Function Frenzy: Advanced Functionality!
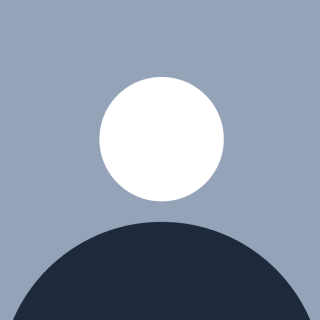
Table of contents
- Defining Functions and Understanding Scope
- Understanding Function Scope
- Flowchart: Function Execution Flow
- Higher-Order Functions and Callbacks
- Callbacks: Passing Functions as Arguments
- Powering the Function Factory
- Building a Function Library
- Advanced Callbacks and Function Composition
- Challenge Time — Build Your Own Function Library
- Additional Challenges for Mastery
- Conclusion — Powering Up with Functions
- Let’s explore Arrow Functions next!
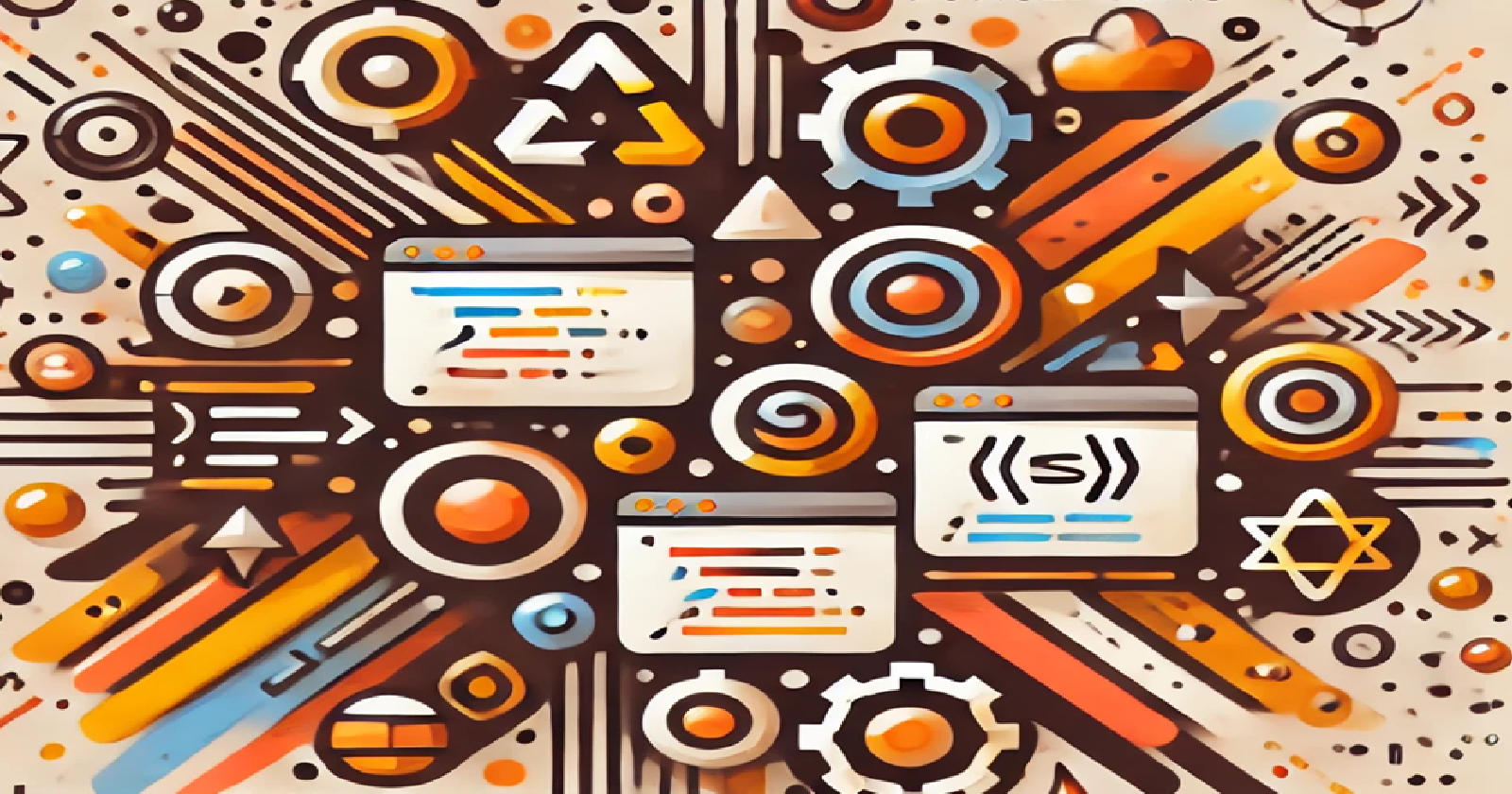
Imagine stepping into a bustling factory where every station produces a different product. Each station in this factory represents a function in JavaScript, meticulously crafting small tasks that contribute to the larger system. In programming, functions are reusable blocks of code that perform specific tasks, making them essential components in building dynamic and efficient applications.
In this article, we’ll explore everything from the basics of defining functions and understanding scope to more advanced concepts like higher-order functions and callbacks. By the end, you’ll have the tools you need to manage and organize your code efficiently, just like a well-oiled function factory.
Defining Functions and Understanding Scope
A function is a reusable block of code designed to perform a specific task. Functions allow you to break your code into smaller, manageable pieces that can be called and executed whenever needed.
In our factory, defining a function is like setting up a workstation that produces a specific product. You give it a name, specify its inputs, and describe what it should produce (output).
Basic Function Syntax
The most common way to define a function in JavaScript is using the function
keyword:
function productMaker(productName) {
console.log(productName + " has been made!");
}
In this simple example, the function productMaker
takes in a parameter (productName
) and prints a message to the console. When you call the function, it will perform this task, just like a workstation in our function factory producing a product.
Code Snippet: Function Definitions
Let’s walk through a more practical example. Imagine you run a toy factory, and each station is responsible for assembling different parts of the toy. Here’s how you’d define a function to create a toy:
function createToy(toyName, color) {
console.log("Creating a " + color + " " + toyName);
}
createToy("car", "red"); // Output: "Creating a red car"
createToy("doll", "blue"); // Output: "Creating a blue doll"
Explanation:
The
createToy
function takes two parameters:toyName
andcolor
.When the function is called with specific values, it outputs a custom message, like an assembly line producing toys.
Understanding Function Scope
In JavaScript, scope refers to the visibility of variables inside and outside of functions. Think of it as the boundary of a workstation in the factory: some tools are only available at a specific station (local scope), while others can be used anywhere in the factory (global scope).
Local Scope
Variables defined inside a function are local to that function and cannot be accessed outside of it. These are like tools that belong to a specific workstation in the factory.
function assemblePart() {
let part = "wheels"; // Local variable
console.log("Assembling " + part);
}
assemblePart(); // Output: "Assembling wheels"
console.log(part); // Error: part is not defined
In this example, the variable part
is only accessible inside the assemblePart
function. Trying to use it outside the function will result in an error.
Global Scope
Variables defined outside of functions are part of the global scope and can be accessed from anywhere in the program. These are like factory-wide tools that every workstation can use.
let factoryName = "Toy Factory"; // Global variable
function buildToy() {
console.log("Building at " + factoryName);
}
buildToy(); // Output: "Building at Toy Factory"
console.log(factoryName); // Output: "Toy Factory"
Here, factoryName
is a global variable that can be accessed both inside and outside of the buildToy
function.
Flowchart: Function Execution Flow
Let’s visualize how functions are called and executed, just like how products flow through a factory:
[ Start ]
|
[ Call Function ]
|
[ Pass Parameters ]
|
[ Execute Code Block ]
|
[ Return Result or Perform Action ]
|
[ End ]
This flowchart represents how a function takes in input (parameters), performs a task, and either returns a result or performs an action.
Higher-Order Functions and Callbacks
In our function factory, some workstations are more advanced—they can handle not just basic tasks but also process other functions as input. These are called higher-order functions, which take other functions as arguments or return functions as results. It’s like having a manager in the factory that oversees and coordinates other workstations.
Higher-Order Functions
A higher-order function is a function that takes one or more functions as arguments or returns a function. These are particularly powerful for creating flexible, reusable code.
Code Snippet: Using Higher-Order Functions
Let’s say you have multiple tasks in your factory, and you want to apply the same process to each one. You can create a higher-order function that takes a task (another function) and applies it:
function processTask(task) {
task(); // Execute the task function passed as an argument
}
function makeWheels() {
console.log("Wheels have been made.");
}
function paintCar() {
console.log("Car has been painted.");
}
processTask(makeWheels); // Output: "Wheels have been made."
processTask(paintCar); // Output: "Car has been painted."
Explanation:
processTask
is a higher-order function that takes another function (likemakeWheels
orpaintCar
) as an argument and executes it.
Callbacks: Passing Functions as Arguments
A callback is a function that is passed as an argument to another function and is executed after some operation. In the factory analogy, a callback is like telling one workstation to notify another when its job is done.
Code Snippet: Using Callbacks
Let’s use a callback function to notify the next station when a task is completed:
function notifyStation(task, callback) {
console.log(task + " has been completed.");
callback(); // Call the callback function
}
function packageProduct() {
console.log("Product has been packaged.");
}
notifyStation("Assembling", packageProduct); // Output: "Assembling has been completed." "Product has been packaged."
Explanation:
notifyStation
takes two arguments: a task and a callback function.After the task is completed, it executes the callback, signaling that the next task can begin.
Powering the Function Factory
In this part, we’ve explored how to define functions, understand scope, and use higher-order functions and callbacks to organize and manage code like a well-run factory. Functions are the core workstations in JavaScript that produce results, and higher-order functions add even more flexibility to how you coordinate tasks.
In the next part, we’ll dive deeper into building a Function Library, explore more advanced examples, and present you with some challenges to strengthen your understanding of functions in JavaScript.
Building a Function Library
Now that you’re familiar with defining functions and working with higher-order functions, let’s take things a step further by building a Function Library. In our Function Factory, this is like setting up a dedicated collection of stations, each with a specific task, all neatly organized for reuse.
A Function Library is simply a collection of related functions stored together, making them easy to call upon when needed. This is a powerful technique for organizing your code, especially as your applications grow larger.
Code Snippet: Creating a Function Library
Let’s create a small library of functions for a toy factory. Each function in this library will perform a specific task, such as making parts, assembling toys, or packaging them.
// Function Library
const toyFactory = {
makePart: function(part) {
console.log("Making " + part);
},
assembleToy: function(toy) {
console.log("Assembling " + toy);
},
packageToy: function(toy) {
console.log(toy + " has been packaged.");
}
};
// Using the library functions
toyFactory.makePart("wheels"); // Output: "Making wheels"
toyFactory.assembleToy("car"); // Output: "Assembling car"
toyFactory.packageToy("car"); // Output: "car has been packaged."
Explanation:
The
toyFactory
object acts as a library that contains different functions for making, assembling, and packaging toys.Each function is stored as a property of the object, making it easy to call on the appropriate function when needed.
This pattern is especially useful when you have a group of related functions that serve a specific purpose, allowing you to keep your code organized and modular.
Advanced Callbacks and Function Composition
Now that we’ve explored basic callbacks, let’s take it up a notch with function composition—the idea of combining multiple functions to perform complex operations. In a factory, this is like chaining together several workstations to complete a product. You can have one station make parts, another assemble them, and yet another package the final product, all in one seamless operation.
Code Snippet: Composing Functions with Callbacks
Let’s use function composition to chain several functions together, simulating a production line in the toy factory:
function makeWheels(callback) {
console.log("Wheels have been made.");
callback(); // Call the next function
}
function assembleCar(callback) {
console.log("Car has been assembled.");
callback(); // Call the next function
}
function packageCar() {
console.log("Car has been packaged.");
}
// Compose the functions to create a full production line
makeWheels(function() {
assembleCar(function() {
packageCar();
});
});
Explanation:
Each function performs a part of the process (making wheels, assembling the car, packaging the car).
Once a function completes its task, it calls the next function in line using a callback, creating a smooth production line.
Code Snippet: Function Composition with Higher-Order Functions
You can also use higher-order functions to create reusable processes. For example, let’s write a higher-order function that handles any task in the factory, applying a callback after each task:
function runTask(task, callback) {
console.log(task + " has been completed.");
callback();
}
function finalize() {
console.log("All tasks are completed. Factory is done for the day.");
}
// Compose multiple tasks using the higher-order function
runTask("Painting the car", function() {
runTask("Attaching the wheels", function() {
runTask("Packaging the toy", finalize);
});
});
Explanation:
The
runTask
function allows you to pass in any task as a string, execute it, and then call a callback function to continue to the next task.In this way, you can compose a sequence of tasks that flow together in a structured manner, ensuring the factory runs smoothly.
Challenge Time — Build Your Own Function Library
Now it’s time to put what you’ve learned into practice. In this challenge, you’ll build a simple function library that manages different types of operations. Imagine you’re running a software company, and each function in your library handles a different task for a project.
Challenge: Create a Software Project Function Library
Create a library with functions that handle tasks such as
writeCode
,testCode
, anddeployProject
.Use function composition to call each function in sequence.
Use callbacks to ensure each task is completed before moving on to the next.
Here’s a starter template:
const projectLibrary = {
writeCode: function(language, callback) {
console.log("Writing code in " + language);
callback();
},
testCode: function(callback) {
console.log("Testing code...");
callback();
},
deployProject: function() {
console.log("Project has been deployed.");
}
};
// Function composition to run the project workflow
projectLibrary.writeCode("JavaScript", function() {
projectLibrary.testCode(function() {
projectLibrary.deployProject();
});
});
Additional Challenges for Mastery
Here are more challenges to deepen your understanding of functions:
Challenge 1: Create a Math Operations Library
Scenario: Build a library of math functions (add
, subtract
, multiply
, divide
) and use function composition to perform a series of calculations.
Example:
const mathLibrary = {
add: function(a, b, callback) {
let result = a + b;
console.log("Addition result: " + result);
callback(result);
},
multiply: function(a, b, callback) {
let result = a * b;
console.log("Multiplication result: " + result);
callback(result);
}
};
mathLibrary.add(5, 3, function(result) {
mathLibrary.multiply(result, 2, function(finalResult) {
console.log("Final result: " + finalResult);
});
});
Challenge 2: Manage a To-Do List
Scenario: Create a function library to manage a to-do list. Functions should include addTask
, removeTask
, and viewTasks
. Use callbacks to notify when tasks are completed or removed.
Example:
const todoLibrary = {
tasks: [],
addTask: function(task, callback) {
this.tasks.push(task);
console.log("Task added: " + task);
callback();
},
removeTask: function(task, callback) {
this.tasks = this.tasks.filter(t => t !== task);
console.log("Task removed: " + task);
callback();
},
viewTasks: function() {
console.log("Current tasks: " + this.tasks.join(", "));
}
};
todoLibrary.addTask("Buy groceries", function() {
todoLibrary.viewTasks();
todoLibrary.removeTask("Buy groceries", function() {
todoLibrary.viewTasks();
});
});
Conclusion — Powering Up with Functions
In this article, we’ve taken a deep dive into the world of functions, learning how to define them, control their scope, and use higher-order functions and callbacks to manage complex workflows. Functions are the heart of your JavaScript applications, allowing you to break down tasks, organize code, and create reusable components that make your programs more dynamic and efficient.
By mastering functions, you gain the ability to manage complex processes, whether you’re building a function library, handling asynchronous tasks, or composing functions to work in sequence.
Let’s explore Arrow Functions next!
In the next article, we’ll dive into Arrow Functions, a more concise way to define functions in JavaScript that comes with some powerful benefits. Get ready for the next chapter of Function Frenzy!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by