Mastering useState: A Beginner's Guide to State Management in React

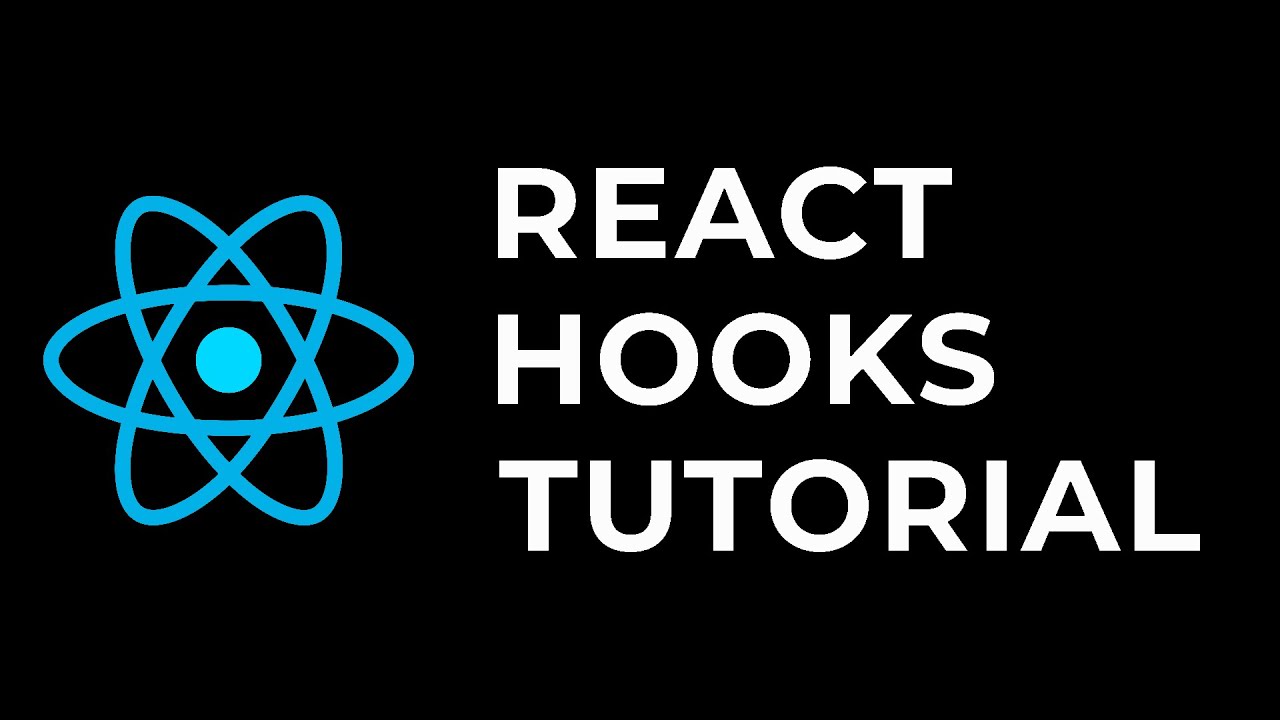
If you're starting out with React, useState
is one of the first hooks you'll encounter. It’s a fundamental part of how React components manage state — that is, how they store and update information that can change over time. In this blog post, we'll explore how useState
works, why it's so important, and how to create a simple counter application to solidify the concept.
What is useState
?
The useState
hook is a built-in function in React that lets you add state to a functional component. Traditionally, state was only available in class components, but with React Hooks, you can now manage state in functional components, making them much more powerful and flexible.
How Does useState
Work?
When you call useState
, it returns an array with two elements:
The current state value
A function to update that state
Syntax:
const [state, setState] = useState(initialState);
state
: The current state value.setState
: A function that lets you updatestate
.initialState
: The initial value of the state.
Each time you call setState
, React re-renders the component with the new state value. This allows you to build dynamic, interactive UIs.
Why Use useState
?
State is essential in many React applications, as it enables components to keep track of information that can change over time — like user input, form data, or counters. useState
allows you to:
Update the UI based on user interactions.
Maintain data that persists across component renders.
Trigger re-renders when data changes.
A Simple Counter Example Using useState
Let’s dive into an example to see how useState
works in action. We’ll create a simple counter that increases or decreases each time a button is clicked.
Here’s the complete code:
import React, { useState } from 'react';
function Counter() {
// Step 1: Declare a state variable called 'count' and initialize it to 0
const [count, setCount] = useState(0);
// Step 2: Define functions to increase and decrease the count
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div>
<h1>Counter</h1>
<p>Current Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
Code Breakdown
Declaring State with useState
:
const [count, setCount] = useState(0);
Here,
count
is our state variable, initialized to 0.setCount
is the function we’ll use to update the value ofcount
.
Updating the State:
The
increment
function adds 1 tocount
each time it’s called.The
decrement
function subtracts 1 fromcount
each time it’s called.
These functions use setCount
to update count
and trigger a re-render with the new state.
Event Handling:
- We use the
onClick
event on each button to callincrement
anddecrement
when the buttons are clicked.
- We use the
How It Works
Each time you click Increment, count
goes up by 1, and Decrement reduces it by 1. When you call setCount
inside these functions, React re-renders the component, and the new count is displayed on the screen.
Understanding Re-renders in useState
Each time you call setCount
, React re-renders the Counter
component with the updated state. This re-rendering mechanism ensures that the UI always reflects the current state.
Handling Complex Updates with useState
Sometimes, you may need to use the previous state to calculate the new state. In these cases, useState
provides a callback form of setState
. Here’s how you can modify the increment
function to use the previous state:
const increment = () => setCount(prevCount => prevCount + 1);
Using this callback form is particularly helpful in cases where multiple updates happen quickly or asynchronously, as it ensures each update correctly calculates the new state based on the latest available state.
Common Pitfalls and Best Practices
Directly Modifying State: Never modify the state directly. For example, avoid doing
count++
. Always usesetState
.State Initialization: If your initial state depends on a calculation, use a function to initialize it:
const [count, setCount] = useState(() => calculateInitialValue());
- This ensures that the calculation only runs once, on the initial render.
Conclusion
The useState
hook is a powerful tool in React, allowing you to manage dynamic data directly within functional components. By practicing with examples like this counter, you'll gain confidence in handling state in your React projects. Happy coding!
Subscribe to my newsletter
Read articles from Abhishek Raut directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Raut
Abhishek Raut
🔭 I’m currently working on Full Stack Project 👯 I’m looking to collaborate on Animated Websites 🌱 I’m currently learning Data Science 💬 Ask me about React GSAP ⚡ Fun fact I am Working on Project and learning tech stack used in That