Exploring Maps and Sets: Advanced Collections!
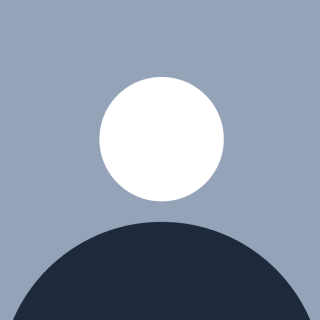
Table of contents
- What Are Maps?
- What Are Sets?
- Flowchart: The Garden of Maps and Sets
- Challenge: Create a Unique Item Tracker with Sets
- Growing Your Collection Skills
- Advanced Methods and Use Cases for Maps and Sets
- Advanced Methods for Maps
- Advanced Methods for Sets
- Combining Maps and Sets for Powerful Data Management
- Challenge Time — Advanced Maps and Sets
- Conclusion — Advanced Data Management with Maps and Sets
- Get ready for Function Frenzy!
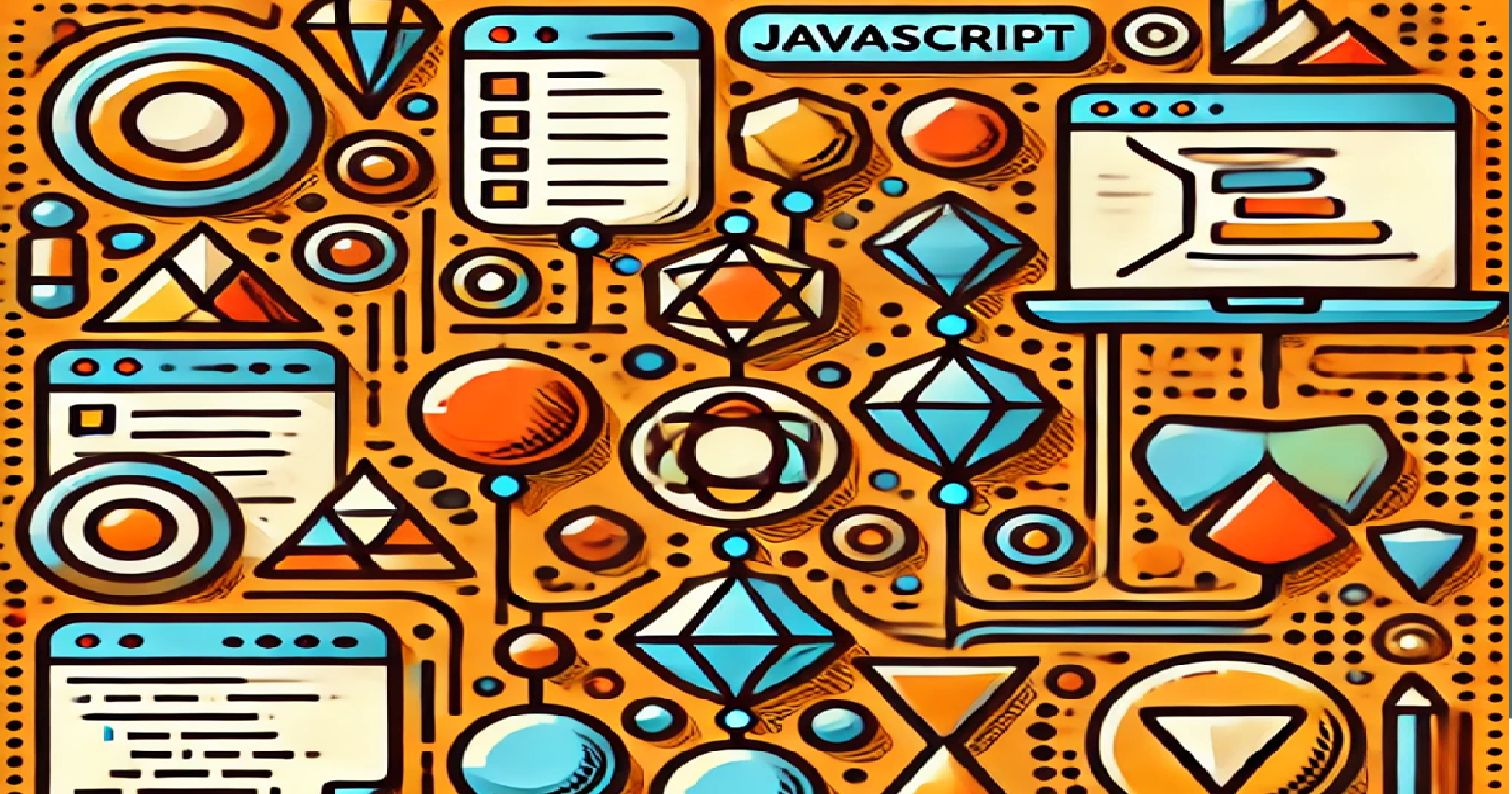
Imagine you’ve stepped into a beautiful garden, where every flower is unique, and every tree has a label mapping its species to its description. In this garden, Sets are like patches of rare flowers—no duplicates allowed—while Maps are like the trees with plaques that tell you exactly what each one is. Maps and Sets are powerful data structures in JavaScript that allow you to manage data in unique and efficient ways.
In this article, we’ll explore Maps and Sets, understanding how to create, use, and benefit from them in JavaScript. Whether you need to store unique items, map keys to values, or ensure no duplicates, these advanced collections are your go-to tools.
Let’s start by planting the seeds of knowledge and dive into Maps and Sets!
What Are Maps?
A Map is a collection of key-value pairs where each key is unique. You can think of a Map as a tree in our garden, where each tree (key) has a label (value) attached to it. Unlike objects, Maps allow any type of key (not just strings), and they preserve the order of the elements.
Maps are particularly useful when you need to associate data with specific keys, allowing for quick lookups, updates, and deletions.
Key Features of Maps:
Keys can be any type (objects, functions, or primitives).
Maps remember the original insertion order of the keys.
Maps provide easy access to methods like
get()
,set()
, anddelete()
.
Code Snippet: Creating and Using Maps
Let’s create a Map in our garden to keep track of different tree species and their descriptions.
// Create a new Map
let treeMap = new Map();
// Add key-value pairs
treeMap.set("Oak", "A sturdy tree with strong branches.");
treeMap.set("Maple", "A tree known for its beautiful fall foliage.");
treeMap.set("Cherry", "A tree that blossoms with delicate flowers in the spring.");
// Access values
console.log(treeMap.get("Oak")); // Output: "A sturdy tree with strong branches."
// Check if a key exists
console.log(treeMap.has("Maple")); // Output: true
// Remove a key-value pair
treeMap.delete("Cherry");
console.log(treeMap.size); // Output: 2
Explanation:
We created a
treeMap
to store tree names as keys and descriptions as values.set()
adds a new key-value pair,get()
retrieves a value by key, anddelete()
removes a key-value pair.
With Maps, you have a flexible tool for managing pairs of related data, perfect for situations where the order of insertion matters or when you need non-string keys.
What Are Sets?
While Maps are used for key-value pairs, a Set is a collection of unique values, where each value can only appear once. Sets are like flowerbeds in our garden where no two flowers are alike. They ensure that every element is unique and provide fast checks for the presence of an item.
Key Features of Sets:
A Set can store any type of value (primitives or objects).
Sets automatically remove duplicates.
Sets are useful when you need to ensure all values are distinct.
Code Snippet: Creating and Using Sets
Let’s plant a flowerbed of unique flowers using a Set:
// Create a new Set
let flowerSet = new Set();
// Add values
flowerSet.add("Rose");
flowerSet.add("Tulip");
flowerSet.add("Daisy");
flowerSet.add("Rose"); // Duplicate value, won't be added again
// Check if a value exists
console.log(flowerSet.has("Tulip")); // Output: true
// Remove a value
flowerSet.delete("Daisy");
// Get the size of the Set
console.log(flowerSet.size); // Output: 2
Explanation:
We created a
flowerSet
to store unique flower names.The
add()
method adds elements, but duplicates are automatically ignored.The
has()
method checks if a specific flower exists, whiledelete()
removes it.
Sets are ideal when you need to ensure uniqueness, such as keeping track of unique items in a collection, making them perfect for cases like managing a unique guest list or tracking unique user IDs.
Flowchart: The Garden of Maps and Sets
Here’s a flowchart to visualize how Maps and Sets work:
[ Start ]
|
V
[ Map ]
|
Key -> Value (Tree -> Description)
|
get(), set(), delete()
|
[ Set ]
|
Value (Flower)
|
add(), has(), delete()
Maps manage key-value pairs where each key maps to a unique value, while Sets manage individual values, ensuring no duplicates.
Challenge: Create a Unique Item Tracker with Sets
Let’s put what you’ve learned into practice with a challenge. Imagine you’re managing a garden where each flower must be unique. Your task is to build a unique item tracker using Sets to track the different types of flowers in the garden.
Challenge Instructions:
Initialize a Set to store flower types.
Add new flower types using
add()
.Check if a flower type is already in the Set using
has()
.Display the total number of unique flowers using
size
.
Here’s a starter example for your flower tracker:
let flowerSet = new Set();
// Add flowers
flowerSet.add("Rose");
flowerSet.add("Lily");
flowerSet.add("Sunflower");
flowerSet.add("Rose"); // Duplicate, won't be added
// Check if a flower exists
console.log(flowerSet.has("Sunflower")); // Output: true
// Display total number of unique flowers
console.log(flowerSet.size); // Output: 3
By the end of this challenge, you’ll have created a simple system that tracks unique items—whether it’s flowers, usernames, or any other data that needs to stay distinct.
Growing Your Collection Skills
In this part, you’ve learned the fundamentals of Maps and Sets, understanding how they help manage unique and key-value data. Maps allow you to store and access data with unique keys, while Sets ensure that all values remain distinct—like ensuring no two flowers in a garden are the same.
In the next part, we’ll dive into more complex use cases, challenges, and advanced methods for managing collections with Maps and Sets.
Advanced Methods and Use Cases for Maps and Sets
Now that you’ve grasped the basics of Maps and Sets, let’s explore some advanced methods and how these structures can be leveraged in real-world applications. These methods provide more flexibility when working with Maps and Sets, allowing you to perform tasks such as iterating over elements, merging collections, or clearing them.
Advanced Methods for Maps
Maps have several useful methods that make them incredibly powerful for handling data. Here are a few key ones:
forEach(): Iterates through each key-value pair in the Map.
keys(): Returns an iterator for the keys in the Map.
values(): Returns an iterator for the values in the Map.
entries(): Returns an iterator for the key-value pairs.
Code Snippet: Iterating Over a Map
Imagine you want to walk through your garden and print out all the trees and their descriptions:
let treeMap = new Map([
["Oak", "A sturdy tree with strong branches."],
["Maple", "Known for its beautiful fall foliage."],
["Cherry", "Blossoms with delicate flowers."]
]);
treeMap.forEach((description, tree) => {
console.log(`${tree}: ${description}`);
});
Output:
Oak: A sturdy tree with strong branches.
Maple: Known for its beautiful fall foliage.
Cherry: Blossoms with delicate flowers.
In this example, forEach()
allows you to iterate over the entire Map, printing both the key (tree name) and value (description).
Advanced Methods for Sets
Sets also have useful methods that help you manage and manipulate collections of unique values:
forEach(): Iterates through each element in the Set.
keys(): Returns the values as keys (since Sets don’t have key-value pairs,
keys()
andvalues()
behave the same).values(): Returns an iterator for the values in the Set.
clear(): Removes all elements from the Set.
Code Snippet: Iterating Over a Set
Let’s walk through the flower garden and print out the names of all the unique flowers:
let flowerSet = new Set(["Rose", "Tulip", "Daisy", "Lily"]);
flowerSet.forEach(flower => {
console.log(flower);
});
Output:
Rose
Tulip
Daisy
Lily
Just like with Maps, forEach()
can be used to iterate over all the elements in a Set.
Combining Maps and Sets for Powerful Data Management
You can use Maps and Sets together to handle more complex scenarios. For example, imagine you’re managing a gardening competition where each participant grows unique flowers. You can use a Map to associate each participant with their Set of unique flowers.
Code Snippet: Managing Unique Items with Maps and Sets
let competition = new Map();
competition.set("Alice", new Set(["Rose", "Daisy", "Lily"]));
competition.set("Bob", new Set(["Tulip", "Sunflower", "Lily"]));
competition.set("Charlie", new Set(["Daisy", "Orchid"]));
// List all flowers for each participant
competition.forEach((flowers, participant) => {
console.log(`${participant}'s flowers:`);
flowers.forEach(flower => console.log(`- ${flower}`));
});
Output:
Alice's flowers:
- Rose
- Daisy
- Lily
Bob's flowers:
- Tulip
- Sunflower
- Lily
Charlie's flowers:
- Daisy
- Orchid
In this example, each participant’s name is mapped to a Set of flowers they’re growing, ensuring no duplicates. You can iterate over the Map to display each participant’s unique collection.
Challenge Time — Advanced Maps and Sets
Now that you’ve learned about advanced Maps and Sets, it’s time to challenge yourself with more complex use cases.
Challenge 1: Track Unique Visitors
Scenario: Create a system to track visitors to a website. Use a Set to ensure no duplicate visitors are recorded and a Map to track the number of visits for each unique visitor.
Example:
let visitors = new Set();
let visitCount = new Map();
function recordVisit(visitor) {
visitors.add(visitor);
// Update visit count
if (visitCount.has(visitor)) {
visitCount.set(visitor, visitCount.get(visitor) + 1);
} else {
visitCount.set(visitor, 1);
}
}
recordVisit("Alice");
recordVisit("Bob");
recordVisit("Alice");
console.log(visitors); // Output: Set { 'Alice', 'Bob' }
console.log(visitCount); // Output: Map { 'Alice' => 2, 'Bob' => 1 }
Challenge 2: Manage Inventory with Unique Items
Scenario: Imagine you’re managing an inventory of unique items in a game. Use a Set to track unique items collected by players, and a Map to associate each player with their unique collection of items.
Example:
let inventory = new Map();
function addItem(player, item) {
if (!inventory.has(player)) {
inventory.set(player, new Set());
}
inventory.get(player).add(item);
}
addItem("Player1", "Sword");
addItem("Player1", "Shield");
addItem("Player1", "Sword"); // Duplicate, won't be added
addItem("Player2", "Bow");
console.log(inventory);
Challenge 3: Filter and Track Data
Scenario: Create a Map to store survey responses where the key is the respondent’s name and the value is a Set of their selected choices. Ensure each choice is unique.
Example:
let survey = new Map();
function addResponse(name, choice) {
if (!survey.has(name)) {
survey.set(name, new Set());
}
survey.get(name).add(choice);
}
addResponse("Alice", "Option A");
addResponse("Alice", "Option B");
addResponse("Alice", "Option A"); // Duplicate, won't be added
console.log(survey.get("Alice")); // Output: Set { 'Option A', 'Option B' }
Conclusion — Advanced Data Management with Maps and Sets
You’ve now explored the full potential of Maps and Sets in JavaScript. With Maps, you can manage key-value pairs efficiently, while Sets help you ensure uniqueness in your data. Together, they provide powerful tools for managing complex collections and ensuring the integrity of your data—whether it’s tracking unique users, managing inventories, or organizing survey responses.
By mastering these structures, you’ll have the ability to handle advanced data management scenarios with ease.
Get ready for Function Frenzy!
In the next article, we’ll dive into the world of functions in JavaScript, exploring how to create, optimize, and leverage functions in dynamic and scalable ways. Stay tuned for Function Frenzy!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by