Understanding JavaScript Objects and Classes: A Simple Guide

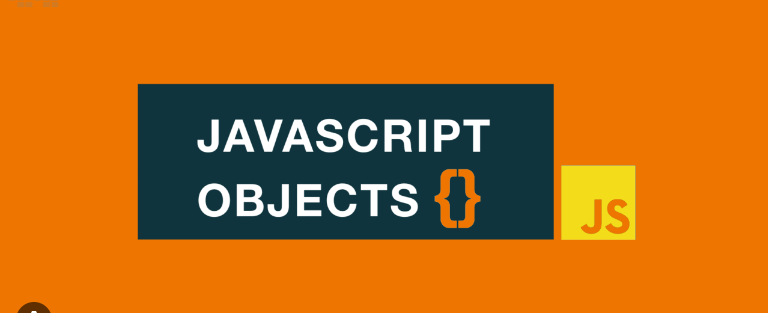
What is an Object?
An object is a container, similar to an array, but slightly different. An array holds only one data type (like a list of numbers), while an object can hold multiple data types, including other objects. Think of an object like a wardrobe where you store various items like clothes, documents, and jewelry. Similarly, an object can hold multiple types of data together in a structured way.
Syntax:
const object_name = {
key: value,
key: value,
key: value
};
Let’s go through each part step by step:
const: This is a keyword that declares a variable that won’t change. We often use const for objects so that we don’t accidentally overwrite the whole object.
object_name: This is the name of the object. You can choose any name that makes sense for what the object represents. For example, if this object is storing information about a person, you might name it
person
.{ } (Curly Braces): The curly braces
{ }
define the boundaries of the object. Everything inside{ }
belongs to the object.key: value: Inside the curly braces, you write pairs of
key: value
:key: This is like a label or name that describes what type of information it’s storing. It’s also called a property name.
value: This is the actual information stored in the key. It could be a number, a string, a boolean, or even another object or function.
Each key-value pair is separated by a comma.
Example: Student Object
const student = {
name: "Vinita", // key: "name", value: "Vinita"
age: 16, // key: "age", value: 16
grade: "9th Grade" // key: "grade", value: "9th Grade"
};
In this example:
name
is a key, and"Vinita"
is its value.age
is a key, and16
is its value.grade
is a key, and"9th Grade"
is its value.
How to Use or Access Object Properties
To access a value inside an object, we use object_name.key
.
console.log(student.name); // Output: "Vinita"
console.log(student.age); // Output: 16
console.log(student.grade); // Output: "9th Grade"
Here, student.name
gives us "Vinita"
because we’re asking for the value of the name
key in the student
object.
Updating Object Properties
You can easily update the value of an existing property in an object. Here's how:
Example: Updating a Property
const student = {
name: "Vinita",
age: 21,
grade: "12th Grade"
};
// Update the age property
student.age = 22;
console.log(student.age); // Output: 22
Deleting Object Properties
You can also remove properties from an object using the delete
keyword.
Example: Deleting a Property
const student = {
name: "Vinita",
age: 21,
grade: "12th Grade"
};
// Delete the grade property
delete student.grade;
console.log(student.grade); // Output: undefined
Adding New Properties
You can also add new properties to an existing object easily.
Example: Adding a Property
const student = {
name: "Vinita",
age: 21
};
// Add a new property
student.grade = "12th Grade";
console.log(student.grade); // Output: "12th Grade"
Where We Use Objects
1. User Data Storage:
We use objects to store user information in a single, organized structure.
const user = {
name: "Vinita",
age: 20,
email: "vinita@example.com"
};
2. Fetching Data from a Database or API:
When we fetch data from a database or API, it often comes as objects.
const product = {
id: 101,
name: "Mobile Phone",
price: 20000,
inStock: true
};
3. Data Organization in Applications:
Objects help manage complex data structures in applications, like user profiles in a social media app.
const profile = {
username: "VinitaG",
followers: 150,
posts: ["Post1", "Post2"]
};
4. Configuring Settings:
Objects are often used to store configuration settings for applications.
const appSettings = {
theme: "dark",
language: "en",
notificationsEnabled: true
};
Why We Use .
(Dot Notation)
Direct Access to Properties: The dot (
.
) helps us quickly reach a property inside the object, almost like opening a drawer to grab something specific.Clear and Simple Syntax: Dot notation makes it easy to read and understand.
Consistency: Dot notation is widely used because it keeps the code clean and consistent, especially when you’re working with many properties.
What is a Class in JavaScript?
A class in JavaScript is an amazing blueprint for creating objects! It sets up a structure that lets us create multiple objects with similar properties and methods without rewriting the same code over and over. Classes make our code super organized and so much easier to maintain!
Why We Use Classes?
When developing applications, we often find ourselves creating multiple objects with the same properties but different values.
For example, if we have different students with similar attributes like name, age, and grade, we would need to create separate objects for each student. This can lead to repetitive code. To address this issue, JavaScript introduced classes in ECMAScript 6 (ES6) in 2015. Classes help us define a common structure for objects, allowing us to create multiple instances easily.
Syntax of a Class
Here’s how we define a class in JavaScript:
class ClassName {
constructor(parameters) {
// Initialize properties
}
// Methods can be defined here
}
What is a Constructor?
A constructor is a unique method within a class in JavaScript that is invoked automatically whenever you create a new instance (or object) from that class. Think of it as a setup function that prepares your object with the necessary properties and initial values right from the moment it is created.
Purpose of a Constructor:
Initialization: The primary role of a constructor is to initialize the properties of the object. This means assigning values to the object's attributes as soon as it is instantiated.
Creating Instances: When you define a constructor, it allows you to create multiple objects that share the same structure but can hold different values. This prevents code repetition, making it easier to manage and maintain your code.
How it Works:
When you define a class and include a constructor, you can pass parameters to it when you create a new object. These parameters are used to set up the initial state of the object. This ensures that each instance can have its own unique data while still adhering to the structure defined by the class.
Here’s a basic example:
class Person {
constructor(name, age) {
this.name = name; // Initializes the name property
this.age = age; // Initializes the age property
}
}
// Creating a new instance of Person
const person1 = new Person("Alice", 30);
const person2 = new Person("Bob", 25);
console.log(person1.name); // Output: Alice
console.log(person2.age); // Output: 25
In this example, the Person
class has a constructor that takes two parameters: name
and age
. When we create new instances (person1
and person2
), these parameters are used to initialize the properties of each object, allowing each person to have different values for name
and age
.
Example of a Class
class Student {
constructor(name, email, age, country, city, pin_code) {
this.name = name;
this.email = email;
this.address = {
country: country,
city: city,
pin_code: pin_code
};
}
greet() {
console.log("Hello " + this.name);
}
getFullAddress() {
console.log(this.address.country + ", " + this.address.city + " - " + this.address.pin_code);
}
}
// Creating instances of the Student class
const forME = new Student("Vinita", "VINI@gmail.com", 21, "India", "Raipur", 492001);
const forFrnd = new Student("ABC", "Abc@gmail.com", 22, "India", "Jaipur", 456985);
const forAnother = new Student("Kabir", "kabir@gmail.com", 23, "USA", "Nusib", 492008);
// Calling methods on the instances
forME.greet(); // Output: Hello Vinita
forME.getFullAddress(); // Output: India, Raipur - 492001
forFrnd.greet(); // Output: Hello ABC
forFrnd.getFullAddress(); // Output: India, Jaipur - 456985
forAnother.greet(); // Output: Hello Kabir
forAnother.getFullAddress(); // Output: USA, Nusib - 492008
Explanation of the Code
Class Definition:
class Student
defines a new class namedStudent
.Constructor: Initializes the object properties. It takes parameters for the student's name, email, age, and address.
Methods:
greet()
: Logs a greeting message using the student's name.getFullAddress()
: Logs the full address.
Creating Instances: Three instances of the Student class are created.
Calling Methods: The methods are called on each instance, producing output in the console.
Why do we use the keyword this
in the example above?
Understanding this
this
is a special keyword in JavaScript that refers to the current object executing the code. It allows us to access the properties and methods of that object.
Reasons for Using this
Referring to the Current Instance: Helps to refer to that specific object.
Distinguishing Between Parameters and Object Properties: Helps differentiate between them when they have the same name.
How this
Functions in the Example
Setting Properties:
this.name
= name;
sets the object's name property to the value passed to the constructor.Accessing Properties: In the
greet()
method, usingthis.name
accesses the name property of the current object.
Conclusion
In summary, objects and classes are fundamental building blocks in JavaScript:
Objects are versatile containers that hold multiple data types and allow easy access, modification, and organization of related data.
Classes serve as blueprints for creating objects, enabling the creation of multiple instances with shared properties and methods, while constructors initialize these objects.
The
this
keyword refers to the current object, making it easy to access its properties.
By mastering these concepts, you can build organized and efficient applications in JavaScript.
Subscribe to my newsletter
Read articles from Vinita Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinita Gupta
Vinita Gupta
Full-stack development student at Navgurukul, blending creativity with technical skills. Experienced in HTML, CSS, and JavaScript. Selected for advanced training by HVA, I have strong leadership abilities and a passion for continuous learning. Aspiring to excel in DSA and become a proficient full-stack developer.