A Comprehensive Guide to QClasses: Generation, Functionality, and Usage with Gradle and Maven

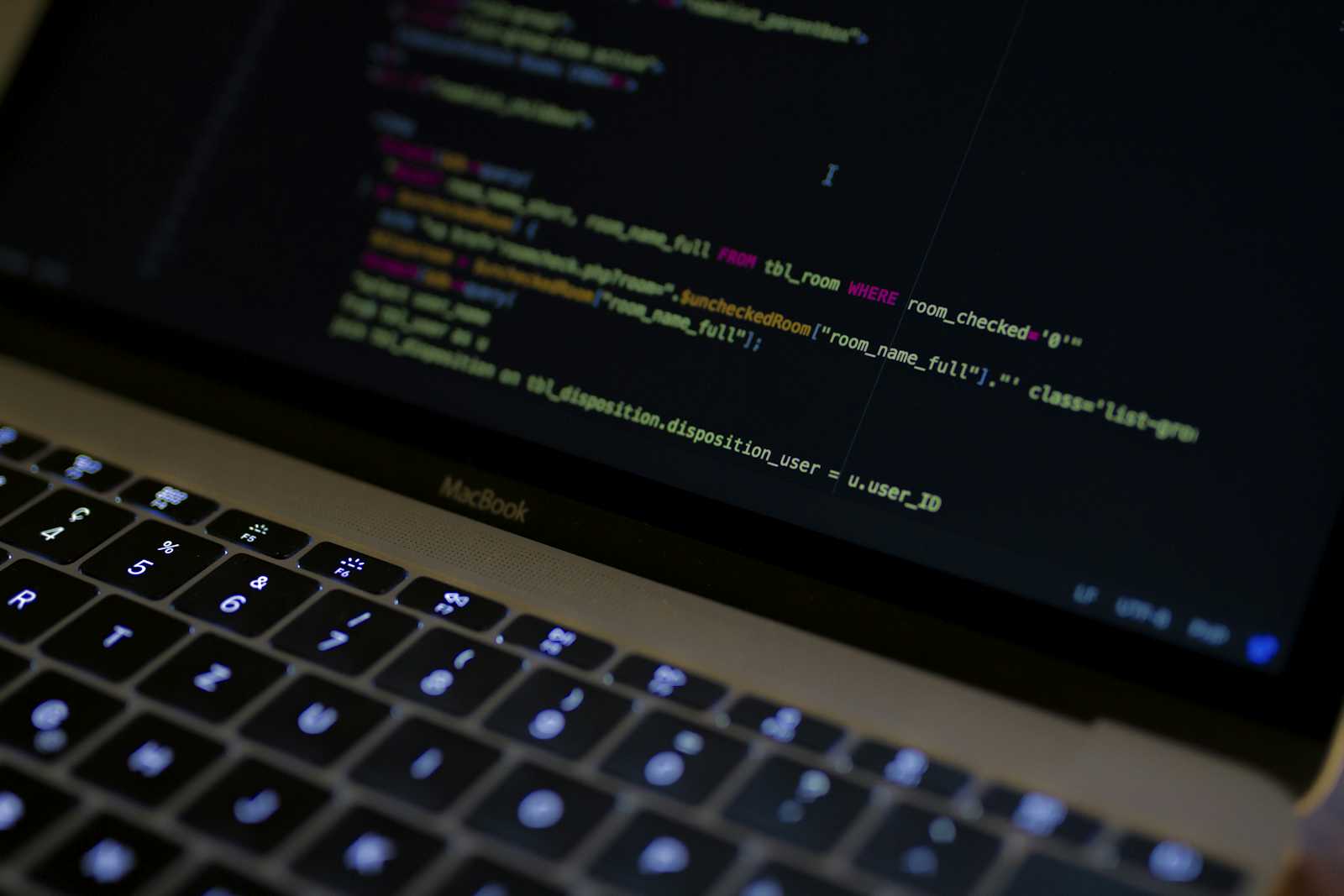
In Java development, QClasses play a crucial role in enabling efficient querying and data manipulation, especially when working with object-relational mapping (ORM) frameworks like Querydsl. This blog will walk you through everything you need to know about QClasses: how they are generated, how they work, and how to integrate them into your project using Gradle and Maven.
What Are QClasses?
QClasses are generated types (classes) that represent your entities in a Querydsl query. They provide a type-safe way to build dynamic queries using Java code instead of writing raw SQL queries. This significantly reduces the risk of errors, improves code readability, and enables IDE auto-completion, making it easier to work with complex queries.
In essence, QClasses map your database entities to Java objects that allow you to create type-safe queries. These classes are typically prefixed with a "Q" followed by the entity name (e.g., QUser for a User entity).
How Are QClasses Generated?
QClasses are automatically generated by Querydsl during the build process. The process generally involves:
Annotating Your Entity Classes:
You use annotations like @Entity
to mark your domain model classes. For Querydsl, the @QueryEntity
annotation is used, though many frameworks like Hibernate automatically integrate Querydsl functionality without explicit annotations.
Executing the Querydsl Annotation Processor:
During compilation, the Querydsl annotation processor scans the classes annotated with @Entity
or @QueryEntity
and generates corresponding QClasses in a specified package (usually under target/generated-sources or build/generated).
For each entity class, a corresponding QClass is generated.@Entity
public class User {
@Id
private Long id;
private String name;
private String email;
}
Querydsl will generate a class named QUser:@Generated("com.querydsl.codegen.EntitySerializer")
public class QUser extends EntityPathBase {
public static final QUser user = new QUser("user");
public final StringPath name = createString("name");
public final StringPath email = createString("email");
public QUser(String variable) {
(User.class, forVariable(variable));
}
public QUser(Path<? extends User> path) {
super(path.getType(), path.getMetadata());
} } This QUser class allows you to construct type-safe queries
QUser user = QUser.user;
JPAQueryFactory queryFactory = new JPAQueryFactory(entityManager);
List users = queryFactory.selectFrom(user).where(
user.name
.eq("John")) .fetch();
How Do QClasses Work?
- Type-Safe Queries: QClasses provide a strongly-typed API to build queries, which helps avoid runtime errors and typos that may occur when writing raw SQL.
For example, using the generated QUser class, you can build a query like this:List users = queryFactory.selectFrom(QUser.user).where(
QUser.user.name
.eq("Alice"))
.fetch();
In this query, QUser.user.name refers to the name property of the User entity, ensuring that the query is both readable and type-safe.
- Fluent API for Query Construction: QClasses allow you to create queries with a fluent API, making it easier to construct dynamic queries without string concatenation. For instance, you can dynamically add conditions based on user input, like:
BooleanBuilder builder = new BooleanBuilder();
if(name != null){ builder.and(
QUser.user.name
.eq(name));
} if (email != null) {
builder.and(
QUser.user.emai
l.eq(email));
}
List results = queryFactory.selectFrom(QUser.user).where(builder)
.fetch();
3. Cross-Platform Support: Querydsl (and consequently QClasses) supports multiple database backends (JPA, SQL, MongoDB, and more). This makes it a highly versatile tool for querying various data sources.
Why Use QClasses? QClasses are beneficial for several reasons:
Type Safety: Since QClasses are generated based on your entity classes, they eliminate potential runtime errors caused by typos or incorrect data types in queries.
Improved Readability: Using QClasses and a fluent API makes the code more readable compared to string-based queries.
IDE Support: Modern IDEs like IntelliJ and Eclipse can provide auto-completion, making it easier to write and maintain queries.
Reusable Queries: Queries built using QClasses can be reused across the application, reducing code duplication.
Working with QClasses Using Gradle To integrate Querydsl with Gradle, you need to set up a few things in your build.gradle file.
Step 1:
Add Dependencies First, add the necessary dependencies for Querydsl and JPA to your build.gradle:
groovyplugins {
id 'java' id 'org.springframework.boot' version '3.0.0'
id 'io.spring.dependency-management' version '1.0.13.RELEASE'
}
dependencies {
implementation 'com.querydsl:querydsl-jpa:5.0.0'
annotationProcessor 'com.querydsl:querydsl-apt:5.0.0:jpa'
implementation 'javax.persistence:javax.persistence-api:2.2'
}
Step 2:
Configure Annotation Processing To enable annotation processing, configure the querydsl source set to generate QClasses in a specific directory: sourceSets { main { java { srcDirs = ['src/main/java', 'build/generated'] } } }
compileJava {
options.annotationProcessorPath = configurations.annotationProcessor
}
Step 3:
Run the Build After setting up the configuration, run the Gradle build task to generate the QClasses:
./gradlew build
The QClasses will be generated in the build/generated directory and can be used in your application.
Working with QClasses Using Maven To work with QClasses in Maven, follow these steps.
Step 1:
Add Dependencies Add the following dependencies to your pom.xml:
com.querydsl querydsl-jpa 5.0.0
javax.persistence
javax.persistence-api 2.2
Step 2:
Configure the Annotation Processor Add the Querydsl annotation processor to the build section of your pom.xml:com.mysema.maven apt-maven-plugin 1.1.3 process target/generated-sources com.querydsl.apt.jpa.JPAAnnotationProcessor
Step 3:
Run the Build Once your configuration is complete, run Maven to build the project and generate the QClasses:
bash mvn clean install
The QClasses will be generated under target/generated-sources and can be referenced in your code.
Conclusion
QClasses offer a type-safe and efficient way to construct queries in Java applications. Whether you're using Gradle or Maven, the integration of Querydsl into your project ensures that complex database queries can be built with ease and confidence. By following the steps outlined in this blog, you can seamlessly generate and use QClasses, helping you to write cleaner, more maintainable code.
Subscribe to my newsletter
Read articles from Njunji Muchai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
