How to Use CASE Statements in SQL

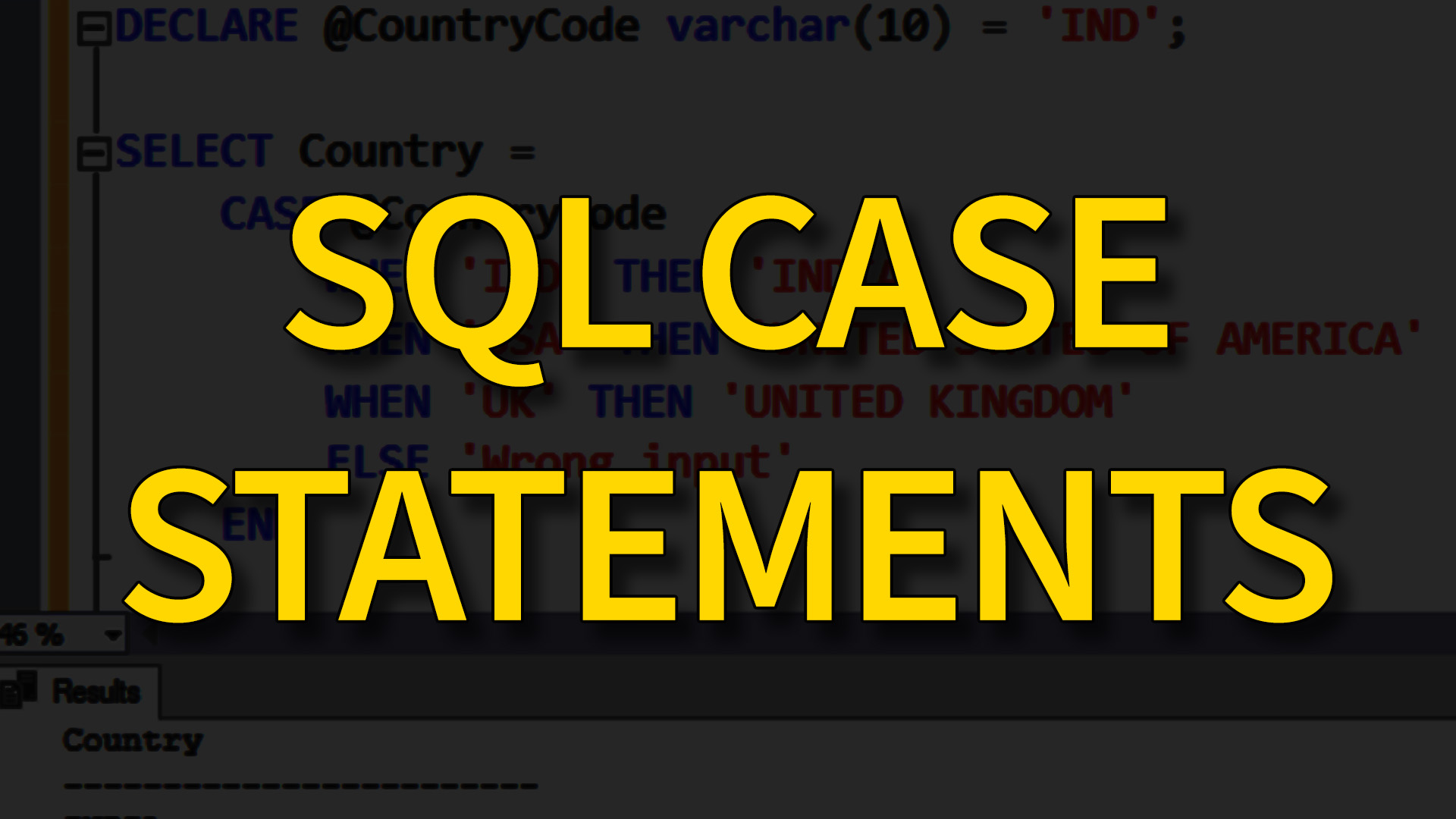
What is the CASE Statement in SQL?
Have you ever needed to show different data based on certain conditions in your SQL queries? Maybe you’re working on a report and want to categorize results based on specific criteria. The SQL CASE statement is your tool for this. It’s like an “if-then” statement in programming, allowing you to check conditions and return values based on those checks.
I recently used CASE statements in a project requiring custom customer data categorization. By using CASE, I could add conditional logic directly in my SQL query, making the report both accurate and efficient. Here’s how the CASE statement works and some examples to get you started.
Why Use the CASE Statement?
The CASE statement can make your queries more powerful by allowing you to:
Categorize Data: Create custom labels based on values.
Simplify Queries: Avoid complex join conditions by embedding logic directly in your SELECT statements.
Optimize Reports: Calculate dynamic values on the fly.
The CASE statement works well with SELECT queries, especially when combined with aggregations or calculations. It can also help you adjust for missing or special data, making your results more meaningful.
Basic Syntax of CASE
The SQL CASE statement has two primary forms: Simple and Searched.
Simple CASE Syntax: This form checks a single column against multiple values.
CASE column_name
WHEN value1 THEN result1
WHEN value2 THEN result2
ELSE default_result
END
Searched CASE Syntax: This form allows you to define conditions that don’t rely on a single column.
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END
You can think of CASE as an inline “if-else” structure, where each condition leads to a specific outcome. Let’s walk through some practical uses of CASE statements to see how they work in real queries.
Using CASE in SELECT Queries
One of the most common uses for CASE is within SELECT queries. For example, imagine you have a table of orders, and you want to label orders over $100 as "High Value" and others as "Standard".
Here’s how to do this:
SELECT
OrderID,
Amount,
CASE
WHEN Amount > 100 THEN 'High Value'
ELSE 'Standard'
END AS OrderCategory
FROM Orders;
Explanation:
This query creates a new column, OrderCategory, based on the Amount.
If Amount is greater than 100, it labels it "High Value." Otherwise, it labels it "Standard."
This kind of categorization is helpful in reports where you want to group or filter by categories without modifying the original data.
Using CASE with Aggregations
The CASE statement becomes even more powerful when combined with aggregate functions like SUM or COUNT. Let’s say you want to find the total revenue from “High Value” and “Standard” orders.
SELECT
SUM(CASE WHEN Amount > 100 THEN Amount ELSE 0 END) AS HighValueTotal,
SUM(CASE WHEN Amount <= 100 THEN Amount ELSE 0 END) AS StandardTotal
FROM Orders;
Explanation:
Here, CASE filters the values being summed based on the Amount.
This query will return separate totals for “High Value” and “Standard” orders.
Using CASE with aggregates allows you to summarize data based on custom criteria, making it easy to get insights from complex datasets.
Nested CASE Statements
Sometimes, you need multiple layers of conditions. In these cases, you can nest CASE statements within each other. Imagine we want to classify orders as “Premium,” “High Value,” or “Standard,” with “Premium” for orders over $500.
SELECT
OrderID,
Amount,
CASE
WHEN Amount > 500 THEN 'Premium'
WHEN Amount > 100 THEN 'High Value'
ELSE 'Standard'
END AS OrderCategory
FROM Orders;
Explanation:
This query checks the Amount in descending order, first testing if it’s above 500, then 100, and labeling each row accordingly.
CASE stops once it finds a true condition, so the first match wins. This structure is useful for creating categories with varying levels.
Nested CASE statements give you control over multiple conditions and help organize data into precise classifications.
Error Handling and Best Practices
To make sure your CASE statements run smoothly:
Use an ELSE clause: This handles unexpected or null values, preventing errors.
Order conditions carefully: Place more specific conditions first.
Test with a subset of data: Try your CASE logic on a smaller dataset to verify results.
These practices make your queries more robust and your results more predictable.
Practical Examples
Let’s walk through a couple more examples to solidify your understanding.
Calculating Discounts
Suppose you want to apply a 10% discount for VIP customers and a 5% discount for others.
SELECT
CustomerID,
PurchaseAmount,
PurchaseAmount *
(CASE WHEN CustomerType = 'VIP' THEN 0.9 ELSE 0.95 END) AS DiscountedAmount
FROM Customers;
Showing Product Availability
In an inventory table, you want to label products based on their stock levels as "In Stock," "Low Stock," or "Out of Stock."
SELECT
ProductID,
Stock,
CASE
WHEN Stock = 0 THEN 'Out of Stock'
WHEN Stock < 5 THEN 'Low Stock'
ELSE 'In Stock'
END AS StockStatus
FROM Inventory;
These examples show how CASE can be used to add meaningful data to your results, making reports more insightful and actionable.
How to Use SQL CASE Statements
The CASE statement in SQL adds flexibility and intelligence to your queries, allowing you to conditionally process data within the database. Whether you’re categorizing data, applying discounts, or managing inventory, CASE statements make it possible to add complex logic to your reports.
Subscribe to my newsletter
Read articles from Steve Hatmaker Jr. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Steve Hatmaker Jr.
Steve Hatmaker Jr.
I’m Steve Hatmaker, Jr., a programmer, coder, and problem solver who thrives on turning complex challenges into elegant solutions. My passion for technology fuels my journey as I navigate the ever-evolving world of programming. With a deep understanding of coding principles and a knack for innovative problem-solving, I’m dedicated to pushing the boundaries of what’s possible in the digital realm. From crafting sleek, functional software to developing intricate algorithms, my focus is on creating technology that not only meets but exceeds expectations. Each project is a new puzzle, and I approach it with the same curiosity and enthusiasm as a musician finding the perfect note or an artist discovering a new medium. While my website primarily highlights my work in programming and coding, my broader creative endeavors enrich my approach, offering a unique perspective on problem-solving. When I’m not immersed in code, I find inspiration in music and art, channels that influence my technical work in subtle yet profound ways. I believe that the creativity and discipline required in these fields complement and enhance my programming skills, allowing me to deliver solutions that are both innovative and practical.