Ruby on Rails 8 Concurrency Guide: Modern Parallel Processing

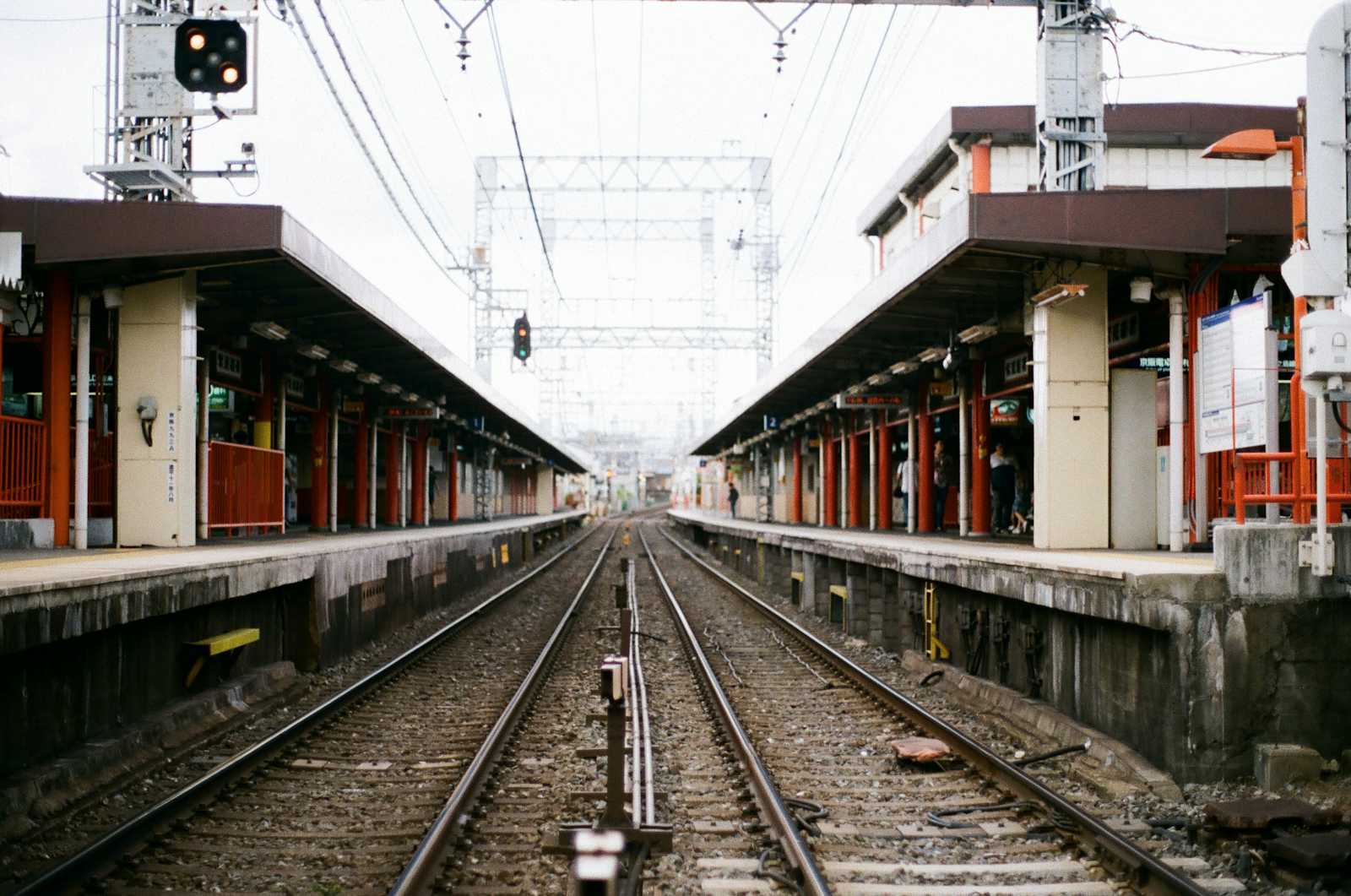
In today's digital landscape, web applications face unprecedented demands for performance, scalability, and responsiveness.
Modern Ruby on Rails applications must handle multiple concurrent users, process large amounts of data, and maintain real-time connections while delivering a seamless user experience.
Ruby on Rails 8 rises to these challenges with enhanced support for concurrency and parallelism, providing developers with powerful tools to build high-performance applications.
How Rails 8 Enhances Support for Concurrent and Parallel Processes
Rails 8 introduces significant improvements in its concurrency model, building upon Ruby's evolving parallel processing capabilities.
The framework now offers better integration with Ruby's native concurrency features, including enhanced support for Ractors, Fibers, and thread management.
These improvements enable developers to write more efficient code that can take full advantage of modern multi-core processors while maintaining Rails' developer-friendly approach.
Current Usage of Concurrency in Rails 8
Async Features in Rails 8 for Efficient I/O Operations
While Rails 8 itself does not have built-in support for native async/await patterns, developers can leverage third-party libraries like the async
and async-await
gems to enable asynchronous processing capabilities.
The async
gem provides a framework for writing asynchronous code in Ruby, and the async-await
gem builds on top of it to provide a more familiar async/await syntax.
Here's an example of how you can use these gems to implement async controller actions in a Rails 8 application:
# Gemfile
gem 'async'
gem 'async-await'
# app/controllers/users_controller.rb
class UsersController < ApplicationController
include AsyncHelper
async def index
@users = await User.async_find_all
@posts = await Post.async_find_recent
respond_to do |format|
format.json { render json: { users: @users, posts: @posts } }
end
end
end
# app/helpers/async_helper.rb
module AsyncHelper
extend ActiveSupport::Concern
class_methods do
def async_find_all
Async do
User.all.to_a
end
end
def async_find_recent
Async do
Post.where('created_at > ?', 24.hours.ago).to_a
end
end
end
end
In this example, we're using the async
and async-await
gems to enable asynchronous processing in the UsersController#index
action. The AsyncHelper
module provides the async_find_all
and async_find_recent
methods, which wrap the database queries in an Async
block.
This allows the queries to be executed concurrently, improving the overall response time of the controller action. This is particularly beneficial for I/O-bound operations, such as database queries, where the application can perform other tasks while waiting for the results.
Leveraging Ractors and Fibers in Rails Applications for Performance Gains
Ractors, introduced in Ruby 3.0 and now fully supported in Rails 8, provide true parallel execution capabilities.
They enable safe parallel execution of Ruby code while maintaining thread safety through message passing.
Here's an example of using Ractors for parallel data processing:
class DataProcessor
def self.parallel_process(data_chunks)
ractors = data_chunks.map do |chunk|
Ractor.new(chunk) do |data|
# Complex processing logic
result = data.map do |item|
# Transform data
processed_item = heavy_computation(item)
processed_item
end
result
end
end
# Collect results from all Ractors
ractors.map(&:take)
end
private
def self.heavy_computation(item)
# Simulate complex processing
sleep(0.1)
item * 2
end
end
# Usage
data = (1..100).to_a
chunks = data.each_slice(25).to_a
results = DataProcessor.parallel_process(chunks)
This code demonstrates parallel processing using Ractors. The DataProcessor
class splits data into chunks and processes them in parallel using multiple Ractors. Each Ractor performs a heavy computation independently, and the results are collected using take
. This is particularly useful for CPU-intensive tasks that can benefit from true parallel execution.
Background Jobs and ActiveJob Updates for Managing Concurrency
Rails 8 introduces improvements to ActiveJob, making it easier to handle background processing with better concurrency control.
The framework now includes enhanced job scheduling and improved error handling for concurrent job execution.
Here's an example:
class ProcessUserDataJob < ApplicationJob
queue_as :default
def perform(user_id)
user = User.find(user_id)
# Use connection pool for concurrent database access
ActiveRecord::Base.connection_pool.with_connection do
process_user_data(user)
end
end
private
def process_user_data(user)
# Process user data concurrently
Parallel.map(user.data_points, in_threads: 4) do |data_point|
process_data_point(data_point)
rescue StandardError => e
Rails.logger.error("Error processing data point: #{e.message}")
nil
end
end
end
This ActiveJob implementation shows how to handle concurrent database operations safely. It uses connection pooling to manage database connections and implements parallel processing of user data points using threads. The code includes error handling to ensure failed operations don't crash the entire job.
Advanced Applications of Concurrency and Parallelism
Best Practices for Implementing Parallel Data Processing in Rails 8
When implementing parallel data processing in Rails 8, it's crucial to follow best practices that ensure both performance and data consistency.
The framework provides several patterns for handling large-scale data processing efficiently.
Here’s an example:
class BatchProcessor
def self.process_in_parallel(records, batch_size: 1000)
records.in_batches(of: batch_size).each_with_index do |batch, index|
Rails.logger.info("Processing batch #{index + 1}")
ActiveRecord::Base.connection_pool.with_connection do
Parallel.each(batch, in_threads: 4) do |record|
begin
process_record(record)
rescue StandardError => e
Rails.error.handle(e, context: { record_id: record.id })
end
end
end
end
end
private
def self.process_record(record)
# Record processing logic
record.with_lock do
# Ensure thread-safe updates
record.process!
end
end
end
The BatchProcessor
shows how to handle large datasets by processing them in batches concurrently. It uses in_batches
to break down the data, connection pooling for database access, and parallel processing with proper error handling. The use of with_lock
ensures thread-safe record updates.
Achieving Real-Time Data Handling with Concurrent Websockets (ActionCable)
Rails 8's ActionCable system has been enhanced to handle concurrent WebSocket connections more efficiently.
Here's an example of implementing a real-time dashboard with concurrent user updates:
# app/channels/dashboard_channel.rb
class DashboardChannel < ApplicationCable::Channel
def subscribed
stream_from "dashboard_updates"
# Initialize concurrent data processing
@processing_fiber = Fiber.new do
loop do
process_updates
Fiber.yield
end
end
end
def receive(data)
# Handle incoming messages concurrently
Async do
processed_data = process_message(data)
broadcast_to "dashboard_updates", processed_data
end
end
private
def process_message(data)
# Process message in parallel using Ractor
Ractor.new(data) do |msg|
# Transform message data
result = perform_heavy_computation(msg)
result
end.take
end
end
This code shows real-time WebSocket handling using ActionCable with concurrent processing. It uses Fibers for continuous processing and Ractors for handling incoming messages in parallel. The channel implements subscription handling and concurrent message processing for real-time dashboard updates.
Utilizing Redis and Cache Stores for Parallel Data Access
Rails 8 optimizes cache access patterns for concurrent operations, particularly when using Redis as a cache store.
Here's an example of implementing a distributed caching system with parallel access:
class CacheManager
def self.parallel_cache_fetch(keys)
# Create a connection pool for Redis
redis_pool = ConnectionPool.new(size: 5, timeout: 5) do
Redis.new(url: ENV['REDIS_URL'])
end
# Fetch multiple keys in parallel
Parallel.map(keys, in_threads: 5) do |key|
redis_pool.with do |redis|
cached_value = redis.get(key)
if cached_value.nil?
value = yield(key)
redis.set(key, value.to_json, ex: 1.hour.to_i)
value
else
JSON.parse(cached_value)
end
end
end
end
end
# Usage
keys = ['user_stats', 'system_metrics', 'performance_data']
results = CacheManager.parallel_cache_fetch(keys) do |key|
# Compute value if not in cache
compute_expensive_value(key)
end
The CacheManager
demonstrates parallel cache access using Redis with a connection pool. It fetches multiple keys concurrently using threads, implements cache miss handling, and manages Redis connections efficiently. The connection pool prevents resource exhaustion when dealing with multiple concurrent requests.
Concurrency Pitfalls and Optimization Strategies
Handling Race Conditions and Data Consistency Challenges
When working with concurrent operations, maintaining data consistency is paramount.
Rails 8 provides several mechanisms to handle race conditions and ensure data integrity:
Optimistic/Pessimistic Locking: Rails offers both locking strategies to prevent concurrent modifications from creating inconsistent data.
Database Transactions: Proper use of transactions ensures atomic operations across multiple records.
Connection Pool Management: Careful management of database connections prevents connection starvation in concurrent scenarios.
Optimizing Resource Use with Thread-Safe Code Practices in Rails 8
Writing thread-safe code is essential for reliable concurrent applications. Key practices include:
Using thread-local variables when appropriate
Implementing proper mutex locks for shared resources
Avoiding global state modifications
Utilizing atomic operations for counters and flags
Profiling and Debugging Concurrent Code for Peak Performance
Rails 8 includes improved tools for profiling and debugging concurrent code:
Enhanced logging for concurrent operations
Better stack traces for async/await operations
Improved visibility into Ractor states and communication
More detailed performance metrics for parallel processes
Conclusion
The future of concurrency in Ruby on Rails looks promising, with Rails 8 setting a strong foundation for handling parallel processing and concurrent operations.
As we look forward to Rails 9, we can expect:
Further improvements in Ractor integration
Enhanced async/await patterns
Better tools for managing concurrent resources
More sophisticated parallel processing capabilities
Developers working with Rails 8 should focus on:
Understanding the appropriate use cases for different concurrency tools
Implementing proper error handling and recovery mechanisms
Maintaining data consistency in concurrent operations
Optimizing resource usage and performance
Preparing for future concurrent processing capabilities
By mastering these concepts and implementing them effectively, developers can build robust, high-performance Ruby on Rails applications that meet the demands of modern web development.
Follow the Rails Guides on “Threading and Code Execution in Rails” to properly implement concurrency and parallelism in your applications.
Subscribe to my newsletter
Read articles from BestWeb Ventures directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

BestWeb Ventures
BestWeb Ventures
From cutting-edge web and app development, using Ruby on Rails, since 2005 to data-driven digital marketing strategies, we build, operate, and scale your MVP (Minimum Viable Product) for sustainable growth in today's competitive landscape.