PHP Cheat Sheet
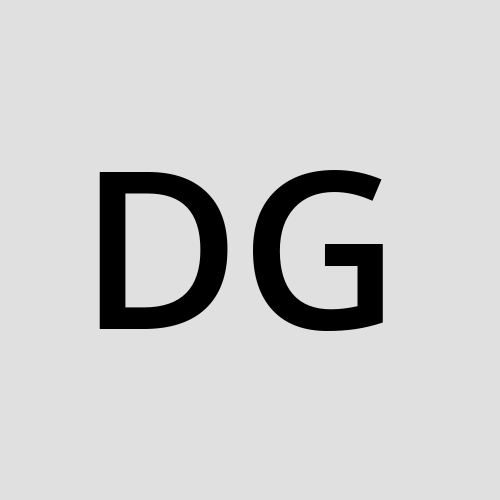
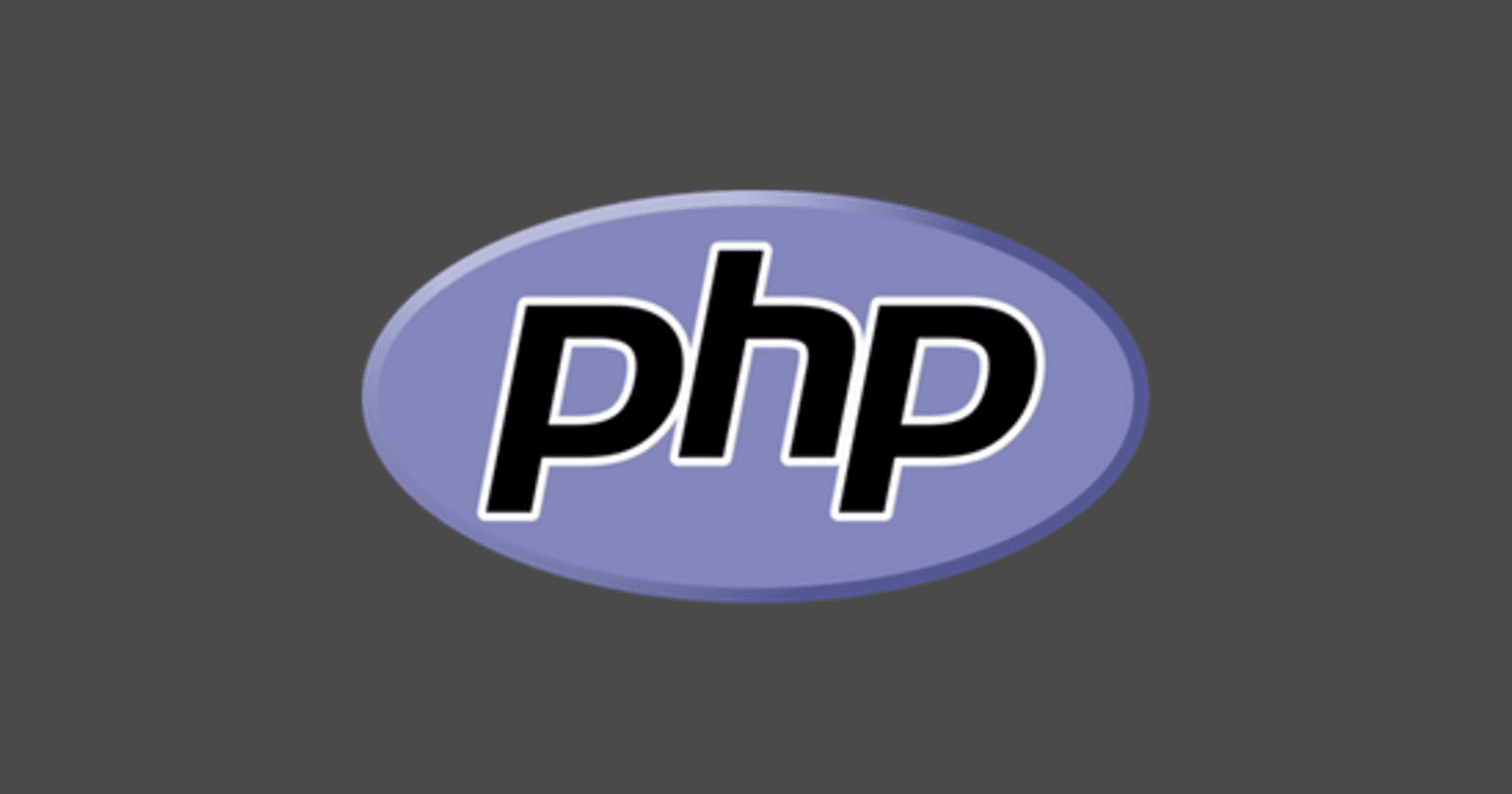
Here's a PHP cheat sheet covering the essentials:
Basic Syntax
Echo / Print:
echo "Hello, World!"; print "Hello, World!";
Variables:
$variable = "Hello!";
Comments:
// Single-line comment # Single-line comment /* Multi-line comment */
Data Types
String:
$string = "Hello, World!";
Integer:
$integer = 42;
Float:
$float = 3.14;
Boolean:
$bool = true;
Array:
$array = ["apple", "banana", "cherry"];
Associative Array:
$assocArray = ["name" => "John", "age" => 30];
Strings
String Concatenation:
$greeting = "Hello, " . "World!";
String Interpolation:
$name = "John"; echo "Hello, $name!";
String Length:
echo strlen("Hello");
Arrays
Indexed Array:
$colors = ["red", "green", "blue"];
Associative Array:
$person = ["name" => "Alice", "age" => 25];
Multidimensional Array:
$matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ];
Array Functions:
array_push($colors, "yellow"); // Add element array_pop($colors); // Remove last element count($colors); // Count elements
Control Structures
If-Else:
if ($a > $b) { echo "a is greater than b"; } elseif ($a == $b) { echo "a is equal to b"; } else { echo "a is less than b"; }
Switch:
$color = "red"; switch ($color) { case "red": echo "Color is red"; break; case "blue": echo "Color is blue"; break; default: echo "Unknown color"; }
For Loop:
for ($i = 0; $i < 10; $i++) { echo $i; }
Foreach Loop:
foreach ($array as $value) { echo $value; }
While Loop:
while ($i < 10) { echo $i; $i++; }
Do-While Loop:
do { echo $i; $i++; } while ($i < 10);
Functions
Define a Function:
function greet($name) { return "Hello, $name!"; }
Function with Default Parameter:
function greet($name = "World") { return "Hello, $name!"; }
Anonymous Function:
$greet = function($name) { return "Hello, $name!"; };
Superglobals
$_GET
and$_POST
:// Using GET $name = $_GET['name']; // Using POST $email = $_POST['email'];
$_SESSION
and$_COOKIE
:// Start Session session_start(); $_SESSION['username'] = 'JohnDoe'; // Set Cookie setcookie("user", "JohnDoe", time() + (86400 * 30), "/");
$_SERVER
:echo $_SERVER['PHP_SELF']; // Current file name echo $_SERVER['SERVER_NAME']; // Server name
File Handling
Open a File:
$file = fopen("file.txt", "r");
Read a File:
$content = fread($file, filesize("file.txt"));
Write to a File:
$file = fopen("file.txt", "w"); fwrite($file, "Hello, World!"); fclose($file);
Database (MySQLi)
Connect to Database:
$conn = new mysqli("localhost", "username", "password", "database");
Check Connection:
if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); }
Select Data:
$result = $conn->query("SELECT * FROM table"); while($row = $result->fetch_assoc()) { echo $row['column_name']; }
Insert Data:
$sql = "INSERT INTO table (column1, column2) VALUES ('value1', 'value2')"; $conn->query($sql);
Close Connection:
$conn->close();
Error Handling
Try-Catch Block:
try { // Code that may throw an exception throw new Exception("An error occurred"); } catch (Exception $e) { echo "Error: " . $e->getMessage(); }
Date and Time
Current Date and Time:
echo date("Y-m-d H:i:s");
Format Date:
$date = date("l, d-M-Y");
Get Timestamp:
echo time();
Object-Oriented Programming (OOP)
Define a Class:
class Car { public $color; function __construct($color) { $this->color = $color; } function getColor() { return $this->color; } } $myCar = new Car("red"); echo $myCar->getColor();
Inheritance:
class Vehicle { protected $brand; public function __construct($brand) { $this->brand = $brand; } } class Car extends Vehicle { public function getBrand() { return $this->brand; } }
Miscellaneous
Define a Constant:
define("SITE_NAME", "My Website"); echo SITE_NAME;
PHP Info:
phpinfo();
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
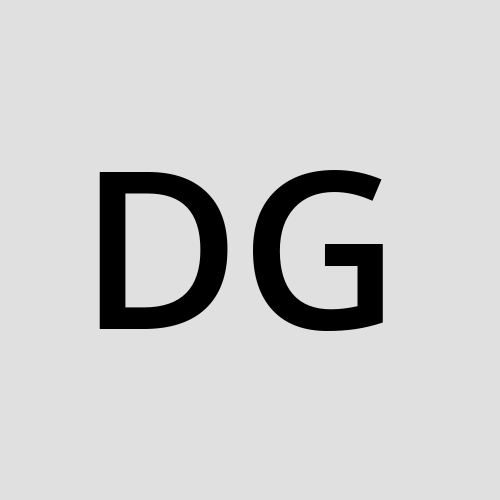
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.