How to Install Nginx and Secure Your .NET Application with SSL Using Certbot
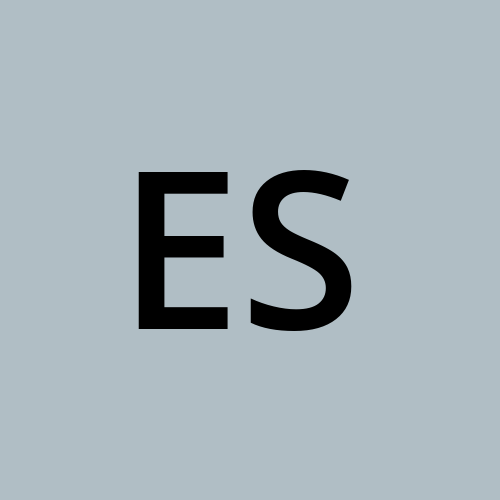
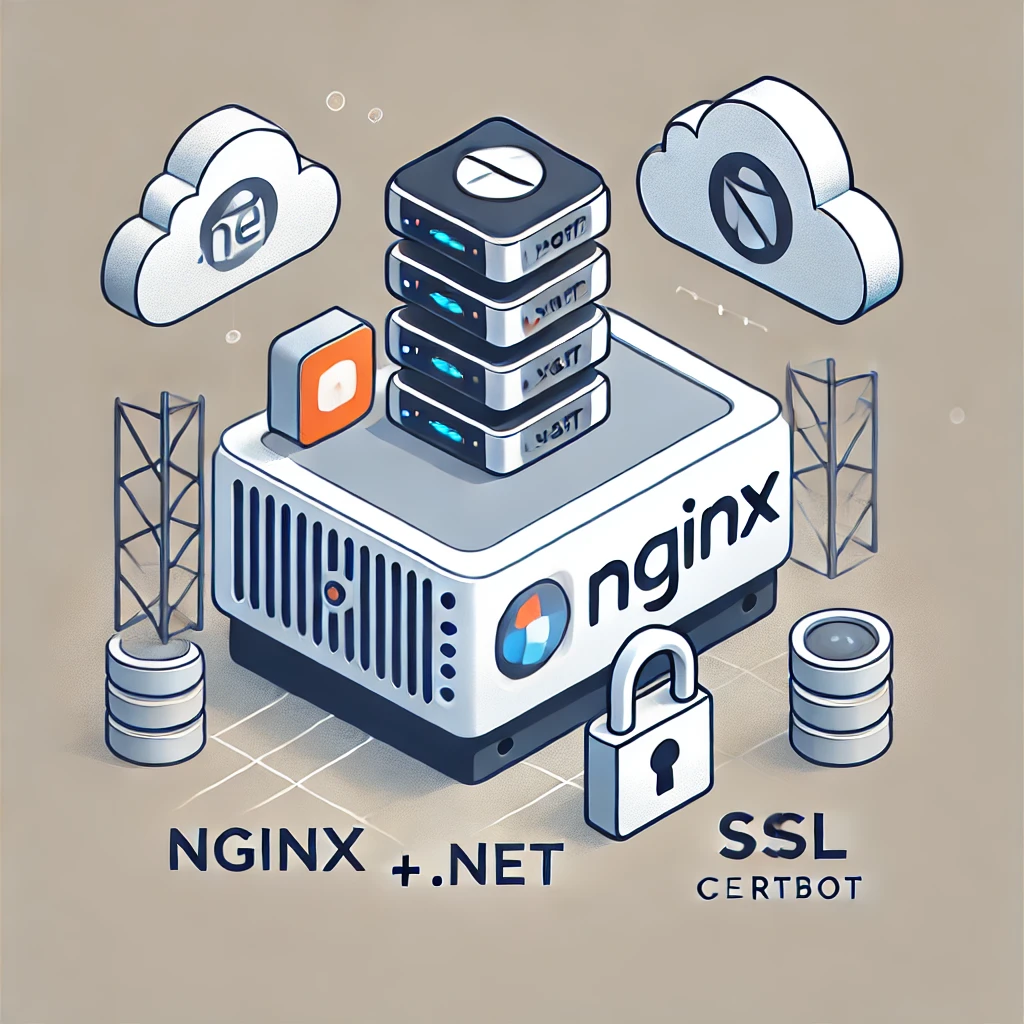
In this article, we’ll cover a comprehensive guide to setting up Nginx as a reverse proxy for a .NET application, running it over HTTP initially, and then adding SSL encryption with a free certificate from Let’s Encrypt using Certbot. We’ll also discuss automating certificate renewal to keep your site secure without manual intervention.
Prerequisites
Before we start, make sure you have:
A Linux server (Ubuntu or Debian is recommended).
A .NET application ready to be deployed.
Nginx installed on your server.
A registered domain name pointed to your server's IP address.
Why You Should Use Nginx for Your .NET Application and How to Set It Up with SSL
In today’s fast-paced digital landscape, delivering a reliable, secure, and efficient web experience is essential. That’s where Nginx comes in—a powerful web server and reverse proxy known for its high performance and flexibility. This article will discuss the key benefits of using Nginx for your .NET application, walk you through the setup process, and guide you on adding SSL encryption using Certbot to secure your site with HTTPS.
Why Use Nginx?
Nginx (pronounced "engine-x") has quickly become one of the most popular web servers worldwide, particularly favored for high-traffic websites and applications requiring exceptional performance. Here’s why Nginx is a valuable addition to your application stack:
1. High Performance and Speed
- Nginx’s event-driven architecture efficiently manages multiple concurrent connections, making it capable of handling large amounts of traffic without overloading the server. This results in faster response times, even under heavy load.
2. Reverse Proxy and Load Balancing
Nginx acts as a reverse proxy, sitting between clients and backend servers (such as your .NET application). It distributes incoming traffic across multiple servers, improving load handling and reducing the risk of downtime.
Load balancing with Nginx enhances both performance and reliability by routing requests to available servers, helping to maintain uptime even if one server fails.
3. Enhanced Security
- By serving as a barrier between users and backend servers, Nginx hides backend details, improving application security. Its ability to handle SSL/TLS makes it easy to secure traffic, protecting against eavesdropping and man-in-the-middle attacks.
4. Efficient Static Content Delivery
- Nginx is highly efficient at serving static files (e.g., images, CSS, JavaScript) from cache, reducing the load on backend servers and speeding up load times for end users.
5. Resource Efficiency
- Nginx’s lightweight resource usage makes it ideal for environments with limited resources or high-performance requirements, helping optimize server costs and efficiency.
6. SEO and Caching Support
- Nginx enables server-level caching, improving load times and user experience. Faster websites also benefit from improved SEO rankings, as search engines prioritize sites with quick load times.
7. Easy SSL/TLS Integration
- With tools like Certbot, you can set up free SSL certificates from Let’s Encrypt in minutes, making it easy to switch to HTTPS and meet modern security standards.
Here are the steps to follow for deploying Nginx:
Step 1: Install Nginx on Linux
Nginx will serve as the reverse proxy for your .NET application, handling requests and forwarding them to your application.
Update your package index:
sudo apt update
Install Nginx:
sudo apt install nginx -y
Enable and Start Nginx:
sudo systemctl enable nginx sudo systemctl start nginx
Verify the Installation: Check if Nginx is running by visiting your server's IP address in a browser (
http://your_server_ip
). You should see the Nginx welcome page if everything is set up correctly.
Step 2: Configure Nginx for Your .NET Application
Now, let's configure Nginx to serve your .NET application over HTTP.
Create a New Nginx Configuration File:
Open a new configuration file for your site:
sudo nano /etc/nginx/sites-available/myapp
Add Configuration for the Reverse Proxy:
Replace the contents of the file with the following configuration, adjusting
server_name
to your domain andproxy_pass
to the port your .NET app runs on (commonly 5000 for Kestrel):server { listen 80; server_name example.com www.example.com; location / { proxy_pass http://localhost:5000; # Use the port your .NET app is running on proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
Enable the Configuration:
Link this configuration file to the Nginx sites-enabled directory:
sudo ln -s /etc/nginx/sites-available/myapp /etc/nginx/sites-enabled/
Test and Reload Nginx:
Test your configuration for syntax errors, then reload Nginx to apply the changes:
sudo nginx -t sudo systemctl reload nginx
At this stage, your .NET application should be accessible via HTTP at http://your_domain
.
Step 3: Install Certbot and Obtain an SSL Certificate
Now that your site is accessible over HTTP, let’s secure it with SSL using Certbot and Let’s Encrypt.
Install Certbot:
Update your package list and install Certbot with the Nginx plugin:
sudo apt update sudo apt install certbot python3-certbot-nginx -y
Obtain an SSL Certificate:
Certbot will help automate the SSL configuration for Nginx.
sudo certbot --nginx
Follow the prompts to enter your email address and agree to the terms of service.
Certbot will detect your domain from the Nginx configuration and ask if you want to redirect all HTTP traffic to HTTPS. Choose to redirect, as this ensures all traffic is encrypted.
Verify SSL Configuration:
Once complete, Certbot will update your Nginx configuration to serve your .NET application securely over HTTPS. You can test this by visiting:
https://your_domain
You should see a padlock icon in the browser, confirming the SSL setup.
Step 4: Update Nginx Configuration (if needed)
If you want to customize your Nginx configuration after installing SSL, you might want to check or update the SSL settings in your Nginx configuration file located in
/etc/nginx/sites-available/myapp
:server { listen 80; server_name example.com www.example.com; # Redirect HTTP to HTTPS return 301 https://$host$request_uri; } server { listen 443 ssl; server_name example.com www.example.com; ssl_certificate /etc/letsencrypt/live/example.com/fullchain.pem; ssl_certificate_key /etc/letsencrypt/live/example.com/privkey.pem; location / { proxy_pass http://localhost:5000; # Change this if your app runs on a different port proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
Step 5: Test and Reload Nginx
After updating your configuration, always test it for syntax errors and then reload Nginx:
sudo nginx -t sudo systemctl reload nginx
Step 6: Automatic Renewal of SSL Certificates
Let's Encrypt certificates are valid for 90 days, but Certbot can automate the renewal process.
Test Automatic Renewal:
You can simulate a renewal test with the following command:
sudo certbot renew --dry-run
If you see no errors, automatic renewal is working correctly.
Check Cron Jobs (Optional):
Certbot is typically set up to run as a cron job automatically. You can check for a cron job by running:
sudo crontab -l
If you don't see an entry for Certbot, you can add one manually. For example, to run the renewal command every day at noon:
0 12 * * * certbot renew --quiet
Step 1: Open the Crontab File
You need to edit the crontab file for the root user since Certbot requires administrative privileges to run the renewal process. Use the following command:
sudo crontab -e
Step 2: Add the Cron Job
This will open the crontab file in a text editor (usually
nano
by default).Scroll to the bottom of the file and add the following line:
0 12 * * * certbot renew --quiet
This line schedules the renewal command to run every day at 12:00 PM (noon).
The
--quiet
option prevents output unless there are errors.
Step 3: Save and Exit
If you're using
nano
, save the changes by pressingCTRL + X
, thenY
, and thenEnter
.If you're using another editor, follow the respective steps to save and exit.
Step 4: Verify the Cron Job
You can verify that the cron job has been added by listing the cron jobs for the root user:
sudo crontab -l
This command will display all scheduled cron jobs for the root user, and you should see your new entry.
Additional Notes
Log Output (Optional): If you want to log the output of the cron job (for troubleshooting purposes), you can modify the cron job like this:
0 12 * * * certbot renew --quiet >> /var/log/certbot-renew.log 2>&1
This will append the output of the command to a log file located at
/var/log/certbot-renew.log
.Systemd Timer (Alternative): If your system uses
systemd
, you might already have a timer set up for Certbot. You can check if it’s active by running:systemctl list-timers
Look for
certbot.timer
in the output. This is an alternative to using cron jobs for managing scheduled tasks.
Conclusion
Congratulations! You’ve successfully set up Nginx to serve your .NET application, secured it with a Let’s Encrypt SSL certificate, and automated the renewal process with Certbot. By following this setup, you ensure that your .NET application remains secure and accessible over HTTPS.
If you have further questions or encounter issues, feel free to ask for more guidance. Happy hosting!
Subscribe to my newsletter
Read articles from Ejan Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
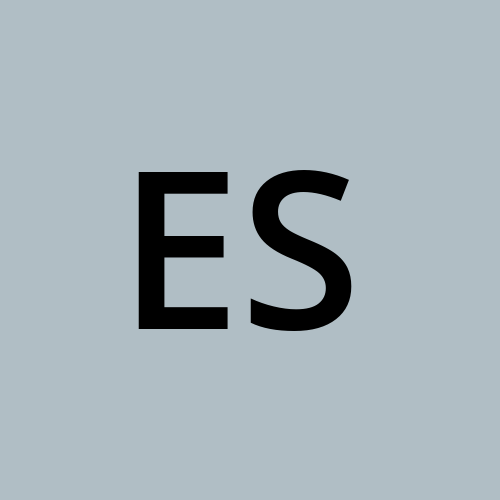