Understanding PHP Garbage Collection: From Basics to Advanced Optimization

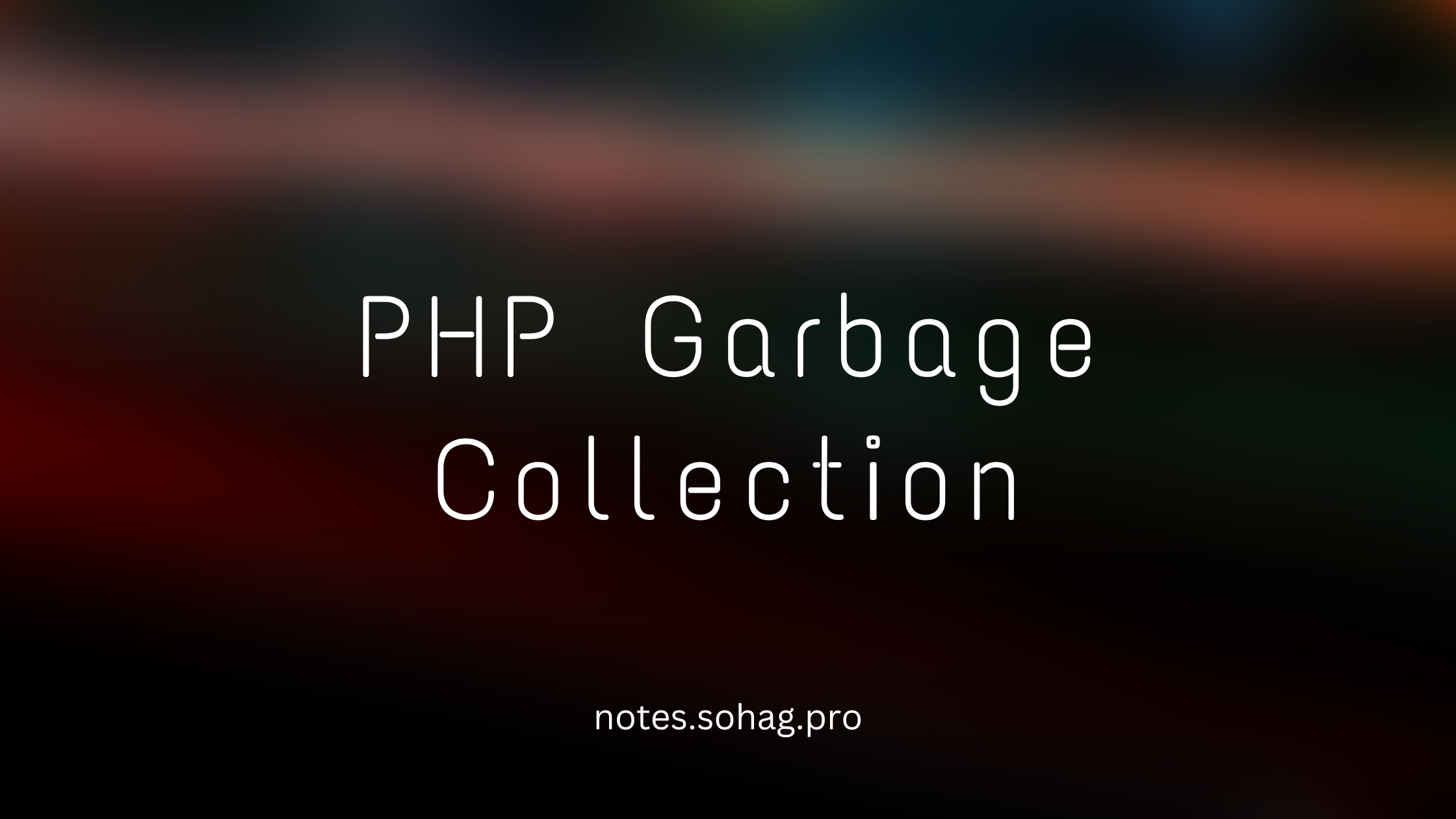
PHP Garbage Collection
Have you ever wondered what happens to the leftovers from your dinner party? Just like we clean up after a gathering, PHP needs to clean up unused variables and objects from memory. This process is called Garbage Collection (GC), and today we'll dive deep into how it works in PHP.
Understanding Garbage Collection
Imagine your kitchen counter space as computer memory. When cooking (running your program), you use various ingredients (variables and objects). Once you're done using an ingredient, you put it back in storage or dispose of it to free up counter space. This is exactly what garbage collection does in PHP - it identifies and removes data that's no longer needed.
Real-life Example
Think of a restaurant kitchen during busy hours:
Chefs (PHP scripts) use ingredients (variables)
Used utensils (objects) need cleaning
Kitchen porter (garbage collector) ensures cleanliness
Limited counter space (memory) needs efficient management
How PHP Memory Management Works
// Creating variables (like taking ingredients out)
$recipe = "Chocolate Cake";
$ingredients = ["flour", "sugar", "eggs"];
// Variables go out of scope (like putting ingredients away)
function makeRecipe() {
$temperature = 180; // Only exists within this function
// $temperature is automatically cleaned up after function ends
}
Reference Counting
PHP uses a reference counting mechanism. Think of it like a library book checkout system:
Each variable has a counter (like a book's checkout card)
When code references the variable, the counter increases
When references are removed, the counter decreases
When counter reaches zero, memory is freed
// Example of reference counting
$book = "PHP Advanced"; // Reference count: 1
$anotherBook = &$book; // Reference count: 2
unset($anotherBook); // Reference count: 1
unset($book); // Reference count: 0, memory can be freed
Circular References
Sometimes we create circular references, like a circular waiting list at a restaurant:
class Restaurant {
public $nextRestaurant;
}
$restaurant1 = new Restaurant();
$restaurant2 = new Restaurant();
// Creating a circular reference
$restaurant1->nextRestaurant = $restaurant2;
$restaurant2->nextRestaurant = $restaurant1;
// Even after unsetting, memory isn't immediately freed
unset($restaurant1);
unset($restaurant2);
Garbage Collection Algorithms
PHP uses a cycle-collecting garbage collector. Here's how it works:
- Collection Cycles
// Manually trigger collection (usually not recommended)
gc_collect_cycles();
// Check garbage collection status
var_dump(gc_status());
- Customizing Collection
// Disable garbage collection
gc_disable();
// Enable garbage collection
gc_enable();
// Check if garbage collection is enabled
var_dump(gc_enabled());
Real-life Example
Think of a busy mall parking lot:
Cars (objects) come and go
Some spots (memory) become available
The parking system (GC) needs to track available spots
During quiet times, thorough cleanup can happen
Best Practices
- Explicit Cleanup
// Good practice: Clean up large objects when done
$largeData = loadBigDataset();
processData($largeData);
$largeData = null; // Explicitly release
- Avoid Circular References
// Instead of circular references, consider using weak references
WeakReference::create($object);
- Memory Monitoring
// Monitor memory usage
$memoryBefore = memory_get_usage();
// Your code here
$memoryAfter = memory_get_usage();
$difference = $memoryAfter - $memoryBefore;
Topics to Explore Further
Zend Memory Manager
PHP OpCache
Memory Leaks Detection
WeakRef and WeakMap
Performance Profiling
PHP-FPM Configuration
Practical Examples
E-commerce Cart System
class ShoppingCart {
private $items = [];
public function addItem($item) {
$this->items[] = $item;
}
public function __destruct() {
// Cleanup cart items
$this->items = [];
}
}
Session Management
// Session handling with proper cleanup
session_start();
$_SESSION['user'] = 'John';
// ... application logic
session_destroy(); // Proper cleanup
Conclusion
Understanding garbage collection in PHP is like managing a well-run kitchen - it's all about efficiency and cleanliness. By following best practices and understanding how PHP manages memory, you can write more efficient and reliable applications.
Remember:
Clean up after yourself when possible
Avoid circular references
Monitor memory usage in critical applications
Use built-in PHP functions to help manage garbage collection
Keep exploring and experimenting with these concepts. The more you understand about memory management, the better developer you'll become!
Did you find this article helpful? Share your thoughts and experiences with memory management in PHP in the comments below!
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author