Flutter trick: using AnimatedBuilder for efficient animations.
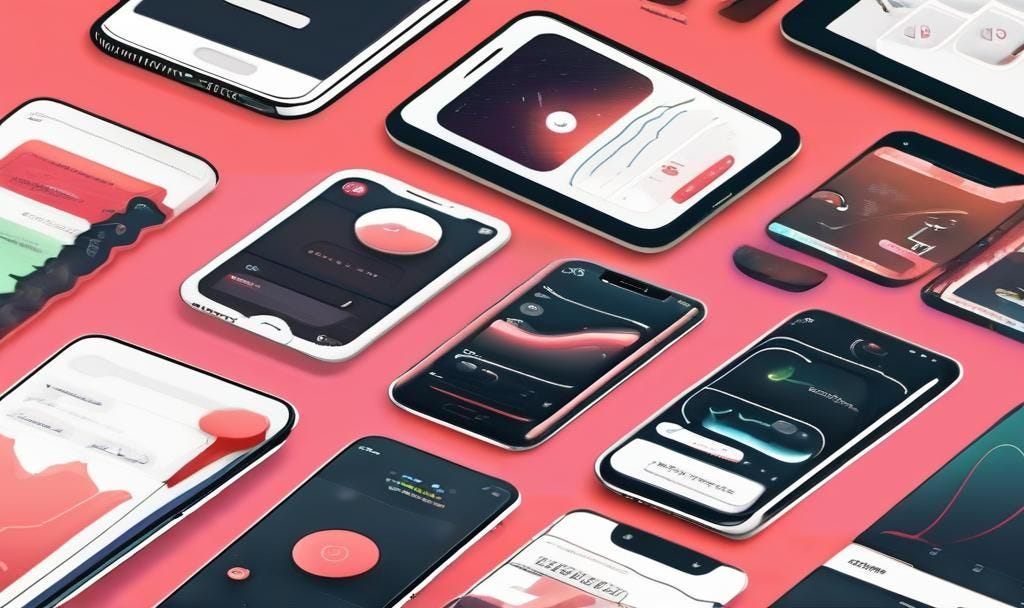
When you want to add animations that don’t rebuild the entire widget tree, AnimatedBuilder is an efficient way to create smooth, high-performance animations.
Example: Rotating Icon Animation
This example shows how to use AnimatedBuilder to smoothly rotate an icon without rebuilding unnecessary parts of the widget tree:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Rotating Icon")),
body: Center(child: RotatingIcon()),
),
);
}
}
class RotatingIcon extends StatefulWidget {
@override
_RotatingIconState createState() => _RotatingIconState();
}
class _RotatingIconState extends State<RotatingIcon>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(); // Repeats indefinitely
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _controller,
builder: (context, child) {
return Transform.rotate(
angle: _controller.value * 2.0 * 3.1416, // Full circle
child: child,
);
},
child: Icon(
Icons.sync,
size: 100,
color: Colors.blue,
),
);
}
}
Explanation
• AnimationController: Controls the animation’s duration and state (we set it to repeat indefinitely here).
• AnimatedBuilder: Efficiently rebuilds only the parts of the widget that need updating, keeping performance smooth.
• Transform.rotate: Rotates the icon based on the controller’s current animation value.
Using AnimatedBuilder like this is a highly efficient way to add animations to your Flutter app without rebuilding the widget tree, which keeps animations smooth and improves app performance.
Subscribe to my newsletter
Read articles from Reme Le Hane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by