Master Maven for DevOps: A Step-by-Step Guide from Beginners to Experts

Table of contents
- ๐ Introduction to Maven
- ๐ Key Maven Concepts
- ๐ Maven Build Lifecycle and Phases
- ๐ Working with Plugins
- ๐ ๏ธ Maven Profiles
- โ๏ธ Dependency Scopes
- ๐ Using Maven in CI/CD Pipelines
- ๐ก Best Practices for Using Maven in DevOps
- ๐ Troubleshooting Common Maven Issues
- ๐ Maven Commands Quick Reference
- ๐ฅ๏ธ Installing Maven on Linux/Ubuntu
- Wrapping Up
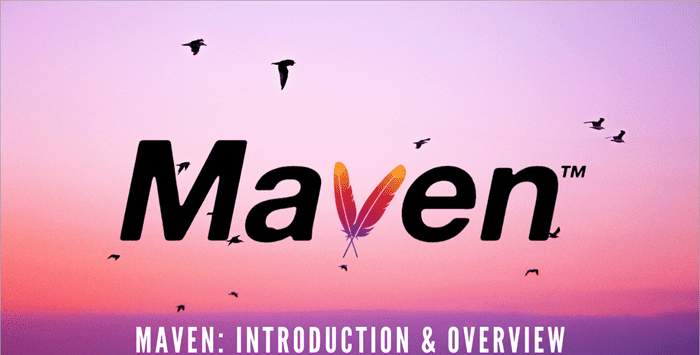
If you're diving into DevOps, you've likely come across Mavenโa powerful build automation tool primarily for Java projects. In this guide, Iโll walk you through the essentials of Maven, from setting it up to using it in CI/CD pipelines.
๐ Introduction to Maven
What is Maven?
Maven is an open-source tool used to automate builds, manage dependencies, and streamline development workflows for Java projects (and even other languages). It simplifies complex DevOps workflows, helping developers standardize project builds and manage project dependencies seamlessly.
Why Use Maven in DevOps?
Standardized Build Process: Consistent builds across teams and projects.
CI/CD Integration: Easily integrates with tools like Jenkins, automating builds and testing.
Dependency Management: Maven ensures all team members work with the same dependencies and versions, reducing conflicts.
๐ Key Maven Concepts
1. Project Object Model (POM)
Maven configurations are defined in a pom.xml
file, which is the core of any Maven project. This file contains:
Project coordinates (like
groupId
,artifactId
,version
).Dependencies: All external libraries needed by the project.
Plugins and build configurations.
Basic pom.xml
Structure:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0</version>
<dependencies>
<!-- Dependency definitions go here -->
</dependencies>
</project>
2. Dependency Management
Maven automatically downloads required libraries from central repositories.
Example Dependency in pom.xml
:
xmlCopy code<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
3. Repositories
Local Repository: Default location on your machine (
~/.m2/repository
).Central Repository: Mavenโs official online repository.
Remote Repository: Custom repositories for internal projects.
๐ Maven Build Lifecycle and Phases
Mavenโs build lifecycle has several stages:
clean: Cleans up previous build files.
default (or build): The main build phases.
site: Generates project documentation.
Key Build Phases:
validate
,compile
,test
,package
,install
,deploy
Common Commands:
mvn clean # Cleans the target directory
mvn compile # Compiles the source code
mvn test # Runs unit tests
mvn package # Packages compiled code into a JAR/WAR file
mvn install # Installs the package into the local repository
mvn deploy # Deploys the package to a remote repository
๐ Working with Plugins
Plugins extend Mavenโs capabilities. Commonly used plugins include:
maven-compiler-plugin: Manages Java compilation settings.
maven-surefire-plugin: Configures testing.
maven-jar-plugin: Configures JAR file creation.
Plugin Example:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
๐ ๏ธ Maven Profiles
Maven profiles are helpful for configuring different build environments (like dev, test, and production).
Profile Example:
<profiles>
<profile>
<id>dev</id>
<properties>
<environment>development</environment>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<environment>production</environment>
</properties>
</profile>
</profiles>
Activate a profile using:
mvn clean install -Pdev
โ๏ธ Dependency Scopes
Dependency scopes define when and where dependencies are available:
compile: Default scope, available everywhere.
test: Only during testing.
provided: Available for compile and test but expected to be provided by the runtime environment.
runtime: Available only during runtime.
๐ Using Maven in CI/CD Pipelines
Jenkins Integration
You can easily integrate Maven with Jenkins to automate your DevOps workflow, allowing Jenkins to execute Maven commands like mvn clean install
.
Docker with Maven
Using Docker with Maven creates a consistent environment across development and production:
FROM maven:3.8.4-jdk-11
WORKDIR /app
COPY . .
RUN mvn clean package
๐ก Best Practices for Using Maven in DevOps
Centralized Dependency Management: Use
pom.xml
to manage dependencies and avoid conflicts.Specific Plugin Versions: Define plugin versions explicitly for consistent builds.
Environment-Specific Profiles: Leverage profiles for different environments.
Automate with CI/CD: Integrate Maven in CI/CD pipelines for efficient DevOps.
๐ Troubleshooting Common Maven Issues
1. Dependency Conflicts
Use mvn dependency:tree
to identify conflicting versions. You can exclude dependencies if necessary.
2. Build Failures
Check the error logs for details. Use -X
for detailed debugging info.
3. Repository Access Issues
Ensure you have access to Mavenโs repositories. If behind a firewall, configure a proxy in settings.xml
.
๐ Maven Commands Quick Reference
Command | Description |
mvn clean | Cleans the project by deleting target directory |
mvn compile | Compiles the project source code |
mvn test | Runs tests |
mvn package | Packages the compiled code into JAR/WAR |
mvn install | Installs the package into the local repository |
mvn deploy | Deploys the package to a remote repository |
mvn dependency:tree | Displays the dependency tree |
mvn -P<profile> | Runs Maven with a specific profile |
mvn help:effective-pom | Displays the effective POM with resolved configs |
๐ฅ๏ธ Installing Maven on Linux/Ubuntu
Install Java:
$ wget https://download.java.net/java/GA/jdk13.0.1/openjdk-13.0.1_linux-x64_bin.tar.gz $ tar -xvf openjdk-13.0.1_linux-x64_bin.tar.gz $ mv jdk-13.0.1 /opt/
Set JAVA_HOME:
JAVA_HOME='/opt/jdk-13.0.1' PATH="$JAVA_HOME/bin:$PATH" export PATH
Download and Install Maven:
$ wget https://dlcdn.apache.org/maven/maven-3/3.9.9/binaries/apache-maven-3.9.9-bin.tar.gz $ tar -xvf apache-maven-3.9.9-bin.tar.gz $ mv apache-maven-3.9.9 /opt/
Set M2_HOME:
M2_HOME='/opt/apache-maven-3.9.9' PATH="$M2_HOME/bin:$PATH" export PATH
Wrapping Up
Maven is a powerful tool for managing Java projects, especially in a DevOps setting. With standardized builds, automated testing, and easy CI/CD integration, Maven can boost your efficiency. Try integrating it into your workflow and enjoy streamlined project builds!
Feel free to comment below if you have questions or share your own Maven tips and tricks. Happy coding!
Subscribe to my newsletter
Read articles from Rohit Jangra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
