Learn to Set Up and Apply Custom Word Count Commands in PowerShell

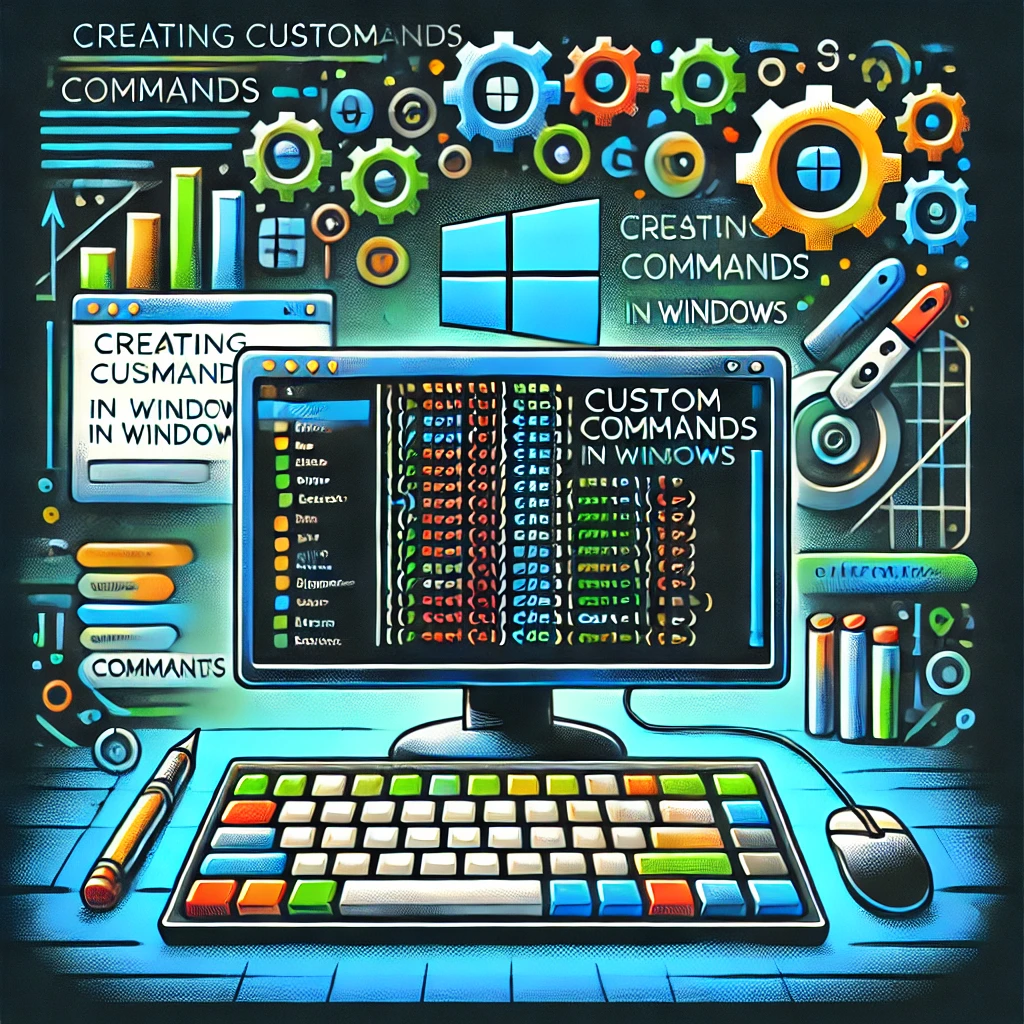
This took me lot to understand and implement custom name to set up unix commands in windows command prompt
instead of using [node index.js] I had to run it as [ccwc]
I got to know about the batch file and its uses filename.bat
Creating custom commands on my terminal is a small yet powerful way to streamline my workflow. Recently, I built a command-line tool called ccwc
(for counting lines, words, and characters in a file) in Node.js, and I wanted to invoke it directly with the ccwc
command in PowerShell and Command Prompt. However, the process wasn’t as simple as I expected. Here’s a quick walkthrough of how I solved it using a .bat
file, turning my script into an easy-to-use command across environments.
Step 1:
Writing the
ccwc
ScriptI first developed my
ccwc
tool in Node.js, which reads a file and outputs line, word, and character counts
#!/usr/bin/env node
console.log("Script started");
const fs = require("fs");
const args = process.argv;
const flags = {
line: args.includes("-l"),
word: args.includes("-w"),
char: args.includes("-c"),
};
let filename = args[args.length - 1];
fs.readFile(filename, "utf-8", (err, file) => {
if (err) {
console.log("There was an error:", err);
return;
}
let linecount = file.split("\n").length;
let wordcount = file.split(/\s+/).filter(word => word).length;
let charcount = file.length;
if (!flags.line && !flags.word && !flags.char) {
console.log(`Lines: ${linecount}, Words: ${wordcount}, Characters: ${charcount}`);
} else {
if (flags.line) console.log(`Lines: ${linecount}`);
if (flags.word) console.log(`Words: ${wordcount}`);
if (flags.char) console.log(`Characters: ${charcount}`);
}
});
After setting up this script, I could run it using Node with node ccwc.js -l test.txt
. But I wanted to run ccwc
directly, without typing the node
command every time.
Step 2: Creating the .bat
File
To make ccwc
run as a command, I created a file called ccwc.bat
in the same directory as my Node.js script. The .bat
file had the following contents:
@echo off
node "%~dp0ccwc.js" %*
Here’s what each part does:
@echo off
prevents the command prompt from displaying each command as it runs.
node "%~dp0ccwc.js" %*
uses node
to execute ccwc.js
, with %*
passing any additional arguments to the script (like -l test.txt
). %~dp0
gives the full directory path to ccwc.js
, ensuring the script runs from its own directory regardless of where I execute the command.
Step 3: Adding the Directory to PATH
For Windows to recognize ccwc
as a command globally, I needed to add the script’s directory to the system’s PATH variable. This way, I could call ccwc
from any location. Here’s how to do it:
Open Environment Variables on Windows.
Under System Variables, find
Path
and edit it.Add the path to the directory containing
ccwc.bat
.
After adding the directory to PATH, I opened a new terminal window and tested the ccwc
command. It worked perfectly! Now, I can simply type ccwc -l test.txt
to get the line count, without specifying node
or the script path.
Benefits of Using a .bat
File
Setting up a .bat
file for custom commands like ccwc
has been a great boost to my productivity. Here are a few reasons why:
Quick Access: I can now invoke
ccwc
directly from any location.Reusable: The
.bat
file setup works well with other custom scripts I might build.Simple Maintenance: I can edit the
ccwc.js
file without changing the.bat
file.
Final Thoughts
Creating custom commands with .bat
files has been a game-changer in my development journey. If you're looking for an efficient way to turn scripts into commands on Windows, give .bat
files a try. Not only does this approach make your tools more accessible, but it also brings you closer to mastering command-line automation!
Subscribe to my newsletter
Read articles from kaverappa c k directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
