Python basics : Variables and Datatypes
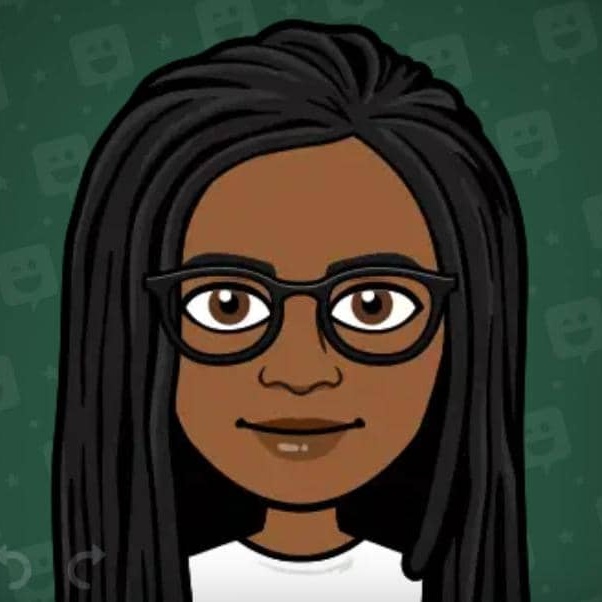
Table of contents
- Welcome Back to "Explaining Python Like You’re 5"!
- 1. Statements: Python Instructions
- 2. Comments: Python's Sticky Notes
- 3. Variables: Python’s Storage Boxes
- 4. Data Types: The Kinds of Data You Can Use
- Combining Text and Variables: Introducing String Concatenation
- Combining Text and Variables: Introducing String Concatenation
- Putting It All Together: A Mini Challenge
- Wrapping Up

Welcome Back to "Explaining Python Like You’re 5"!
This is the second lesson in our Explaining Python series! If you’ve made it here, congratulations! You’re on your way to becoming a Python pro. If this is your first time joining, no worries—you haven’t missed much. Check out the first article here to catch up.
In the last lesson, you wrote your very first line of Python code, which is a pretty big deal. (Did you get those beginner butterflies? I hope so!) Today, we're going to build on that excitement by introducing some key concepts that will set the foundation for your Python journey. We’ll cover:
Statements
Comments
Variables
Data Types
Let's begin!
1. Statements: Python Instructions
In Python, a statement is simply an instruction that Python can read and act on. Think of it as telling Python what to do, one step at a time. If you tell Python, "Hey, print my name," Python will follow the instruction and display your name on the screen.
Example of a Simple Statement:
print("Hello, Python!")
Here, print("Hello, Python!")
is a statement. It tells Python, "Show the words 'Hello, Python!' on the screen." Pretty straightforward, right?
In a nutshell:
Anything you write in Python that gives an instruction is a statement.
Statements can be short, like
print("Hello!")
, or longer and more complex as you go further.
2. Comments: Python's Sticky Notes
Comments are like little sticky notes in your code. They help you (and others) understand what’s going on. Python ignores comments when running the code, so they’re just there for humans to read.
You create a comment by starting a line with the #
symbol:
# This is a comment
print("Hello, World!") # This line prints a greeting
Why Use Comments?
Comments explain what your code does, which is helpful when you (or someone else) read it later.
They’re also great for “turning off” lines of code temporarily while testing.
Think of comments as “whispers” in your code—they’re invisible to Python but helpful for you.
3. Variables: Python’s Storage Boxes
In programming, variables are like labeled boxes where you can store information. A variable gives a name to a piece of data, making it easy to refer to that data later on.
For example:
name = "Ada" # Stores "Alex" in a variable called name
age = 25 # Stores 25 in a variable called age
Now, if you use name
or age
in your code, Python will know to substitute them with "Ada"
and 25
respectively.
Key Things to Know About Variables:
Variable names should be descriptive and meaningful (e.g.,
height
,price
,).Variables are assigned values using the equality
=
symbol.Variable names cannot contain spaces, use underscore instead. (eg
first_name = Steve
)They make your code easier to read and organize.
Fun Twist: Think of variables as tags or labels, just like the ones you use to organize your files. Each one is a shortcut to the data it contains.
4. Data Types: The Kinds of Data You Can Use
Data in Python comes in different types, each suited for different tasks. Here are some of the most common ones:
String (str): A string is a sequence of characters enclosed in a single or double quotation mark, like
"Hello, world!"
or"Python is fun!"
- Example:
greeting = "Hello!"
- Example:
Integer (int): An Integer is a data type used to represent whole integer values. it can be used to store positive or negative integers, like
10
,0
,-5
- Example:
age = 25
- Example:
Float: A float is a data type used to represent decimal values or floating point. It can be used for negative or positive decimals, like
3.14
,9.99
,-0.5
- Example:
price = 19.99
- Example:
Boolean (bool): The python boolean data type is one of the built in data types. it is used to represent the truth value of an expression. The value can only be
true
orfalse
- Example:
is_student = True
- Example:
Each data type serves a purpose. For example, you’d use a string for text, integers for counting, floats for measurements, and booleans for yes/no decisions.
Combining Text and Variables: Introducing String Concatenation
In Python, data types (like strings, numbers, and booleans) each have their own unique roles. When you try to combine them, Python wants to be sure that everything fits together smoothly. For instance, if you’re combining text (a string) with a number (like an age), Python needs a little extra help to understand that they should display together as one message.
This is why we need methods like str()
or f-strings: they help convert all the parts into strings, allowing Python to display them together in a sentence.
You’re right—showing the output helps clarify! Here’s the expanded section with example outputs included:
Combining Text and Variables: Introducing String Concatenation
In Python, data types (like strings, numbers, and booleans) each serve different purposes, and sometimes they need a little help to work together. For example, if you try to combine text (a string) with a number (like an age), Python needs you to explicitly convert the number into a string. Otherwise, it won’t know how to mix them in the same sentence!
This is why we need methods like str()
or f-strings: they make sure everything in the sentence is treated as text, allowing Python to display it all smoothly.
Method 1: Using +
to Join Strings and Variables
The +
operator lets you combine text (strings) and variables by converting any non-string parts with str()
.
Example:
name = "Alex"
age = 21
print("Hello, my name is " + name + " and I am " + str(age) + " years old.")
Output:
Hello, my name is Alex and I am 21 years old.
In this example:
name
is already a string, so we can directly combine it with other text.age
is an integer, so we convert it to a string withstr(age)
to avoid errors when combining.
Method 2: Using f-strings
(Formatted Strings)
With f-strings, you can place variables directly in curly braces {}
within a string. Just add f
before the quotes, and Python takes care of the rest.
Example:
print(f"Hello, my name is {name} and I am {age} years old.")
Output:
Hello, my name is Alex and I am 21 years old.
Putting It All Together: A Mini Challenge
Challenge: Let's create a short description about yourself using variables.
Define variables for your name, age, and a hobby:
name = "Your name" age = 21 #your age hobby = "your hobby"
Use these variables to print a sentence about yourself:
print("Hello, my name is " + name + ". I am " + str(age) + " years old and I enjoy " + hobby + ".")
Bonus: Try changing the variables and see how the sentence changes! Replace your age or hobby and re-run the code to see the new message.
Wrapping Up
In this lesson, we explored statements, comments, variables, and data types. These are the essentials for working with data in Python and help lay the groundwork for more exciting code!
In the next lesson, we’ll talk about more ways of presenting data: lists, tuples, dictionaries and various methods and use cases. Keep experimenting and remember—every bit of code you write brings you closer to being a Python pro!
Subscribe to my newsletter
Read articles from Munachi Elekwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
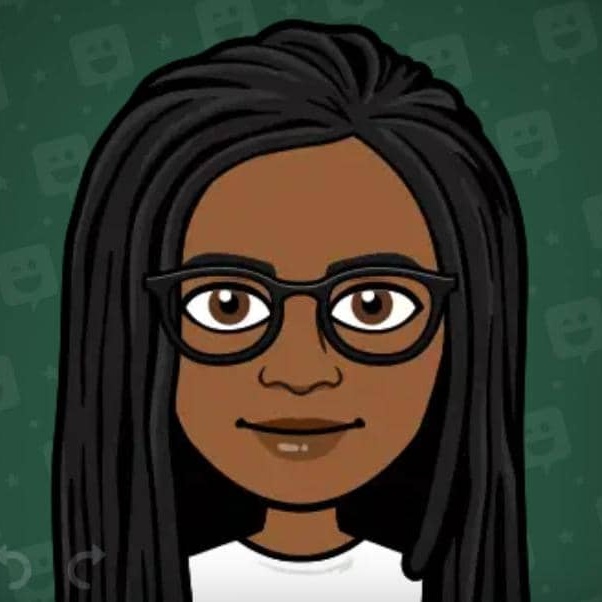
Munachi Elekwa
Munachi Elekwa
I'm Software developer with a sweet tooth.