Node.js Cheat Sheet
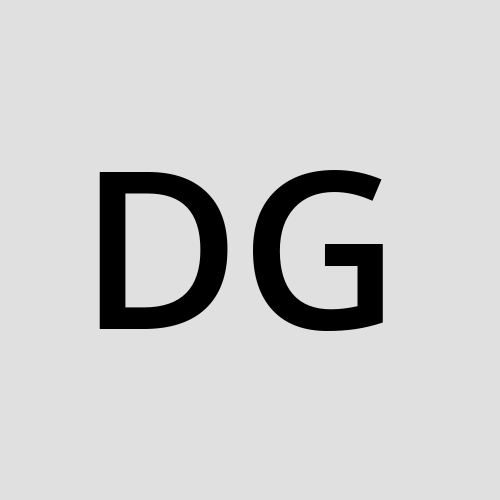
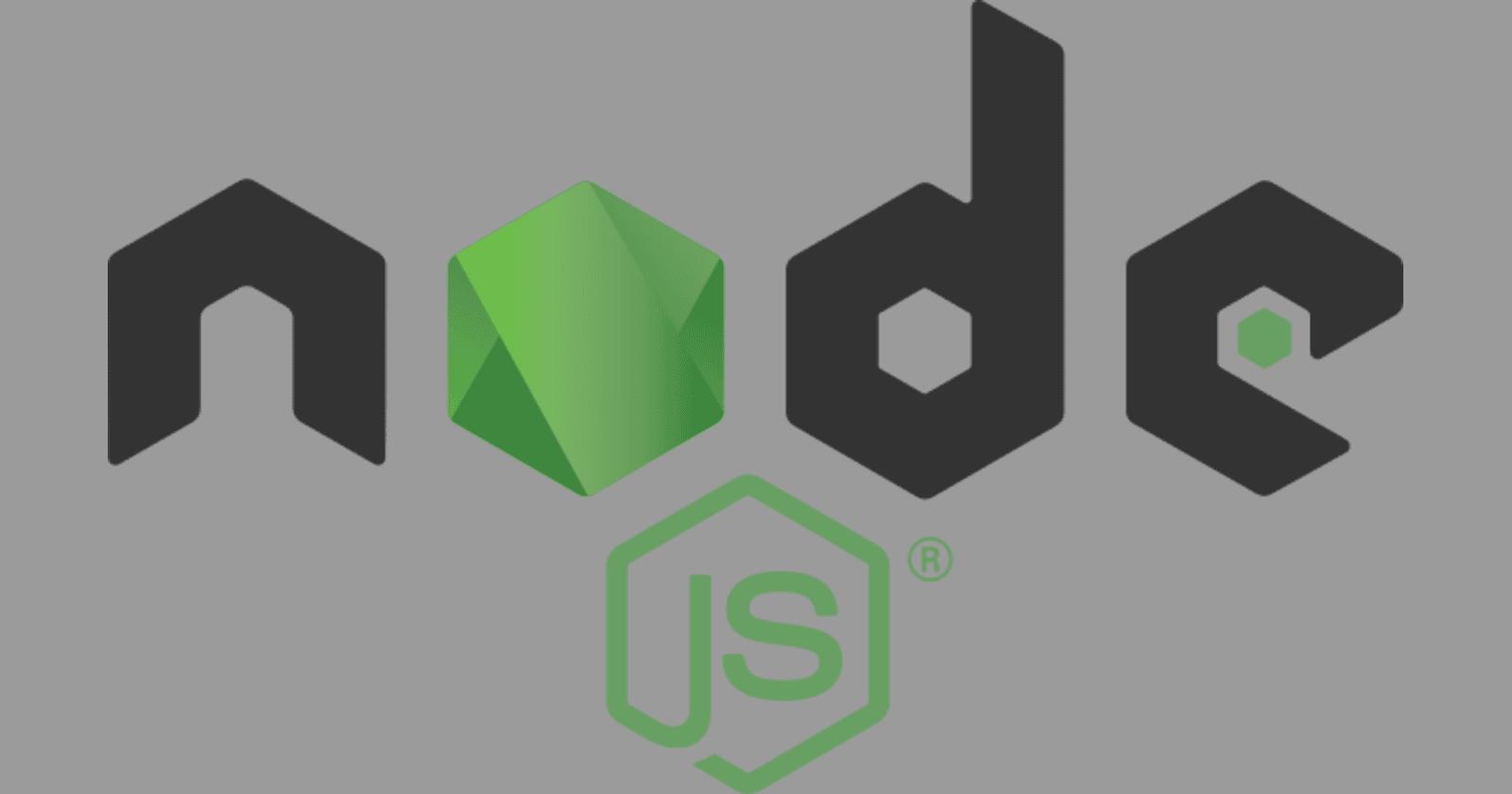
Here’s a Node.js cheat sheet with common commands and snippets to help you with various tasks:
Basic Setup
Initialize a New Project:
npm init -y
Install a Package:
npm install package_name
Install a Package Globally:
npm install -g package_name
Uninstall a Package:
npm uninstall package_name
Run a Script:
npm run script_name
Basic Server with HTTP Module
Simple HTTP Server:
const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, world!\n'); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
Express Framework
Install Express:
npm install express
Basic Express Server:
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, world!'); }); app.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
Parse JSON Request Body:
app.use(express.json());
Route Parameters:
app.get('/user/:id', (req, res) => { res.send(`User ID: ${req.params.id}`); });
Middleware Example:
const logger = (req, res, next) => { console.log(`${req.method} ${req.url}`); next(); }; app.use(logger);
File System (fs) Module
Read a File:
const fs = require('fs'); fs.readFile('file.txt', 'utf8', (err, data) => { if (err) throw err; console.log(data); });
Write to a File:
fs.writeFile('file.txt', 'Hello, world!', (err) => { if (err) throw err; console.log('File has been saved!'); });
Append to a File:
fs.appendFile('file.txt', 'More data\n', (err) => { if (err) throw err; console.log('Data was appended to file!'); });
Environment Variables
Using Environment Variables (.env):
Install dotenv:
npm install dotenv
Load Environment Variables:
require('dotenv').config(); const port = process.env.PORT || 3000;
.env
file example:PORT=3000
Asynchronous Patterns
Promises:
const asyncFunction = () => { return new Promise((resolve, reject) => { setTimeout(() => resolve('Done!'), 1000); }); }; asyncFunction().then(result => console.log(result)).catch(error => console.error(error));
Async/Await:
const asyncFunction = async () => { try { const result = await asyncTask(); console.log(result); } catch (error) { console.error(error); } }; asyncFunction();
Working with APIs (HTTP Requests)
Using Axios:
Install Axios:
npm install axios
Make a GET Request:
const axios = require('axios'); axios.get('https://api.example.com/data') .then(response => console.log(response.data)) .catch(error => console.error(error));
Using Fetch (in Node 18+):
const fetch = require('node-fetch'); fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
Modules
Exporting a Module:
// in math.js const add = (a, b) => a + b; module.exports = { add };
Importing a Module:
// in main.js const { add } = require('./math'); console.log(add(2, 3));
Error Handling
Basic Error Handling:
try { const result = riskyOperation(); console.log(result); } catch (error) { console.error('Error:', error.message); }
Handle Promise Rejections:
process.on('unhandledRejection', (reason, promise) => { console.error('Unhandled Rejection:', reason); });
Debugging
Run with Debugger:
node --inspect-brk app.js
Using console.log() for Debugging:
const variable = 'test'; console.log({ variable });
Package Management
List Installed Packages:
npm list
View Package Version:
npm view package_name version
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
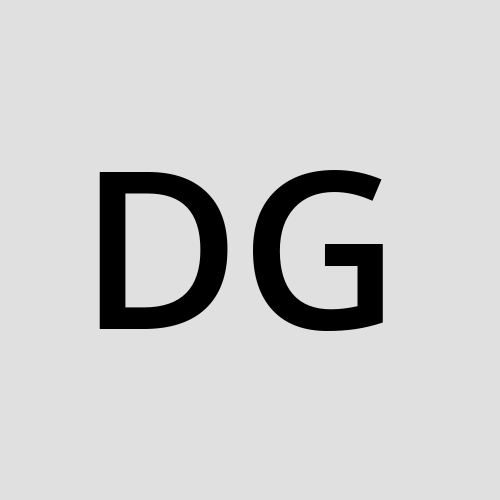
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.