10 Common Flutter Mistakes and How to Avoid Them
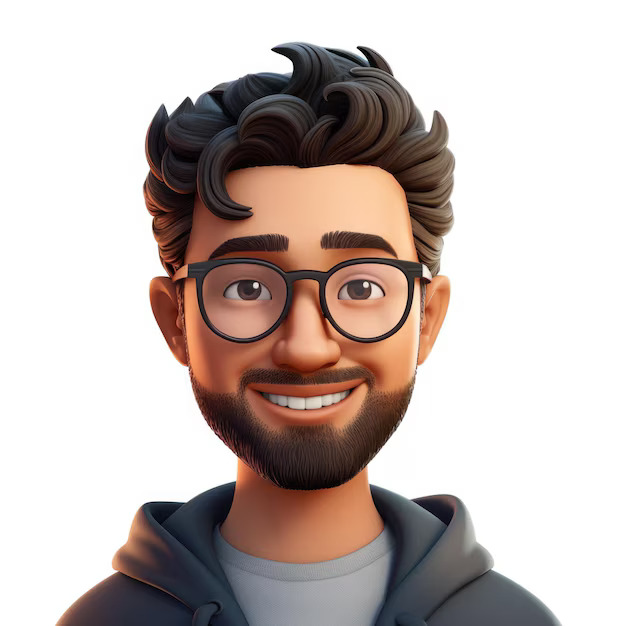
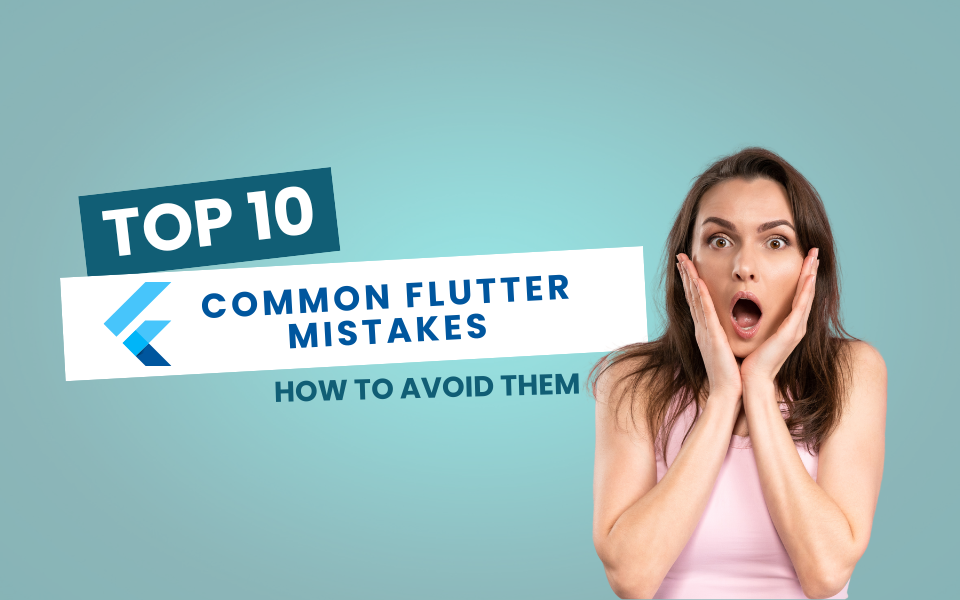
Because of its versatility, user-friendliness & capacity to generate natively compiled desktop, web & mobile applications from a single codebase, Flutter is one of the cross-platform app development frameworks with the highest rate of growth. But like any powerful tool, developers—whether seasoned pros or enthusiastic learners—can make mistakes that negatively impact user experience, maintainability & performance. To make sure your Flutter app shines, it's essential to comprehend these typical errors and know how to avoid them.
Let's examine the top ten Flutter errors that developers frequently make and how to prevent them.
1. Ignoring Widget Reusability
The ability to reuse widgets throughout an application is one of Flutter's primary advantages. Widgets are the foundation of the framework. However, by failing to reuse widgets, developers can occasionally make the mistake of writing repeated code. In addition to raising the possibility of inconsistency, this bloats the codebase and makes maintenance more difficult.
Solution - Identify reusable components early. For example, instead of creating a separate Text widget for every label, consider extracting it into a custom widget with configurable properties like text style & alignment. This will streamline your development process & improve code maintainability.
2. Misusing Stateful & Stateless Widgets
A common misstep for Flutter beginners is using StatefulWidget
when a StatelessWidget
would suffice & vice versa. StatefulWidget
manages state, which makes it heavier in terms of memory & performance. Using it unnecessarily can slow down your app.
Solution - Only use StatefulWidget
when the widget's state needs to change. For example, if a widget is merely presenting information & not responding to any dynamic changes (like static text or an image), go for StatelessWidget
. This small optimization can make a big difference in app performance.
3. Overcomplicating State Management
State management can feel overwhelming, especially with so many options like Provider, Riverpod, Bloc, Redux, etc. Some developers over complicate their apps by choosing a heavy handed solution when simpler alternatives would do the job.
Solution - Choose the right state management technique based on the app’s complexity. For simple apps, setState
or Provider might be sufficient. For more complex apps, consider using more structured approaches like Bloc or Riverpod. Always ask yourself - Does this app need complex state management or am I over engineering the solution?
4. Neglecting Performance Optimizations
In the rush to build features, many developers overlook optimizing their app’s performance. A sluggish app, however, can ruin the user experience & drive users away. Common issues include heavy widget trees, unnecessary rebuilds & inefficient animations.
Solution - Use tools like the Flutter DevTools to analyze performance. Optimize widget trees by breaking them down into smaller components where possible. Avoid unnecessary rebuilds by using widgets like const to make widgets immutable. Additionally, if you are working with complex animations, consider using the AnimatedBuilder
to control rendering.
5. Using Too Many ThirdParty Packages
Flutter’s rich ecosystem of third party packages makes it tempting to lean on external solutions for everything. However, using too many packages can introduce unnecessary dependencies & compatibility issues & make it harder to keep your app updated.
Solution - Be selective with your packages. Choose well maintained & highly rated packages & always evaluate whether you really need them. Sometimes building a custom solution can save you from future headaches.
6. Not Testing Thoroughly
Skipping tests or underestimating their importance is a major pitfall. Some developers only test manually, which is not only time consuming but also prone to missing edge cases. Automated testing unit, widget & integration tests can save time & catch bugs early.
Solution - Integrate testing into your development process from day one. Flutter provides excellent testing support, so there’s no excuse not to use it. Write unit tests for logic, widget tests for UI & integration tests to simulate user interaction.
7. Overloading the Build Method
In Flutter, the build
method is called frequently, so it’s essential to keep it as lightweight as possible. Some developers mistakenly overload this method with logic, making the app slow & unresponsive.
Solution - Keep the build
method clean. Extract heavy logic into separate functions or, better yet, utilize initState
for any initialization. Think of the build
method as a blueprint keep it simple & quick to render.
8. Overlooking PlatformSpecific Adaptations
A big draw of Flutter is its ability to run across platforms. However, a common mistake is forgetting to make platform specific adjustments, resulting in apps that don’t feel native on either Android or iOS.
Solution - Use Theme.of(context)
& MediaQuery.of(context)
to tailor your app’s appearance & behavior based on the platform. For example, iOS apps typically use Cupertino widgets for a native feel, while Android leans on Material Design.
9. Not Using Null Safety Effectively
Since Flutter 2.0, null safety has been an integral part of the framework, preventing null related bugs. However, many developers don’t fully leverage this feature, leading to null errors that could have been avoided.
Solution - Embrace null safety from the start. When defining variables, use non nullable types wherever possible & utilize them ? & ! operators wisely. Null safety isn’t just a feature it’s a safeguard against one of the most common programming errors. A comprehensive Flutter Online Course can help you master null safety and write robust, error-free code by fully exploring null-aware types and operators.
10. Failing to Prioritize the User Experience
It's easy to concentrate too much on functionality and overlook the user experience (UX), even while Flutter makes it possible to create useful apps fast. No matter how feature-rich your software is, a clumsy or unclear user interface might drive people away.
Solution - Always consider the viewpoint of the user. Create logical navigation, tidy layouts & fluid animations with Flutter's robust widget system. Keep in mind that creating an app that people enjoy using is more important than simply creating an app.
Conclusion
You can make sure that your Flutter app is not only functional but also effective, scalable & user-friendly by steering clear of these typical blunders. Whether you are a professional working on your next major project or a student just starting out, paying attention to these things can help you avoid future problems and make apps that stand out in a crowded market. Continue to grow and learn & never forget that little progress made now will result in major achievements later. Have fun with your coding!
You can also read: Mastering Flutter
Subscribe to my newsletter
Read articles from Steve Smith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
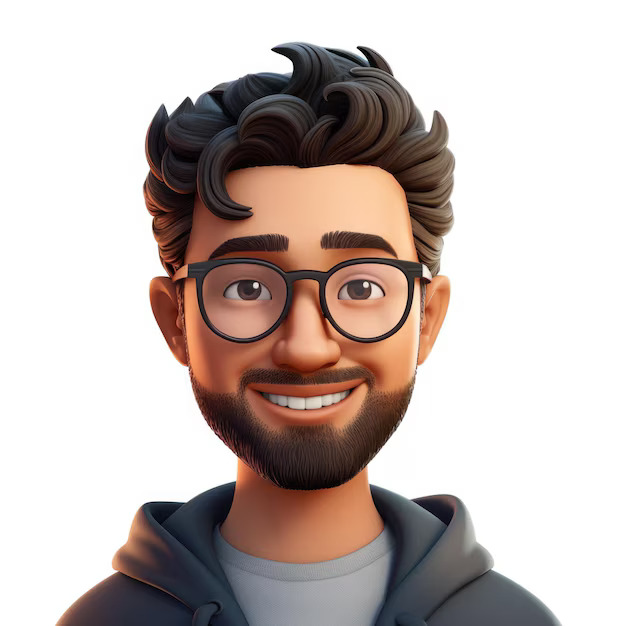
Steve Smith
Steve Smith
I am a seasoned DevOps Designer with over a decade of experience in tech industry. I have extensive experience in cloud infrastructure management, system administration, and software development. My journey into the universe of DevOps started during my initial days as a product engineer, where I immediately understood the significance of joint effort among improvement and tasks groups to accomplish proficient and solid programming conveyance.