Multithreading in java

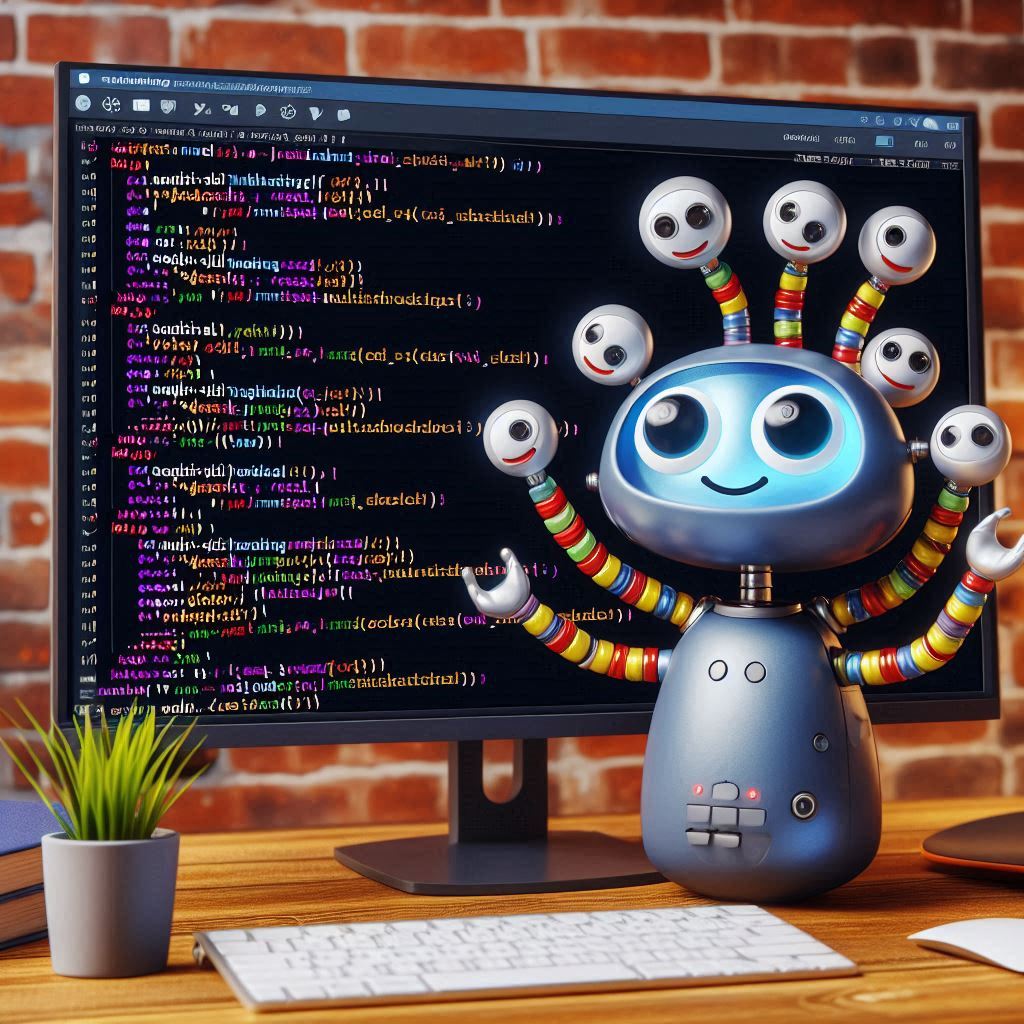
Understanding Multithreading in Java
Java is renowned for its robustness and versatility, and one of its standout features is the ability to handle multiple tasks simultaneously through multithreading. In this blog post, we'll delve deep into the concept of multithreading and walk through a practical example to illustrate how it works.
What is Multithreading?
Multithreading is a feature that allows concurrent execution of two or more parts of a program, which are referred to as threads. This ability is essential for tasks that can be executed in parallel, thereby improving the efficiency and performance of applications.
Key Concepts:
Thread: The smallest unit of a process, which has its own path of execution.
Runnable Interface: A functional interface that defines a single method,
run()
, which contains the code to be executed by the thread.Thread Class: A class that provides constructors and methods to create and perform operations on threads.
Benefits of Multithreading:
Better Utilization of CPU: Multithreading makes efficient use of the CPU by performing multiple tasks simultaneously.
Improved Performance: Applications can be more responsive as different parts of the program are executed independently.
Resource Sharing: Threads within the same process share the same memory and resources, reducing the overhead compared to multiple processes.
Practical Example:
Let's explore a practical example to understand how multithreading works in Java. We'll create two threads: one by extending the Thread
class and another by implementing the Runnable
interface.
MyThread Class (Extending Thread)
package Multithreading_injava;
public class Mythread extends Thread {
public Mythread() {
this.setName("MyThread");
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
System.out.println(this.getName() + "#" + i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println(this.getName() + " was interrupted");
return; // Exit the run method if interrupted
}
}
System.out.println(this.getName() + " is executed");
}
}
In this class, we extend the Thread
class and override its run()
method. The run()
method contains a loop that prints numbers from 0 to 9, with a one-second pause between each print.
MyRunnable Class (Implementing Runnable)
package Multithreading_injava;
public class MyRunnable implements Runnable {
@Override
public void run() {
for (int i = 10; i > 0; i--) {
System.out.println("Countdown Thread: " + i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// Log the exception and restore the interrupted status
System.err.println("Thread was interrupted: " + e.getMessage());
Thread.currentThread().interrupt();
}
}
System.out.println("Countdown Thread: Ended");
}
}
In this class, we implement the Runnable
interface and override its run()
method. The run()
method contains a loop that counts down from 10 to 1, with a one-second pause between each print.
Main Class
package Multithreading_injava;
public class Main {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyRunnable runnable = new MyRunnable();
Thread thread2 = new Thread(runnable);
thread1.start();
try {
thread1.join(); // Wait for thread1 to finish
} catch (InterruptedException e) {
System.err.println("Thread was interrupted: " + e.getMessage());
Thread.currentThread().interrupt(); // Restore interrupted status
}
thread2.start();
// Check if thread2 is alive and thread1 is not alive
if (thread2.isAlive() && !thread1.isAlive()) {
System.out.println("rocket launched");
}
}
}
In the Main
class, we create instances of Mythread
and Myrunnable
, and then start both threads. The start()
method initiates the run()
method of each thread, allowing them to execute concurrently.
In above code i used “rocket launched” for making it fun…as used countdown example.
Conclusion
Multithreading in Java enables efficient execution of multiple tasks by leveraging the power of concurrency. By understanding and implementing threads through the Thread
class and the Runnable
interface, developers can create responsive and high-performing applications.
Feel free to experiment with the code above and see the magic of multithreading in action! If you have any questions or run into issues, leave a comment below. Happy coding!
By diving into this example, I hope you gain a better understanding of how multithreading works in Java. It's a powerful tool that, when used correctly, can greatly enhance the performance and responsiveness of your applications. 🌟🚀
Subscribe to my newsletter
Read articles from Rohan Ghosh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohan Ghosh
Rohan Ghosh
👨💻 About Me I'm a Computer Science undergrad with a passion for low-level development and full-stack projects in Java and the MERN stack. I love diving deep into system-level programming, having built projects like a Virtual Machine and a Garbage Collector in C. 🎓 Certifications Certified in Machine Learning by Stanford University, which has honed my skills in data analysis and predictive modeling. 🌐 Community & Open Source Avid contributor to tech communities and open-source projects. I enjoy collaborating, sharing knowledge, and learning from fellow developers. Let's connect and create something amazing together!