: '/dashboard',
element: (
<div>
<Navbar />
<Dashboard />
</div>
),
children: [
{
path: 'courses',
element: <Courses />
},
{
path: 'mock-tests',
element: <MockTest />
},
{
path: 'reports',
element: <Reports />
}
]
},
{
path: '/student/:id',
element: (
<div>
<Navbar />
<Params />
</div>
),
},
{
path: '*',
element: <NotFound />
}
]);
return <RouterProvider router={router} />;
}
export default App;
Explanation of the Code
In this component, we’re defining our routes using createBrowserRouter
. Each route points to a specific component that will be displayed when the user navigates to that path.
Navbar Component
Next, let’s create a simple Navbar
component to allow users to navigate through the application easily.
import React from 'react';
import { NavLink } from 'react-router-dom';
import './Navbar.css';
const Navbar = () => {
return (
<div>
<ul>
<li>
<NavLink to="/" className={({ isActive }) => (isActive ? "active-link" : "")}>
Home
</NavLink>
</li>
<li>
<NavLink to="/about" className={({ isActive }) => (isActive ? "active-link" : "")}>
About
</NavLink>
</li>
<li>
<NavLink to="/dashboard" className={({ isActive }) => (isActive ? "active-link" : "")}>
Dashboard
</NavLink>
</li>
</ul>
</div>
);
};
export default Navbar;
This Navbar
component uses NavLink
from React Router, allowing you to highlight the active link easily.
Home and About Pages
Next, we have our Home
and About
components. They provide buttons to navigate to other parts of the application.
Home Component:
import React from 'react';
import { useNavigate } from 'react-router-dom';
const Home = () => {
const navigate = useNavigate();
function handleClick() {
navigate('/about');
}
return (
<div>
Home
<button onClick={handleClick}>
Move to About Page
</button>
</div>
);
}
export default Home;
About Component:
import React from 'react';
import { useNavigate } from 'react-router-dom';
const About = () => {
const navigate = useNavigate();
function handleClick() {
navigate('/dashboard');
}
return (
<div>
About
<button onClick={handleClick}>
Move to Dashboard
</button>
</div>
);
}
export default About;
Nested Routing with Dashboard
The Dashboard
component serves as a parent route, allowing us to define nested routes for courses, mock tests, and reports.
import React from 'react';
import { Outlet } from 'react-router-dom';
const Dashboard = () => {
return (
<div>
Dashboard
<Outlet />
</div>
);
}
export default Dashboard;
Creating Other Components
We’ll also create simple components for Courses
, MockTest
, Reports
, and a NotFound
page to handle unknown routes.
import React from 'react';
const Courses = () => {
return <div>Courses</div>;
}
export default Courses;
const MockTest = () => {
return <div>MockTest</div>;
}
export default MockTest;
const Reports = () => {
return <div>Reports</div>;
}
export default Reports;
const NotFound = () => {
return <div>404 - Page Not Found - Amar</div>;
}
export default NotFound;
Using Route Parameters
In our Params
component, we utilize the useParams
hook to access route parameters, making our application even more dynamic.
import React from 'react';
import { useParams } from 'react-router-dom';
const Params = () => {
const { id } = useParams();
return (
<div>
Params: {id}
</div>
);
}
export default Params;
Styling the Navbar
Lastly, let’s add some basic styles for our Navbar to make it visually appealing.
ul {
list-style: none;
display: flex;
justify-content: space-between;
padding-right: 10px;
background-color: beige;
}
.active-link {
color: aliceblue;
background-color: black;
}
Conclusion
And there you have it! You’ve successfully built a dynamic React application with routing. This tutorial covered everything from setting up routes to creating nested routes and handling parameters.
Feel free to experiment with the code and customize it to fit your project needs. If you have any questions or ideas, drop a comment below. Happy coding!
SEO Best Practices
Keywords: Incorporate relevant keywords like "React Router," "dynamic React application," and "building React apps" throughout the article.
Headers: Use clear and descriptive headers (H1, H2) to organize the content for easy navigation.
Meta Tags: Optimize the article with an engaging meta title and description to improve visibility in search results.
Subscribe to my newsletter
Read articles from Amar Jondhalekar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amar Jondhalekar
Amar Jondhalekar
👨💻 Amar Jondhalekar | Front End Web Developer at Cognizant | Content Creator | Founder at Campuslight With over 3 years of experience in web development, I specialize in HTML5, CSS3, JavaScript (ES6+), React.js, and Node.js. As a Full Stack Developer at Cognizant, I gained hands-on experience building dynamic, responsive applications focused on user-centric design and performance. I also founded Campuslight, where I create impactful digital solutions in the education sector, driven by a mission to make learning more accessible. Through my blogs, I share daily technical insights, coding tips, and career advice, aiming to inspire developers and impress recruiters alike. I’m dedicated to leveraging technology to create a more connected and accessible world. Let’s connect, collaborate, and make the web a better place for everyone!