MVC: The Superhero Trio of Web Development
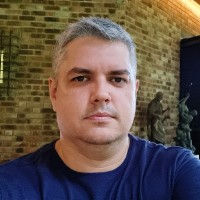
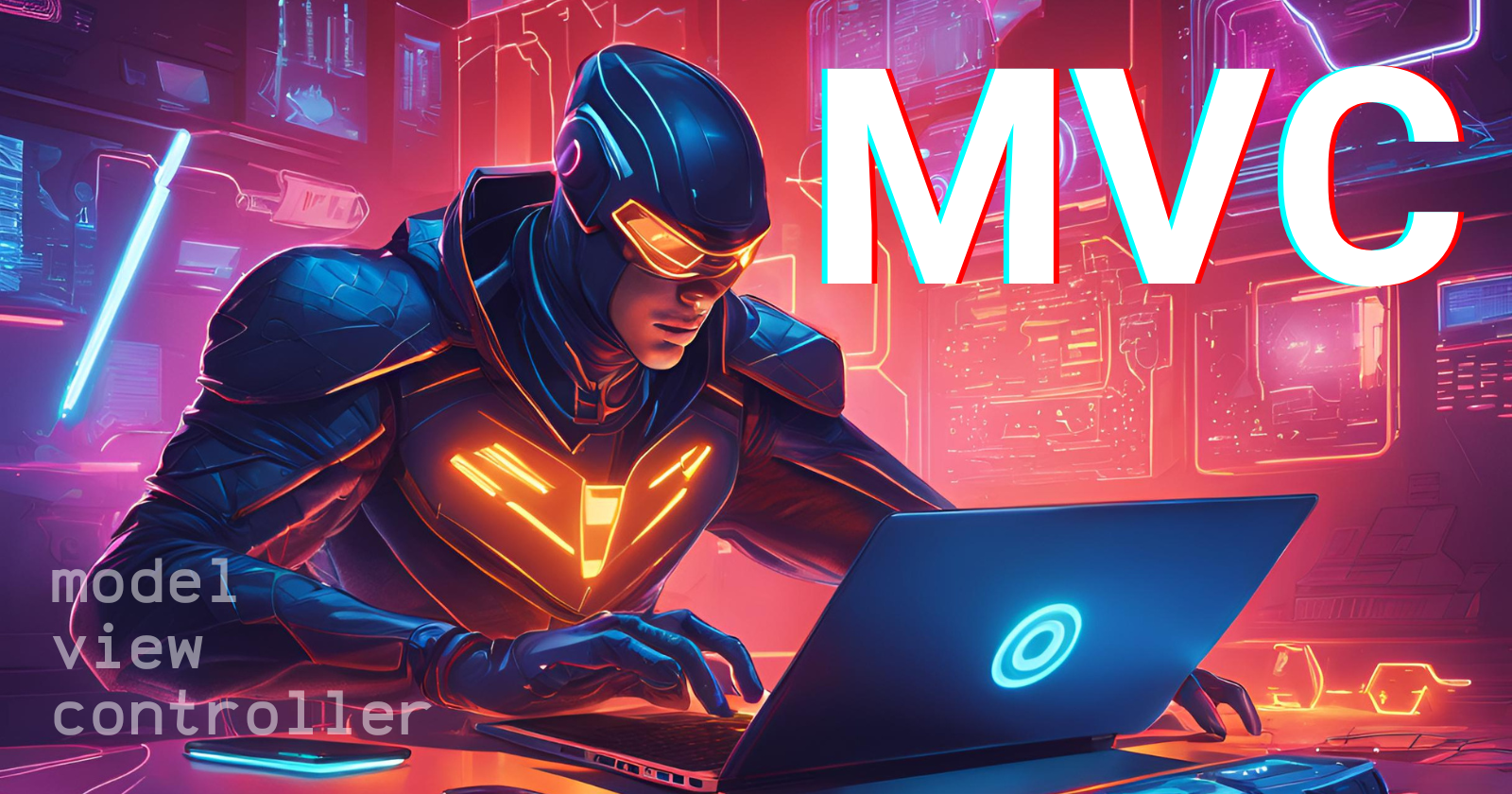
When building web apps, the MVC (Model-View-Controller) pattern provides a structured approach that enhances manageability, scalability, and collaboration. Models handle data, Views display information, and Controllers manage interactions, making development more organized. Widely adopted by frameworks like Laravel, Express.js, Django, and Ruby on Rails, MVC's separation of concerns allows for reusable code and efficient teamwork. The article explores MVC's benefits, practical applications, and provides resources to delve deeper into this architecture.
When it comes to building web apps, developers often look for structure – something like a good recipe to make sure everything runs smoothly. Enter the MVC pattern – the Batman, Superman, and Wonder Woman of web development. Together, they form a dynamic trio that saves us from messy, tangled code and disorganized projects.
But wait... what is MVC, you ask? Let me break it down for you in a way that even Alfred would approve of.
What is MVC?
MVC stands for Model-View-Controller, and it’s a popular architecture used to structure your web applications. Here’s how each part plays a role:
Model: The brain of the operation. The Model handles all the data and logic behind the scenes, like fetching data from the database or manipulating it.
View: This is the face of your app. The View displays the data (from the Model) and presents it to the user. It’s what the user interacts with, and it’s where you’d find your HTML, CSS, and JavaScript.
Controller: The Controller is the mediator, the bridge between the View and the Model. It takes user input, talks to the Model to get the data, and then decides what to show the View. It makes sure everything flows smoothly – like a really good personal assistant!
Think of MVC like a restaurant:
Model: The chef, who prepares your meal (data).
Controller: The waiter, who takes your order, tells the chef what to cook, and brings the meal to you.
View: The plate of delicious food served to you (what the user sees).
Why Should You Care About MVC?
You might wonder, "Why bother with MVC when I can just put everything in one place?" Well, here are the key reasons:
Separation of Concerns: MVC splits your app into three distinct parts. This makes your code easier to manage, scale, and debug. Want to change how your app looks? Edit the View. Want to tweak the logic? Adjust the Model.
Reusability: By separating logic from presentation, you can reuse code across different parts of your app. For example, the same Model can serve data to multiple Views.
Collaborative Superpower: In larger teams, designers can work on Views, while developers focus on Controllers and Models. This speeds up development and reduces chaos.
Languages and Frameworks That Love MVC
The MVC pattern is like that popular kid in high school – almost everyone uses it. Here are some languages and frameworks that make MVC their BFF:
PHP: Laravel (MVC masterclass!) and CodeIgniter both use MVC.
JavaScript: Express.js (in the Node.js world) is a big fan of this pattern, and even React’s architectural patterns can align with MVC principles.
Python: Django and Flask follow MVC patterns, though Django uses its own spin called MVT (Model-View-Template).
Ruby: Ruby on Rails is built around the MVC pattern.
Java: Spring Framework uses MVC to handle web applications.
Setting Up a Simple MVC Project
Let’s get into some code! Here’s a basic example of how an MVC structure would look in Node.js using Express.js.
Folder Structure:
Your folder structure will look something like this:├── models/ │ └── userModel.js ├── controllers/ │ └── userController.js ├── views/ │ └── userView.js ├── routes/ │ └── userRoutes.js └── app.js
Model (userModel.js): Handles the data – here’s where you might fetch users from a database.
const users = [ { id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Doe' } ]; module.exports = { getUsers: () => users };
Controller (userController.js): Handles the logic of your app.
const userModel = require('../models/userModel'); module.exports = { getUserData: (req, res) => { const users = userModel.getUsers(); res.json(users); } };
View (userView.js): This part typically involves HTML/CSS, but in a REST API, you can respond with JSON, like so:
const express = require('express'); const router = express.Router(); const userController = require('../controllers/userController'); router.get('/users', userController.getUserData); module.exports = router;
Router (userRoutes.js): The link between user input (URLs) and the controller.
const express = require('express'); const app = express(); const userRoutes = require('./routes/userRoutes'); app.use('/api', userRoutes); app.listen(3000, () => console.log('Server is running on port 3000'));
Perfect Projects for MVC
Now that you know the basics, what can you build with MVC? Here are a few project ideas:
To-Do List: A simple CRUD app where users can add, update, delete, and view tasks. The Controller handles the logic for each action, the Model handles the data, and the View shows the task list.
Blog Platform: A basic blog with posts stored in a database (Model), fetched via a Controller, and displayed on the site (View).
User Authentication: Build a login system where the Model manages users, the Controller handles login logic, and the View shows a form for entering credentials.
Resources to Learn More
Ready to dive into MVC? Here are some resources to help you get started:
Laravel Docs – For PHP lovers.
Express.js Guide – JavaScript fans, this one’s for you.
Django Tutorials – For Python enthusiasts.
Microsoft Learn – For a range of languages, including PHP, Python, and JavaScript.
Conclusion
Whether you’re a beginner or an experienced developer, the MVC architecture can make your life easier. It helps keep your projects organized, scalable, and easier to maintain. Plus, with its widespread use across various languages and frameworks, it’s a skill that will serve you well, no matter what tech stack you choose.
So, next time you’re starting a project, remember: there’s no need to play the lone wolf. MVC has your back, ready to fight off disorganized code and chaotic folder structures. Now go forth and build something awesome!
Image Credits
Photo by Rene Terp on Pexels
Photo by Andrea Piacquadio on Pexels
Photo by Terje Sollie on Pexels
Image by Polytropic Services
Subscribe to my newsletter
Read articles from Hugo Tavares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
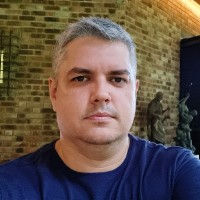
Hugo Tavares
Hugo Tavares
Hello World, my name is Hugo. I’m a Christian, husband, and father. I hold a degree in Administration and have experience in the military as an air traffic controller. Additionally, I’m a frontend developer, fascinated by technology, and constantly seeking to learn new things.