Fixing "Blocked by CORS Policy" Errors in Vue.js and Laravel: A Practical Guide

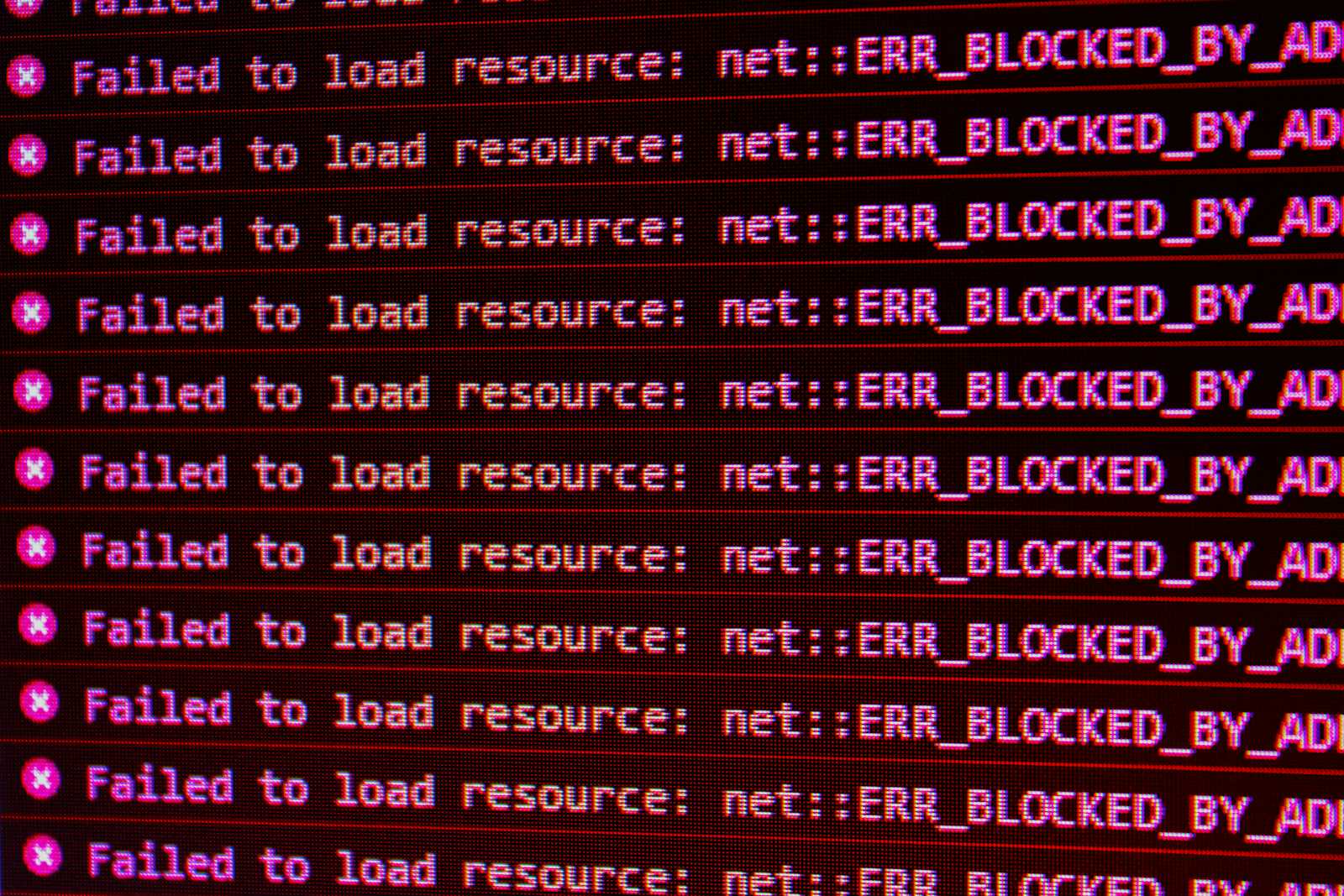
Have you ever run into the dreaded “CORS Policy” error when connecting your Vue.js frontend with a Laravel backend? If you’re seeing messages like:
“The request client is not a secure context and the resource is in a more-private address space…”
…you’re definitely not alone! This error is especially common in development setups where the frontend and backend are running separately and often on different addresses or even protocols (like HTTP vs. HTTPS). Here’s a breakdown of what causes it and, more importantly, how to fix it.
What’s Causing the CORS Error?
In simple terms, the error is about security:
Your request is coming from an unsecure context (like
http://localhost
).The resource you’re trying to access is in a more-private address space, like
localhost
or127.0.0.1
.
Since these “private” resources are supposed to stay secure, browsers will block access to them from any less-secure or public context. It’s their way of keeping things safe — though it can be a pain during development!
Step-by-Step: Setting Up CORS in Laravel
Laravel makes it fairly easy to configure CORS, which controls how your server allows or denies requests from other domains. Here’s how to set it up:
Edit the CORS Config: Open up
config/cors.php
in your Laravel project. This file will let you control which domains, headers, and methods can access your API.Set the Rules:
'paths' => ['api/*', 'sanctum/csrf-cookie'], // Routes to allow CORS for. 'allowed_methods' => ['*'], // All HTTP methods are allowed. 'allowed_origins' => ['*'], // All origins are allowed. Limit to specific domains in production. 'allowed_headers' => ['*'], // Allows all headers. 'supports_credentials' => true, // Set to true if using cookies/authentication.
⚠️ Pro Tip: For security in production, replace the wildcard (
'*'
) with specific domains!
Making Your Frontend Secure with HTTPS
The CORS error often happens when your frontend is running on http://localhost
. Browsers treat this as an unsecure context. Switching to HTTPS can solve this issue.
For Vue.js
You can enable HTTPS locally for Vue by adding a vue.config.js
file in your project root:
module.exports = {
devServer: {
https: true, // Enable HTTPS in development
}
};
When you reload, your app should now be accessible over https://localhost:8080
. If you see SSL certificate warnings, you can create a trusted SSL certificate with tools like mkcert.
Handling Backend-Frontend Communication Over HTTPS
With the frontend on HTTPS, make sure the Vue app connects to a secure backend URL. Here’s a quick setup if you’re using axios
:
import axios from 'axios';
axios.defaults.baseURL = 'https://localhost:8000/api'; // Point to secure backend URL.
Can’t Set Up HTTPS? Try a Reverse Proxy!
If setting up HTTPS is tricky or just not possible, try using a reverse proxy tool like ngrok or localhost.run. They expose your local server over HTTPS with minimal hassle.
Setting Up ngrok
Install ngrok and start it up:
ngrok http 8000
You’ll get a secure
https
URL that you can use to connect to your backend server!
Configuring Laravel for Proxies
If you’re using a reverse proxy, Laravel needs to trust it. Go to App\Http\Middleware\TrustProxies
and set:
protected $proxies = '*'; // Allow all proxies for development.
For Authentication: Consider Laravel Sanctum
If your app needs to handle user authentication, Laravel Sanctum is a great choice. It simplifies secure SPA (Single Page Application) authentication and solves many CORS and CSRF issues by providing a reliable way to manage API tokens and cookies.
Quick Checklist to Beat CORS Errors
Here’s a wrap-up of the steps we covered:
Configure CORS in Laravel: Open up
config/cors.php
and set the paths, methods, and headers.Switch to HTTPS in Vue.js: Enable HTTPS for secure frontend requests.
Use a Reverse Proxy if Needed: Tools like ngrok make it easy to expose your server with HTTPS.
Set Laravel’s Trusted Proxies: Let Laravel know which proxies to trust.
Use Laravel Sanctum for Authentication: Simplifies CORS and CSRF management in SPA setups.
By following these steps, you should be able to troubleshoot and solve CORS issues between Vue.js and Laravel, allowing your apps to communicate smoothly and securely.
Happy coding, and may your API requests go forth unblocked!
Subscribe to my newsletter
Read articles from Shital Mainali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shital Mainali
Shital Mainali
Well, hello there! I'm what you might call an enthusiastically frustrated developer. You see, I have a love-hate relationship with coding. On the one hand, I can't get enough of it - I get all giddy at the thought of creating something new and exciting. On the other hand, it's like trying to solve a Rubik's cube blindfolded while standing on one foot. It's frustrating, it's maddening, and it's just a little bit hilarious. But hey, that's the life of a developer! I'm always up for a challenge, and I'm constantly trying to improve my skills. So, if you need someone to code you a website or an app, just know that I'll be over here banging my head against the keyboard until something magical happens. Cheers to the joys and frustrations of coding!