Boto3 Module in a Nutshell
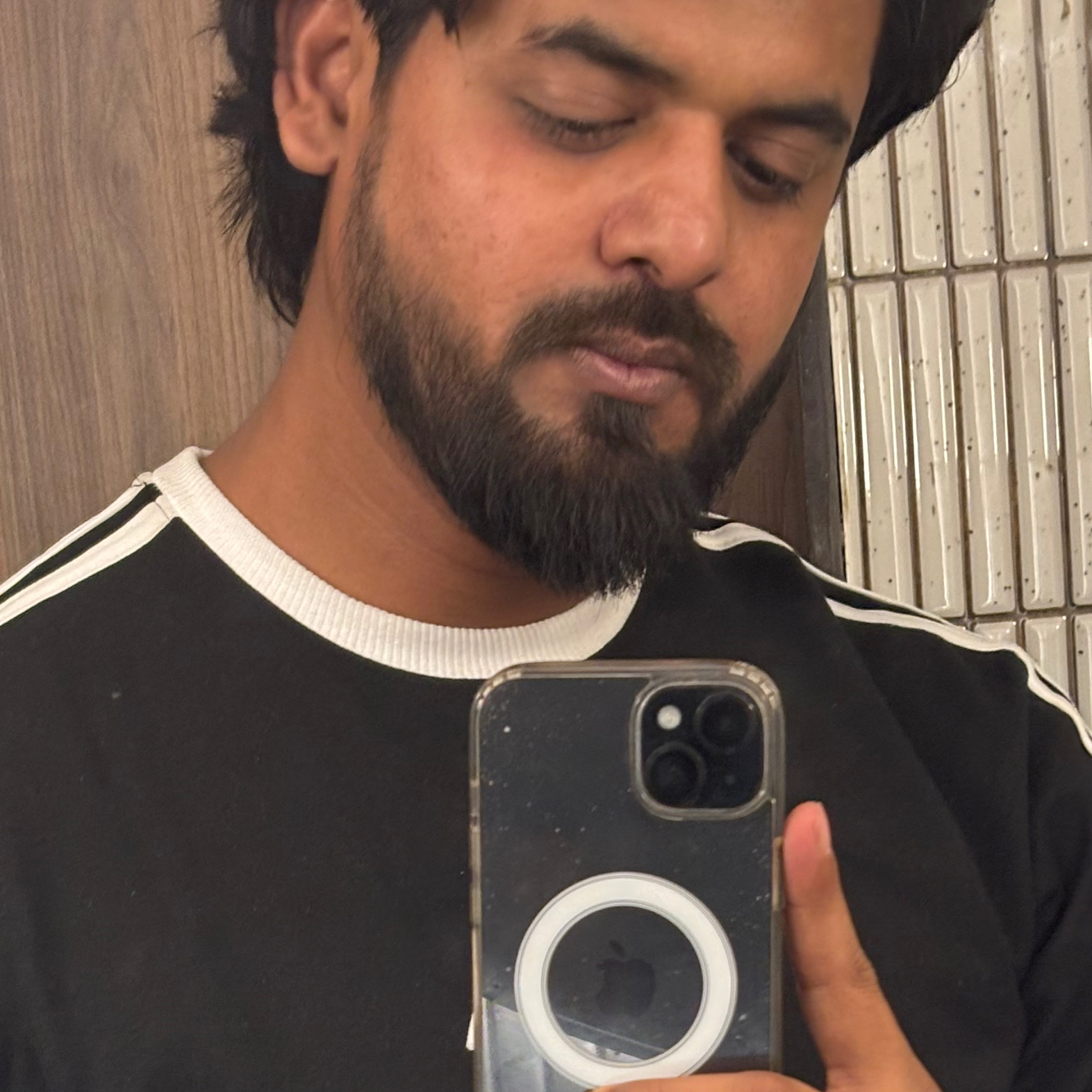
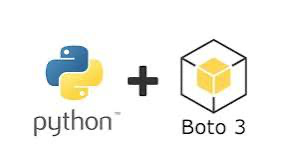
In the world of Automation Why IAC plays an important role lets have brief overview on one such AWS SDK module known as Boto3 and how we can leverage it to automate infrastructure provisioning 😇
Boto3 is the official Python library for working with Amazon Web Services (AWS). With Boto3, you can easily set up, manage, and control AWS services like S3, EC2, and DynamoDB all from within your Python code. It simplifies AWS tasks by providing a more Python way of working with the AWS API.
Setting Up Boto3
To get started with Boto3, you will need to install it and configure your AWS credentials.
1. Install Boto3: Run the following command in your terminal:
pip install boto3
2. Set Up AWS Credentials: Configure your AWS access by running:
aws configure
You will be prompted to enter your AWS Access Key ID AWS Secret Access Key and default region name.
Example 1: Uploading a File to S3
One of the common things people use Boto3 for is uploading files to Amazon S3 AWS’s cloud storage service. Here is a simple example of how to upload a file to an S3 bucket.
import boto3
s3 = boto3.client('s3')
bucket_name = 'follow-vickonfire'
file_path = 'path/to/your/infinity.txt'
object_name = 'infinity-file.txt'
try:
s3.upload_file(file_path, bucket_name, object_name)
print("File uploaded successfully.")
except Exception as e:
print(f"Error uploading file: {e}")
Here is a quick breakdown:
• boto3.client('s3') initializes a connection to S3.
• upload_file() is a Boto3 method that uploads your local file (file_path) to the specified S3 bucket under the name object_name.
Example 2: Starting an EC2 Instance
Boto3 also makes it easy to manage EC2 instances. Here’s how you can start an EC2 instance with a given instance ID:
import boto3
ec2 = boto3.client('ec2')
instance_id = 'i-1234567890abcdef0'
try:
ec2.start_instances(InstanceIds=[instance_id])
print("Instance started successfully.")
except Exception as e:
print(f"Error starting instance: {e}")
In this example:
• boto3.client('ec2') creates a connection to the EC2 service.
• start_instances() takes a list of instance IDs and starts them.
Summary:
Boto3 is an essential tool for Python developers working with AWS. It streamlines interactions with AWS services by converting complex API requests into easy-to-use Python functions. Whether you’re managing storage with S3, handling compute resources with EC2, or using other AWS services, Boto3 gives you the flexibility to handle everything from within your Python code.
Subscribe to my newsletter
Read articles from Vikas Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
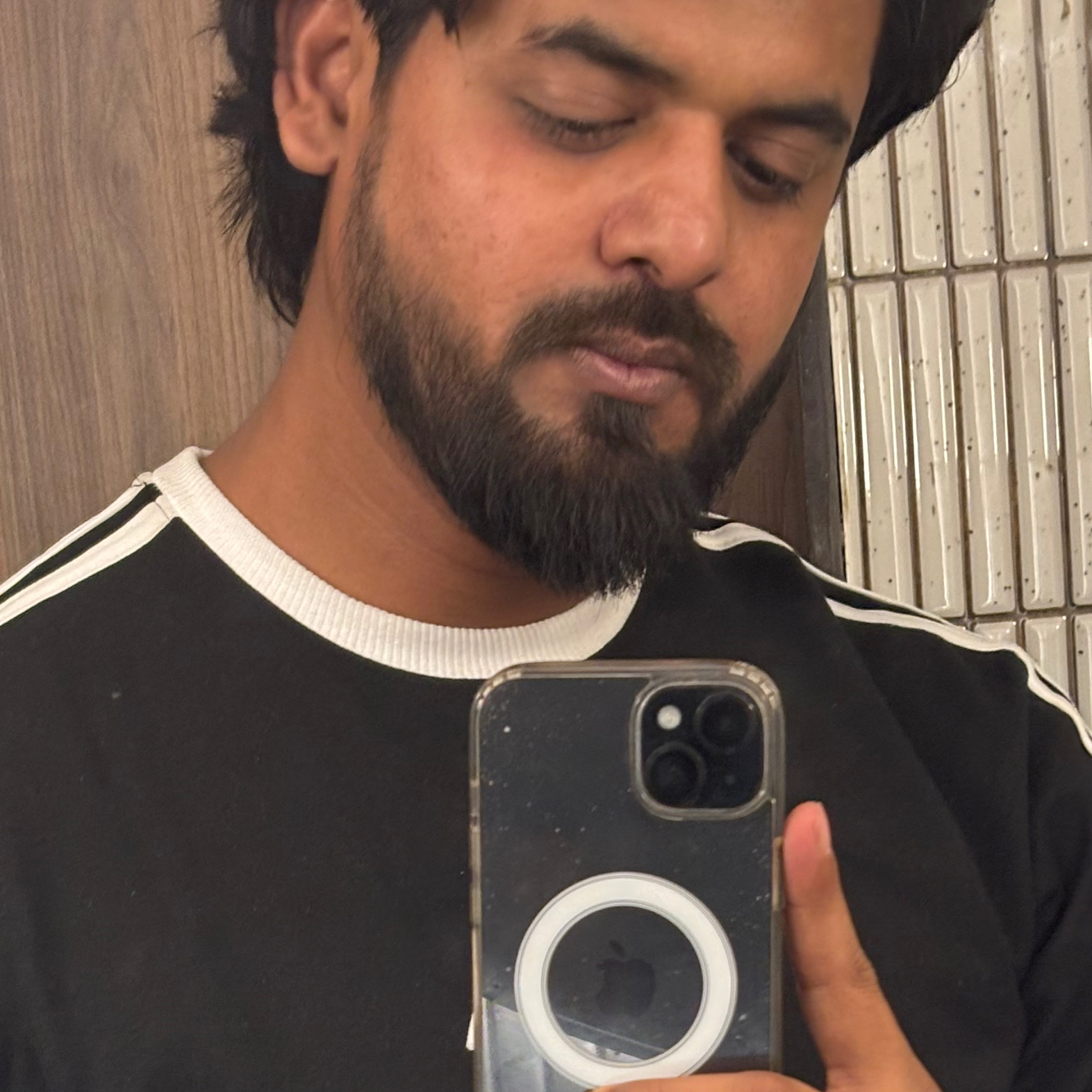
Vikas Dubey
Vikas Dubey
Cloud Solution Architect, can drag you out of problem