Understanding Laravel Queues: A Beginner's Guide

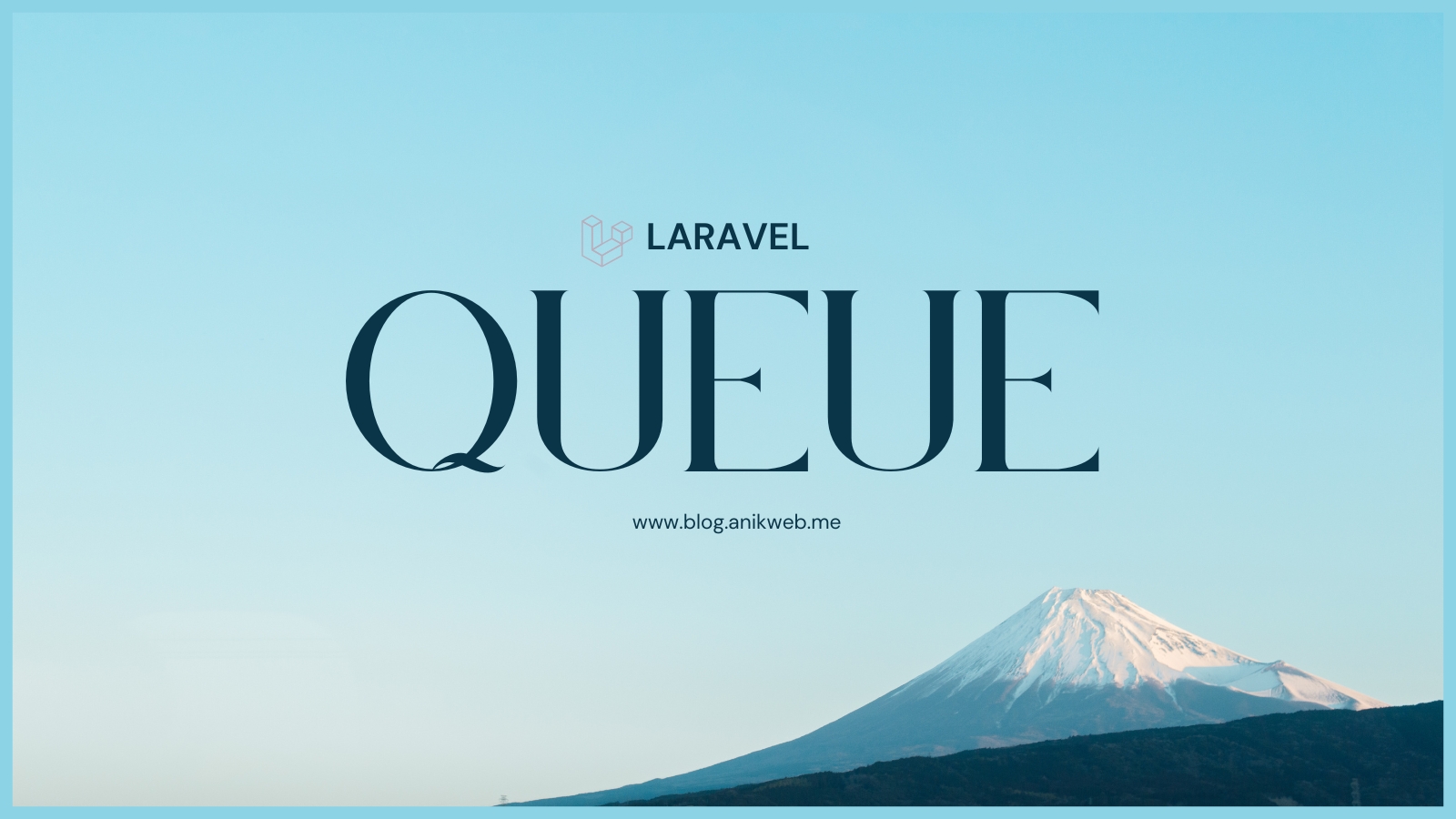
What is a Queue?
In a typical web application, some tasks, like sending an email or processing large amounts of data, can take time. If these tasks run directly within the request lifecycle, they can slow down the application and result in a poor user experience. Laravel Queues allow you to push these time-consuming tasks to the background, enabling them to run asynchronously and allowing the user to get a quicker response.
Laravel’s queue system supports several backends, including:
Database
Redis
Amazon SQS
Beanstalkd
Each of these backends stores jobs in a queue and manages the execution of these tasks.
Setting Up Laravel Queues
Step 1: Configure Queue Driver
In your .env
file, set the QUEUE_CONNECTION
QUEUE_CONNECTION=database
Next, update the config/queue.php
configuration file if needed, though the default settings for database
are usually sufficient.
Step 2: Creating Queue Tables
If you're using the database driver, run:
php artisan queue:table
php artisan migrate
Note: Laravel 11 creates the queue table by default, so check your database/migrations
directory. If you see the ......create_jobs_table.php
file, you can skip the php artisan queue:table
command. To check the migration status, run the php artisan migrate:status
command. If this table hasn't been migrated, just run the php artisan migrate
command.
Creating Jobs
To create a new job, use the make:job
Artisan command. For instance, let’s create a job that sends a welcome email to a new user.
php artisan make:job SendWelcomeEmail
This command creates a job class in the app/Jobs
directory. Open SendWelcomeEmail.php
to see the default structure. Here, you can define the logic for the job in the handle
method.
Example Job Class
Let's say we want to send a welcome email to a user when they register. We can define the SendWelcomeEmail
job like this:
namespace App\Jobs;
use App\Models\User;
use App\Mail\WelcomeEmail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
class SendWelcomeEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $user;
/**
* Create a new job instance.
*
* @return void
*/
public function __construct(User $user)
{
$this->user = $user;
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
Mail::to($this->user->email)->send(new WelcomeEmail($this->user));
}
}
In this example, the SendWelcomeEmail
job takes a User
object as a parameter and uses it to send an email. The ShouldQueue
interface tells Laravel to queue this job instead of running it immediately.
Dispatching the Job
To push this job onto the queue, you simply call the dispatch
method:
use App\Jobs\SendWelcomeEmail;
use App\Models\User;
$user = User::find(1);
SendWelcomeEmail::dispatch($user);
This will add the SendWelcomeEmail
job to the jobs
table in your database, waiting for a worker to pick it up and process it.
Running the Queue Worker
To start processing jobs on the queue, run the following command:
php artisan queue:work
This command will start a queue worker.
or you can also run:
php artisan queue:listen
When using the queue:listen
command, you don't have to manually restart the worker when you want to reload your updated code or reset the application state; however, this command is significantly less efficient than the queue:work
command:
To run the queue in a production environment, it is necessary to set up Supervisor or a similar worker on your server. Learn more about Supervisor:
Basic Example: Queueing a Job on User Registration
Let’s put everything together with a simple example. Suppose you want to send a welcome email whenever a new user registers. You could add this logic in your UserController
or a listener for the Registered
event.
namespace App\Http\Controllers;
use App\Models\User;
use App\Jobs\SendWelcomeEmail;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function register(Request $request)
{
// Validate and create the user
$user = User::create($request->all());
// Dispatch the welcome email job
SendWelcomeEmail::dispatch($user);
return response()->json(['message' => 'User registered and email sent!']);
}
}
This example demonstrates how Laravel queues allow you to defer sending a welcome email by pushing the job to a queue. As a result, the user receives a faster response after registration.
Handling Failed Jobs
Sometimes, jobs may fail due to network issues or other errors. Laravel provides an easy way to handle these failures. When a job fails, Laravel writes the failed job’s information to a failed_jobs
table.
php artisan queue:failed
If you want to retry all failed jobs, use:
php artisan queue:retry all
if you want to retry specific job
php artisan queue:retry {failed_job_ID}
If necessary, you may pass multiple IDs to the command:
php artisan queue:retry {failed_job_ID} {failed_job_ID}
Conclusion
Laravel Queues offer a powerful way to improve the responsiveness and scalability of your application by offloading time-consuming tasks. With a straightforward setup and robust error-handling features, Laravel queues allow you to easily process tasks asynchronously, enhancing the overall user experience.
Subscribe to my newsletter
Read articles from Anik Kumar Nandi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anik Kumar Nandi
Anik Kumar Nandi
Hi there! I'm Anik Kumar Nandi, passionate programmer, strongly focus on backend technologies. I specialize in PHP and Laravel, and I'm always exploring new ways to enhance the robustness and efficiency of my code. In addition to PHP and Laravel, my skill set includes RESTful API development, JavaScript, jQuery, Bootstrap, Ajax, and WordPress customization. Also deeply interested in AWS. Feel free to check out my GitHub profile and my portfolio site to learn more about my work and projects. Let's connect and create something amazing together!